有效地處理異常對於編寫健全的 Java 應用程式至關重要,尤其是在處理檔案操作時。僅僅列印堆疊追蹤(e.printStackTrace())是不夠的;這是一個常見的錯誤,可能會使您的應用程式容易受到攻擊,並且您的日誌中會充斥著無用的信息。這篇文章將探討如何撰寫適合不同文件類型和場景的有意義且資訊豐富的 catch 區塊。我們還將討論可能需要特別注意的邊緣情況。
1.有效異常處理的一般原則
在深入研究特定文件類型之前,讓我們先建立一些處理異常的指導原則:
- 提供清晰、可操作的訊息:您的錯誤訊息應該告訴您出了什麼問題,如果可能的話,也應該告訴您如何修復它。
- 明智地使用日誌記錄:不要列印堆疊跟踪,而是使用適當的嚴重程度(資訊、警告、錯誤)來記錄錯誤。
- 優雅地失敗:如果發生錯誤,請確保您的應用程式可以正常恢復或關閉,而不是意外崩潰。
- 避免捕獲通用異常: 捕獲特定異常(例如 FileNotFoundException、IOException)而不是 Exception,以避免掩蓋潛在問題。
2.處理文字檔異常
場景:讀取遺失或損壞的文字檔案
範例:
import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.nio.charset.MalformedInputException; public class TextFileHandler { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("example.txt"))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (FileNotFoundException e) { logError("Text file not found: 'example.txt'. Please ensure the file path is correct.", e); } catch (MalformedInputException e) { logError("The file 'example.txt' appears to be corrupted or contains invalid characters.", e); } catch (IOException e) { logError("An I/O error occurred while reading 'example.txt'. Please check if the file is accessible.", e); } } private static void logError(String message, Exception e) { // Use a logging framework like Log4j or SLF4J System.err.println(message); e.printStackTrace(); // Consider logging this instead of printing } }
重點:
- FileNotFoundException: 明確指示檔案遺失並提供潛在的修復方法。
- MalformedInputException: 當檔案編碼不正確或檔案損壞時發生的不太常見的異常。
- IOException: 使用它來處理一般 I/O 錯誤,但仍提供上下文(例如權限問題、檔案鎖定)。
3.處理二進位檔案異常
場景:寫入唯讀二進位檔案
範例:
import java.io.FileOutputStream; import java.io.IOException; import java.nio.file.AccessDeniedException; public class BinaryFileHandler { public static void main(String[] args) { try (FileOutputStream outputStream = new FileOutputStream("readonly.dat")) { outputStream.write(65); } catch (AccessDeniedException e) { logError("Failed to write to 'readonly.dat'. The file is read-only or you don't have the necessary permissions.", e); } catch (IOException e) { logError("An unexpected error occurred while writing to 'readonly.dat'.", e); } } private static void logError(String message, Exception e) { System.err.println(message); e.printStackTrace(); } }
重點:
- AccessDeniedException: 此特定異常通知使用者該檔案可能是唯讀的或缺乏足夠的權限。
- 使用描述性訊息:建議使用者檢查檔案權限。
4.處理 ZIP 檔案異常
場景:從損壞的 ZIP 檔案中提取檔案
範例:
import java.io.FileInputStream; import java.io.IOException; import java.util.zip.ZipException; import java.util.zip.ZipInputStream; public class ZipFileHandler { public static void main(String[] args) { try (ZipInputStream zipStream = new ZipInputStream(new FileInputStream("archive.zip"))) { // Process ZIP entries } catch (ZipException e) { logError("Failed to open 'archive.zip'. The ZIP file may be corrupted or incompatible.", e); } catch (IOException e) { logError("An I/O error occurred while accessing 'archive.zip'.", e); } } private static void logError(String message, Exception e) { System.err.println(message); e.printStackTrace(); } }
重點:
- ZipException: 表示 ZIP 格式或檔案損壞的問題。
- 提供復原建議:建議使用者嘗試使用不同的工具來開啟檔案或重新下載它。
5.處理 Office 檔案異常
場景:讀取無法辨識的 Excel 檔案格式
範例:
import org.apache.poi.openxml4j.exceptions.InvalidFormatException; import org.apache.poi.ss.usermodel.WorkbookFactory; import java.io.FileInputStream; import java.io.IOException; public class ExcelFileHandler { public static void main(String[] args) { try (FileInputStream fis = new FileInputStream("spreadsheet.xlsx")) { WorkbookFactory.create(fis); } catch (InvalidFormatException e) { logError("The file 'spreadsheet.xlsx' is not a valid Excel file or is in an unsupported format.", e); } catch (IOException e) { logError("An error occurred while reading 'spreadsheet.xlsx'. Please check the file's integrity.", e); } } private static void logError(String message, Exception e) { System.err.println(message); e.printStackTrace(); } }
重點:
- InvalidFormatException: 特定於檔案格式問題。透過建議正確的格式或工具來幫助使用者。
- 清楚地解釋問題:使用者可能不明白為什麼他們的文件無法開啟;引導他們找到解決方案。
6.處理 XML 檔案異常
場景:解析無效的 XML 檔案
範例:
