將大檔案上傳到雲端可能具有挑戰性 - 網路中斷、瀏覽器限制和龐大的檔案大小很容易破壞這個過程。 Amazon S3(簡單儲存服務)是一種可擴展、高速、基於 Web 的雲端儲存服務,專為資料和應用程式的線上備份和歸檔而設計。然而,將大檔案上傳到 S3 需要小心處理,以確保可靠性和效能。
AWS S3 的分段上傳:這是一個強大的解決方案,可以將大檔案分成更小的區塊,透過獨立處理每個部分甚至並行上傳部分來實現更快、更可靠的上傳。這種方法不僅克服了檔案大小限制(S3 需要對大於 5GB 的檔案進行分段上傳),而且還最大限度地降低了失敗的風險,使其非常適合需要無縫、穩健的檔案上傳的應用程式。
在本指南中,我們將詳細介紹客戶端分段上傳到 S3 的細節,向您展示為什麼它是處理大文件的明智選擇、如何安全地啟動和運行它以及需要注意哪些挑戰出去。我將提供逐步說明、程式碼範例和最佳實踐,以幫助您實現可靠的客戶端文件上傳解決方案。
準備好升級您的檔案上傳體驗了嗎?讓我們開始吧!
伺服器與客戶端上傳
設計檔案上傳系統時,您有兩個主要選擇:透過伺服器上傳檔案(伺服器端)或直接從客戶端上傳檔案到 S3(客戶端)。每種方法都有其優點和缺點。
伺服器端上傳
優點:
增強的安全性:所有上傳均由伺服器管理,確保 AWS 憑證的安全性。
更好的錯誤處理:伺服器可以更穩健地管理重試、日誌記錄和錯誤處理。
集中處理:檔案可以在儲存到 S3 之前在伺服器上進行驗證、處理或轉換。
缺點:
更高的伺服器負載:大量上傳會消耗伺服器資源(CPU、記憶體、頻寬),這會影響效能並增加營運成本。
潛在瓶頸:在高上傳流量期間,伺服器可能成為單點故障或效能瓶頸,導致上傳緩慢或停機。
成本增加:在伺服器端處理上傳可能需要擴展基礎設施以處理尖峰負載,從而增加營運費用。
客戶端上傳
優點:
減少伺服器負載:檔案直接從使用者裝置傳送到 S3,釋放伺服器資源。
速度提高:由於繞過應用程式伺服器,使用者體驗更快的上傳速度。
成本效率:無需伺服器基礎設施來處理大型上傳,從而可能降低成本。
可擴充性:非常適合在不給後端伺服器造成壓力的情況下擴展檔案上傳。
缺點:
安全風險:需要仔細處理 AWS 憑證和權限。必須安全地產生預簽名 URL,以防止未經授權的存取。
有限控制:伺服器端對上傳的監督較少;錯誤處理和重試通常在客戶端進行管理。
瀏覽器限制:瀏覽器有記憶體和 API 限制,這可能會阻礙處理非常大的檔案或影響低階裝置上的效能。
實施安全客戶端上傳的逐步指南
安全地實現客戶端上傳涉及前端應用程式和安全後端服務之間的協調。後端服務的主要作用是產生預簽名 URL,讓用戶端將檔案直接上傳到 S3,而無需暴露敏感的 AWS 憑證。
先決條件
- AWS 帳戶:存取有權使用 S3 的 AWS 帳戶。
- AWS 開發工具包知識:熟悉適用於 JavaScript 的 AWS 開發工具包 (v3) 或直接對 AWS 服務進行 API 呼叫。
- 前端和後端開發技能:了解客戶端(JavaScript、React 等)和伺服器端(Node.js、Express 等)程式設計。
1. 設定正確的架構
要有效實現客戶端上傳,您需要:
- 前端應用程式:處理文件選擇、根據需要將文件拆分為多個部分,以及使用預簽名 URL 將各個部分上傳到 S3。
- 後端服務:一個安全伺服器,提供用於產生預簽名 URL 以及初始化或完成分段上傳的 API。它可以保證您的 AWS 憑證的安全性並強制執行任何必要的業務邏輯或驗證。
此架構確保敏感操作在後端安全處理,而前端則管理上傳過程。
2. 後端建立上傳服務
為什麼使用預簽名 URL?
