編寫單元測試時,一個關鍵挑戰是確保您的測試專注於被測程式碼,而不受外部系統或依賴項的干擾。這就是模擬物件在PHPUnit中發揮作用的地方。它們允許您以受控方式模擬真實物件的行為,使您的測試更可靠且更易於維護。在本文中,我們將探討什麼是模擬物件、它們為何有用以及如何在 PHPUnit 中有效地使用它們。
什麼是模擬物件?
模擬物件是在單元測試中使用的真實物件的模擬版本。它們允許您:
- 隔離被測程式碼:模擬物件模擬依賴項的行為,確保測試結果不受這些依賴項實際實現的影響。
- 控制依賴行為:您可以指定在呼叫某些方法時模擬應如何表現,使您能夠測試不同的場景。
- 驗證互動:模擬追蹤方法呼叫及其參數,確保被測試的程式碼與其依賴項正確互動。
為什麼要使用模擬物件?
模擬在以下場景特別有用:
- 複雜的依賴關係:如果您的程式碼依賴資料庫、API 或第三方服務等外部系統,則模擬物件可以透過消除與這些系統互動的需要來簡化測試。
- 互動測試:模擬可讓您驗證是否使用正確的參數呼叫特定方法,確保您的程式碼能如預期運作。
- 更快的測試執行:資料庫查詢或 API 請求等實際操作可能會減慢測試速度。模擬這些依賴項可確保更快的測試執行。
存根與模擬:有什麼差別?
使用模擬物件時,您會遇到兩個術語:stubbing 和 mocking:
- 存根:指在模擬物件上定義方法的行為,例如指示方法傳回特定值。
- 模擬:涉及設定對如何呼叫方法的期望,例如驗證方法呼叫的數量及其參數。
如何在 PHPUnit 中建立和使用模擬對象
PHPUnit 透過 createMock() 方法可以輕鬆建立和使用模擬物件。以下是一些範例,示範如何有效地使用模擬物件。
範例 1:基本模擬物件用法
在此範例中,我們為類別依賴項建立一個模擬物件並指定其行為。
use PHPUnit\Framework\TestCase; class MyTest extends TestCase { public function testMockExample() { // Create a mock for the SomeClass dependency $mock = $this->createMock(SomeClass::class); // Specify that when the someMethod method is called, it returns 'mocked value' $mock->method('someMethod') ->willReturn('mocked value'); // Pass the mock object to the class under test $unitUnderTest = new ClassUnderTest($mock); // Perform the action and assert that the result matches the expected value $result = $unitUnderTest->performAction(); $this->assertEquals('expected result', $result); } }
說明:
- createMock(SomeClass::class) 為 SomeClass 建立一個模擬物件。
- method('someMethod')->willReturn('mocked value') 定義模擬的行為。
- 模擬物件被傳遞給正在測試的類,確保不使用真正的 SomeClass 實作。
範例 2:驗證方法調用
有時,您需要驗證是否使用正確的參數呼叫了方法。具體方法如下:
public function testMethodCallVerification() { // Create a mock object $mock = $this->createMock(SomeClass::class); // Expect the someMethod to be called once with 'expected argument' $mock->expects($this->once()) ->method('someMethod') ->with($this->equalTo('expected argument')) ->willReturn('mocked value'); // Pass the mock to the class under test $unitUnderTest = new ClassUnderTest($mock); // Perform an action that calls the mock's method $unitUnderTest->performAction(); }
重點:
- Expects($this->once()) 確保 someMethod 被呼叫一次。
- with($this->equalTo('expected argument')) 驗證是否使用正確的參數呼叫該方法。
範例:使用 PaymentProcessor 進行測試
為了示範模擬物件的實際應用,我們以依賴外部 PaymentGateway 介面的 PaymentProcessor 類別為例。我們想要測試 PaymentProcessor 的 processPayment 方法,而不依賴 PaymentGateway 的實際實作。
這是 PaymentProcessor 類別:
class PaymentProcessor { private $gateway; public function __construct(PaymentGateway $gateway) { $this->gateway = $gateway; } public function processPayment(float $amount): bool { return $this->gateway->charge($amount); } }
現在,我們可以為 PaymentGateway 建立一個模擬來測試 processPayment 方法,而無需與實際的支付網關互動。
使用模擬物件測試 PaymentProcessor
use PHPUnit\Framework\TestCase; class PaymentProcessorTest extends TestCase { public function testProcessPayment() { // Create a mock object for the PaymentGateway interface $gatewayMock = $this->createMock(PaymentGateway::class); // Define the expected behavior of the mock $gatewayMock->method('charge') ->with(100.0) ->willReturn(true); // Inject the mock into the PaymentProcessor $paymentProcessor = new PaymentProcessor($gatewayMock); // Assert that processPayment returns true $this->assertTrue($paymentProcessor->processPayment(100.0)); } }
檢定分解:
- createMock(PaymentGateway::class) creates a mock object simulating the PaymentGateway interface.
