讓我們建立應用程式的幾個元件。
在這種情況下,我們需要以下 UI:
- 手風琴 - 手風琴;
- autocomplete — 自動完成輸入;
- 按鈕 - 按鈕;
- 卡片 - 卡片;
- 複選框 - 複選框;
- 容器 - 內容居中;
- datepicker - 日期選擇器;
- 對話框 - 模態視窗;
- 標題 - 促銷文字;
- 圖示 — svg 圖示集;
- 輸入 - 輸入;
- label - 標籤;
- 佈局-佈局;
- 導航 - 選單;
- 部分 — 內容中各部分的後台任務;
- 標題 - 標題。
複雜元素將使用 Angular/cdk(對話框、手風琴)來簡化過程。
所以讓我們加入包裝:
yarn add -D @angular/cdk
還有一個 scss 的小魔法:
{ "inlineStyleLanguage": "scss", "stylePreprocessorOptions": { "includePaths": ["node_modules", "./"] } }
創建實用程式
向 ui 新增新的 utils 目錄:
mkdir src/app/ui/utils mkdir src/app/ui/utils/lib echo >src/app/ui/utils/index.ts
在 tsconfig.json 中我們將寫別名:
{ "paths": { "@baf/ui/utils": ["src/app/ui/utils/index.ts"] } }
讓我們在 types.ts 中定義幾種類型:
export type ButtonMode = 'primary' | 'secondary' | 'tertiary' | undefined; export type Size = 'small' | 'medium' | 'large' | undefined; export type Align = 'left' | 'center' | 'right' | undefined; export type ExtraSize = 'xsmall' | 'small' | 'medium' | 'large' | 'xlarge' | undefined; export type Width = 'max' | 'initial' | undefined;
它們都是可選的,因此它們包含未定義的。
組件的一些常見屬性:
- 對齊 - 左、右居中;
- 停用 - 停用狀態(與表單相關);
- 尺寸、額外尺寸 - 文字尺寸小、中、大
- mode - 按鈕類型;
- width - 元素寬度。
對齊指令
對齊的範例實作:
import { Directive, inject, input } from '@angular/core'; import { ExtraClassService, toClass } from '@baf/core'; import type { Align } from './types'; @Directive({ selector: '[bafAlign]', standalone: true, providers: [ExtraClassService], }) export class AlignDirective { private readonly extraClassService = inject(ExtraClassService); readonly align = input<align align>(undefined, { alias: 'bafAlign', transform: (value) => { this.extraClassService.update('align', toClass(value, 'align')); return value; }, }); } </align>
ExtraClassService - 新增對應類別的服務。
作為替代方案,您可以使用 @HostBinding('class.align-center') 或透過主機設定規則。
由於 Angular 不允許您在指令中包含樣式,因此我們新增一個需要為每個元件匯入的 mixin。
在src/stylesheets建立檔案align.scss:
@mixin make-align() { &.align-left { text-align: left; } &.align-center { text-align: center; } &.align-right { text-align: right; } }
使用範例:
@use 'src/stylesheets/align' as align; :host { @include align.make-align(); }
其餘指令類似。
容器
新增容器並輸入別名。
mkdir src/app/ui/container mkdir src/app/ui/container/lib echo >src/app/ui/container/index.ts
生成組件:
yarn ng g c container
將其移至 src/app/ui/container/lib 並編輯 ContainerComponent:
import { ChangeDetectionStrategy, Component } from '@angular/core'; import { RouterOutlet } from '@angular/router'; import { AlignDirective } from '@baf/ui/utils'; import { FluidDirective } from './fluid.directive'; import { MobileDirective } from './mobile.directive'; @Component({ selector: 'baf-container', standalone: true, imports: [RouterOutlet], template: '<ng-content></ng-content>', styleUrl: './container.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-container', }, hostDirectives: [ { directive: FluidDirective, inputs: ['bafFluid'], }, { directive: MobileDirective, inputs: ['bafMobile'], }, { directive: AlignDirective, inputs: ['bafAlign'], }, ], }) export class ContainerComponent {}
新增樣式:
@use 'src/stylesheets/align' as align; @use 'src/stylesheets/device' as device; :host { display: flex; flex-direction: column; margin-left: auto; margin-right: auto; width: 100%; &.fluid { max-width: 100%; } &:not(.mobile-no-gutter) { padding-left: 1rem; padding-right: 1rem; } @include align.make-align(); @include device.media-tablet-portrait() { &:not(.fluid) { max-width: 788px; } } @include device.media-tablet-landscape() { &:not(.fluid) { max-width: 928px; } } @include device.media-web-portrait() { &:not(.fluid) { max-width: 808px; } } @include device.media-web-landscape() { &:not(.fluid) { max-width: 1200px; } } }
取自材質的寬度混合:
@mixin media-handset() { @media (max-width: 599.98px) and (orientation: portrait), (max-width: 959.98px) and (orientation: landscape) { @content; } } @mixin media-handset-up() { @media (min-width: 0) and (orientation: portrait), (min-width: 0) and (orientation: landscape) { @content; } } @mixin media-handset-portrait() { @media (max-width: 599.98px) and (orientation: portrait) { @content; } } @mixin media-handset-landscape() { @media (max-width: 959.98px) and (orientation: landscape) { @content; } } @mixin media-tablet() { @media (min-width: 600px) and (max-width: 839.98px) and (orientation: portrait), (min-width: 960px) and (max-width: 1279.98px) and (orientation: landscape) { @content; } } @mixin media-tablet-up() { @media (min-width: 600px) and (orientation: portrait), (min-width: 960px) and (orientation: landscape) { @content; } } @mixin media-tablet-landscape() { @media (min-width: 960px) and (max-width: 1279.98px) and (orientation: landscape) { @content; } } @mixin media-tablet-portrait() { @media (min-width: 600px) and (max-width: 839.98px) and (orientation: portrait) { @content; } } @mixin media-web() { @media (min-width: 840px) and (orientation: portrait), (min-width: 1280px) and (orientation: landscape) { @content; } } @mixin media-web-up() { @media (min-width: 840px) and (orientation: portrait), (min-width: 1280px) and (orientation: landscape) { @content; } } @mixin media-web-portrait() { @media (min-width: 840px) and (orientation: portrait) { @content; } } @mixin media-web-landscape() { @media (min-width: 1280px) and (orientation: landscape) { @content; } }
我們也會建立兩個指令 FluidDirective 和 MobileDirective:
