Foreach 循環中的Lambda 表達式失敗
考慮以下初始化委託列表的程式碼,每個委託都呼叫具有特定類型和問候語的SayGreetingToType 方法:
<code class="csharp">public class MyClass { public delegate string PrintHelloType(string greeting); public void Execute() { Type[] types = new Type[] { typeof(string), typeof(float), typeof(int) }; List<printhellotype> helloMethods = new List<printhellotype>(); foreach (var type in types) { // Initialize the lambda expression with the captured variable 'type' var sayHello = new PrintHelloType(greeting => SayGreetingToType(type, greeting)); helloMethods.Add(sayHello); } // Call the delegates with the greeting "Hi" foreach (var helloMethod in helloMethods) { Console.WriteLine(helloMethod("Hi")); } } public string SayGreetingToType(Type type, string greetingText) { return greetingText + " " + type.Name; } }</printhellotype></printhellotype></code>
執行此程式碼後,您會期望看到:
Hi String Hi Single Hi Int32
但是,由於閉包行為,類型數組中的最後一個類型Int32 被所有lambda 捕獲表達式。因此,所有委託都以相同的類型呼叫SayGreetingToType,導致意外輸出:
Hi Int32 Hi Int32 Hi Int32
解決方案
要解決此問題,我們需要捕獲lambda 表達式中循環變數的值而不是變數本身:
<code class="csharp">public class MyClass { public delegate string PrintHelloType(string greeting); public void Execute() { Type[] types = new Type[] { typeof(string), typeof(float), typeof(int) }; List<printhellotype> helloMethods = new List<printhellotype>(); foreach (var type in types) { // Capture a copy of the current 'type' value using a new variable var newType = type; // Initialize the lambda expression with the new variable 'newType' var sayHello = new PrintHelloType(greeting => SayGreetingToType(newType, greeting)); helloMethods.Add(sayHello); } // Call the delegates with the greeting "Hi" foreach (var helloMethod in helloMethods) { Console.WriteLine(helloMethod("Hi")); } } public string SayGreetingToType(Type type, string greetingText) { return greetingText + " " + type.Name; } }</printhellotype></printhellotype></code>
此修改可確保每個lambda 表達式都有自己的類型副本,從而允許它使用正確的值呼叫SayGreetingToType輸入參數。
以上是為什麼 Foreach 迴圈內的 Lambda 表達式會捕捉循環變數的最後一個值?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
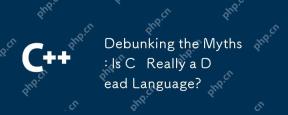
C 沒有死,反而在許多關鍵領域蓬勃發展:1)遊戲開發,2)系統編程,3)高性能計算,4)瀏覽器和網絡應用,C 依然是主流選擇,展現了其強大的生命力和應用場景。
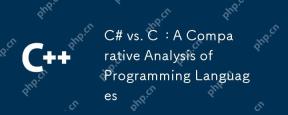
C#和C 的主要區別在於語法、內存管理和性能:1)C#語法現代,支持lambda和LINQ,C 保留C特性並支持模板。 2)C#自動內存管理,C 需要手動管理。 3)C 性能優於C#,但C#性能也在優化中。
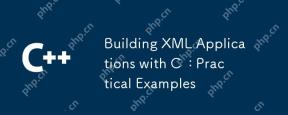
在C 中處理XML數據可以使用TinyXML、Pugixml或libxml2庫。 1)解析XML文件:使用DOM或SAX方法,DOM適合小文件,SAX適合大文件。 2)生成XML文件:將數據結構轉換為XML格式並寫入文件。通過這些步驟,可以有效地管理和操作XML數據。
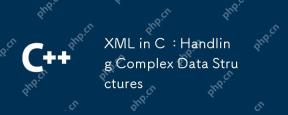
在C 中處理XML數據結構可以使用TinyXML或pugixml庫。 1)使用pugixml庫解析和生成XML文件。 2)處理複雜的嵌套XML元素,如書籍信息。 3)優化XML處理代碼,建議使用高效庫和流式解析。通過這些步驟,可以高效處理XML數據。
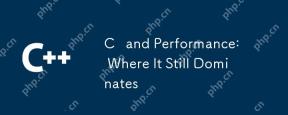
C 在性能優化方面仍然佔據主導地位,因為其低級內存管理和高效執行能力使其在遊戲開發、金融交易系統和嵌入式系統中不可或缺。具體表現為:1)在遊戲開發中,C 的低級內存管理和高效執行能力使得它成為遊戲引擎開發的首選語言;2)在金融交易系統中,C 的性能優勢確保了極低的延遲和高吞吐量;3)在嵌入式系統中,C 的低級內存管理和高效執行能力使得它在資源有限的環境中非常受歡迎。
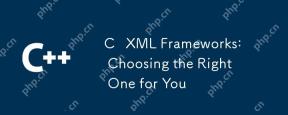
C XML框架的選擇應基於項目需求。 1)TinyXML適合資源受限環境,2)pugixml適用於高性能需求,3)Xerces-C 支持複雜的XMLSchema驗證,選擇時需考慮性能、易用性和許可證。
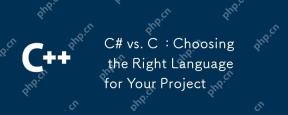
C#适合需要开发效率和类型安全的项目,而C 适合需要高性能和硬件控制的项目。1)C#提供垃圾回收和LINQ,适用于企业应用和Windows开发。2)C 以高性能和底层控制著称,广泛用于游戏和系统编程。
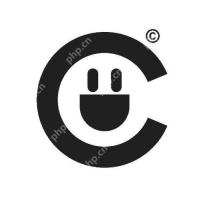
C 代碼優化可以通過以下策略實現:1.手動管理內存以優化使用;2.編寫符合編譯器優化規則的代碼;3.選擇合適的算法和數據結構;4.使用內聯函數減少調用開銷;5.應用模板元編程在編譯時優化;6.避免不必要的拷貝,使用移動語義和引用參數;7.正確使用const幫助編譯器優化;8.選擇合適的數據結構,如std::vector。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

記事本++7.3.1
好用且免費的程式碼編輯器
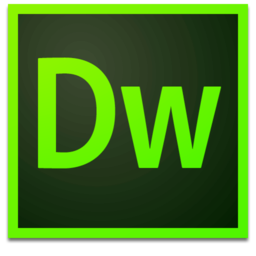
Dreamweaver Mac版
視覺化網頁開發工具
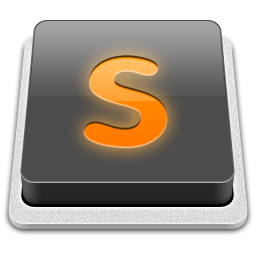
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!