在 Go 中,序列化自訂類型的方法有:使用 JSON 序列化時實作 json.Marshaler 接口,使用 Gob 序列化時實作 encoding/gob 套件中的 GobEncoder 和 GobDecoder 介面。
使用Golang 對自訂類型進行序列化
在Golang 中,序列化是指將物件的狀態轉換為可儲存或傳輸的格式。對於自訂類型,需要實作 encoding/json
或 encoding/gob
套件中的序列化介面。
使用 JSON 序列化
- 實作
json.Marshaler
接口,實作MarshalJSON
方法。 -
MarshalJSON
方法接收自訂類型的值並傳回其 JSON 表示。
實戰案例:序號員工結構體
package main import ( "encoding/json" "fmt" ) // Employee is a custom type representing an employee. type Employee struct { Name string Age int Skills []string } // MarshalJSON implements the json.Marshaler interface. func (e Employee) MarshalJSON() ([]byte, error) { type Alias Employee return json.Marshal(&struct{ Alias }{e}) } func main() { emp := Employee{Name: "John Doe", Age: 30, Skills: []string{"golang", "javascript"}} encoded, err := json.Marshal(emp) if err != nil { fmt.Println("Error:", err) return } fmt.Println("JSON:", string(encoded)) }
使用Gob 序列化
- ##實現
- encoding/gob
套件中的
GobEncoder和
GobDecoder介面。
- GobEncode
方法接收自訂類型的值並將其寫入緩衝區。
- GobDecode
方法從緩衝區讀取資料並恢復自訂類型的值。
實戰案例:序號一個複雜結構
package main import ( "encoding/gob" "fmt" "io/ioutil" "os" ) // ComplexStruct represents a complex data structure. type ComplexStruct struct { Map map[string]int Slice []int InnerStruct struct { Field1 string Field2 int } } func main() { // Register the ComplexStruct type for serialization. gob.Register(ComplexStruct{}) // Create a ComplexStruct instance. cs := ComplexStruct{ Map: map[string]int{"key1": 1, "key2": 2}, Slice: []int{3, 4, 5}, InnerStruct: struct { Field1 string Field2 int }{"value1", 6}, } // Encode the ComplexStruct to a file. f, err := os.Create("data.gob") if err != nil { fmt.Println("Error creating file:", err) return } defer f.Close() enc := gob.NewEncoder(f) if err := enc.Encode(cs); err != nil { fmt.Println("Error encoding:", err) return } // Decode the ComplexStruct from the file. data, err := ioutil.ReadFile("data.gob") if err != nil { fmt.Println("Error reading file:", err) return } dec := gob.NewDecoder(bytes.NewReader(data)) var decoded ComplexStruct if err := dec.Decode(&decoded); err != nil { fmt.Println("Error decoding:", err) return } // Print the decoded struct. fmt.Println("Decoded:", decoded) }
以上是使用 Golang 時如何對自訂類型進行序列化?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
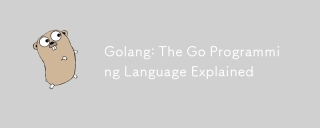
Go語言的核心特性包括垃圾回收、靜態鏈接和並發支持。 1.Go語言的並發模型通過goroutine和channel實現高效並發編程。 2.接口和多態性通過實現接口方法,使得不同類型可以統一處理。 3.基本用法展示了函數定義和調用的高效性。 4.高級用法中,切片提供了動態調整大小的強大功能。 5.常見錯誤如競態條件可以通過gotest-race檢測並解決。 6.性能優化通過sync.Pool重用對象,減少垃圾回收壓力。
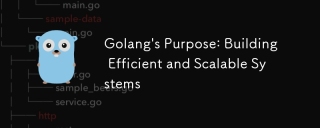
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
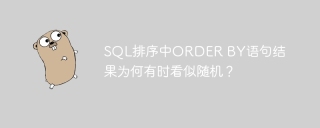
關於SQL查詢結果排序的疑惑學習SQL的過程中,常常會遇到一些令人困惑的問題。最近,筆者在閱讀《MICK-SQL基礎�...
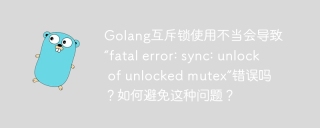
golang ...
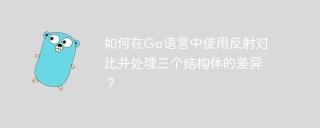
Go語言中如何對比並處理三個結構體在Go語言編程中,有時需要對比兩個結構體的差異,並將這些差異應用到第�...
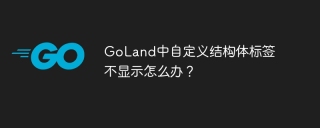
GoLand中自定義結構體標籤不顯示怎麼辦?在使用GoLand進行Go語言開發時,很多開發者會遇到自定義結構體標籤在�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
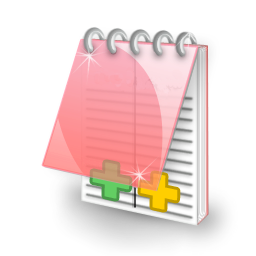
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

SublimeText3 Linux新版
SublimeText3 Linux最新版
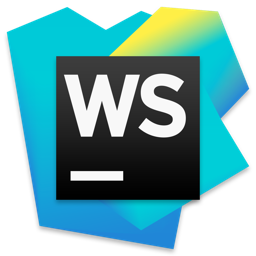
WebStorm Mac版
好用的JavaScript開發工具

禪工作室 13.0.1
強大的PHP整合開發環境

Atom編輯器mac版下載
最受歡迎的的開源編輯器