慣用的 Goroutine 終止和錯誤處理
在 Go 並發領域,無縫處理 Goroutine 的終止和錯誤至關重要。考慮一個簡單的用例,我們的目標是同時從多個遠端伺服器檢索數據,並傳回第一個遇到的錯誤。
洩漏Goroutines
最初的嘗試可能涉及洩漏Goroutine,如下所示程式碼片段:
func fetchAll() error { wg := sync.WaitGroup{} errs := make(chan error) leaks := make(map[int]struct{}) //... }
要解決這個問題,我們可以使用上下文或使用errgroup 套件:
錯誤群組解決方案
errgroup包提供了一種便捷的方法管理 goroutine 終止和錯誤。它會自動等待所有提供的 goroutine 完成,或在出現任何錯誤時取消剩餘的 goroutine。
func fetchAll(ctx context.Context) error { errs, ctx := errgroup.WithContext(ctx) //... return errs.Wait() }
這段程式碼優雅地處理 goroutine 終止並回傳遇到的第一個錯誤。
以上是如何優雅地終止 Go 中的 Goroutine 並處理錯誤?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
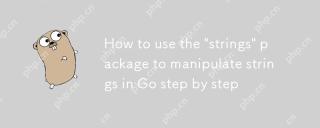
Go的strings包提供了多種字符串操作功能。 1)使用strings.Contains檢查子字符串。 2)用strings.Split將字符串分割成子字符串切片。 3)通過strings.Join合併字符串。 4)用strings.TrimSpace或strings.Trim去除字符串首尾的空白或指定字符。 5)用strings.ReplaceAll替換所有指定子字符串。 6)使用strings.HasPrefix或strings.HasSuffix檢查字符串的前綴或後綴。
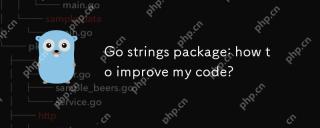
使用Go語言的strings包可以提升代碼質量。 1)使用strings.Join()優雅地連接字符串數組,避免性能開銷。 2)結合strings.Split()和strings.Contains()處理文本,注意大小寫敏感問題。 3)避免濫用strings.Replace(),考慮使用正則表達式進行大量替換。 4)使用strings.Builder提高頻繁拼接字符串的性能。
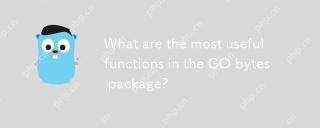
Go的bytes包提供了多種實用的函數來處理字節切片。 1.bytes.Contains用於檢查字節切片是否包含特定序列。 2.bytes.Split用於將字節切片分割成smallerpieces。 3.bytes.Join用於將多個字節切片連接成一個。 4.bytes.TrimSpace用於去除字節切片的前後空白。 5.bytes.Equal用於比較兩個字節切片是否相等。 6.bytes.Index用於查找子切片在largerslice中的起始索引。
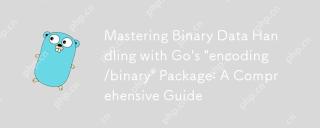
theEncoding/binarypackageingoisesenebecapeitProvidesAstandArdArdArdArdArdArdArdArdAndWriteBinaryData,確保Cross-cross-platformCompatibilitiational and handhandlingdifferentendenness.itoffersfunctionslikeread,寫下,寫,dearte,readuvarint,andwriteuvarint,andWriteuvarIntforPreciseControloverBinary
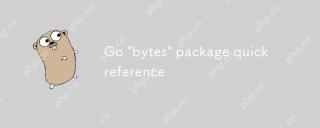
回顧bytespackageingoiscialforhandlingbytesliceSandBuffers,offeringToolsforeffitedMemoryManagement和datamanipulation.1)itprovidesfunctionalitiesLikeCreatingBuffers,比較,搜索/更換/reportacingwithinslices.2)forlargedatAsetSets.n
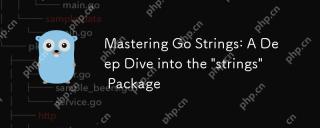
你應該關心Go語言中的"strings"包,因為它提供了處理文本數據的工具,從基本的字符串拼接到高級的正則表達式匹配。 1)"strings"包提供了高效的字符串操作,如Join函數用於拼接字符串,避免性能問題。 2)它包含高級功能,如ContainsAny函數,用於檢查字符串是否包含特定字符集。 3)Replace函數用於替換字符串中的子串,需注意替換順序和大小寫敏感性。 4)Split函數可以根據分隔符拆分字符串,常用於正則表達式處理。 5)使用時需考慮性能,如
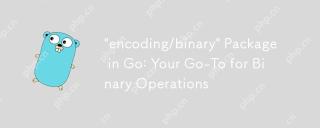
“編碼/二進制”軟件包interingoisentialForHandlingBinaryData,oferingToolSforreDingingAndWritingBinaryDataEfficely.1)Itsupportsbothlittle-endianandBig-endianBig-endianbyteorders,CompialforOss-System-System-System-compatibility.2)
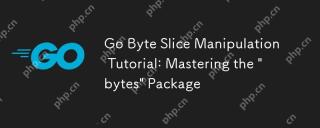
掌握Go語言中的bytes包有助於提高代碼的效率和優雅性。 1)bytes包對於解析二進制數據、處理網絡協議和內存管理至關重要。 2)使用bytes.Buffer可以逐步構建字節切片。 3)bytes包提供了搜索、替換和分割字節切片的功能。 4)bytes.Reader類型適用於從字節切片讀取數據,特別是在I/O操作中。 5)bytes包與Go的垃圾回收器協同工作,提高了大數據處理的效率。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
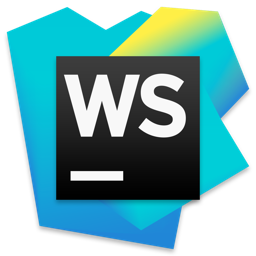
WebStorm Mac版
好用的JavaScript開發工具
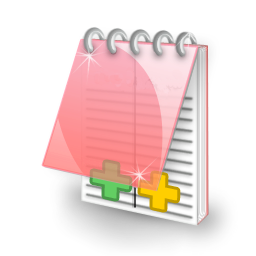
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
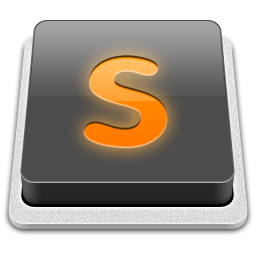
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Atom編輯器mac版下載
最受歡迎的的開源編輯器