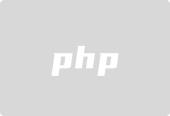
在機器學習領域,評估模型的表現至關重要。此評估有助於我們了解模型預測或分類資料的效果。在眾多可用指標中,平均絕對誤差 (MAE)、均方誤差 (MSE) 和均方根誤差 (RMSE) 是最常用的三個指標。但我們為什麼要使用它們呢?是什麼讓它們如此重要?
1. 平均絕對誤差(MAE)
MAE是什麼?
平均絕對誤差測量一組預測中誤差的平均大小,而不考慮其方向。它是預測值和實際值之間的絕對差的平均值。
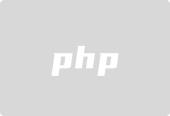
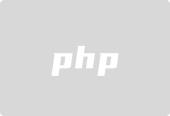
為什麼要使用 MAE?
-
可解釋性: MAE 提供了對平均誤差的清晰、直接的解釋。如果 MAE 為 5,則模型的預測平均與實際值相差 5 個單位。
- **穩健性:**MAE 與 MSE 和 RMSE 相比對異常值較不敏感,因為它不平方誤差項。
何時使用 MAE?
當您想要直接了解平均誤差而不誇大大誤差的影響時,MAE 是首選。當資料集存在異常值或錯誤成本呈線性時,它特別有用。
2. 均方誤差(MSE)
什麼是 MSE?
均方誤差是預測值與實際值之間的平方差的平均值。
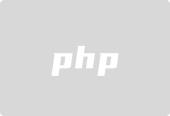
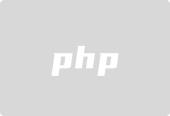
為什麼要用 MSE?
-
誤差放大:透過對誤差進行平方,MSE 為較大誤差賦予更多權重,當大誤差特別不受歡迎時,MSE 成為一個很好的指標。
-
數學屬性: MSE 是可微分的,並且經常在梯度下降等優化演算法中用作損失函數,因為它的導數很容易計算。
何時使用 MSE?
當大錯誤比小錯誤更成問題,以及當您希望指標對大偏差進行更嚴厲的懲罰時,通常會使用 MSE。它在模型訓練過程中也很常用,因為它計算方便。
3.均方根誤差(RMSE)
什麼是 RMSE?
均方根誤差是 MSE 的平方根。它將指標恢復到資料的原始規模,使其比 MSE 更容易解釋。
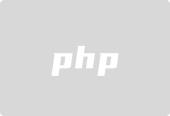
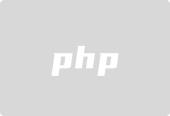
為什麼要使用 RMSE?
尺度上的可解釋性:RMSE 與 MSE 不同,它與原始資料處於同一尺度,使其更具可解釋性。
對大誤差敏感:與 MSE 一樣,RMSE 也會懲罰大誤差,但由於它是在原始尺度上,因此可以提供更直觀的誤差大小測量。
何時使用 RMSE?
