前言:學習了JavaScript之後,你可以使用JavaScript來實現一些有趣的效果。本文介紹如何純粹使用 JavaScript 在網頁上實現電子蜘蛛。
在開始學習如何寫網路蜘蛛之前,我們先來看看這個電子蜘蛛長什麼樣子:
可以看到它會隨著我們的滑鼠移動,那麼如何實現這個效果呢?讓我們開始解釋吧。
HTML 程式碼
<meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dreaming</title> <!-- External JavaScript files --> <script src=".test.js"></script> <style> /* Remove default padding and margins from body */ body { margin: 0px; padding: 0px; position: fixed; /* Set the background color of webpage to black */ background: rgb(0, 0, 0); } </style> <!-- Create a canvas for drawing --> <canvas id="canvas"></canvas>
如您所見,我們的 HTML 程式碼非常簡單,讓我們開始吧!
在開始編寫 JavaScript 程式碼之前,先制定一個計畫:
整體流程
頁面載入時,畫布元素和繪圖上下文將被初始化。
定義觸手物件。每根觸手由多個部分組成。
監聽滑鼠移動事件並即時更新滑鼠位置。
觸手透過動畫循環繪製,並根據滑鼠的位置動態變化,創造出流暢的動畫效果。
大致的流程就是上面的步驟,不過相信在你完成這段程式碼的編寫之前,你可能還不太理解上面的流程,不過沒關係,下面我們就開始寫我們的網路蜘蛛:
前言:為了幫助大家更好的理解程式碼邏輯,我為每段程式碼都加入了註解。希望您能在註解的幫助下一點點理解程式碼:
JavaScript 程式碼
// Define requestAnimFrame function window.requestAnimFrame = function () { // Check if the browser supports requestAnimFrame function return ( window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || // If all these options are unavailable, use setTimeout to call the callback function function (callback) { window.setTimeout(callback) } ) } // Initialization function to get canvas element and return related information function init(elemid) { // Get canvas element let canvas = document.getElementById(elemid) // Get 2d drawing context, note that 'd' is lowercase c = canvas.getContext('2d') // Set canvas width to window inner width and height to window inner height w = (canvas.width = window.innerWidth) h = (canvas.height = window.innerHeight) // Set fill style to semi-transparent black c.fillStyle = "rgba(30,30,30,1)" // Fill the entire canvas with the fill style c.fillRect(0, 0, w, h) // Return drawing context and canvas element return { c: c, canvas: canvas } } // Execute function when page is fully loaded window.onload = function () { // Get drawing context and canvas element let c = init("canvas").c, canvas = init("canvas").canvas, // Set canvas width to window inner width and height to window inner height w = (canvas.width = window.innerWidth), h = (canvas.height = window.innerHeight), // Initialize mouse object mouse = { x: false, y: false }, last_mouse = {} // Function to calculate distance between two points function dist(p1x, p1y, p2x, p2y) { return Math.sqrt(Math.pow(p2x - p1x, 2) + Math.pow(p2y - p1y, 2)) } // Define segment class class segment { // Constructor to initialize segment object constructor(parent, l, a, first) { // If it's the first tentacle segment, position is the tentacle top position // Otherwise, position is the nextPos coordinates of the previous segment object this.first = first if (first) { this.pos = { x: parent.x, y: parent.y, } } else { this.pos = { x: parent.nextPos.x, y: parent.nextPos.y, } } // Set segment length and angle this.l = l this.ang = a // Calculate coordinates for the next segment this.nextPos = { x: this.pos.x + this.l * Math.cos(this.ang), y: this.pos.y + this.l * Math.sin(this.ang), } } // Method to update segment position update(t) { // Calculate angle between segment and target point this.ang = Math.atan2(t.y - this.pos.y, t.x - this.pos.x) // Update position coordinates based on target point and angle this.pos.x = t.x + this.l * Math.cos(this.ang - Math.PI) this.pos.y = t.y + this.l * Math.sin(this.ang - Math.PI) // Update nextPos coordinates based on new position coordinates this.nextPos.x = this.pos.x + this.l * Math.cos(this.ang) this.nextPos.y = this.pos.y + this.l * Math.sin(this.ang) } // Method to return segment to initial position fallback(t) { // Set position coordinates to target point coordinates this.pos.x = t.x this.pos.y = t.y this.nextPos.x = this.pos.x + this.l * Math.cos(this.ang) this.nextPos.y = this.pos.y + this.l * Math.sin(this.ang) } show() { c.lineTo(this.nextPos.x, this.nextPos.y) } } // Define tentacle class class tentacle { // Constructor to initialize tentacle object constructor(x, y, l, n, a) { // Set tentacle top position coordinates this.x = x this.y = y // Set tentacle length this.l = l // Set number of tentacle segments this.n = n // Initialize tentacle target point object this.t = {} // Set random movement parameter for tentacle this.rand = Math.random() // Create first segment of the tentacle this.segments = [new segment(this, this.l / this.n, 0, true)] // Create other segments for (let i = 1; i = 0; i--) { this.segments[i].update(this.segments[i + 1].pos) } if ( dist(this.x, this.y, target.x, target.y) <p>這裡我大致梳理一下上面程式碼的流程:</p> <p><strong>初始化階段</strong></p>
- initFunction:當頁面載入時,呼叫函數init來取得canvas元素並將其寬度和高度設定為視窗的大小。所獲得的2D繪圖上下文用於後續繪圖。
- window.onload:頁面載入後,初始化canvas,並將context設定為滑鼠的初始狀態。
觸手物體的定義
- SegmentClass:這是觸手的一段。每一段都有起點(pos)、長度(l)、角度(ang),透過角度(nextPos)計算下一段的位置。
- tentacleClass:表示一個完整的觸手,由若干節組成。觸手的起點位於螢幕的中心,每個觸手包含多個部分。
觸手的主要方法有:
move:根據滑鼠位置更新每一段的位置。
show: 畫出觸手的路徑。
事件監控
- canvas.addEventListener('mousemove', ...):當滑鼠移動時,捕捉滑鼠位置並將其儲存在滑鼠變數中。每次滑鼠移動都會更新 mouse 和 last_mouse 的座標,以用於後續動畫。
動畫循環
drawFunction :這是一個用來建立動畫效果的遞歸函式。
- 首先,它在每一幀中用半透明背景填充畫布,導致先前繪製的內容逐漸消失,產生塗抹效果。
- 然後,迭代所有觸手,呼叫它們的 move 和 show 方法,更新它們的位置並繪製每一幀。
- 最後,使用 requestAnimFrame(draw) 進行連續遞歸繪製調用,形成動畫迴圈。
觸手行為
- 觸手移動的動作是透過函數實現的。觸手的最後一段先更新其位置,然後其他段依序更新。
- 觸手的繪製是透過show函數完成的,該函數會迭代所有的線段並繪製線條,最後將其顯示在螢幕上。
這樣,你就完成了電子蜘蛛的製作啦! ! !
最後我們來看看最終效果:
以上是前端刷新專案—電子蜘蛛的詳細內容。更多資訊請關注PHP中文網其他相關文章!
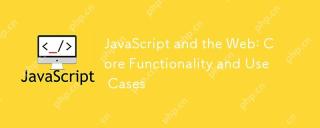
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
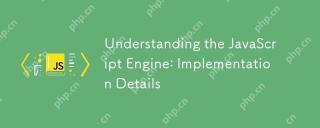
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。

Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
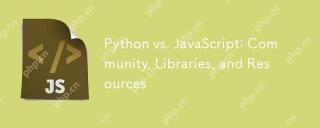
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
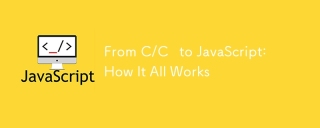
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
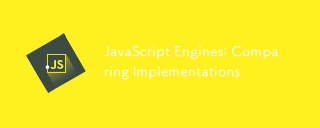
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
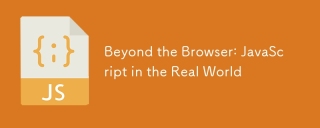
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
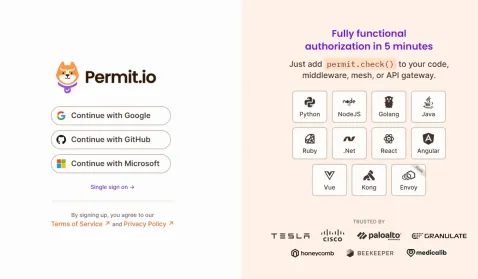
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。
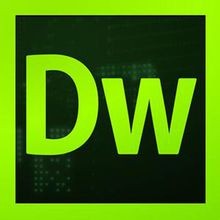
Dreamweaver CS6
視覺化網頁開發工具
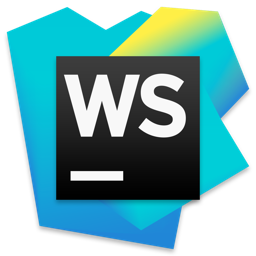
WebStorm Mac版
好用的JavaScript開發工具
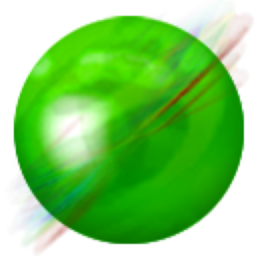
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

記事本++7.3.1
好用且免費的程式碼編輯器