預簽名 URL 允許客戶端直接與 S3 交互,執行上傳文件等操作,而無需在用戶端提供 AWS 憑證。它們是安全的,因為:
- 它們是有時間限制的,並在指定期限後過期。
- 它們可以被限制為特定操作(例如,PUT 用於上傳)。
- 它們特定於特定的 S3 物件金鑰。
實作S3UploadService
在伺服器上建立一個服務類,負責:
a.定義 S3 儲存桶和區域
b.安全地建立 AWS 憑證。
c.提供產生預簽名 URL 和管理分段上傳的方法。
// services/S3UploadService.js import { S3Client, CreateMultipartUploadCommand, CompleteMultipartUploadCommand, UploadPartCommand, AbortMultipartUploadCommand, PutObjectCommand, GetObjectCommand, DeleteObjectCommand, } from '@aws-sdk/client-s3'; import { getSignedUrl } from '@aws-sdk/s3-request-presigner'; // Import credential providers import { fromIni, fromInstanceMetadata, fromEnv, fromProcess, } from '@aws-sdk/credential-providers'; export class S3UploadService { constructor() { this.s3BucketName = process.env.S3_BUCKET_NAME; this.s3Region = process.env.S3_REGION; this.s3Client = new S3Client({ region: this.s3Region, credentials: this.getS3ClientCredentials(), }); } // Method to generate AWS credentials securely getS3ClientCredentials() { if (process.env.NODE_ENV === 'development') { // In development, use credentials from environment variables return fromEnv(); } else { // In production, use credentials from EC2 instance metadata or another secure method return fromInstanceMetadata(); } } // Generate a presigned URL for single-part upload (PUT), download (GET), or deletion (DELETE) async generatePresignedUrl(key, operation) { let command; switch (operation) { case 'PUT': command = new PutObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'GET': command = new GetObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'DELETE': command = new DeleteObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; default: throw new Error(`Invalid operation "${operation}"`); } // Generate presigned URL return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); // Expires in 1 hour } // Methods for multipart upload async createMultipartUpload(key) { const command = new CreateMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, }); const response = await this.s3Client.send(command); return response.UploadId; } async generateUploadPartUrl(key, uploadId, partNumber) { const command = new UploadPartCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, PartNumber: partNumber, }); return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); } async completeMultipartUpload(key, uploadId, parts) { const command = new CompleteMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, MultipartUpload: { Parts: parts }, }); return await this.s3Client.send(command); } async abortMultipartUpload(key, uploadId) { const command = new AbortMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, }); return await this.s3Client.send(command); } }
注意:確保您的 AWS 憑證已安全管理。在生產中,建議使用附加到 EC2 執行個體或 ECS 任務的 IAM 角色,而不是硬編碼憑證或使用環境變數。
3. 實作後端 API 端點
在後端建立 API 端點來處理來自前端的請求。這些端點將會利用 S3UploadService 來執行操作。
// controllers/S3UploadController.js import { S3UploadService } from '../services/S3UploadService'; const s3UploadService = new S3UploadService(); export const generatePresignedUrl = async (req, res, next) => { try { const { key, operation } = req.body; // key is the S3 object key (file identifier) const url = await s3UploadService.generatePresignedUrl(key, operation); res.status(200).json({ url }); } catch (error) { next(error); } }; export const initializeMultipartUpload = async (req, res, next) => { try { const { key } = req.body; const uploadId = await s3UploadService.createMultipartUpload(key); res.status(200).json({ uploadId }); } catch (error) { next(error); } }; export const generateUploadPartUrls = async (req, res, next) => { try { const { key, uploadId, parts } = req.body; // parts is the number of parts