import org.xml.sax.SAXException; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.ParserConfigurationException; import java.io.File; import java.io.IOException; public class XMLFileHandler { public static void main(String[] args) { try { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); factory.newDocumentBuilder().parse(new File("config.xml")); } catch (SAXException e) { logError("Failed to parse 'config.xml'. The XML file may be malformed.", e); } catch (IOException e) { logError("An error occurred while reading 'config.xml'. Please ensure the file exists and is accessible.", e); } catch (ParserConfigurationException e) { logError("An internal error occurred while configuring the XML parser.", e); } } private static void logError(String message, Exception e) { System.err.println(message); e.printStackTrace(); } }
重點:
- SAXException: 特定於解析錯誤。告知使用者 XML 結構中可能存在的問題。
- ParserConfigurationException: 表示解析器設定有問題,這種情況很少見,但需要捕捉。
7.邊緣案例與創意捕獲塊
場景:處理中斷的 I/O 操作
如果您的應用程式處理大檔案或正在執行長時間運行的 I/O 操作,則執行緒可能會中斷。處理 InterruptedException 以及 I/O 異常可以提供更強大的解決方案。
範例:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class InterruptedFileReader { public static void main(String[] args) { Thread fileReaderThread = new Thread(() -> { try (BufferedReader reader = new BufferedReader(new FileReader("largefile.txt"))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); // Simulate processing time Thread.sleep(100); } } catch (IOException e) { logError("I/O error while reading 'largefile.txt'.", e); } catch (InterruptedException e) { logError("File reading operation was interrupted. Rolling back changes or cleaning up.", e); Thread.currentThread().interrupt(); // Restore the interrupt status } }); fileReaderThread.start(); // Assume some condition requires interruption fileReaderThread.interrupt(); } private static void logError(String message, Exception e) { System.err.println(message); e.printStackTrace(); } }
重點:
- InterruptedException: Important for operations that might need to be aborted or resumed.
- Clean Up and Restore State: Always clean up or roll back if an operation is interrupted.
Conclusion
Creating meaningful catch blocks is an art that goes beyond simply printing stack traces. By writing specific, informative, and actionable error messages, your Java applications become more robust and easier to maintain. These examples and edge cases should serve as a template for handling exceptions effectively in different file-handling scenarios.
Tips & Tricks:
- Customize Your Logging Framework: Integrate with logging frameworks like Log4j, SLF4J, or java.util.logging to manage different log levels and outputs.
- Leverage Custom Exceptions: Create your own exception classes for specific cases, providing even more context and control over the handling process.
- Don't Over-Catch: Avoid catching exceptions that you can’t handle meaningfully. It’s better to let those bubble up to higher levels where they can be managed more effectively.
- Regularly Review Catch Blocks: As your application evolves, ensure your catch blocks remain relevant and informative.
This guide should help you create more reliable and maintainable Java applications by improving how you handle file-related exceptions. Save this for later and refer back when crafting your own meaningful catch blocks!
以上是在 Java 中製作有意義的「catch」區塊以進行檔案處理的詳細內容。更多資訊請關注PHP中文網其他相關文章!
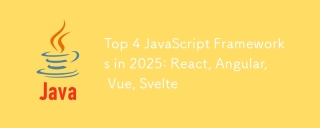
本文分析了2025年的前四個JavaScript框架(React,Angular,Vue,Susve),比較了它們的性能,可伸縮性和未來前景。 儘管由於強大的社區和生態系統,所有這些都保持占主導地位,但它們的相對人口
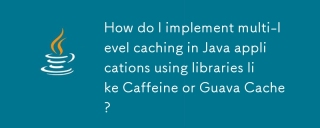
本文討論了使用咖啡因和Guava緩存在Java中實施多層緩存以提高應用程序性能。它涵蓋設置,集成和績效優勢,以及配置和驅逐政策管理最佳PRA
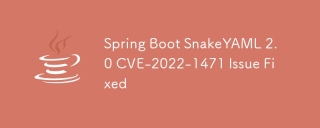
本文介紹了SnakeyAml中的CVE-2022-1471漏洞,這是一個允許遠程代碼執行的關鍵缺陷。 它詳細介紹瞭如何升級春季啟動應用程序到Snakeyaml 1.33或更高版本的降低風險,強調了依賴性更新
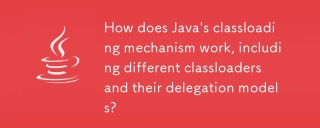
Java的類上載涉及使用帶有引導,擴展程序和應用程序類負載器的分層系統加載,鏈接和初始化類。父代授權模型確保首先加載核心類別,從而影響自定義類LOA
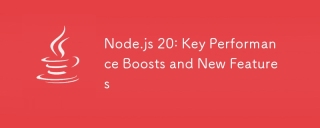
Node.js 20通過V8發動機改進可顯著提高性能,特別是更快的垃圾收集和I/O。 新功能包括更好的WebSembly支持和精製的調試工具,提高開發人員的生產率和應用速度。
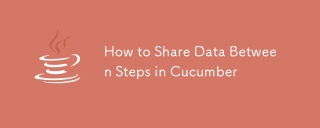
本文探討了在黃瓜步驟之間共享數據的方法,比較方案上下文,全局變量,參數傳遞和數據結構。 它強調可維護性的最佳實踐,包括簡潔的上下文使用,描述性
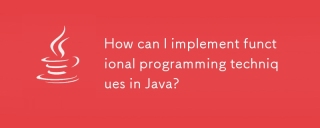
本文使用lambda表達式,流API,方法參考和可選探索將功能編程集成到Java中。 它突出顯示了通過簡潔性和不變性改善代碼可讀性和可維護性等好處


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

SublimeText3 Linux新版
SublimeText3 Linux最新版

SublimeText3漢化版
中文版,非常好用

記事本++7.3.1
好用且免費的程式碼編輯器
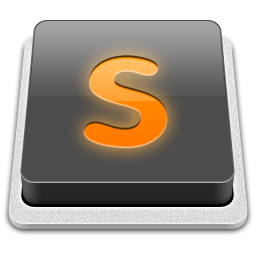
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)