import { coerceBooleanProperty } from '@angular/cdk/coercion'; import { Directive, inject, input } from '@angular/core'; import type { CoerceBoolean } from '@baf/core'; import { ExtraClassService } from '@baf/core'; @Directive({ selector: 'baf-container[bafFluid]', standalone: true, providers: [ExtraClassService], }) export class FluidDirective { private readonly extraClassService = inject(ExtraClassService); readonly fluid = input<coerceboolean coerceboolean>(undefined, { alias: 'bafFluid', transform: (value) => { this.extraClassService.patch('fluid', coerceBooleanProperty(value)); return value; }, }); } </coerceboolean>
import { coerceBooleanProperty } from '@angular/cdk/coercion'; import { Directive, inject, input } from '@angular/core'; import type { CoerceBoolean } from '@baf/core'; import { ExtraClassService } from '@baf/core'; @Directive({ selector: 'baf-container[bafMobile]', standalone: true, providers: [ExtraClassService], }) export class MobileDirective { private readonly extraClassService = inject(ExtraClassService); readonly mobile = input<coerceboolean coerceboolean>(undefined, { alias: 'bafMobile', transform: (value) => { this.extraClassService.patch('mobile-no-gutter', coerceBooleanProperty(value)); return value; }, }); } </coerceboolean>
標題、標籤、標題、部分和卡片
新增標題並輸入別名:
mkdir src/app/ui/title mkdir src/app/ui/title/lib echo >src/app/ui/title/index.ts
生成組件:
yarn ng g c title
將其移至 src/app/ui/title/lib 並編輯 TitleComponent:
import { ChangeDetectionStrategy, Component } from '@angular/core'; import { AlignDirective, SizeDirective } from '@baf/ui/utils'; @Component({ selector: 'baf-title,[baf-title],[bafTitle]', standalone: true, template: '<ng-content></ng-content>', styleUrl: './title.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-title', }, hostDirectives: [ { directive: SizeDirective, inputs: ['bafSize'], }, { directive: AlignDirective, inputs: ['bafAlign'], }, ], }) export class TitleComponent {}
一些款式:
@use 'src/stylesheets/align' as align; @use 'src/stylesheets/size' as size; @use 'src/stylesheets/typography' as typography; :host { @include size.make-size() using ($size) { @if $size == small { @include typography.title-small(); } @else if $size == medium { @include typography.title-medium(); } @else if $size == large { @include typography.title-large(); } } @include align.make-align(); }
剩下的我們以同樣的方式進行。
按鈕
讓我們繼續創建更複雜的小部件。
新增按鈕並輸入別名:
mkdir src/app/ui/buttons mkdir src/app/ui/buttons/lib echo >src/app/ui/buttons/index.ts
由於需要多種類型的按鈕,我們將設定基本類型 -ButtonBase 和 AnchorBase:
import type { FocusOrigin } from '@angular/cdk/a11y'; import { FocusMonitor } from '@angular/cdk/a11y'; import { coerceBooleanProperty } from '@angular/cdk/coercion'; import type { AfterViewInit, OnDestroy, OnInit } from '@angular/core'; import { Directive, ElementRef, inject, NgZone } from '@angular/core'; @Directive() export class ButtonBase implements AfterViewInit, OnDestroy { protected readonly elementRef = inject(ElementRef); private isDisabled = false; private readonly focusMonitor = inject(FocusMonitor); get disabled(): boolean { return this.isDisabled; } set disabled(value: string | boolean | null | undefined) { const disabled = coerceBooleanProperty(value); if (disabled !== this.isDisabled) { this.isDisabled = disabled; } } ngAfterViewInit() { this.focusMonitor.monitor(this.elementRef, true); } ngOnDestroy() { this.focusMonitor.stopMonitoring(this.elementRef); } focus(origin: FocusOrigin = 'program', options?: FocusOptions): void { if (origin) { this.focusMonitor.focusVia(this.elementRef.nativeElement, origin, options); } else { this.elementRef.nativeElement.focus(options); } } } @Directive() export class AnchorBase extends ButtonBase implements OnInit, OnDestroy { private readonly ngZone = inject(NgZone); protected readonly haltDisabledEvents = (event: Event) => { if (this.disabled) { event.preventDefault(); event.stopImmediatePropagation(); } }; ngOnInit(): void { this.ngZone.runOutsideAngular(() => { this.elementRef.nativeElement.addEventListener('click', this.haltDisabledEvents); }); } override ngOnDestroy(): void { super.ngOnDestroy(); this.elementRef.nativeElement.removeEventListener('click', this.haltDisabledEvents); } }
實作取自 Angular Material 2。
我還沒看過 Angular 中的 Material 3,我認為不值得。組件的複雜度略大於無窮大。
從範例中可以看到,定義了停用狀態以及焦點觀察者。
讓我們建立一個簡單的按鈕。在我們的例子中,這是標準包裝的包裝。
模板 -
import { ChangeDetectionStrategy, Component } from '@angular/core'; import { DisabledDirective, ExtraSizeDirective, ModeDirective, WidthDirective } from '@baf/ui/utils'; import { AnchorBase, ButtonBase } from '../base/button-base'; @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'button[baf-button]', standalone: true, template: '<ng-content></ng-content>', styleUrl: './button.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-button', }, hostDirectives: [ { directive: ModeDirective, inputs: ['bafMode'], }, { directive: ExtraSizeDirective, inputs: ['bafSize'], }, { directive: DisabledDirective, inputs: ['disabled'], }, { directive: WidthDirective, inputs: ['bafWidth'], }, ], }) export class ButtonComponent extends ButtonBase {} @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'a[baf-button]', standalone: true, template: '<ng-content></ng-content>', styleUrls: ['./button.component.scss'], changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-button', }, hostDirectives: [ { directive: ModeDirective, inputs: ['bafMode'], }, { directive: ExtraSizeDirective, inputs: ['bafSize'], }, { directive: DisabledDirective, inputs: ['disabled'], }, { directive: WidthDirective, inputs: ['bafWidth'], }, ], }) export class AnchorComponent extends AnchorBase {}
В компоненте определены общие директивы, в которые и вынесена вся логика.