- method('charge')->with(100.0)->willReturn(true) specifies that when the charge method is called with 100.0 as an argument, it should return true.
- The mock object is passed to the PaymentProcessor class, allowing you to test processPayment without relying on a real payment gateway.
Verifying Interactions
You can also verify that the charge method is called exactly once when processing a payment:
public function testProcessPaymentCallsCharge() { $gatewayMock = $this->createMock(PaymentGateway::class); // Expect the charge method to be called once with the argument 100.0 $gatewayMock->expects($this->once()) ->method('charge') ->with(100.0) ->willReturn(true); $paymentProcessor = new PaymentProcessor($gatewayMock); $paymentProcessor->processPayment(100.0); }
In this example, expects($this->once()) ensures that the charge method is called exactly once. If the method is not called, or called more than once, the test will fail.
Example: Testing with a Repository
Let’s assume you have a UserService class that depends on a UserRepository to fetch user data. To test UserService in isolation, you can mock the UserRepository.
class UserService { private $repository; public function __construct(UserRepository $repository) { $this->repository = $repository; } public function getUserName($id) { $user = $this->repository->find($id); return $user->name; } }
To test this class, we can mock the repository:
use PHPUnit\Framework\TestCase; class UserServiceTest extends TestCase { public function testGetUserName() { // Create a mock for the UserRepository $mockRepo = $this->createMock(UserRepository::class); // Define that the find method should return a user object with a predefined name $mockRepo->method('find') ->willReturn((object) ['name' => 'John Doe']); // Instantiate the UserService with the mock repository $service = new UserService($mockRepo); // Assert that the getUserName method returns 'John Doe' $this->assertEquals('John Doe', $service->getUserName(1)); } }
Best Practices for Using Mocks
- Use Mocks Only When Necessary: Mocks are useful for isolating code, but overuse can make tests hard to understand. Only mock dependencies that are necessary for the test.
- Focus on Behavior, Not Implementation: Mocks should help test the behavior of your code, not the specific implementation details of dependencies.
- Avoid Mocking Too Many Dependencies: If a class requires many mocked dependencies, it might be a sign that the class has too many responsibilities. Refactor if needed.
- Verify Interactions Sparingly: Avoid over-verifying method calls unless essential to the test.
Conclusion
Mock objects are invaluable tools for writing unit tests in PHPUnit. They allow you to isolate your code from external dependencies, ensuring that your tests are faster, more reliable, and easier to maintain. Mock objects also help verify interactions between the code under test and its dependencies, ensuring that your code behaves correctly in various scenarios
以上是了解 PHPUnit 測試中的模擬對象的詳細內容。更多資訊請關注PHP中文網其他相關文章!
