理解 JavaScript 中的變數:初學者指南
歡迎回到我們的 JavaScript 世界之旅!在這篇文章中,我們將深入探討程式設計中的基本概念之一:變數。變數對於在 JavaScript 程式中儲存和操作資料至關重要。我們將介紹什麼是變數、如何宣告它們以及 JavaScript 中變數的不同類型。讓我們開始吧!
什麼是變數?
變數是儲存資料值的容器。在 JavaScript 中,您可以將變數視為保存值的盒子。您可以使用變數來儲存數字、字串、物件和其他類型的資料。變數可讓您根據需要儲存和更新值,從而使您的程式碼更加靈活和可重複使用。
宣告變數
在 JavaScript 中,您可以使用 var、let 和 const 關鍵字聲明變數。每個關鍵字都有自己的特點和用例。
1. 使用var
var 關鍵字用於宣告可以重新分配且具有函數作用域的變數。
範例:
var name = "John"; console.log(name); // Output: John name = "Jane"; console.log(name); // Output: Jane
重點:
- 可重新指派:您可以變更var變數的值。
- 函數作用域: 使用 var 宣告的變數的作用域為宣告它們的函數。
- 提升: var 變數被提升到其作用域的頂部,這意味著您可以在聲明它們之前使用它們。但是,在遇到實際聲明之前,它們將未定義。
2. 使用 let
let 關鍵字用於聲明可以重新分配且具有區塊作用域的變數。
範例:
let age = 30; console.log(age); // Output: 30 age = 35; console.log(age); // Output: 35
重點:
- 可重新指派:您可以變更let變數的值。
- 區塊作用域: 用 let 宣告的變數的作用域為宣告它們的區塊(例如,在 {} 內)。
- 不提升: let 變數不會提升到其作用域的頂端。在聲明之前您不能使用它們。
3. 使用 const
const關鍵字用於聲明不能重新賦值且具有區塊作用域的變數。
範例:
const pi = 3.14; console.log(pi); // Output: 3.14 // pi = 3.15; // This will cause an error because `const` variables cannot be reassigned.
重點:
- 無法重新指派: 您無法變更 const 變數的值。
- 區塊作用域: 用 const 宣告的變數的作用域為宣告它們的區塊。
- 不提升: const 變數不會提升到其作用域的頂端。在聲明之前您不能使用它們。
命名變數
命名變數時,使用描述性且有意義的名稱很重要。這使您的程式碼更具可讀性和更容易理解。
命名變數的最佳實踐:
- 使用駝峰式命名法: 變數名稱應採用駝峰式命名法,其中第一個字母小寫,後續每個單字以大寫字母開頭(例如,userName、 totalPrice).
- 具有描述性: 選擇能夠清楚描述變數用途的名稱(例如,userAge 而不是 a)。
- 避免保留字: 不要使用 JavaScript 保留字作為變數名稱(例如,let、const、var)。
範例:
let userName = "John"; let totalPrice = 100; let isLoggedIn = true;
變數類型
JavaScript 是一種動態類型語言,這表示您在宣告變數時無需指定變數的類型。類型是在運行時根據分配給變數的值確定的。
Common Variable Types:
- Number: Represents numeric values (e.g., let age = 30;).
- String: Represents text values (e.g., let name = "John";).
- Boolean: Represents true or false values (e.g., let isStudent = true;).
- Object: Represents complex data structures (e.g., let person = { name: "John", age: 30 };).
- Array: Represents a list of values (e.g., let fruits = ["apple", "banana", "cherry"];).
- Null: Represents the intentional absence of any object value (e.g., let empty = null;).
- Undefined: Represents a variable that has been declared but not assigned a value (e.g., let x;).
Example:
let age = 30; // Number let name = "John"; // String let isStudent = true; // Boolean let person = { name: "John", age: 30 }; // Object let fruits = ["apple", "banana", "cherry"]; // Array let empty = null; // Null let x; // Undefined
Conclusion
Understanding variables is a crucial step in learning JavaScript. Variables allow you to store and manipulate data, making your code more dynamic and flexible. By using the var, let, and const keywords, you can declare variables with different scopes and behaviors. Remember to use meaningful and descriptive names for your variables to make your code more readable.
In the next blog post, we'll dive deeper into JavaScript data types and explore how to work with numbers, strings, and other types of data. Stay tuned as we continue our journey into the world of JavaScript!
以上是了解 JavaScript 中的變數:初學者指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!
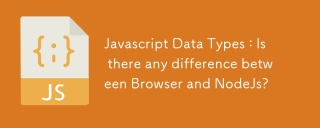
JavaScript核心數據類型在瀏覽器和Node.js中一致,但處理方式和額外類型有所不同。 1)全局對像在瀏覽器中為window,在Node.js中為global。 2)Node.js獨有Buffer對象,用於處理二進制數據。 3)性能和時間處理在兩者間也有差異,需根據環境調整代碼。
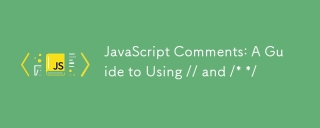
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
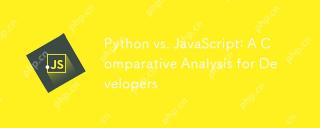
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
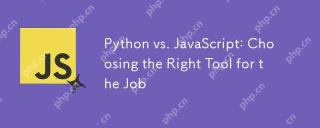
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
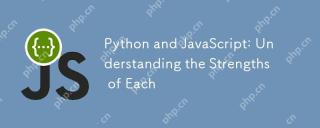
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
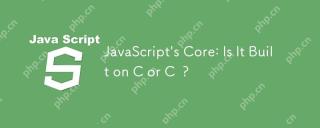
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
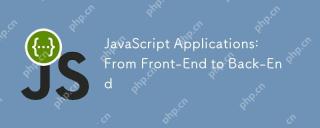
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
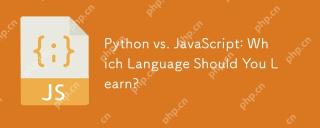
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
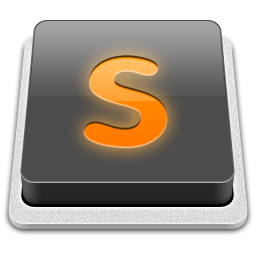
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。