透過 Hooks 對元素數組使用多個引用
useRef 鉤子提供對 React 元件中元素的持久引用。它允許您直接存取和修改 DOM 元素。對於單一元素,使用 useRef 很簡單。但是,如果您想對元素數組使用多個引用怎麼辦?
問題
考慮以下程式碼:
<code class="js">const { useRef, useState, useEffect } = React; const App = () => { const elRef = useRef(); const [elWidth, setElWidth] = useState(); useEffect(() => { setElWidth(elRef.current.offsetWidth); }, []); return ( <div> {[1, 2, 3].map(el => ( <div ref="{elRef}" style="{{" width:> Width is: {elWidth} </div> ))} </div> ); }; ReactDOM.render( <app></app>, document.getElementById("root") );</code>
此程式碼會導致錯誤,因為 elRef.current 不是陣列。它是對數組中第一個元素的引用。
使用外部引用的解決方案
此問題的可能解決方案是使用外部引用來保留多個元素的追蹤。以下是一個範例:
<code class="js">const itemsRef = useRef([]); const App = (props) => { useEffect(() => { itemsRef.current = itemsRef.current.slice(0, props.items.length); }, [props.items]); return props.items.map((item, i) => ( <div key="{i}" ref="{el"> (itemsRef.current[i] = el)} style={{ width: `${(i + 1) * 100}px` }} > ... </div> )); };</code>
在本例中,itemsRef.current 是一個陣列,其中保存對陣列中所有元素的參考。您可以使用 itemsRef.current[n].
注意: 重要的是要記住鉤子不能在循環內使用。因此,在循環外部使用 useEffect 鉤子來更新 itemsRef 陣列。
以上是如何對元素數組使用帶有鉤子的多個引用?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
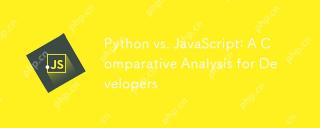
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
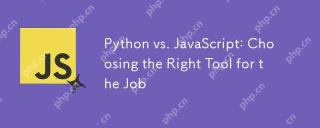
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
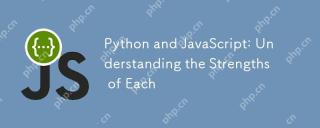
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
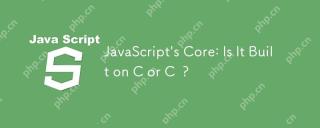
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
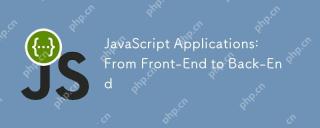
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
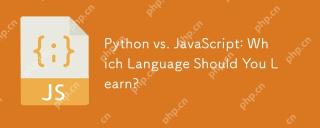
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。
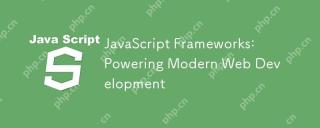
JavaScript框架的強大之處在於簡化開發、提升用戶體驗和應用性能。選擇框架時應考慮:1.項目規模和復雜度,2.團隊經驗,3.生態系統和社區支持。
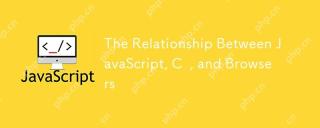
引言我知道你可能會覺得奇怪,JavaScript、C 和瀏覽器之間到底有什麼關係?它們之間看似毫無關聯,但實際上,它們在現代網絡開發中扮演著非常重要的角色。今天我們就來深入探討一下這三者之間的緊密聯繫。通過這篇文章,你將了解到JavaScript如何在瀏覽器中運行,C 在瀏覽器引擎中的作用,以及它們如何共同推動網頁的渲染和交互。 JavaScript與瀏覽器的關係我們都知道,JavaScript是前端開發的核心語言,它直接在瀏覽器中運行,讓網頁變得生動有趣。你是否曾經想過,為什麼JavaScr


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
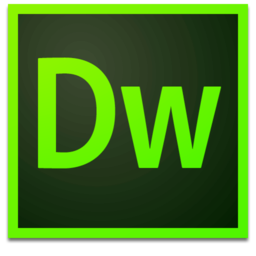
Dreamweaver Mac版
視覺化網頁開發工具

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
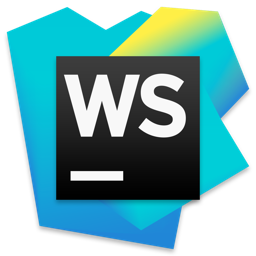
WebStorm Mac版
好用的JavaScript開發工具
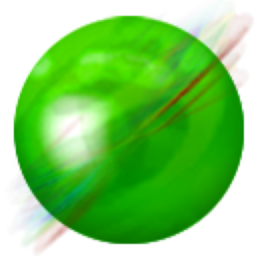
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境