為了簡化在各種事件(例如使用者建立、密碼重設等)後發送多封電子郵件通知,您可以採取一些步驟來集中通知和作業處理。這種方法將使您的工作更輕鬆且更具可擴展性,而無需為每個事件建立單獨的作業或通知。
簡化電子郵件通知處理的策略:
- 使用一般電子郵件通知作業。
- 利用事件監聽器架構。
- 將類似通知分組。
1. 建立通用電子郵件通知作業:
您可以建立一個將通知和使用者作為參數的單一可重複使用作業,而不是為每個通知建立單獨的作業。這樣,同一個作業可以用來處理不同的通知。
通用 SendEmailNotificationJob:
namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; use Illuminate\Notifications\Notification; use App\Models\User; class SendEmailNotificationJob implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public $user; public $notification; /** * Create a new job instance. * * @param User $user * @param Notification $notification * @return void */ public function __construct(User $user, Notification $notification) { $this->user = $user; $this->notification = $notification; } /** * Execute the job. * * @return void */ public function handle() { // Send the notification $this->user->notify($this->notification); } }
透過這個通用作業,您可以使用相同作業發送不同類型的電子郵件通知:
用法範例:
use App\Jobs\SendEmailNotificationJob; use App\Notifications\UserWelcomeNotification; use App\Models\User; $user = User::find(1); // Example user // Dispatch a welcome email notification SendEmailNotificationJob::dispatch($user, new UserWelcomeNotification()); // Dispatch a password reset notification SendEmailNotificationJob::dispatch($user, new PasswordResetNotification());
2. 利用事件監聽器架構:
Laravel 的 事件監聽器架構 無需在每個事件後手動調度作業,而是允許您根據特定事件(例如使用者建立)自動觸發通知和作業。
第 1 步:定義事件:
您可以定義一個事件,例如UserCreated:
php artisan make:event UserCreated
使用者建立事件範例:
namespace App\Events; use App\Models\User; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class UserCreated { use Dispatchable, SerializesModels; public $user; public function __construct(User $user) { $this->user = $user; } }
第2步:建立監聽器:
您可以建立一個監聽器,在事件觸發時發送通知:
php artisan make:listener SendUserWelcomeNotification --event=UserCreated
監聽器範例:
namespace App\Listeners; use App\Events\UserCreated; use App\Jobs\SendEmailNotificationJob; use App\Notifications\UserWelcomeNotification; class SendUserWelcomeNotification { public function handle(UserCreated $event) { // Dispatch the email notification job SendEmailNotificationJob::dispatch($event->user, new UserWelcomeNotification()); } }
第 3 步:建立使用者時觸發事件:
每當建立使用者時,您都可以觸發該事件,Laravel 將自動處理其餘的事情:
use App\Events\UserCreated; $user = User::create($data); event(new UserCreated($user));
這種方法可讓您將處理通知的邏輯與業務邏輯解耦,從而使系統更具可擴展性。
3. 將類似通知分組:
如果您有許多類似的通知(例如,歡迎電子郵件、密碼重設等與使用者相關的通知),您可以建立一個通知服務來集中處理所有使用者通知。
通知服務範例:
namespace App\Services; use App\Models\User; use App\Jobs\SendEmailNotificationJob; use App\Notifications\UserWelcomeNotification; use App\Notifications\PasswordResetNotification; class NotificationService { public function sendUserWelcomeEmail(User $user) { SendEmailNotificationJob::dispatch($user, new UserWelcomeNotification()); } public function sendPasswordResetEmail(User $user) { SendEmailNotificationJob::dispatch($user, new PasswordResetNotification()); } // You can add more methods for different types of notifications }
用法範例:
在您的控制器或事件偵聽器中,您現在可以簡單地呼叫該服務:
$notificationService = new NotificationService(); $notificationService->sendUserWelcomeEmail($user);
結論:
- 單一作業:您可以使用通用作業(SendEmailNotificationJob)來處理不同類型的通知。
- 事件監聽器架構:利用Laravel的事件監聽器系統依照系統事件自動觸發通知。
- 集中通知服務:將類似的通知分組到一個服務中,以實現更好的管理和可重複使用性。
這種方法有助於保持程式碼乾燥(不要重複自己),並且當您有多個電子郵件通知要發送時,可以更輕鬆地維護。
以上是集中您的通知和作業處理的詳細內容。更多資訊請關注PHP中文網其他相關文章!