const urls = await Promise.all( [...Array(parts).keys()].map(async (index) => { const partNumber = index + 1; const url = await s3UploadService.generateUploadPartUrl(key, uploadId, partNumber); return { partNumber, url }; }) ); res.status(200).json({ urls }); } catch (error) { next(error); } }; export const completeMultipartUpload = async (req, res, next) => { try { const { key, uploadId, parts } = req.body; // parts is an array of { ETag, PartNumber } const result = await s3UploadService.completeMultipartUpload(key, uploadId, parts); res.status(200).json({ result }); } catch (error) { next(error); } }; export const abortMultipartUpload = async (req, res, next) => { try { const { key, uploadId } = req.body; await s3UploadService.abortMultipartUpload(key, uploadId); res.status(200).json({ message: 'Upload aborted' }); } catch (error) { next(error); } };
在 Express 應用程式或您使用的任何框架中設定這些端點的路由。
4. 實作前端上傳器類
前端將處理選擇文件,根據文件大小決定是否執行單部分或分段上傳,並管理上傳過程。
一般來說,AWS 建議「當您的物件大小達到 100 MB 時,您應該考慮使用分段上傳,而不是在單一操作中上傳物件。」來源
// services/S3UploadService.js import { S3Client, CreateMultipartUploadCommand, CompleteMultipartUploadCommand, UploadPartCommand, AbortMultipartUploadCommand, PutObjectCommand, GetObjectCommand, DeleteObjectCommand, } from '@aws-sdk/client-s3'; import { getSignedUrl } from '@aws-sdk/s3-request-presigner'; // Import credential providers import { fromIni, fromInstanceMetadata, fromEnv, fromProcess, } from '@aws-sdk/credential-providers'; export class S3UploadService { constructor() { this.s3BucketName = process.env.S3_BUCKET_NAME; this.s3Region = process.env.S3_REGION; this.s3Client = new S3Client({ region: this.s3Region, credentials: this.getS3ClientCredentials(), }); } // Method to generate AWS credentials securely getS3ClientCredentials() { if (process.env.NODE_ENV === 'development') { // In development, use credentials from environment variables return fromEnv(); } else { // In production, use credentials from EC2 instance metadata or another secure method return fromInstanceMetadata(); } } // Generate a presigned URL for single-part upload (PUT), download (GET), or deletion (DELETE) async generatePresignedUrl(key, operation) { let command; switch (operation) { case 'PUT': command = new PutObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'GET': command = new GetObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'DELETE': command = new DeleteObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; default: throw new Error(`Invalid operation "${operation}"`); } // Generate presigned URL return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); // Expires in 1 hour } // Methods for multipart upload async createMultipartUpload(key) { const command = new CreateMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, }); const response = await this.s3Client.send(command); return response.UploadId; } async generateUploadPartUrl(key, uploadId, partNumber) { const command = new UploadPartCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, PartNumber: partNumber, }); return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); } async completeMultipartUpload(key, uploadId, parts) { const command = new CompleteMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, MultipartUpload: { Parts: parts }, }); return await this.s3Client.send(command); } async abortMultipartUpload(key, uploadId) { const command = new AbortMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, }); return await this.s3Client.send(command); } }
使用範例