Немного SCSS:
@use 'src/stylesheets/button' as button; @use 'src/stylesheets/width' as width; :host { display: inline-flex; flex-direction: row; align-items: center; justify-content: center; padding: 0.5rem 1.5rem; border: none; box-shadow: none; border-radius: 3px; cursor: pointer; text-decoration: none; &.mode-primary { @include button.mode(--md-sys-color-primary-container, --md-sys-color-on-primary, --md-sys-color-primary); } &.mode-secondary { @include button.mode(--md-sys-color-secondary-container, --md-sys-color-on-secondary, --md-sys-color-secondary); } &.mode-tertiary { @include button.mode(--md-sys-color-tertiary-container, --md-sys-color-on-tertiary, --md-sys-color-tertiary); } @include button.disabled(); @include button.sizes(); @include width.make-width(); }
Теперь создадим icon-button.
Макет:
<span class="icon-content"> <ng-content></ng-content> </span> <span class="state-layer"></span>
import { ChangeDetectionStrategy, Component } from '@angular/core'; import { DisabledDirective, ExtraSizeDirective, ModeDirective } from '@baf/ui/utils'; import { AnchorBase, ButtonBase } from '../base/button-base'; @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'button[baf-icon-button]', standalone: true, templateUrl: './icon-button.component.html', styleUrl: './icon-button.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-icon-button', }, hostDirectives: [ { directive: ModeDirective, inputs: ['bafMode'], }, { directive: ExtraSizeDirective, inputs: ['bafSize'], }, { directive: DisabledDirective, inputs: ['disabled'], }, ], }) export class IconButtonComponent extends ButtonBase {} @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'a[baf-icon-button]', standalone: true, templateUrl: './icon-button.component.html', styleUrl: './icon-button.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-icon-button', }, hostDirectives: [ { directive: ModeDirective, inputs: ['bafMode'], }, { directive: ExtraSizeDirective, inputs: ['bafSize'], }, { directive: DisabledDirective, inputs: ['disabled'], }, ], }) export class IconAnchorComponent extends AnchorBase {}
Стилизуем кнопки:
:host { display: inline-flex; flex-direction: row; flex-wrap: nowrap; align-items: center; justify-content: center; height: 48px; width: 48px; padding: 4px; border: none; z-index: 0; gap: 8px; white-space: nowrap; user-select: none; background-color: transparent; text-decoration: none; cursor: pointer; border-radius: var(--md-sys-shape-corner-full); position: relative; } .state-layer { position: absolute; width: 100%; height: 100%; left: 0; top: 0; right: 0; bottom: 0; display: block; z-index: 1; opacity: 0; border-radius: inherit; } .icon-content { display: flex; align-items: center; justify-content: center; color: var(--md-sys-color-on-surface-variant); fill: var(--md-sys-color-on-surface-variant); line-height: 1; :host:hover & { color: var(--md-sys-color-on-surface); fill: var(--md-sys-color-on-surface); } }
Icons
Добавим компонент для иконок.
mkdir src/app/ui/icons mkdir src/app/ui/icons/lib echo >src/app/ui/icons/index.ts
Запускаем команду:
yarn ng g c icon
Переносим его в src/app/ui/title/lib и отредактируем IconComponent:
import { ChangeDetectionStrategy, Component } from '@angular/core'; @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'svg[baf-icon]', standalone: true, imports: [], templateUrl: './icon.component.html', styleUrl: './icon.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, }) export class IconComponent {}
Стили:
src/app/ui/icons/lib/icon/icon.component.scss
Пример использования на иконке домой:
<svg baf-icon xmlns="http://www.w3.org/2000/svg" height="24px" viewbox="0 -960 960 960" width="24px"> <path d="M240-200h120v-240h240v240h120v-360L480-740 240-560v360Zm-80 80v-480l320-240 320 240v480H520v-240h-80v240H160Zm320-350Z"></path> </svg>
import { ChangeDetectionStrategy, Component } from '@angular/core'; import { IconComponent } from '../icon/icon.component'; @Component({ selector: 'baf-icon-home', standalone: true, imports: [IconComponent], templateUrl: './home.component.html', styleUrl: './home.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, }) export class HomeComponent {}
Так же созданы все остальные иконки:
- arrow-down - стрелка вниз;
- arrow-up - стрелка вверх;
- chevron-left - стрелка влево;
- chevron-right - стрелка вправо;
- home - дом;
- logo - лого;
- star - звезда;
- sync-alt - рефреш.