當您想要一個懲罰大錯誤的指標但仍需要結果與原始資料採用相同單位時,首選 RMSE。它廣泛用於誤差幅度分佈很重要以及與數據處於相同規模至關重要的環境中。
選擇正確的指標
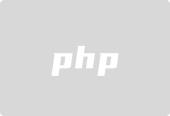
-
MAE 對異常值更加穩健,並給出與數據相同單位的平均誤差,使其易於解釋。
-
MSE 放大較大的誤差,當較大的誤差代價特別昂貴時,它非常有用,並且它經常在模型訓練中用作損失函數。
-
RMSE 結合了 MSE 和 MAE 的優點,提供了一種錯誤度量,可以懲罰大錯誤並保持可解釋性。
在實務中,MAE、MSE 和 RMSE 之間的選擇取決於當前問題的特定要求。如果您的應用程式需要簡單、可解釋的指標,MAE 可能是最佳選擇。如果您需要更嚴厲地懲罰較大的錯誤,MSE 或 RMSE 可能會更合適。
圖形表示
1. 建立和迴歸模型
以下是我們如何使用迴歸模型產生 MAE、MSE 和 RMSE 的圖形表示:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_absolute_error, mean_squared_error
# Generate some synthetic data for demonstration
np.random.seed(42)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
# Train a simple linear regression model
model = LinearRegression()
model.fit(X, y)
y_pred = model.predict(X)
# Calculate MAE, MSE, and RMSE
mae = mean_absolute_error(y, y_pred)
mse = mean_squared_error(y, y_pred)
rmse = np.sqrt(mse)
# Plotting the regression line with errors
plt.figure(figsize=(12, 8))
# Scatter plot of actual data points
plt.scatter(X, y, color='blue', label='Actual Data')
# Regression line
plt.plot(X, y_pred, color='red', label='Regression Line')
# Highlighting errors (residuals)
for i in range(len(X)):
plt.vlines(X[i], y[i], y_pred[i], color='gray', linestyle='dashed')
# Adding annotations for MAE, MSE, RMSE
plt.text(0.5, 8, f'MAE: {mae:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
plt.text(0.5, 7.5, f'MSE: {mse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
plt.text(0.5, 7, f'RMSE: {rmse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
# Titles and labels
plt.title('Linear Regression with MAE, MSE, and RMSE')
plt.xlabel('X')
plt.ylabel('y')
plt.legend()
plt.show()
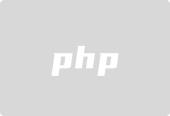
2. 劇情說明
- **藍點:**這些代表實際資料點。
-
紅線:這是代表模型預測值的迴歸線。
-
灰線:這些虛線代表每個資料點的殘差或誤差。這些線的長度對應於誤差大小。
-
MAE、MSE、RMSE: 在圖中進行註釋,顯示這些值可協助視覺化如何評估模型的效能。
3. 解釋
-
MAE:給出與資料相同單位的平均誤差,顯示資料點與迴歸線的平均距離。
-
MSE:將誤差平方,更強調較大的誤差,常在迴歸模型的訓練過程中使用。
-
RMSE:提供與原始資料相同規模的指標,使其比 MSE 更易於解釋,同時仍懲罰較大的錯誤。
訓練機器學習模型
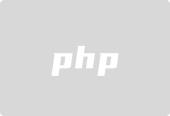
在訓練機器學習模型時,尤其是在迴歸任務中,選擇正確的誤差測量至關重要,因為它會影響模型的學習方式及其效能評估方式。讓我們來分解MAE、MSE、RMSE在模型訓練中的意義:
1.MAE(平均絕對誤差)
定義:MAE 是預測值與實際值之間絕對差的平均值。
在模型訓練中的意義:
-
對異常值的穩健性:與 MSE 和 RMSE 相比,MAE 對異常值不太敏感,因為它平等地對待所有錯誤,而不對它們進行平方。這意味著在訓練過程中,模型的目標是最小化平均誤差,而不會過度專注於較大的誤差。
-
線性懲罰:MAE 的線性性質意味著每個錯誤對模型學習過程的影響與該錯誤的大小成正比。
-
可解釋性:MAE 與原始資料的單位相同,較容易解釋。如果 MAE 為 5,則表示模型的預測平均偏差 5 個單位。
2.MSE(均方誤差)
定義:MSE 是預測值和實際值之間的平方差的平均值。
Significance in Model Training:
-
Sensitivity to Outliers: MSE is sensitive to outliers because it squares the error, making larger errors much more significant in the calculation. This causes the model to prioritize reducing large errors during training.
-
Punishing Large Errors: The squaring effect means that the model will penalize larger errors more severely, which can lead to a better fit for most data points but might overfit to outliers.
-
Smooth Gradient: MSE is widely used in optimization algorithms like gradient descent because it provides a smooth gradient, making it easier for the model to converge during training.