// controllers/S3UploadController.js import { S3UploadService } from '../services/S3UploadService'; const s3UploadService = new S3UploadService(); export const generatePresignedUrl = async (req, res, next) => { try { const { key, operation } = req.body; // key is the S3 object key (file identifier) const url = await s3UploadService.generatePresignedUrl(key, operation); res.status(200).json({ url }); } catch (error) { next(error); } }; export const initializeMultipartUpload = async (req, res, next) => { try { const { key } = req.body; const uploadId = await s3UploadService.createMultipartUpload(key); res.status(200).json({ uploadId }); } catch (error) { next(error); } }; export const generateUploadPartUrls = async (req, res, next) => { try { const { key, uploadId, parts } = req.body; // parts is the number of parts const urls = await Promise.all( [...Array(parts).keys()].map(async (index) => { const partNumber = index + 1; const url = await s3UploadService.generateUploadPartUrl(key, uploadId, partNumber); return { partNumber, url }; }) ); res.status(200).json({ urls }); } catch (error) { next(error); } }; export const completeMultipartUpload = async (req, res, next) => { try { const { key, uploadId, parts } = req.body; // parts is an array of { ETag, PartNumber } const result = await s3UploadService.completeMultipartUpload(key, uploadId, parts); res.status(200).json({ result }); } catch (error) { next(error); } }; export const abortMultipartUpload = async (req, res, next) => { try { const { key, uploadId } = req.body; await s3UploadService.abortMultipartUpload(key, uploadId); res.status(200).json({ message: 'Upload aborted' }); } catch (error) { next(error); } };
5. 安全注意事項與最佳實踐
- 限制預簽名 URL 權限:確保預簽名 URL 僅授予必要的權限(例如,僅允許上傳的 PUT 操作)。
- 設定適當的過期時間:預簽 URL 應在合理的時間(例如 15 分鐘到 1 小時)後過期,以最大限度地減少誤用的時間。
- 驗證檔案元資料:在後端,驗證從客戶端發送的任何元資料或參數以防止操縱(例如,強制執行允許的檔案類型或大小)。
- 使用 HTTPS:請務必使用 HTTPS 進行客戶端和後端之間的通信,以及存取 S3 時,以保護傳輸中的資料。
- 監控和日誌:在後端和 S3 上實作日誌記錄和監控,以偵測任何異常活動或錯誤。
6. 其他注意事項
限制物件大小
雖然AWS S3 支援大小高達5 TiB(太字節)的對象,但由於瀏覽器限制和客戶端資源限制,直接從瀏覽器上傳如此大的檔案是不切實際的,而且通常是不可能的。處理非常大的檔案時,瀏覽器可能會崩潰或變得無回應,特別是需要在記憶體中處理它們時。
推薦:
- 設定實際限制:定義您的應用程式支援用戶端上傳的最大檔案大小(例如,100 GB 或更少)。
- 通知使用者:向使用者提供有關允許的最大檔案大小的回饋,並在開始上傳之前在客戶端處理驗證。
重試策略
上傳大檔案會增加上傳過程中網路中斷或失敗的風險。實施穩健的重試策略對於增強使用者體驗並確保成功上傳至關重要。
策略
- 自動重試:在提示使用者之前自動重試失敗的部分有限次數。
- 可恢復上傳:追蹤上傳的部分,以便上傳可以從中斷處恢復,而不是重新開始。
- 錯誤處理:如果重試失敗,提供使用者資訊豐富的錯誤訊息,可能會建議檢查網路連線等操作。
分段上傳清理
不完整的分段上傳可能會累積在您的 S3 儲存桶中,消耗儲存空間並可能產生費用。
注意事項
- 中止未完成的上傳:如果上傳失敗或取消,請確保您的應用程式呼叫 AbortMultipartUpload API 來清理所有已上傳的部分。
- 生命週期規則:配置 S3 生命週期策略以在一段時間(例如 7 天)後自動中止不完整的分段上傳。這有助於管理儲存成本並保持水桶清潔。
生命週期規則配置範例:
// services/S3UploadService.js import { S3Client, CreateMultipartUploadCommand, CompleteMultipartUploadCommand, UploadPartCommand, AbortMultipartUploadCommand, PutObjectCommand, GetObjectCommand, DeleteObjectCommand, } from '@aws-sdk/client-s3'; import { getSignedUrl } from '@aws-sdk/s3-request-presigner'; // Import credential providers import { fromIni, fromInstanceMetadata, fromEnv, fromProcess, } from '@aws-sdk/credential-providers'; export class S3UploadService { constructor() { this.s3BucketName = process.env.S3_BUCKET_NAME; this.s3Region = process.env.S3_REGION; this.s3Client = new S3Client({ region: this.s3Region, credentials: this.getS3ClientCredentials(), }); } // Method to generate AWS credentials securely getS3ClientCredentials() { if (process.env.NODE_ENV === 'development') { // In development, use credentials from environment variables return fromEnv(); } else { // In production, use credentials from EC2 instance metadata or another secure method return fromInstanceMetadata(); } } // Generate a presigned URL for single-part upload (PUT), download (GET), or deletion (DELETE) async generatePresignedUrl(key, operation) { let command; switch (operation) { case 'PUT': command = new PutObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'GET': command = new GetObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; case 'DELETE': command = new DeleteObjectCommand({ Bucket: this.s3BucketName, Key: key, }); break; default: throw new Error(`Invalid operation "${operation}"`); } // Generate presigned URL return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); // Expires in 1 hour } // Methods for multipart upload async createMultipartUpload(key) { const command = new CreateMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, }); const response = await this.s3Client.send(command); return response.UploadId; } async generateUploadPartUrl(key, uploadId, partNumber) { const command = new UploadPartCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, PartNumber: partNumber, }); return await getSignedUrl(this.s3Client, command, { expiresIn: 3600 }); } async completeMultipartUpload(key, uploadId, parts) { const command = new CompleteMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, MultipartUpload: { Parts: parts }, }); return await this.s3Client.send(command); } async abortMultipartUpload(key, uploadId) { const command = new AbortMultipartUploadCommand({ Bucket: this.s3BucketName, Key: key, UploadId: uploadId, }); return await this.s3Client.send(command); } }
從主線程處理分段上傳
上傳大檔案可能會佔用大量資源,並可能導致瀏覽器主執行緒無回應,從而導致使用者體驗不佳。
解決方案:
- 使用 Web Workers:將上傳程序卸載至 Web Worker。 Web Workers 在背景運行,與 Web 應用程式的主執行緒分開,讓您執行資源密集型操作而不阻塞 UI。
好處:
- 提高效能:釋放主線程,確保 UI 在上傳過程中保持回應。
- 減少內存使用:幫助更有效地管理內存,因為可以在工作線程內處理大數據處理。
- 增強穩定性:降低瀏覽器在大量上傳期間無回應或崩潰的風險。
7. 瀏覽器相容性注意事項
在實作客戶端分段上傳時,瀏覽器相容性確實是一個問題。不同的瀏覽器可能對處理大文件上傳所需的 API 和功能有不同程度的支持,例如 *文件 API、Blob 切片、Web Workers 和網路請求處理* 。成功應對這些差異對於確保在所有支援的瀏覽器上獲得一致且可靠的使用者體驗至關重要。
相容性問題:
- 檔案 API 和 Blob 方法:大多數現代瀏覽器支援 Blob.slice(),但較舊的瀏覽器可能使用 Blob.webkitSlice() 或 Blob.mozSlice()。
- Web Workers:在現代瀏覽器中受支持,但在某些較舊的瀏覽器中不受支持,或在 Internet Explorer 中受到限制。
- Fetch API 和 XMLHttpRequest:雖然 fetch() 得到廣泛支持,但使用 fetch() 的上傳進度事件並非在所有瀏覽器上一致可用。
- 最大並發連線數:根據支援的瀏覽器之間的最低公分母限制同時上傳的數量(例如 6 個並發連線)。
- 記憶體限制:以小塊的形式處理文件,並避免一次將整個文件載入記憶體。
- CORS:設定 S3 CORS 策略以支援必要的 HTTP 方法(例如,PUT、POST)和標頭。
結論
透過使用預簽名 URL 和分段上傳實現客戶端上傳,您可以有效地直接處理任意大小的檔案上傳到 S3,從而減少伺服器負載並提高效能。請記住,透過安全地管理 AWS 憑證並限制預簽 URL 的權限和生命週期,將安全性放在首位。
本指南提供了使用 AWS S3、AWS SDK for JavaScript 和預簽名 URL 設定安全且可擴充的檔案上傳系統的逐步方法。透過提供的程式碼範例和最佳實踐,您就可以很好地增強應用程式的檔案上傳功能。
以上是優化大檔案上傳:安全地將客戶端分段上傳到 AWS S3的詳細內容。更多資訊請關注PHP中文網其他相關文章!
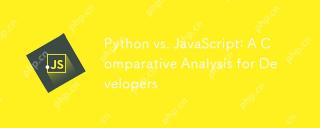
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
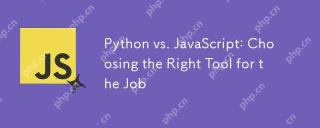
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
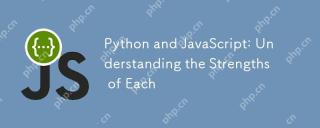
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
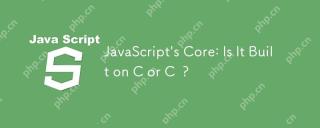
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
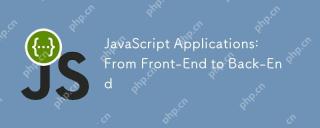
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
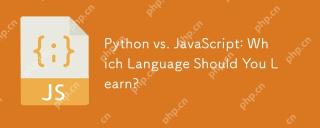
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。
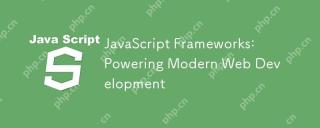
JavaScript框架的強大之處在於簡化開發、提升用戶體驗和應用性能。選擇框架時應考慮:1.項目規模和復雜度,2.團隊經驗,3.生態系統和社區支持。
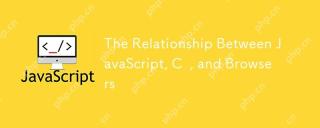
引言我知道你可能會覺得奇怪,JavaScript、C 和瀏覽器之間到底有什麼關係?它們之間看似毫無關聯,但實際上,它們在現代網絡開發中扮演著非常重要的角色。今天我們就來深入探討一下這三者之間的緊密聯繫。通過這篇文章,你將了解到JavaScript如何在瀏覽器中運行,C 在瀏覽器引擎中的作用,以及它們如何共同推動網頁的渲染和交互。 JavaScript與瀏覽器的關係我們都知道,JavaScript是前端開發的核心語言,它直接在瀏覽器中運行,讓網頁變得生動有趣。你是否曾經想過,為什麼JavaScr


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
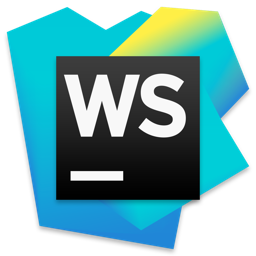
WebStorm Mac版
好用的JavaScript開發工具
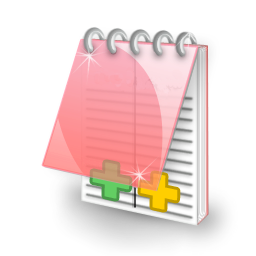
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),
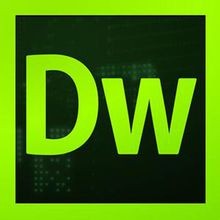
Dreamweaver CS6
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。