Accordion
Реализуем аккордеон:
mkdir src/app/ui/accordion mkdir src/app/ui/accordion/lib echo >src/app/ui/accordion/index.ts
Запускаем команду:
yarn ng g c accordion
Добавим интерфейс:
export interface AccordionItem { readonly title: string; readonly description: string; }
В компоненте будем выводить список элементов:
import { CdkAccordionModule } from '@angular/cdk/accordion'; import { ChangeDetectionStrategy, Component, input } from '@angular/core'; import { ArrowDownComponent, ArrowUpComponent } from '@baf/ui/icons'; export interface AccordionItem { readonly title: string; readonly description: string; } @Component({ selector: 'baf-accordion', standalone: true, imports: [CdkAccordionModule, ArrowDownComponent, ArrowUpComponent], templateUrl: './accordion.component.html', styleUrl: './accordion.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, }) export class AccordionComponent { readonly items = input.required<accordionitem>(); } </accordionitem>
Шаблон:
<cdk-accordion> @for (item of items(); track item.title; let index = $index) { <cdk-accordion-item class="accordion" role="button" tabindex="0" index> <div class="accordion-header"> @if (accordionItem.expanded) { <baf-arrow-down></baf-arrow-down> } @else { <baf-arrow-up></baf-arrow-up> } <span> {{ item.title }} </span> </div> <div class="accordion-body" role="region" : index> {{ item.description }} </div> </cdk-accordion-item> } </cdk-accordion>
Стили:
.accordion { display: block; &:not(:last-child) { border-bottom: 1px solid var(--md-sys-color-surface-variant); padding-bottom: 1rem; margin-bottom: 1rem; } } .accordion-header { display: flex; flex-direction: row; align-items: center; gap: 0.5rem; cursor: pointer; line-height: 1; user-select: none; } .accordion-body { padding: 1rem 1rem 0 2rem; }
Checkbox
Создадим чекбокс.
Отмечу, что оформление я взял из проекта мериалайз
mkdir src/app/ui/checkbox mkdir src/app/ui/checkbox/lib echo >src/app/ui/checkbox/index.ts
Запускаем команду:
yarn ng g c checkbox
Разметка:
<label> <input type="checkbox"> <span><ng-content></ng-content></span> </label>
Украду немного стилей из Material CSS:
[type='checkbox']:not(:checked), [type='checkbox']:checked { position: absolute; opacity: 0; pointer-events: none; } [type='checkbox']:checked { + span:before { top: -4px; left: -5px; width: 12px; height: 22px; border-top: 2px solid transparent; border-left: 2px solid transparent; border-right: 2px solid var(--md-sys-color-primary); border-bottom: 2px solid var(--md-sys-color-primary); transform: rotate(40deg); backface-visibility: hidden; transform-origin: 100% 100%; } &:disabled + span:before { border-right: 2px solid var(--md-sys-color-shadow); border-bottom: 2px solid var(--md-sys-color-shadow); } } [type='checkbox'] { + span { position: relative; padding-left: 35px; cursor: pointer; display: inline-block; height: 25px; line-height: 25px; font-size: 1rem; user-select: none; } &:not(:checked):disabled + span:before { border: none; background-color: var(--md-sys-color-shadow); } // General + span:after { border-radius: 2px; } + span:before, + span:after { content: ''; left: 0; position: absolute; /* .1s delay is for check animation */ transition: border 0.25s, background-color 0.25s, width 0.2s 0.1s, height 0.2s 0.1s, top 0.2s 0.1s, left 0.2s 0.1s; z-index: 1; } // Unchecked style &:not(:checked) + span:before { width: 0; height: 0; border: 3px solid transparent; left: 6px; top: 10px; transform: rotateZ(37deg); transform-origin: 100% 100%; } &:not(:checked) + span:after { height: 20px; width: 20px; background-color: transparent; border: 2px solid var(--md-sys-color-outline); top: 0; z-index: 0; } // Checked style &:checked { + span:before { top: 0; left: 1px; width: 8px; height: 13px; border-top: 2px solid transparent; border-left: 2px solid transparent; border-right: 2px solid var(--md-sys-color-on-primary); border-bottom: 2px solid var(--md-sys-color-on-primary); transform: rotateZ(37deg); transform-origin: 100% 100%; } + span:after { top: 0; width: 20px; height: 20px; border: 2px solid var(--md-sys-color-primary); background-color: var(--md-sys-color-primary); z-index: 0; } } // Disabled style &:disabled:not(:checked) + span:before { background-color: transparent; border: 2px solid transparent; } &:disabled:not(:checked) + span:after { border-color: transparent; background-color: var(--md-sys-color-outline); } &:disabled:checked + span:before { background-color: transparent; } &:disabled:checked + span:after { background-color: var(--md-sys-color-outline); border-color: var(--md-sys-color-outline); } }
Сам компонент:
import { ChangeDetectionStrategy, Component, input } from '@angular/core'; import type { FormControl } from '@angular/forms'; import { ReactiveFormsModule } from '@angular/forms'; export interface CheckboxOptions { readonly [key: string]: unknown; readonly name?: string; } @Component({ selector: 'baf-checkbox', standalone: true, imports: [ReactiveFormsModule], templateUrl: './checkbox.component.html', styleUrl: './checkbox.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, }) export class CheckboxComponent { readonly control = input.required<formcontrol>>(); readonly options = input<checkboxoptions>({}); } </checkboxoptions></formcontrol>
Input
Реализуем инпут:
mkdir src/app/ui/input mkdir src/app/ui/input/lib echo >src/app/ui/input/index.ts
Выполним инструкцию:
yarn ng g c input
InputComponent будет оберткой над input.
