簡介:
資料流平台對於金融、物聯網、醫療保健和社交媒體等各行業高效處理即時數據至關重要。然而,實現一個強大的資料流平台來處理即時攝取、處理、容錯和可擴展性需要仔細考慮幾個關鍵因素。
在本文中,我們將使用 Kafka 進行訊息代理程式建立一個基於 Python 的資料流平台,探索即時系統中的各種挑戰,並討論擴展、監控、資料一致性和容錯的策略。我們將超越基本範例,涵蓋不同領域的用例,例如詐欺偵測、預測分析和物聯網監控。
1.深入探討流架構
除了基本元件之外,我們還可以擴展針對不同用例設計的特定架構:
Lambda 架構:
- 批次層:處理大量歷史資料(例如,使用 Apache Spark 或 Hadoop)。
- 速度層:處理即時串流資料(使用Kafka Streams)。
- 服務層:組合兩層的結果以提供低延遲查詢。
Kappa 建築:
一個簡化版本,只專注於即時資料處理,沒有批次層。非常適合需要連續處理資料流的環境。
包括這些架構如何在各種場景下處理資料的圖表和解釋。
2.進階 Kafka 設定
在 Docker 中執行 Kafka(用於雲端部署)
不用在本地運行 Kafka,而是在 Docker 中運行 Kafka,可以輕鬆部署在雲端或生產環境:
version: '3' services: zookeeper: image: wurstmeister/zookeeper ports: - "2181:2181" kafka: image: wurstmeister/kafka ports: - "9092:9092" environment: KAFKA_ADVERTISED_LISTENERS: INSIDE://kafka:9092,OUTSIDE://localhost:9092 KAFKA_LISTENER_SECURITY_PROTOCOL_MAP: INSIDE:PLAINTEXT,OUTSIDE:PLAINTEXT KAFKA_INTER_BROKER_LISTENER_NAME: INSIDE depends_on: - zookeeper
使用此 Docker 設定可以在生產和雲端環境中實現更好的可擴充性。
3.使用 Apache Avro 進行架構管理
由於流系統中的資料通常是異質的,因此管理模式對於生產者和消費者之間的一致性至關重要。 Apache Avro 提供緊湊、快速的二進位格式,用於高效序列化大型資料流。
具有 Avro 架構的生產者程式碼:
from confluent_kafka import avro from confluent_kafka.avro import AvroProducer value_schema_str = """ { "namespace": "example.avro", "type": "record", "name": "User", "fields": [ {"name": "name", "type": "string"}, {"name": "age", "type": "int"} ] } """ value_schema = avro.loads(value_schema_str) def avro_produce(): avroProducer = AvroProducer({ 'bootstrap.servers': 'localhost:9092', 'schema.registry.url': 'http://localhost:8081' }, default_value_schema=value_schema) avroProducer.produce(topic='users', value={"name": "John", "age": 30}) avroProducer.flush() if __name__ == "__main__": avro_produce()
說明:
- 模式註冊表: 確保生產者和消費者就模式達成協議。
- AvroProducer: 使用 Avro 處理訊息序列化。
4.使用 Apache Kafka Streams 進行流處理
除了使用streamz之外,還引入Kafka Streams作為更高級的流處理庫。 Kafka Streams 提供內建的容錯、狀態處理和一次性語義。
Kafka 流處理器範例:
from confluent_kafka import Consumer, Producer from confluent_kafka.avro import AvroConsumer import json def process_stream(): c = Consumer({ 'bootstrap.servers': 'localhost:9092', 'group.id': 'stream_group', 'auto.offset.reset': 'earliest' }) c.subscribe(['sensor_data']) while True: msg = c.poll(1.0) if msg is None: continue message_data = json.loads(msg.value().decode('utf-8')) # Process the sensor data and detect anomalies if message_data['temperature'] > 100: print(f"Warning! High temperature: {message_data['temperature']}") c.close() if __name__ == "__main__": process_stream()
流處理的關鍵用例:
- 即時異常偵測 (IoT):偵測感測器資料中的異常。
- 詐欺偵測(金融):即時標記可疑交易。
- 預測分析:預測未來事件,例如股價變動。
5.處理複雜事件處理 (CEP)
複雜事件處理是資料流平台的關鍵方面,其中分析多個事件以檢測隨時間變化的模式或趨勢。
用例範例:詐欺偵測
我們可以實現事件模式,例如在短時間內偵測多次失敗的登入嘗試。
from streamz import Stream # Assuming the event source is streaming failed login attempts def process_event(event): if event['login_attempts'] > 5: print(f"Fraud Alert: Multiple failed login attempts from {event['ip']}") def source(): # Simulate event stream yield {'ip': '192.168.1.1', 'login_attempts': 6} yield {'ip': '192.168.1.2', 'login_attempts': 2} # Apply pattern matching in the stream stream = Stream.from_iterable(source()) stream.map(process_event).sink(print) stream.start()
這展示如何應用 CEP 進行即時詐欺偵測。
6.資料流平台的安全性
在處理即時資料時,安全性常常被忽視,但卻至關重要。在本節中,討論 Kafka 和流平台的加密、身份驗證和授權策略。
Kafka Security Configuration:
- TLS Encryption: Secure data in transit by enabling TLS on Kafka brokers.
- SASL Authentication: Implement Simple Authentication and Security Layer (SASL) with either Kerberos or SCRAM.
