介紹
很多時候,我們的應用程式需要提供一種簡單的方式來支付購買產品或服務的費用。 Stripe 是接收付款的好選擇。在這篇文章中,我們將學習如何建立條紋付款鏈接,以便您可以將用戶重新導向到這些連結以發送付款。
什麼是條紋?
Stripe 是一家提供線上支付處理服務的科技公司,允許企業透過網路接受付款。它提供了一套工具和 API,使公司能夠管理線上交易、訂閱和其他與付款相關的任務。
開始寫程式碼之前,我們必須先了解以下Stripe元件:
- 產品:產品代表您想要銷售的商品或服務。其中包括名稱、描述和定價詳細資訊等。
-
價格:價格是產品的特定定價配置。它定義了客戶為產品支付的金額,以及任何其他定價詳細信息,例如貨幣、計費週期和定價等級。例如,對於名為「社區會員」的服務,您可以有兩個價格:
- 按年付費,用戶每年支付 50 美元。
- 按月付費,用戶每月支付 3.5 美元。
付款連結:付款連結是允許客戶以特定價格付款的 URL。當客戶點擊付款連結時,他們會被重定向到 Stripe 託管的付款頁面,可以在其中輸入付款資訊並完成交易。付款連結可以透過電子郵件、訊息應用程式分享,或嵌入您的網站。
價格也允許我們定義付款類型,可以是「定期」或「一次性」。對於“社區會員資格範例”,年度付款可以是 one_type,每月付款可以是經常性的。每年,用戶都會續訂(或不續訂)會員資格,並重新選擇付款方式。
安裝 Stripe PHP 元件
stripe php 庫可以使用 Composer 安裝,因此,要安裝它,您只需在專案根資料夾中執行以下命令:
composer require stripe/stripe-php
檢索開發者 API 金鑰
在檢索 API 金鑰之前,您必須在 Stripe 上註冊。註冊後,您可以按照以下步驟操作:
- 登入您的 Stripe 帳號。
- 點擊螢幕右上角的齒輪圖標,然後選擇「開發者」。
- 點擊“API 金鑰”,您就可以建立它們。
建立條紋價格
我們可以先建立產品,然後建立價格,或將產品名稱嵌入價格選項中來建立 Stripe Price。讓我們使用第一種方式進行編碼,這樣我們就可以看到所有的過程。
$stripe = new \Stripe\StripeClient(<your_stripe_api_key>); $product = $stripe->products->create([ 'name' => 'Community Subscription', 'description' => 'A Subscription to our community', ]); $price = $stripe->prices->create([ 'currency' => 'usd', 'unit_amount' => 5025, 'product': $product->id, 'type' => 'one_time' ]); </your_stripe_api_key>
我們一步一步解釋一下上面的程式碼:
- 我們建立一個新的 StripeClient 並傳遞我們的 stripe api 金鑰。
- 然後,我們建立一個名為「社群訂閱」的新產品。
- 最後,我們為最近創建的產品創建一個價格,具有以下功能:
- 它使用美元貨幣。
- 它透過 ID 引用所建立的產品。
- 付款不會重複進行。
unit_amount 參數值得特別注意。 Stripe 文件對unit_amount 進行瞭如下描述:「以美分為單位的正整數(或免費價格為0),表示收費金額。」這意味著我們必須將價格乘以100 以將其轉換為美分,然後再傳遞給unit_amount 參數。例如,如果價格為 10.99 美元,我們會將 unit_amount 設定為 1099。這是一個常見問題,因此請務必仔細檢查您的程式碼,以避免任何意外的定價問題。
例如,如果您有一個「$amount」變量,它會保存一個浮點值作為金額,您可以編寫以下程式碼:
$formattedAmount = (int)($amount * 100);
建立付款連結
到目前為止,我們已經創建了一個格式正確的金額的價格。現在是時候建立付款連結了。
$stripe = new \Stripe\StripeClient(<your_stripe_api_key>); $paymentLink = $stripe->paymentLinks->create([ 'line_items' => [ [ 'price' => $price->id, 'quantity' => 1, ] ], 'after_completion' => [ 'type' => 'redirect', 'redirect' => [ 'url' => <your redirect link> ] ] ]); </your></your_stripe_api_key>
讓我們一步一步解釋一下:
- We create the StripeClient object as before.
- Then, we create the payment link by using the following options:
- line_items: Here you can include all the items we want to add for selling. In this case we only add the created price and we only sell one unit.
- after_completion: We instruct Stripe to redirect to an url after the payment is completed.
We could redirect to an intermediate url which could perform some stuff such as updating our database register payment. The following code shows a simple Symfony controller which would perform the required tasks and then would redirect to the final url where the user will see that the payment has been completed.
class StripeController extends AbstractController { #[Route('/confirm-payment', name: 'confirm-payment', methods: ['GET'])] public function confirmPayment(Request $request): Response { // Here you perform the necessary stuff $succeedUrl = '...'; return new RedirectResponse($succeedUrl); } }
After we have created the PaymentLink object, we can access the string payment url by the url property:
$paymentUrl = $paymentLink->url;
Conclusion
In this post we have learned how to configure our php backend to easily accept payments with stripe using the stripe-php component.
