如何建立可以接受 Form 或 JSON body 的 FastAPI 端點?
在 FastAPI 中,您可以定義處理各種類型請求正文的端點,例如JSON 或表單資料。這允許您建立可以接受任一格式的端點,而無需單獨的端點。
要實現此目的,您可以遵循以下方法之一:
選項1:使用依賴函數
您可以利用依賴函數來驗證請求的Content-Type 標頭,然後使用Starlette 的方法適當地解析正文。請注意,僅依賴 Content-Type 標頭可能無法始終保證請求正文的有效性,因此建議包含錯誤處理。
<code class="python">import os, sys from fastapi import FastAPI, Depends, HTTPException from starlette.requests import Request app = FastAPI() # Generating file open("./app.txt", "w").write("hello from a file") async def body_parser(request: Request): ct = request.headers.get("Content-Type", "") if ct == "application/json": try: d = await request.json() if not isinstance(d, dict): raise HTTPException(status_code=400, details={"error":"request body must be a dict"}) return d except JSONDecodeError: raise HTTPException(400, "Could not parse request body as JSON") elif ct == "multipart/form-data": await request.stream() # this is required for body parsing. d = await request.form() if not d: raise HTTPException(status_code=400, details={"error":"no form parameters found"}) return d else: raise HTTPException(405, "Content-Type must be either JSON or multipart/form-data") @app.post("/", dependencies=[Depends(body_parser)]) async def body_handler(d: dict): if "file" in d: return {"file": d["file"]} return d</code>
選項 2:利用選用表單/檔案參數
在此方法中,您可以在端點中將表單/檔案參數定義為可選。如果這些參數中的任何一個具有值,則它假定是表單資料請求。否則,它將驗證請求正文為 JSON。
<code class="python">from fastapi import FastAPI, Form, File, UploadFile app = FastAPI() @app.post("/") async def file_or_json( files: List[UploadFile] = File(None), some_data: str = Form(None) ): if files: return {"files": len(files)} return {"data": some_data}</code>
選項 3:為每種類型定義單獨的端點
您也可以建立單獨的端點,一個用於 JSON,另一個用於表單資料。使用中間件,您可以檢查 Content-Type 標頭並將請求重新路由到適當的端點。
<code class="python">from fastapi import FastAPI, Request, Form, File, UploadFile from fastapi.responses import JSONResponse app = FastAPI() @app.middleware("http") async def middleware(request: Request, call_next): ct = request.headers.get("Content-Type", "") if ct == "application/json": request.scope["path"] = "/json" elif ct in ["multipart/form-data", "application/x-www-form-urlencoded"]: request.scope["path"] = "/form" return await call_next(request) @app.post("/json") async def json_endpoint(json_data: dict): pass @app.post("/form") async def form_endpoint(file: UploadFile = File(...)): pass</code>
選項4:引用另一個答案以獲得替代方法
此外,您可以在Stack Overflow 上找到這個答案很有幫助,因為它提供了在單一端點中處理JSON 和表單資料的不同視角:
https://stackoverflow.com/a/67003163/10811840
測試選項1、2 和3
出於測試目的,您可以使用請求庫:
<code class="python">import requests url = "http://127.0.0.1:8000" # for testing Python 3.7 and above use: # url = "http://localhost:8000" # form-data request files = [('files', ('a.txt', open('a.txt', 'rb'), 'text/plain'))] response = requests.post(url, files=files) print(response.text) # JSON request data = {"some_data": "Hello, world!"} response = requests.post(url, json=data) print(response.text)</code>
這些方法提供了不同的方法來建立可以處理JSON 和表單的端點-FastAPI 中的資料。選擇最適合您的要求和用例的方法。
以上是如何建立接受表單或 JSON 正文的 FastAPI 端點?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
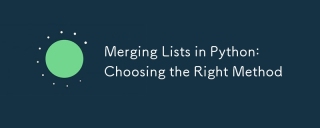
Tomergelistsinpython,YouCanusethe操作員,estextMethod,ListComprehension,Oritertools
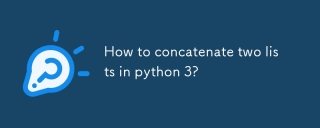
在Python3中,可以通過多種方法連接兩個列表:1)使用 運算符,適用於小列表,但對大列表效率低;2)使用extend方法,適用於大列表,內存效率高,但會修改原列表;3)使用*運算符,適用於合併多個列表,不修改原列表;4)使用itertools.chain,適用於大數據集,內存效率高。
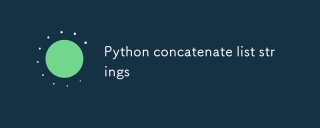
使用join()方法是Python中從列表連接字符串最有效的方法。 1)使用join()方法高效且易讀。 2)循環使用 運算符對大列表效率低。 3)列表推導式與join()結合適用於需要轉換的場景。 4)reduce()方法適用於其他類型歸約,但對字符串連接效率低。完整句子結束。
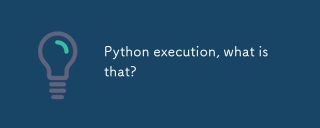
pythonexecutionistheprocessoftransformingpypythoncodeintoExecutablestructions.1)InternterPreterReadSthecode,ConvertingTingitIntObyTecode,whepythonvirtualmachine(pvm)theglobalinterpreterpreterpreterpreterlock(gil)the thepythonvirtualmachine(pvm)
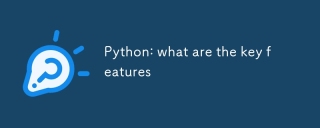
Python的關鍵特性包括:1.語法簡潔易懂,適合初學者;2.動態類型系統,提高開發速度;3.豐富的標準庫,支持多種任務;4.強大的社區和生態系統,提供廣泛支持;5.解釋性,適合腳本和快速原型開發;6.多範式支持,適用於各種編程風格。
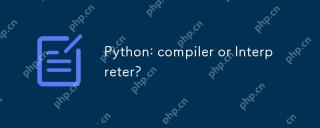
Python是解釋型語言,但也包含編譯過程。 1)Python代碼先編譯成字節碼。 2)字節碼由Python虛擬機解釋執行。 3)這種混合機制使Python既靈活又高效,但執行速度不如完全編譯型語言。
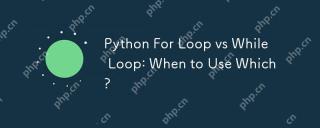
UseeAforloopWheniteratingOveraseQuenceOrforAspecificnumberoftimes; useAwhiLeLoopWhenconTinuingUntilAcIntiment.forloopsareIdealForkNownsences,而WhileLeleLeleLeleLeleLoopSituationSituationsItuationsItuationSuationSituationswithUndEtermentersitations。
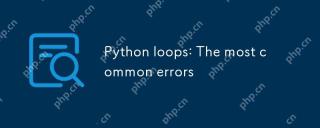
pythonloopscanleadtoerrorslikeinfiniteloops,modifyingListsDuringteritation,逐個偏置,零indexingissues,andnestedloopineflinefficiencies


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
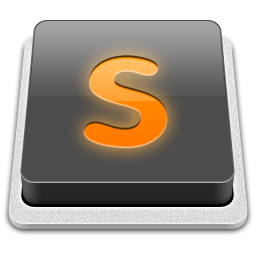
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

禪工作室 13.0.1
強大的PHP整合開發環境

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具