import { ChangeDetectionStrategy, Component, ElementRef, inject } from '@angular/core'; import { NgControl } from '@angular/forms'; @Component({ // eslint-disable-next-line @angular-eslint/component-selector selector: 'input[baf-input]', standalone: true, imports: [], template: '<ng-content></ng-content>', styleUrl: './input.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-input', }, }) export class InputComponent { readonly elementRef: ElementRef<htmlinputelement> = inject(ElementRef); readonly ngControl = inject(NgControl); } </htmlinputelement>
Добавим SCSS:
:host { display: block; background-color: transparent; height: 100%; width: 100%; padding: 0; border: none; outline: none; &:hover, &:focus, &:active { outline: none; } &::placeholder { color: var(--md-sys-color-on-surface-variant); } :host-context(.is-invalid) { color: var(--md-sys-color-error); } }
Перенесем концепты из mat-form-field:
yarn ng g c input-control
Разметка и стили:
<div class="input-container"> <ng-content select="[baf-input-prefix]"></ng-content> <div class="input-box"> <ng-content select="label[baf-label],baf-label"></ng-content> <div class="input"> <ng-content select="input[baf-input],baf-input"></ng-content> </div> </div> <ng-content select="[baf-input-suffix]"></ng-content> </div> <ng-content></ng-content>
:host { display: flex; flex-direction: column; position: relative; width: 100%; &.is-disabled { cursor: not-allowed; pointer-events: none; color: rgba(var(--md-sys-color-on-surface-rgba), 0.38); .input { color: rgba(var(--md-sys-color-on-surface-rgba), 0.38); } } &.is-pressed, &.is-value { .input { opacity: 1; } } } .input-box { position: relative; margin: 0 16px; justify-content: center; height: 100%; flex-grow: 1; } .input-container { display: flex; flex-direction: row; flex-wrap: nowrap; align-items: center; background-color: var(--md-sys-color-surface-variant); color: var(--md-sys-color-on-surface-variant); border-radius: var(--md-sys-shape-corner-extra-small-top); height: 3rem; } .input { opacity: 0; height: 100%; width: 100%; position: relative; z-index: 2; transition: opacity 0.1s; padding: 12px 0 0 0; }
Возможно можно и разбить на несколько дочерних компонентов, но и так получается достаточно сложно.
Используемые вспомогательные директивы для префиксов и прочего.
src/app/ui/input/lib/input-display.directive.ts:
import { Directive, ElementRef, forwardRef, inject, input } from '@angular/core'; import type { ControlValueAccessor } from '@angular/forms'; import { NG_VALUE_ACCESSOR } from '@angular/forms'; import type { ChangeFn, DisplayFn, TouchedFn } from '@baf/core'; @Directive({ selector: 'input[formControlName][bafInputDisplay],input[formControl][bafInputDisplay]', standalone: true, providers: [ { provide: NG_VALUE_ACCESSOR, useExisting: forwardRef(() => InputDisplayDirective), multi: true, }, ], host: { '(blur)': 'onTouched()', '(input)': 'onInput($event)', }, }) export class InputDisplayDirective implements ControlValueAccessor { private readonly elementRef = inject(ElementRef<htmlinputelement>); readonly display = input.required<displayfn>({ alias: 'bafInputDisplay' }); onChange!: ChangeFn; onTouched!: TouchedFn; registerOnChange(fn: ChangeFn): void { this.onChange = fn; } registerOnTouched(fn: TouchedFn): void { this.onTouched = fn; } writeValue(value: unknown): void { this.elementRef.nativeElement.value = this.display()(value); } onInput(event: Event): void { const { value } = event.target as HTMLInputElement; this.elementRef.nativeElement.value = this.display()(value); this.onChange(value); } } </displayfn></htmlinputelement>
src/app/ui/input/lib/input-mask.directive.ts:
import type { OnInit } from '@angular/core'; import { Directive, ElementRef, forwardRef, inject, InjectionToken, input } from '@angular/core'; import type { ControlValueAccessor } from '@angular/forms'; import { NG_VALUE_ACCESSOR } from '@angular/forms'; import type { ChangeFn, MaskFn, TouchedFn } from '@baf/core'; export const INPUT_MASK_VALUES = new InjectionToken<record regexp>>('INPUT_MASK_VALUES'); const DEFAULT_INPUT_MASK_VALUES: Record<string regexp> = { 0: /[0-9]/, a: /[a-z]/, A: /[A-Z]/, B: /[a-zA-Z]/ }; export const DEFAULT_MASK_FN: MaskFn = (value) => value; @Directive({ selector: 'input[formControlName][bafInputMask],input[formControl][bafInputMask]', standalone: true, providers: [ { provide: NG_VALUE_ACCESSOR, useExisting: forwardRef(() => InputMaskDirective), multi: true, }, ], host: { '(blur)': 'onTouched()', '(input)': 'onInput($event)', }, }) export class InputMaskDirective implements ControlValueAccessor, OnInit { private readonly maskValues = inject(INPUT_MASK_VALUES, { optional: true }) ?? DEFAULT_INPUT_MASK_VALUES; private readonly elementRef = inject(ElementRef<htmlinputelement>); private lastValue?: string; private readonly maskFormats = `(${Object.keys(this.maskValues) .map((key) => { const regexStr = this.maskValues[key].toString(); return regexStr.substring(1, regexStr.length - 1); }) .join('|')})`; readonly mask = input.required<string>({ alias: 'bafInputMask' }); readonly maskFrom = input<maskfn>(DEFAULT_MASK_FN, { alias: 'bafInputMaskFrom' }); readonly maskTo = input<maskfn>(DEFAULT_MASK_FN, { alias: 'bafInputMaskTo' }); onChange!: ChangeFn; onTouched!: TouchedFn; registerOnChange(fn: ChangeFn): void { this.onChange = fn; } registerOnTouched(fn: TouchedFn): void { this.onTouched = fn; } writeValue(value: string | undefined | null): void { this.elementRef.nativeElement.value = this.getMaskedValue(this.maskTo()(value)); } onInput(event: Event): void { const { value } = event.target as HTMLInputElement; const masked = this.getMaskedValue(value); this.elementRef.nativeElement.value = masked; this.onChange(this.maskFrom()(masked)); } ngOnInit(): void { if (!this.mask()) { console.warn(`Property mask should not be empty for input:`, this.elementRef.nativeElement); } } getMaskedValue(value: string | undefined | null): string | undefined | null { if (!this.mask() || !value || value === this.lastValue) { return value; } const masked = this.valueToFormat(value, this.mask(), this.lastValue ? this.lastValue.length > value.length : false, this.lastValue); this.lastValue = masked; return masked; } /** * @see https://gist.github.com/rami-alloush/3ee792fd0647b73de5f863a2719c78c6 */ private valueToFormat(value: string, format: string, goingBack?: boolean, prevValue?: string): string { let maskedValue = ''; const unmaskedValue = value.replace(' ', '').match(new RegExp(this.maskFormats, 'g'))?.join('') ?? ''; const formats = new RegExp(this.maskFormats); const isLastCharFormatter = !formats.test(value[value.length - 1]); const isPrevLastCharFormatter = prevValue && !formats.test(prevValue[prevValue.length - 1]); let formatOffset = 0; for (let index = 0, max = Math.min(unmaskedValue.length, format.length); index <p><strong>src/app/ui/input/lib/input-prefix.directive.ts</strong>:<br> </p> <pre class="brush:php;toolbar:false">import { Directive } from '@angular/core'; @Directive({ selector: '[bafInputPrefix]', standalone: true, host: { class: 'input-prefix', '[style.margin-left]': '"12px"', }, }) export class InputPrefixDirective {}
src/app/ui/input/lib/input-suffix.directive.ts:
import { Directive } from '@angular/core'; @Directive({ selector: '[bafInputSuffix]', standalone: true, host: { class: 'baf-input-suffix', '[style.margin-right]': '"12px"', }, }) export class InputSuffixDirective {}
Логика работы достаточно проста:
import type { AfterViewInit, OnDestroy } from '@angular/core'; import { ChangeDetectionStrategy, Component, contentChild, DestroyRef, ElementRef, inject, Renderer2 } from '@angular/core'; import { takeUntilDestroyed } from '@angular/core/rxjs-interop'; import type { FormControlStatus } from '@angular/forms'; import { TouchedChangeEvent } from '@angular/forms'; import { filter, startWith, tap } from 'rxjs'; import { LabelComponent } from '@baf/ui/label'; import { InputComponent } from './input.component'; @Component({ selector: 'baf-input-control', templateUrl: './input-control.component.html', styleUrls: ['./input-control.component.scss'], changeDetection: ChangeDetectionStrategy.OnPush, standalone: true, host: { class: 'baf-input-control', }, }) export class InputControlComponent implements AfterViewInit, OnDestroy { readonly destroyRef = inject(DestroyRef); readonly elementRef: ElementRef<htmlinputelement> = inject(ElementRef); readonly renderer = inject(Renderer2); readonly label = contentChild<labelcomponent>(LabelComponent); readonly input = contentChild.required<inputcomponent>(InputComponent); private isDisabled = false; ngAfterViewInit(): void { const input = this.input(); if (!input) { console.warn('Input[baf-input] not found. Add child <input baf-input> in <baf-input-control></baf-input-control>'); return; } input.elementRef.nativeElement.addEventListener('click', this.onFocusin); input.elementRef.nativeElement.addEventListener('focusout', this.onFocusout); input.elementRef.nativeElement.addEventListener('input', this.onInput); input.elementRef.nativeElement.addEventListener('change', this.onInput); this.onInput({ target: input.elementRef.nativeElement }); input.ngControl.control?.events .pipe( filter((event) => event instanceof TouchedChangeEvent), tap(() => this.check()), takeUntilDestroyed(this.destroyRef), ) .subscribe(); input.ngControl.valueChanges ?.pipe( tap(() => { if (!input.ngControl.value && this.elementRef.nativeElement.classList.contains('is-value')) { this.renderer.removeClass(this.elementRef.nativeElement, 'is-value'); } this.onInput({ target: input.elementRef.nativeElement }); }), takeUntilDestroyed(this.destroyRef), ) .subscribe(); input.ngControl.statusChanges ?.pipe( startWith(input.ngControl.status), tap((status: FormControlStatus) => { this.isDisabled = status === 'DISABLED'; this.disable(); }), takeUntilDestroyed(this.destroyRef), ) .subscribe(); } ngOnDestroy(): void { const input = this.input(); if (!input) { return; } input.elementRef.nativeElement.removeEventListener('click', this.onFocusin); input.elementRef.nativeElement.removeEventListener('focusout', this.onFocusout); input.elementRef.nativeElement.removeEventListener('input', this.onInput); input.elementRef.nativeElement.removeEventListener('change', this.onInput); } private onFocusin = () => { if (!this.isDisabled) { this.renderer.addClass(this.elementRef.nativeElement, 'is-pressed'); } }; private onFocusout = () => { if (!this.isDisabled) { this.renderer.removeClass(this.elementRef.nativeElement, 'is-pressed'); } this.check(); }; private onInput = (event: Event | { target: HTMLInputElement }) => { if (!this.isDisabled) { const target = event.target as HTMLInputElement; if (target.value?.length > 0) { this.renderer.addClass(this.elementRef.nativeElement, 'is-value'); } else { this.renderer.removeClass(this.elementRef.nativeElement, 'is-value'); } this.check(); } }; private disable(): void { if (this.isDisabled) { this.renderer.addClass(this.elementRef.nativeElement, 'is-disabled'); } else { this.renderer.removeClass(this.elementRef.nativeElement, 'is-disabled'); } } private check(): void { if (this.input().ngControl.touched) { if (this.input().ngControl.errors) { this.renderer.addClass(this.elementRef.nativeElement, 'is-invalid'); } else { this.renderer.removeClass(this.elementRef.nativeElement, 'is-invalid'); } } } } </inputcomponent></labelcomponent></htmlinputelement>