-
Model’s Focus on Large Errors: Since large errors have a bigger impact, the model might focus on reducing these at the cost of slightly increasing smaller errors, which can be beneficial if large errors are particularly undesirable in the application.
3. RMSE (Root Mean Squared Error)
Definition: RMSE is the square root of the average of the squared differences between the predicted and actual values.
Significance in Model Training:
-
Balance between MAE and MSE: RMSE retains the sensitivity to outliers like MSE but brings the error metric back to the original scale of the data, making it more interpretable than MSE.
-
Penalizes Large Errors: Similar to MSE, RMSE also penalizes larger errors more due to the squaring process, but because it takes the square root, it doesn’t exaggerate them as much as MSE does.
-
Interpretable Units: Since RMSE is on the same scale as the original data, it’s easier to understand in the context of the problem. For instance, an RMSE of 5 means that on average, the model’s prediction errors are about 5 units away from the actual values.
-
Optimization in Complex Models: RMSE is often used in models where the distribution of errors is important, such as in complex regression models or neural networks.
Visual Example to Show Significance in Model Training:
Let’s consider a graphical representation that shows how these metrics affect the model’s training process.
-
MAE Focuses on Reducing Average Error: Imagine the model adjusting the regression line to minimize the average height of the gray dashed lines (errors) equally for all points.
-
MSE Prioritizes Reducing Large Errors: The model might adjust the line more drastically to reduce the longer dashed lines (larger errors), even if it means increasing some smaller ones.
-
RMSE Balances Both: The model will make adjustments that reduce large errors but will not overemphasize them to the extent of distorting the overall fit.
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_absolute_error, mean_squared_error
# Generate synthetic data with an outlier
np.random.seed(42)
X = 2 * np.random.rand(100, 1)
y = 4 + 3 * X + np.random.randn(100, 1)
y[98] = 30 # Adding an outlier
# Train a simple linear regression model
model = LinearRegression()
model.fit(X, y)
y_pred = model.predict(X)
# Calculate MAE, MSE, and RMSE
mae = mean_absolute_error(y, y_pred)
mse = mean_squared_error(y, y_pred)
rmse = np.sqrt(mse)
# Plotting the regression line with errors
plt.figure(figsize=(12, 8))
# Scatter plot of actual data points
plt.scatter(X, y, color='blue', label='Actual Data')
# Regression line
plt.plot(X, y_pred, color='red', label='Regression Line')
# Highlighting errors (residuals)
for i in range(len(X)):
plt.vlines(X[i], y[i], y_pred[i], color='gray', linestyle='dashed')
# Annotating one of the residual lines
plt.text(X[0] + 0.1, (y[0] + y_pred[0]) / 2, 'Error (Residual)', color='gray')
# Adding annotations for MAE, MSE, RMSE
plt.text(0.5, 20, f'MAE: {mae:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
plt.text(0.5, 18, f'MSE: {mse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
plt.text(0.5, 16, f'RMSE: {rmse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5))
# Titles and labels
plt.title('Linear Regression with MAE, MSE, and RMSE - Impact on Model Training')
plt.xlabel('X')
plt.ylabel('y')
plt.legend()
plt.show()
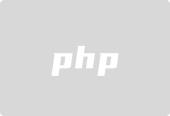
Explanation:
Outlier Impact: Notice how the model tries to adjust for the outlier in the upper region, which affects MSE and RMSE more significantly.
Model Training Implications:
-
With MAE: The model may place less emphasis on the outlier, leading to a fit that is more balanced but less sensitive to extreme deviations.
-
With MSE and RMSE: The model might adjust more aggressively to minimize the impact of the outlier, which can lead to a more distorted fit if outliers are rare.
Choosing the right approach for training a model depends on the specific problem you’re trying to solve, the nature of your data, and the goals of your model. Here’s a guide to help you decide which metric (MAE, MSE, RMSE) to focus on, along with considerations for training your model:
1. Nature of the Data
Presence of Outliers:
-
MAE: If your data contains outliers, and you don’t want these outliers to disproportionately affect your model, MAE is a good choice. It treats all errors equally, so a few large errors won’t dominate the metric.
