在 PyGame 中向遊標發射子彈
在 PyGame 中,創建滑鼠遊標方向的射彈可能會帶來挑戰。跟隨。讓我們檢查一下提供的程式碼並解決其缺點。
現有程式碼分析
目前程式碼的目標是:
- 建立一個 Bullet 類別來表示一個 Bullet 類別來表示射彈。
- 初始化一個 Game 類別來處理子彈的產生和移動。
但是,實作有一些缺陷:
- 它使用 pygame .transform.rotate 錯誤,傳回一個新的旋轉表面,而不是變換原始物件。
- 它試圖動態計算移動方向和旋轉角度,這會導致不可預測的子彈行為。
正確的方法
為了解決這些問題,我們採用不同的方法:
-
在建立時初始化項目符號參數:
- 建立新子彈時,計算其起始位置和朝向滑鼠遊標的方向向量。
- 標準化方向向量以建立單位向量。
-
預旋轉子彈:
- 旋轉子彈表面以與計算的方向向量對齊。
-
連續位置更新:
- 透過將縮放的方向向量增量加到其目前位置來更新子彈的位置。
範例實作
<code class="python">import pygame import math # Bullet Class class Bullet: def __init__(self, x, y): # Calculate initial position and direction self.pos = (x, y) mx, my = pygame.mouse.get_pos() self.dir = (mx - x, my - y) # Normalize direction vector length = math.hypot(*self.dir) self.dir = (self.dir[0]/length, self.dir[1]/length) # Create bullet surface and rotate it self.bullet = pygame.Surface((7, 2)).convert_alpha() self.bullet.fill((255, 255, 255)) angle = math.degrees(math.atan2(-self.dir[1], self.dir[0])) self.bullet = pygame.transform.rotate(self.bullet, angle) self.speed = 2 # Adjust bullet speed as desired def update(self): # Update position based on scaled direction vector self.pos = (self.pos[0]+self.dir[0]*self.speed, self.pos[1]+self.dir[1]*self.speed) def draw(self, surface): # Draw bullet aligned with the correct direction bullet_rect = self.bullet.get_rect(center=self.pos) surface.blit(self.bullet, bullet_rect) # PyGame Main Loop pygame.init() window = pygame.display.set_mode((500, 500)) clock = pygame.time.Clock() bullets = [] # List to store bullet objects while True: clock.tick(60) # Set desired frame rate for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() elif event.type == pygame.MOUSEBUTTONDOWN: # Create a new bullet and add it to the list bullets.append(Bullet(*pygame.mouse.get_pos())) # Update and draw bullets for bullet in bullets[:]: bullet.update() if not window.get_rect().collidepoint(bullet.pos): # Remove bullets that leave the window boundary bullets.remove(bullet) else: # Draw bullet at its current position bullet.draw(window) # Render the updated display pygame.display.update()</code>
以上是如何在 PyGame 中讓子彈跟隨滑鼠遊標?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
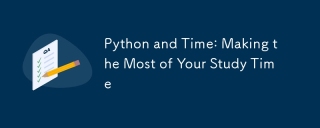
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
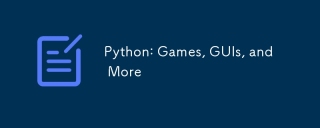
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
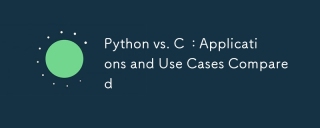
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
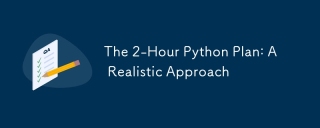
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
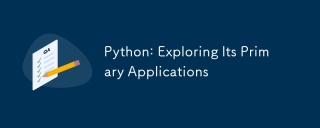
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
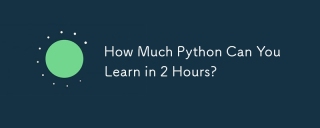
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
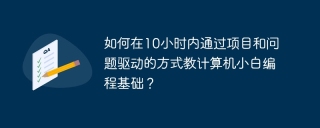
如何在10小時內教計算機小白編程基礎?如果你只有10個小時來教計算機小白一些編程知識,你會選擇教些什麼�...
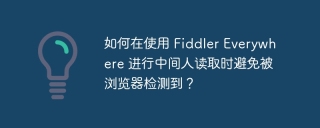
使用FiddlerEverywhere進行中間人讀取時如何避免被檢測到當你使用FiddlerEverywhere...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

禪工作室 13.0.1
強大的PHP整合開發環境

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
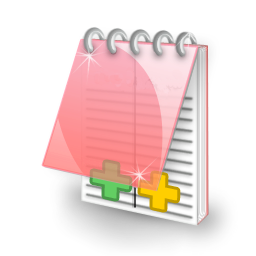
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
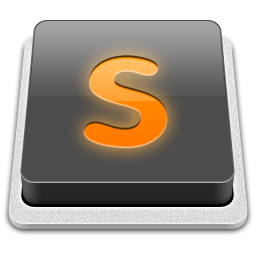
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。