假設您有一場體育賽事或比賽。結果很可能會儲存在資料庫中,並且必須在網站上列出。您可以使用 Fetch API 從後端取得資料。本文檔中不會對此進行解釋。我假設資料已經被檢索並且是一個記錄數組。此記錄數組必須按正確的順序排列,但來源函數可以在報表引擎中動態過濾和排序該數組。
本文檔描述如何輕鬆定義頁眉和頁腳以及如何透過比較函數安排記錄分組。
每個標頭函數都會根據靜態文字和參數currentRecord、objWork和splitPosition傳回html。每個頁腳函數都會根據靜態文字和參數 previousRecord、objWork 和 splitPosition 傳回 html。
非常靈活,但你必須自己做html!不要指望所見即所得的編輯器。
報告的一般結構
- 報告有報告頁首和頁尾。可以是文字或只是 html,或兩者兼而有之。
- 報告具有一個或多個部分等級。部分等級 N 從頁首等級 N 開始,以頁腳等級 N 結束。
- 局部等級 N 包含一次或多次部分等級 N 1,最高部分等級除外。
- 最高部分層級包含基於陣列中的記錄所建立的資料。 在大多數情況下,最高部分等級只是一個 html 表格或 html 彈性項目。
報告結構範例
稱為reportDefinition的報告定義物件的結構
const reportDefinition = {}; reportDefinition.headers = [report_header, header_level_1, header_level_2, header_level_3, ...]; // default = [] reportDefinition.footers = [report_footer, footer_level_1, footer_level_2, footer_level_3, ...]; // default = [] reportDefinition.compare = (previousRecord, currentRecord, objWork) => { // default = () => -1 // code that returns an integer (report level break number) }; reportDefinition.display = (currentRecord, objWork) => { // code that returns a string, for example return `${currentRecord.team} - ${currentRecord.player}`; }; // source array can be preprocessed, for example filter or sort reportDefinition.source = (arr, objWork) => preProcessFuncCode(arr); // optional function to preprocess data array // example to add extra field for HOME and AWAY and sort afterwards reportDefinition.source = (arr, objWork) => arr.flatMap(val => [{ team: val.team1, ...val }, { team: val.team2, ...val }]) .sort((a, b) => a.team.localeCompare(b.team)); // optional method 'init' which should be a function. It will be called with argument objWork // can be used to initialize some things. reportDefinition.init = objWork => { ... };
頁首和頁尾數組元素的範例
reportDefinition.headers = []; // currentRecord=current record, objWork is extra object, // splitPosition=0 if this is the first header shown at this place, otherwise it is 1, 2, 3 ... reportDefinition.headers[0] = (currentRecord, objWork, splitPosition) => { // code that returns a string }; reportDefinition.headers[1] = '<div>Some string</div>'; // string instead of function is allowed; reportDefinition.footers = []; // previousRecord=previous record, objWork is extra object, // splitPosition=0 if this is the last footer shown at this place, otherwise it is 1, 2, 3 ... reportDefinition.footers[0] = (previousRecord, objWork, splitPosition) => { // code that returns a string }; reportDefinition.footers[1] = '<div>Some string</div>'; // string instead of function is allowed;
比較函數範例
// previousRecord=previous record, currentRecord=current record, objWork is extra object, reportDefinition.compare = (previousRecord, currentRecord, objWork) => { // please never return 0! headers[0] will be displayed automagically on top of report // group by date return 1 (lowest number first) if (previousRecord.date !== currentRecord.date) return 1; // group by team return 2 if (previousRecord.team !== currentRecord.team) return 2; // assume this function returns X (except -1) then: // footer X upto and include LAST footer will be displayed (in reverse order). In case of footer function the argument is previous record // header X upto and include LAST header will be displayed. In case of header function the argument is current record // current record will be displayed // // if both records belong to same group return -1 return -1; };
運行計數器
如果您想要實作一個正在運行的計數器,您必須在正確的位置初始化/重置它。可以透過在相關頭檔中放入一些程式碼來實現:
reportDefinition.headers[2] = (currentRecord, objWork, splitPosition) => { // this is a new level 2 group. Reset objWork.runningCounter to 0 objWork.runningCounter = 0; // put extra code here return `<div>This is header number 2: ${currentRecord.team}</div>`; };
如果您只想在報告開頭初始化objWork.runningCounter,您可以透過在reportDefinition.headers[0]中放置正確的程式碼來實現。我稱之為屬性 runningCounter,但您可以給它任何您想要的名稱。
您必須增加程式碼中某處的運行計數器,因為...否則它不會運行;-) 例如:
const reportDefinition = {}; reportDefinition.headers = [report_header, header_level_1, header_level_2, header_level_3, ...]; // default = [] reportDefinition.footers = [report_footer, footer_level_1, footer_level_2, footer_level_3, ...]; // default = [] reportDefinition.compare = (previousRecord, currentRecord, objWork) => { // default = () => -1 // code that returns an integer (report level break number) }; reportDefinition.display = (currentRecord, objWork) => { // code that returns a string, for example return `${currentRecord.team} - ${currentRecord.player}`; }; // source array can be preprocessed, for example filter or sort reportDefinition.source = (arr, objWork) => preProcessFuncCode(arr); // optional function to preprocess data array // example to add extra field for HOME and AWAY and sort afterwards reportDefinition.source = (arr, objWork) => arr.flatMap(val => [{ team: val.team1, ...val }, { team: val.team2, ...val }]) .sort((a, b) => a.team.localeCompare(b.team)); // optional method 'init' which should be a function. It will be called with argument objWork // can be used to initialize some things. reportDefinition.init = objWork => { ... };
如何建立多個部分層級的總計、運行總計甚至編號標題
reportDefinition.headers = []; // currentRecord=current record, objWork is extra object, // splitPosition=0 if this is the first header shown at this place, otherwise it is 1, 2, 3 ... reportDefinition.headers[0] = (currentRecord, objWork, splitPosition) => { // code that returns a string }; reportDefinition.headers[1] = '<div>Some string</div>'; // string instead of function is allowed; reportDefinition.footers = []; // previousRecord=previous record, objWork is extra object, // splitPosition=0 if this is the last footer shown at this place, otherwise it is 1, 2, 3 ... reportDefinition.footers[0] = (previousRecord, objWork, splitPosition) => { // code that returns a string }; reportDefinition.footers[1] = '<div>Some string</div>'; // string instead of function is allowed;
如何動態預處理來源數組(例如在按一下事件中)
// previousRecord=previous record, currentRecord=current record, objWork is extra object, reportDefinition.compare = (previousRecord, currentRecord, objWork) => { // please never return 0! headers[0] will be displayed automagically on top of report // group by date return 1 (lowest number first) if (previousRecord.date !== currentRecord.date) return 1; // group by team return 2 if (previousRecord.team !== currentRecord.team) return 2; // assume this function returns X (except -1) then: // footer X upto and include LAST footer will be displayed (in reverse order). In case of footer function the argument is previous record // header X upto and include LAST header will be displayed. In case of header function the argument is current record // current record will be displayed // // if both records belong to same group return -1 return -1; };
如何產生報告
reportDefinition.headers[2] = (currentRecord, objWork, splitPosition) => { // this is a new level 2 group. Reset objWork.runningCounter to 0 objWork.runningCounter = 0; // put extra code here return `<div>This is header number 2: ${currentRecord.team}</div>`; };
原始碼
以下是我創建的原始程式碼以使這一切正常工作。它是所有頁首和頁尾的包裝函數。請隨意複製貼上它並在您自己的模組中使用它。
reportDefinition.display = (currentRecord, objWork) => { objWork.runningCounter++; // put extra code here return `<div>This is record number ${objWork.runningCounter}: ${currentRecord.team} - ${currentRecord.player}</div>`; };
什麼是 objWork
objWork 是一個 javascript 對象,作為第二個參數傳遞給 createOutput 函數(可選參數,預設為 {})。它作為淺拷貝傳遞給頁首函數、頁尾函數、比較函數、初始化函數、來源函數和顯示函數。所有這些函數共享這個物件。例如,您可以將其用於配置資訊或顏色主題。 objWork 會自動使用 { rawData: thisData } 進行擴充。例如 createOutput(reportDefinition, { font: 'Arial', font_color: 'blue' }).
範例
下面列出的範例是用荷蘭語寫的。
撞球俱樂部的報道
桌球成績報告
更多關於開立球的報道
滾球報告
還有更多......
以上是使用 javascript 函數建立資料報告的詳細內容。更多資訊請關注PHP中文網其他相關文章!
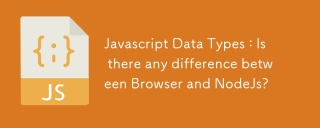
JavaScript核心數據類型在瀏覽器和Node.js中一致,但處理方式和額外類型有所不同。 1)全局對像在瀏覽器中為window,在Node.js中為global。 2)Node.js獨有Buffer對象,用於處理二進制數據。 3)性能和時間處理在兩者間也有差異,需根據環境調整代碼。
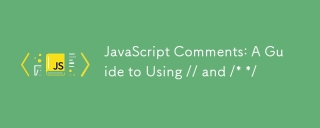
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
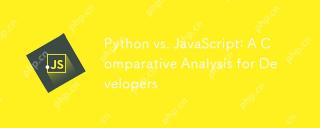
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
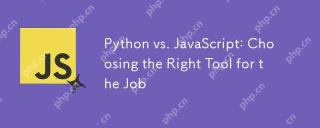
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
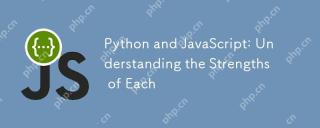
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
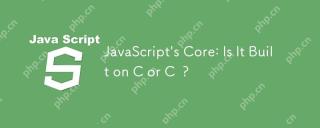
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
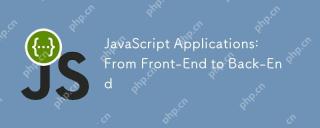
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
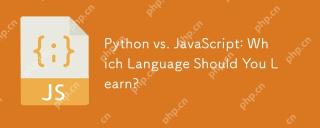
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
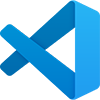
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

SublimeText3漢化版
中文版,非常好用
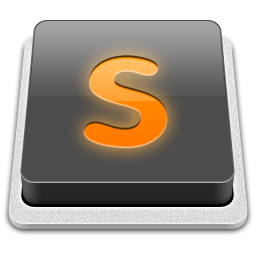
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)