Processing the payments in your php backend offers some advantages such as:
- Keep sensitive payment information, such as the API keys, secure.
- Gives more control over the payment flow and allows for greater flexibility in handling different payment scenarios.
If you like my content and enjoy reading it and you are interested in learning more about PHP, you can read my ebook about how to create an operation-oriented API using PHP and the Symfony Framework. You can find it here: Building an Operation-Oriented Api using PHP and the Symfony Framework: A step-by-step guide
以上是使用 Stripe 和 PHP 輕鬆接收付款的詳細內容。更多資訊請關注PHP中文網其他相關文章!

TheSecretTokeEpingAphp-PowerEdwebSiterUnningSmoothlyShyunderHeavyLoadInVolvOLVOLVOLDEVERSALKEYSTRATICES:1)emplactopCodeCachingWithOpcachingWithOpCacheToreCescriptexecution Time,2)使用atabasequercachingCachingCachingWithRedataBasEndataBaseLeSendataBaseLoad,3)
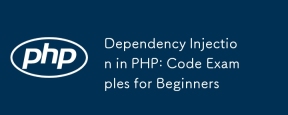
你應該關心DependencyInjection(DI),因為它能讓你的代碼更清晰、更易維護。 1)DI通過解耦類,使其更模塊化,2)提高了測試的便捷性和代碼的靈活性,3)使用DI容器可以管理複雜的依賴關係,但要注意性能影響和循環依賴問題,4)最佳實踐是依賴於抽象接口,實現鬆散耦合。
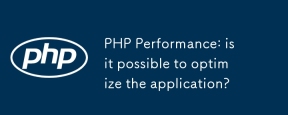
是的,優化papplicationispossibleandessential.1)empartcachingingcachingusedapcutorediucedsatabaseload.2)優化的atabaseswithexing,高效Quereteries,and ConconnectionPooling.3)EnhanceCodeWithBuilt-unctions,避免使用,避免使用ingglobalalairaiables,並避免使用
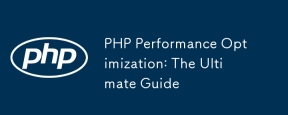
theKeyStrategiestosigantificallyBoostPhpaPplicationPerformenCeare:1)UseOpCodeCachingLikeLikeLikeLikeLikeCacheToreDuceExecutiontime,2)優化AtabaseInteractionswithPreparedStateTementStatementStatementAndProperIndexing,3)配置
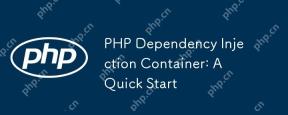
aphpdepentioncontiveContainerIsatoolThatManagesClassDeptions,增強codemodocultion,可驗證性和Maintainability.itactsasaceCentralHubForeatingingIndections,因此reducingTightCightTightCoupOulplingIndeSingantInting。
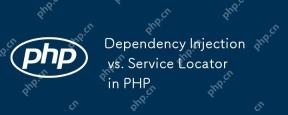
選擇DependencyInjection(DI)用於大型應用,ServiceLocator適合小型項目或原型。 1)DI通過構造函數注入依賴,提高代碼的測試性和模塊化。 2)ServiceLocator通過中心註冊獲取服務,方便但可能導致代碼耦合度增加。
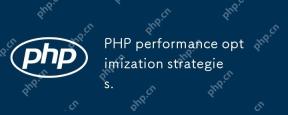
phpapplicationscanbeoptimizedForsPeedAndeffificeby:1)啟用cacheInphp.ini,2)使用preparedStatatementSwithPdoforDatabasequesies,3)3)替換loopswitharray_filtaray_filteraray_maparray_mapfordataprocrocessing,4)conformentnginxasaseproxy,5)
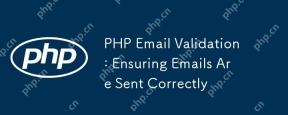
phpemailvalidation invoLvesthreesteps:1)格式化進行regulareXpressecthemailFormat; 2)dnsvalidationtoshethedomainhasavalidmxrecord; 3)


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
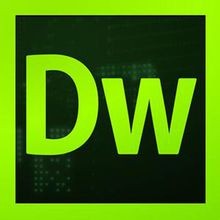
Dreamweaver CS6
視覺化網頁開發工具
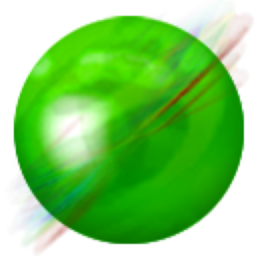
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境
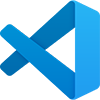
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
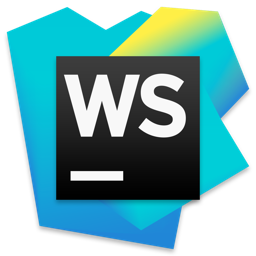
WebStorm Mac版
好用的JavaScript開發工具