Так как input является потомком, ищем его после рендера и добавляем обработчики:
input.elementRef.nativeElement.addEventListener('click', this.onFocusin); input.elementRef.nativeElement.addEventListener('focusout', this.onFocusout); input.elementRef.nativeElement.addEventListener('input', this.onInput); input.elementRef.nativeElement.addEventListener('change', this.onInput);
Листенеры:
- onFocusin - фосус;
- onFocusout - блюр;
- onInput - ввод значения в input.
Также подписываемся на изменение состояния:
input.ngControl.control?.events .pipe( filter((event) => event instanceof TouchedChangeEvent), tap(() => this.check()), takeUntilDestroyed(this.destroyRef), ) .subscribe(); input.ngControl.valueChanges ?.pipe( tap(() => { if (!input.ngControl.value && this.elementRef.nativeElement.classList.contains('is-value')) { this.renderer.removeClass(this.elementRef.nativeElement, 'is-value'); } this.onInput({ target: input.elementRef.nativeElement }); }), takeUntilDestroyed(this.destroyRef), ) .subscribe(); input.ngControl.statusChanges ?.pipe( startWith(input.ngControl.status), tap((status: FormControlStatus) => { this.isDisabled = status === 'DISABLED'; this.disable(); }), takeUntilDestroyed(this.destroyRef), ) .subscribe();
Autocomplete
Реализуем autocomplete:
mkdir src/app/ui/autocomplete mkdir src/app/ui/autocomplete/lib echo >src/app/ui/autocomplete/index.ts
Выполним команду:
yarn ng g c autocomplete
Разметка и стили:
<baf-input-control cdkoverlayorigin> <label baf-label>{{ options().label }}</label> <input baf-input type="text"> </baf-input-control> <ng-template cdkconnectedoverlay> <div class="autocomplete-overlay"> @for (option of data() | async; track option.id; let index = $index) { <a class="autocomplete-option" index></a> } </div> </ng-template>
.autocomplete-overlay { background-color: var(--md-sys-color-surface-variant); color: var(--md-sys-color-on-surface-variant); width: 100%; padding: 1rem; border-radius: 0.25rem; box-shadow: 0 2px 5px 0 rgba(0, 0, 0, 0.15); display: flex; flex-direction: column; gap: 0.25rem; } .autocomplete-option { text-decoration: none; padding: 0.5rem; color: var(--md-sys-color-on-surface-variant); cursor: pointer; &:not(:last-child) { border-bottom: 1px solid var(--md-sys-color-surface); } &:hover { color: var(--md-sys-color-primary-container); } }
Логика компонента:
import { CdkConnectedOverlay, CdkOverlayOrigin } from '@angular/cdk/overlay'; import { AsyncPipe, NgForOf } from '@angular/common'; import type { Signal } from '@angular/core'; import { ChangeDetectionStrategy, Component, ElementRef, input, output, signal, viewChild } from '@angular/core'; import type { FormControl } from '@angular/forms'; import { ReactiveFormsModule } from '@angular/forms'; import type { Observable } from 'rxjs'; import { take, tap } from 'rxjs'; import type { DisplayFn } from '@baf/core'; import { InputComponent, InputControlComponent, InputDisplayDirective } from '@baf/ui/input'; import { LabelComponent } from '@baf/ui/label'; // eslint-disable-next-line @typescript-eslint/no-explicit-any export type AutocompleteVariant = Record<string any> & { readonly id: number | string }; export interface AutocompleteOptions { readonly label: string; readonly placeholder?: string; readonly id: string; readonly key: string; readonly displayFn: DisplayFn; readonly inputDisplayFn: DisplayFn; } @Component({ selector: 'baf-autocomplete', standalone: true, imports: [ ReactiveFormsModule, CdkConnectedOverlay, CdkOverlayOrigin, InputComponent, NgForOf, AsyncPipe, InputControlComponent, InputDisplayDirective, LabelComponent, ], templateUrl: './autocomplete.component.html', styleUrl: './autocomplete.component.scss', changeDetection: ChangeDetectionStrategy.OnPush, host: { class: 'baf-input-control', }, }) export class AutocompleteComponent { readonly control = input.required<formcontrol autocompletevariant>>(); readonly options = input.required<autocompleteoptions>(); readonly data = input.required<observable>>(); readonly changed = output<string>(); readonly opened = output(); readonly closed = output(); readonly input: Signal<elementref>> = viewChild.required('input', { read: ElementRef<htmlinputelement> }); readonly open = signal<boolean>(false); get width(): string { return this.input().nativeElement.clientWidth > 200 ? `${this.input().nativeElement.clientWidth}px` : '200px'; } onOpen(): void { if (!this.open()) { this.open.set(true); this.opened.emit(); } } onClose(): void { this.closed.emit(); this.open.set(false); this.data() .pipe( take(1), tap((options) => { if ( options.length && this.control().value && (typeof this.control().value === 'string' || JSON.stringify(this.control().value) !== JSON.stringify(options[0])) ) { this.control().patchValue(options[0], { emitEvent: false }); } }), ) .subscribe(); } onInput(event: Event): void { this.changed.emit((event.target as HTMLInputElement).value); } onSelect(option: AutocompleteVariant): void { this.control().patchValue(option, { emitEvent: false }); this.closed.emit(); this.open.set(false); } } </boolean></htmlinputelement></elementref></string></observable></autocompleteoptions></formcontrol></string>
Суть работы следующая:
- при клике на поле показать выпадающее окно;
- при вводе значений, вывести подсказки.
