MongoDB is a popular choice for applications requiring scalability and flexibility, but to make the most of its features, performance tuning is essential. In this post, we’ll explore best practices for Java developers to optimize queries, writes, and proper configurations to ensure that your Java and MongoDB applications run efficiently.
As your MongoDB database grows, maintaining performance can become challenging. For Java developers working with MongoDB, understanding how to optimize queries and write operations is crucial to ensuring your application stays fast and scalable.
In this post, we’ll cover the key factors that impact MongoDB performance and how you can tune them to enhance the efficiency of your Java application.
- Indexing: The Key to Fast Queries One of the most effective ways to improve read performance in MongoDB is through indexing. MongoDB uses indexes to speed up queries, much like relational databases. Without proper indexing, MongoDB will perform a full collection scan, which can be costly for large collections.
How to Set Up Indexes
Using the Java MongoDB driver, you can easily create indexes with the following approach:
MongoCollection<document> collection = database.getCollection("myCollection"); collection.createIndex(Indexes.ascending("fieldToBeIndexed")); Ensure that frequently queried fields have indexes. It's essential to monitor your queries and adjust indexes accordingly, removing unused indexes and adding new ones where needed. </document>
Compound Indexes
If your queries filter based on more than one field, compound indexes can boost performance. For example:
collection.createIndex(Indexes.compoundIndex(Indexes.ascending("name"), Indexes.ascending("age")));
- Efficient Memory Usage: Limit Document Size MongoDB loads entire documents into memory when retrieved, so keeping documents small and optimized is critical. Avoid storing large blobs or binary data directly in MongoDB. If you need to store large files, consider using GridFS, a tool built into MongoDB for handling large files more efficiently.
Also, use field projection to retrieve only the necessary data:
FindIterable<document> docs = collection.find() .projection(Projections.include("field1", "field2")); This helps to avoid overloading memory by fetching unnecessary fields in queries. </document>
- Connection Pooling Connection management can also significantly impact performance. MongoDB provides a connection pool that should be properly configured to avoid bottlenecks under heavy load.
In Java, when using MongoClient, you can configure the connection pool as follows:
MongoClientOptions options = MongoClientOptions.builder() .connectionsPerHost(100) // Maximum number of connections .minConnectionsPerHost(10) .build();
Adjust these values based on your workload requirements.
- Batch Operations To improve write performance, consider using batch operations. Instead of inserting documents one by one, you can insert multiple at once:
List<writemodel>> operations = new ArrayList(); operations.add(new InsertOneModel(new Document("field", "value"))); operations.add(new InsertOneModel(new Document("field", "value2"))); collection.bulkWrite(operations); </writemodel>
This reduces the number of network operations and can significantly boost throughput.
- Continuous Monitoring and Adjustments Monitoring your database performance is crucial for making continuous adjustments. MongoDB offers tools like the MongoDB Atlas Performance Advisor and Profiler that help identify slow queries and suggest indexes to improve performance.
On the Java side, you can use performance monitoring libraries like Micrometer to collect detailed metrics from your application and spot potential bottlenecks.
- Sharding and Replication If your database starts growing exponentially, considering sharding (data partitioning) might be necessary. Sharding distributes data across multiple servers, allowing MongoDB to scale horizontally.
Additionally, replication is important for ensuring high availability and fault tolerance. MongoDB replicates data across multiple servers, which can also improve read performance by distributing read operations across replica members.
MongoDB is a powerful NoSQL solution, but like any database, it requires tuning to ensure maximum efficiency. Java developers who understand how to configure indexes, manage connections, and optimize queries have a significant advantage in building scalable, high-performance applications.
By implementing these tuning practices in MongoDB, you can make a critical difference in your application's performance. Keep monitoring, adjusting, and scaling as your database grows, and you’ll see how these optimizations can help maintain a fast and responsive system.
If you have any questions or want to learn more about optimizing MongoDB with Java, feel free to leave a comment or get in touch!
以上是面向 Java 開發人員的 MongoDB 效能調優的詳細內容。更多資訊請關注PHP中文網其他相關文章!
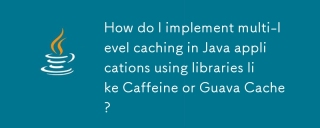
本文討論了使用咖啡因和Guava緩存在Java中實施多層緩存以提高應用程序性能。它涵蓋設置,集成和績效優勢,以及配置和驅逐政策管理最佳PRA
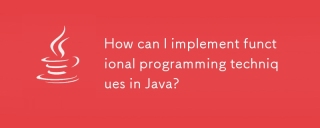
本文使用lambda表達式,流API,方法參考和可選探索將功能編程集成到Java中。 它突出顯示了通過簡潔性和不變性改善代碼可讀性和可維護性等好處
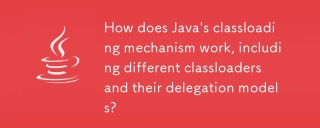
Java的類上載涉及使用帶有引導,擴展程序和應用程序類負載器的分層系統加載,鏈接和初始化類。父代授權模型確保首先加載核心類別,從而影響自定義類LOA

本文討論了使用JPA進行對象相關映射,並具有高級功能,例如緩存和懶惰加載。它涵蓋了設置,實體映射和優化性能的最佳實踐,同時突出潛在的陷阱。[159個字符]

本文討論了使用Maven和Gradle進行Java項目管理,構建自動化和依賴性解決方案,以比較其方法和優化策略。

本文使用選擇器和頻道使用單個線程有效地處理多個連接的Java的NIO API,用於非阻滯I/O。 它詳細介紹了過程,好處(可伸縮性,性能)和潛在的陷阱(複雜性,
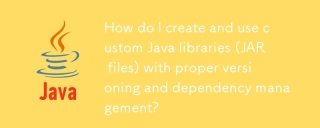
本文使用Maven和Gradle之類的工具討論了具有適當的版本控制和依賴關係管理的自定義Java庫(JAR文件)的創建和使用。
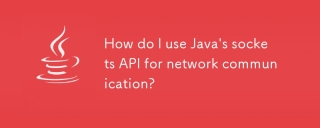
本文詳細介紹了用於網絡通信的Java的套接字API,涵蓋了客戶服務器設置,數據處理和關鍵考慮因素,例如資源管理,錯誤處理和安全性。 它還探索了性能優化技術,我


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),
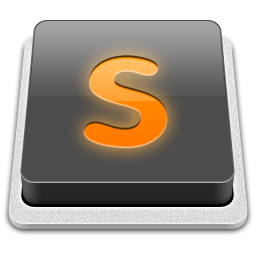
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SublimeText3 Linux新版
SublimeText3 Linux最新版