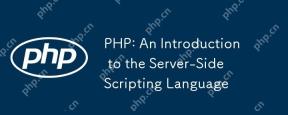
PHP是一種服務器端腳本語言,用於動態網頁開發和服務器端應用程序。 1.PHP是一種解釋型語言,無需編譯,適合快速開發。 2.PHP代碼嵌入HTML中,易於網頁開發。 3.PHP處理服務器端邏輯,生成HTML輸出,支持用戶交互和數據處理。 4.PHP可與數據庫交互,處理表單提交,執行服務器端任務。
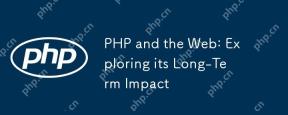
PHP在過去幾十年中塑造了網絡,並將繼續在Web開發中扮演重要角色。 1)PHP起源於1994年,因其易用性和與MySQL的無縫集成成為開發者首選。 2)其核心功能包括生成動態內容和與數據庫的集成,使得網站能夠實時更新和個性化展示。 3)PHP的廣泛應用和生態系統推動了其長期影響,但也面臨版本更新和安全性挑戰。 4)近年來的性能改進,如PHP7的發布,使其能與現代語言競爭。 5)未來,PHP需應對容器化、微服務等新挑戰,但其靈活性和活躍社區使其具備適應能力。
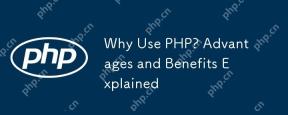
PHP的核心優勢包括易於學習、強大的web開發支持、豐富的庫和框架、高性能和可擴展性、跨平台兼容性以及成本效益高。 1)易於學習和使用,適合初學者;2)與web服務器集成好,支持多種數據庫;3)擁有如Laravel等強大框架;4)通過優化可實現高性能;5)支持多種操作系統;6)開源,降低開發成本。
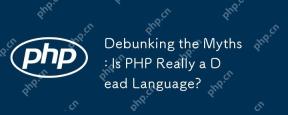
PHP沒有死。 1)PHP社區積極解決性能和安全問題,PHP7.x提升了性能。 2)PHP適合現代Web開發,廣泛用於大型網站。 3)PHP易學且服務器表現出色,但類型系統不如靜態語言嚴格。 4)PHP在內容管理和電商領域仍重要,生態系統不斷進化。 5)通過OPcache和APC等優化性能,使用OOP和設計模式提升代碼質量。
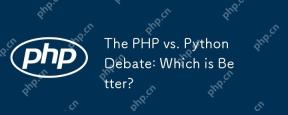
PHP和Python各有優劣,選擇取決於項目需求。 1)PHP適合Web開發,易學,社區資源豐富,但語法不夠現代,性能和安全性需注意。 2)Python適用於數據科學和機器學習,語法簡潔,易學,但執行速度和內存管理有瓶頸。
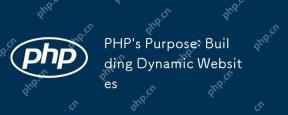
PHP用於構建動態網站,其核心功能包括:1.生成動態內容,通過與數據庫對接實時生成網頁;2.處理用戶交互和表單提交,驗證輸入並響應操作;3.管理會話和用戶認證,提供個性化體驗;4.優化性能和遵循最佳實踐,提升網站效率和安全性。

PHP在數據庫操作和服務器端邏輯處理中使用MySQLi和PDO擴展進行數據庫交互,並通過會話管理等功能處理服務器端邏輯。 1)使用MySQLi或PDO連接數據庫,執行SQL查詢。 2)通過會話管理等功能處理HTTP請求和用戶狀態。 3)使用事務確保數據庫操作的原子性。 4)防止SQL注入,使用異常處理和關閉連接來調試。 5)通過索引和緩存優化性能,編寫可讀性高的代碼並進行錯誤處理。
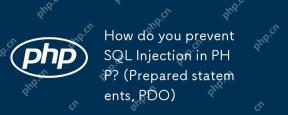
在PHP中使用預處理語句和PDO可以有效防範SQL注入攻擊。 1)使用PDO連接數據庫並設置錯誤模式。 2)通過prepare方法創建預處理語句,使用佔位符和execute方法傳遞數據。 3)處理查詢結果並確保代碼的安全性和性能。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
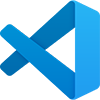
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!
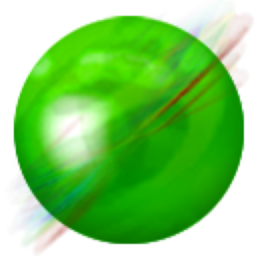
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境