Методы:
- onOpen - показать окно;
- onInput - ввод значения;
- onSelect - выбор подсказки;
- onClose - событие закрытия.
Показанных компонентов достаточно, чтобы перейти к разработке страниц.
Ссылки
Все исходники находятся на github, в репозитории - github.com/Fafnur/buy-and-fly
Демо можно посмотреть здесь - buy-and-fly.fafn.ru/
Мои группы: telegram, medium, vk, x.com, linkedin, site
以上是Angular 18 中的 UI KIT 開發的詳細內容。更多資訊請關注PHP中文網其他相關文章!
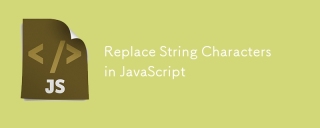
JavaScript字符串替換方法詳解及常見問題解答 本文將探討兩種在JavaScript中替換字符串字符的方法:在JavaScript代碼內部替換和在網頁HTML內部替換。 在JavaScript代碼內部替換字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 該方法僅替換第一個匹配項。要替換所有匹配項,需使用正則表達式並添加全局標誌g: str = str.replace(/fi
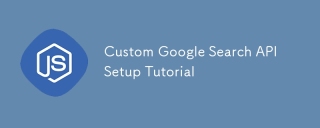
本教程向您展示瞭如何將自定義的Google搜索API集成到您的博客或網站中,提供了比標準WordPress主題搜索功能更精緻的搜索體驗。 令人驚訝的是簡單!您將能夠將搜索限制為Y
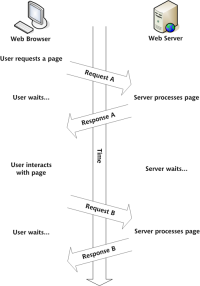
因此,在這裡,您準備好了解所有稱為Ajax的東西。但是,到底是什麼? AJAX一詞是指用於創建動態,交互式Web內容的一系列寬鬆的技術。 Ajax一詞,最初由Jesse J創造
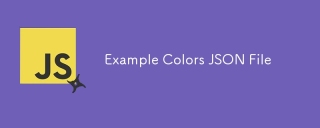
本文系列在2017年中期進行了最新信息和新示例。 在此JSON示例中,我們將研究如何使用JSON格式將簡單值存儲在文件中。 使用鍵值對符號,我們可以存儲任何類型的
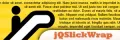
利用輕鬆的網頁佈局:8 ESTISSEL插件jQuery大大簡化了網頁佈局。 本文重點介紹了簡化該過程的八個功能強大的JQuery插件,對於手動網站創建特別有用
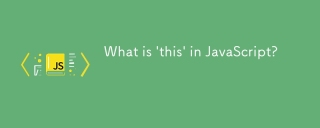
核心要點 JavaScript 中的 this 通常指代“擁有”該方法的對象,但具體取決於函數的調用方式。 沒有當前對象時,this 指代全局對象。在 Web 瀏覽器中,它由 window 表示。 調用函數時,this 保持全局對象;但調用對象構造函數或其任何方法時,this 指代對象的實例。 可以使用 call()、apply() 和 bind() 等方法更改 this 的上下文。這些方法使用給定的 this 值和參數調用函數。 JavaScript 是一門優秀的編程語言。幾年前,這句話可

jQuery是一個很棒的JavaScript框架。但是,與任何圖書館一樣,有時有必要在引擎蓋下發現發生了什麼。也許是因為您正在追踪一個錯誤,或者只是對jQuery如何實現特定UI感到好奇
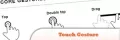
該帖子編寫了有用的作弊表,參考指南,快速食譜以及用於Android,BlackBerry和iPhone應用程序開發的代碼片段。 沒有開發人員應該沒有他們! 觸摸手勢參考指南(PDF)是Desig的寶貴資源


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

SublimeText3 Linux新版
SublimeText3 Linux最新版

SublimeText3漢化版
中文版,非常好用

記事本++7.3.1
好用且免費的程式碼編輯器
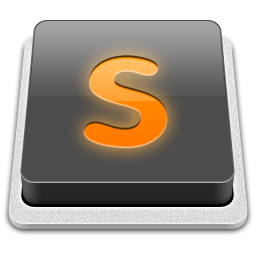
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)