陣列搜尋演算法大全:線性搜尋:遍歷數組,時間複雜度 O(n)。二分搜尋(僅限有序數組):將數組二分,時間複雜度 O(log n)。哈希表:使用鍵值快速查找,時間複雜度 O(1)。
陣列搜尋演算法大全
在電腦科學中,陣列搜尋演算法用於在有序或無序陣列中找到特定元素。本文將探討各種陣列搜尋演算法,包括其時間複雜度和實戰案例。
線性搜尋
時間複雜度: O(n)
線性搜尋是最簡單、最直接的搜尋演算法。它從數組的開頭開始,並逐一比較元素,直到找到目標元素或到達數組的末尾。
def linear_search(arr, target): for i in range(len(arr)): if arr[i] == target: return i return -1
二分搜尋
時間複雜度: O(log n)
二分搜尋用於在有序數組中搜尋。它透過重複將數組分成兩半來縮小搜尋範圍。
def binary_search(arr, target): left, right = 0, len(arr) - 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: left = mid + 1 else: right = mid - 1 return -1
哈希表
時間複雜度: O(1)
哈希表是一種資料結構,它允許我們通過鍵值快速查找元素。數組可以用作哈希表的底層資料結構,其中索引用作鍵。
def hash_search(arr, target): hash_table = {} for i in range(len(arr)): hash_table[arr[i]] = i if target in hash_table: return hash_table[target] else: return -1
實戰案例
考慮以下查找學生分數的陣列搜尋案例:
students = [ {'name': 'John Doe', 'score': 85}, {'name': 'Jane Doe', 'score': 90}, {'name': 'Bill Smith', 'score': 75}, {'name': 'Alice Johnson', 'score': 95} ]
如果我們想找到"Alice Johnson" 的分數,我們可以使用線性搜尋:
for student in students: if student['name'] == 'Alice Johnson': print(student['score']) # 输出:95
或者,如果陣列按名稱排序,我們可以使用二分搜尋:
students.sort(key=lambda x: x['name']) left, right = 0, len(students) - 1 while left <= right: mid = (left + right) // 2 if students[mid]['name'] == 'Alice Johnson': print(students[mid]['score']) # 输出:95 break elif students[mid]['name'] < 'Alice Johnson': left = mid + 1 else: right = mid - 1
以上是數組的搜尋演算法有哪些?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
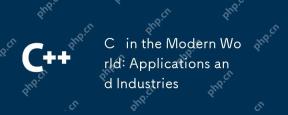
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
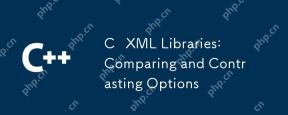
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
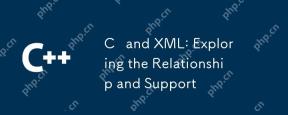
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
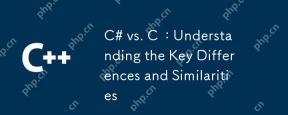
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
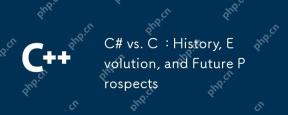
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
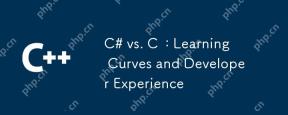
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
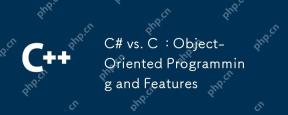
C#和C 在面向对象编程(OOP)中的实现方式和特性上有显著差异。1)C#的类定义和语法更为简洁,支持如LINQ等高级特性。2)C 提供更细粒度的控制,适用于系统编程和高性能需求。两者各有优势,选择应基于具体应用场景。
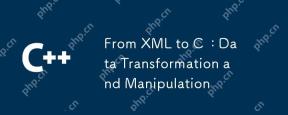
從XML轉換到C 並進行數據操作可以通過以下步驟實現:1)使用tinyxml2庫解析XML文件,2)將數據映射到C 的數據結構中,3)使用C 標準庫如std::vector進行數據操作。通過這些步驟,可以高效地處理和操作從XML轉換過來的數據。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

禪工作室 13.0.1
強大的PHP整合開發環境

SublimeText3漢化版
中文版,非常好用

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
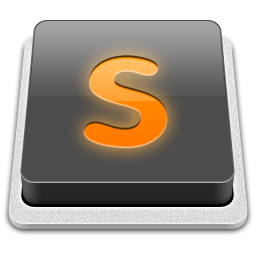
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)