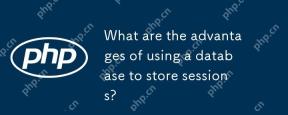
使用數據庫存儲會話的主要優勢包括持久性、可擴展性和安全性。 1.持久性:即使服務器重啟,會話數據也能保持不變。 2.可擴展性:適用於分佈式系統,確保會話數據在多服務器間同步。 3.安全性:數據庫提供加密存儲,保護敏感信息。
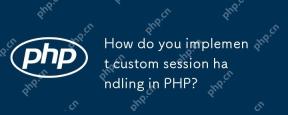
在PHP中實現自定義會話處理可以通過實現SessionHandlerInterface接口來完成。具體步驟包括:1)創建實現SessionHandlerInterface的類,如CustomSessionHandler;2)重寫接口中的方法(如open,close,read,write,destroy,gc)來定義會話數據的生命週期和存儲方式;3)在PHP腳本中註冊自定義會話處理器並啟動會話。這樣可以將數據存儲在MySQL、Redis等介質中,提升性能、安全性和可擴展性。
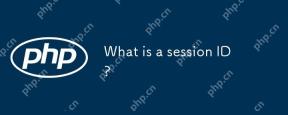
SessionID是網絡應用程序中用來跟踪用戶會話狀態的機制。 1.它是一個隨機生成的字符串,用於在用戶與服務器之間的多次交互中保持用戶的身份信息。 2.服務器生成並通過cookie或URL參數發送給客戶端,幫助在用戶的多次請求中識別和關聯這些請求。 3.生成通常使用隨機算法保證唯一性和不可預測性。 4.在實際開發中,可以使用內存數據庫如Redis來存儲session數據,提升性能和安全性。
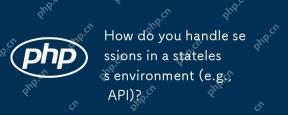
在無狀態環境如API中管理會話可以通過使用JWT或cookies來實現。 1.JWT適合無狀態和可擴展性,但大數據時體積大。 2.Cookies更傳統且易實現,但需謹慎配置以確保安全性。
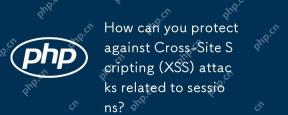
要保護應用免受與會話相關的XSS攻擊,需採取以下措施:1.設置HttpOnly和Secure標誌保護會話cookie。 2.對所有用戶輸入進行輸出編碼。 3.實施內容安全策略(CSP)限制腳本來源。通過這些策略,可以有效防護會話相關的XSS攻擊,確保用戶數據安全。
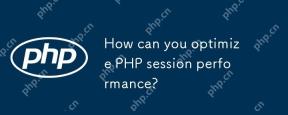
优化PHP会话性能的方法包括:1.延迟会话启动,2.使用数据库存储会话,3.压缩会话数据,4.管理会话生命周期,5.实现会话共享。这些策略能显著提升应用在高并发环境下的效率。
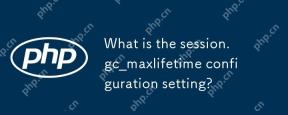
theSession.gc_maxlifetimesettinginphpdeterminesthelifespanofsessiondata,setInSeconds.1)它'sconfiguredinphp.iniorviaini_set().2)abalanceisesneededeededeedeedeededto toavoidperformance andunununununexpectedLogOgouts.3)

在PHP中,可以使用session_name()函數配置會話名稱。具體步驟如下:1.使用session_name()函數設置會話名稱,例如session_name("my_session")。 2.在設置會話名稱後,調用session_start()啟動會話。配置會話名稱可以避免多應用間的會話數據衝突,並增強安全性,但需注意會話名稱的唯一性、安全性、長度和設置時機。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

禪工作室 13.0.1
強大的PHP整合開發環境
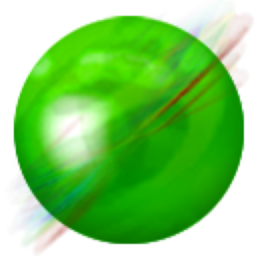
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

SublimeText3漢化版
中文版,非常好用

Atom編輯器mac版下載
最受歡迎的的開源編輯器