# server.properties (Kafka Broker) listeners=SASL_SSL://localhost:9093 ssl.keystore.location=/var/private/ssl/kafka.server.keystore.jks ssl.keystore.password=test1234 ssl.key.password=test1234
Access Control in Kafka:
Use ACLs (Access Control Lists) to define who can read, write, or manage Kafka topics.
7. Monitoring & Observability
Real-time monitoring is crucial to ensure smooth functioning. Discuss how to set up monitoring for Kafka and Python applications using tools like Prometheus, Grafana, and Kafka Manager.
Prometheus Metrics for Kafka:
scrape_configs: - job_name: 'kafka' static_configs: - targets: ['localhost:9092'] metrics_path: /metrics scrape_interval: 15s
Logging and Metrics with Python:
Integrate logging and monitoring libraries to track errors and performance:
import logging logging.basicConfig(level=logging.INFO) def process_message(msg): logging.info(f"Processing message: {msg}")
8. Data Sink Options: Batch and Real-time Storage
Discuss how processed data can be stored for further analysis and exploration.
Real-Time Databases:
- TimescaleDB: A PostgreSQL extension for time-series data.
- InfluxDB: Ideal for storing real-time sensor or event data.
Batch Databases:
- PostgreSQL/MySQL: Traditional relational databases for storing transactional data.
- HDFS/S3: For long-term storage of large volumes of data.
9. Handling Backpressure & Flow Control
In data streaming, producers can often overwhelm consumers, causing a bottleneck. We need mechanisms to handle backpressure.
Backpressure Handling with Kafka:
- Set consumer max.poll.records to control how many records the consumer retrieves in each poll.
max.poll.records=500
Implementing Flow Control in Python:
# Limit the rate of message production import time from confluent_kafka import Producer def produce_limited(): p = Producer({'bootstrap.servers': 'localhost:9092'}) for data in range(100): p.produce('stock_prices', key=str(data), value=f"Price-{data}") p.poll(0) time.sleep(0.1) # Slow down the production rate p.flush() if __name__ == "__main__": produce_limited()
10. Conclusion and Future Scope
In this expanded version, we’ve delved into a broad spectrum of challenges and solutions in data streaming platforms. From architecture to security, monitoring, stream processing, and fault tolerance, this guide helps you build a production-ready system for real-time data processing using Python.
Future Enhancements:
- Explore **state
full stream processing** in more detail.
- Add support for exactly-once semantics using Kafka transactions.
- Use serverless frameworks like AWS Lambda to auto-scale stream processing.
Join me to gain deeper insights into the following topics:
- Python
- Data Streaming
- Apache Kafka
- Big Data
- Real-Time Data Processing
- Stream Processing
- Data Engineering
- Machine Learning
- Artificial Intelligence
- Cloud Computing
- Internet of Things (IoT)
- Data Science
- Complex Event Processing
- Kafka Streams
- APIs
- Cybersecurity
- DevOps
- Docker
- Apache Avro
- Microservices
- Technical Tutorials
- Developer Community
- Data Visualization
- Programming
Stay tuned for more articles and updates as we explore these areas and beyond.
以上是使用 Python 建立強大的資料流平台:即時資料處理綜合指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!
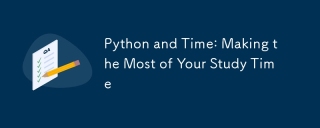
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
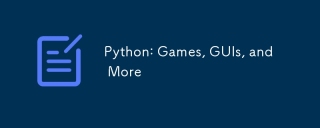
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
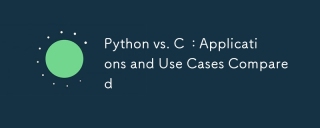
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
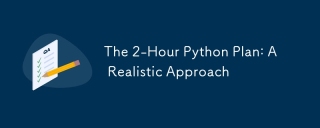
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
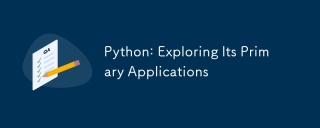
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
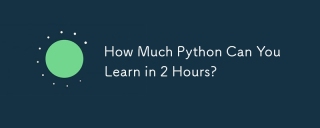
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
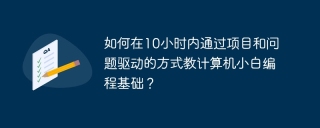
如何在10小時內教計算機小白編程基礎?如果你只有10個小時來教計算機小白一些編程知識,你會選擇教些什麼�...
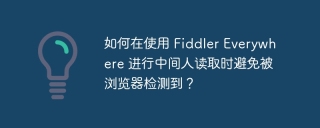
使用FiddlerEverywhere進行中間人讀取時如何避免被檢測到當你使用FiddlerEverywhere...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
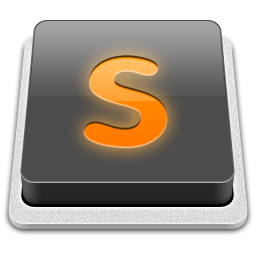
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Atom編輯器mac版下載
最受歡迎的的開源編輯器
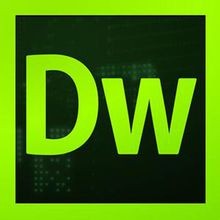
Dreamweaver CS6
視覺化網頁開發工具
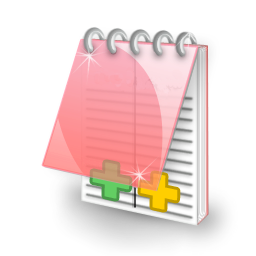
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能