-
MSE/RMSE: If outliers are expected and meaningful (e.g., extreme but valid cases), and you want your model to account for them strongly, MSE or RMSE might be more appropriate.
Homogeneous Data:
If your data is relatively homogeneous, without significant outliers, MSE or RMSE can help capture the overall performance, focusing more on the general fit of the model.
2. 模型的目標
可解釋性:
-
MAE:提供更容易的解釋,因為它與目標變數有相同的單位。如果原始單位的可解釋性很重要,並且您想用簡單的術語理解平均誤差,那麼 MAE 是更好的選擇。
-
RMSE:也可以用相同的單位解釋,但重點是更多地懲罰較大的錯誤。
注意較大的錯誤:
-
MSE/RMSE:如果您更關心較大的誤差,因為它們在您的應用中成本特別高或風險很大(例如,預測醫療劑量、財務預測),那麼MSE 或RMSE 應該是您的重點。這些指標對較大的錯誤懲罰更多,這可以指導模型優先考慮減少重大偏差。
-
MAE:如果您的應用程式平等對待所有錯誤並且您不希望模型過度關注大偏差,那麼 MAE 是更好的選擇。
3. 模型類型與複雜度
簡單線性模型:
-
MAE:適用於簡單的線性模型,其目標是最小化平均偏差,而不必過多擔心異常值。
-
MSE/RMSE:也可以使用,特別是如果模型需要考慮所有資料點,包括極端情況。
複雜模型(例如神經網路、整合方法):
-
MSE/RMSE:這些通常用於更複雜的模型,因為它們提供更平滑的梯度,這對於梯度下降等最佳化技術至關重要。對較大錯誤的懲罰也有助於微調複雜模型。
4. 最佳化與收斂
梯度下降和優化:
-
MSE/RMSE:在最佳化演算法中通常是首選,因為它們提供平滑且連續的誤差表面,這對於梯度下降等方法的有效收斂至關重要。
-
MAE:可能不太平滑,這可能會使最佳化稍微更具挑戰性,尤其是在大型模型中。然而,現代優化技術在許多情況下可以解決這個問題。
5. 背景考慮
應用特定要求:
-
MAE:非常適合需要避免異常值影響或錯誤成本線性時的應用,例如估計交付時間或預測分數。
-
MSE/RMSE:最適合特別不希望出現大錯誤以及應用程式要求對這些錯誤進行更高懲罰的情況,例如在高風險的財務預測、安全關鍵系統中或在優化模型時競爭環境。
結論:採取哪一種方法
-
如果異常值不是主要問題:使用 MSE 或 RMSE。它們幫助模型注意較大的錯誤,這在許多應用中至關重要。
-
如果您想要平衡的方法:RMSE 通常是一個很好的折衷方案,因為它以與目標變數相同的單位提供度量,同時仍然比較小的誤差對較大的誤差進行更多的懲罰。
-
如果您需要對異常值的穩健性:使用 MAE。它確保異常值不會對模型產生不成比例的影響,使其適合您想要更平衡模型的情況。
-
對於原始單位的可解釋性:MAE 或 RMSE 較容易解釋,因為它們與目標變數的單位相同。這在您需要向非技術利害關係人解釋結果的領域尤其重要。
在實踐中,您可以根據這些考慮因素從一個指標開始,然後進行實驗,看看您的模型在每個指標上的表現如何。在訓練期間監控多個指標以全面了解模型的表現也很常見。
Medium 文章 - 了解 MAE、MSE 和 RMSE:機器學習中的關鍵指標
@mondalsabbha
以上是了解 MAE、MSE 和 RMSE:機器學習中的關鍵指標的詳細內容。更多資訊請關注PHP中文網其他相關文章!