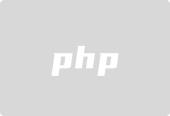
在 PHP 中從陣列中刪除特定元素
在 PHP 中管理陣列對於組織和操作資料至關重要。一項常見任務是從陣列中刪除特定元素。假設您有一個包含水果等項目的數組,並且使用者選擇從清單中刪除特定水果,例如“草莓”。
解決方案:
要在知道其值時從數組中刪除元素,可以使用array_search 和unset函數:
說明:
- **array_search('strawberry', $array)**:此函數在$array 中搜尋值'strawberry',如果找到則傳回其鍵索引。
-
unset($array[$key]):如果鍵不是false,則unset 會從陣列中刪除該鍵索引處的元素,從而有效地從清單中刪除「草莓」 .
多次出現:
如果同一個元素多次出現,可以使用array_keys檢索鍵所有實例:
在此範例中,將從陣列中刪除所有出現的「草莓」。
其他資源:
- [array_search](https://www.php.net/manual/en/function.array-search.php)
- [取消設定](https://www.php.net/manual /en/function.unset.php)
- [array_keys](https://www.php.net /manual/en/function.array-keys.php)
以上是如何從 PHP 陣列中刪除特定元素?的詳細內容。更多資訊請關注PHP中文網其他相關文章!