服務定位器模式
服務定位器模式(Service Locator Pattern)用在我們想要使用 JNDI 查詢定位各種服務的時候。考慮到為某項服務尋找 JNDI 的代價很高,服務定位器模式充分利用了快取技術。在首次要求某個服務時,服務定位器會在 JNDI 中尋找服務,並快取該服務物件。當再次請求相同的服務時,服務定位器會在它的快取中查找,這可以在很大程度上提高應用程式的效能。以下是這種設計模式的實體。
服務(Service) - 實際處理請求的服務。對這種服務的引用可以在 JNDI 伺服器中查找到。
Context / 初始的 Context - JNDI Context 帶有對要尋找的服務的參考。
服務定位器(Service Locator) - 服務定位器是透過 JNDI 尋找和快取服務來取得服務的單點接觸。
快取(Cache) - 快取儲存服務的引用,以便重複使用它們。
客戶端(Client) - Client 是透過 ServiceLocator 呼叫服務的物件。
實作
我們將建立ServiceLocator、InitialContext、Cache、Service 作為表示實體的各種物件。 Service1 和 Service2 表示實體服務。
ServiceLocatorPatternDemo,我們的示範類別在這裡是作為一個客戶端,將使用 ServiceLocator 來示範服務定位器設計模式。
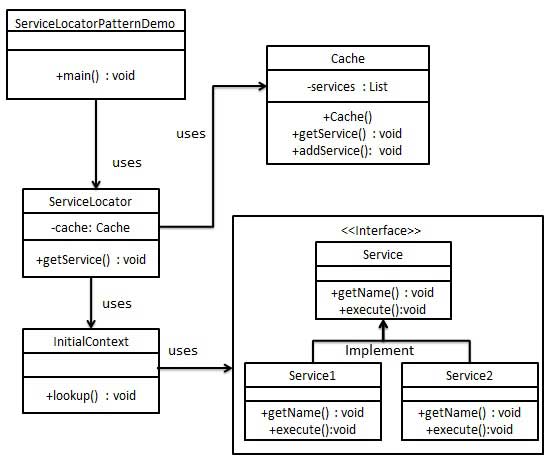
步驟 1
建立服務介面 Service。
Service.java
public interface Service { public String getName(); public void execute(); }
步驟 2
#建立實體服務。
Service1.java
public class Service1 implements Service { public void execute(){ System.out.println("Executing Service1"); } @Override public String getName() { return "Service1"; } }
Service2.java
public class Service2 implements Service { public void execute(){ System.out.println("Executing Service2"); } @Override public String getName() { return "Service2"; } }
步驟3
為JNDI 查詢建立InitialContext。
InitialContext.java
public class InitialContext { public Object lookup(String jndiName){ if(jndiName.equalsIgnoreCase("SERVICE1")){ System.out.println("Looking up and creating a new Service1 object"); return new Service1(); }else if (jndiName.equalsIgnoreCase("SERVICE2")){ System.out.println("Looking up and creating a new Service2 object"); return new Service2(); } return null; } }
步驟 4
建立快取 Cache。
Cache.java
import java.util.ArrayList; import java.util.List; public class Cache { private List<Service> services; public Cache(){ services = new ArrayList<Service>(); } public Service getService(String serviceName){ for (Service service : services) { if(service.getName().equalsIgnoreCase(serviceName)){ System.out.println("Returning cached "+serviceName+" object"); return service; } } return null; } public void addService(Service newService){ boolean exists = false; for (Service service : services) { if(service.getName().equalsIgnoreCase(newService.getName())){ exists = true; } } if(!exists){ services.add(newService); } } }
步驟 5
建立服務定位器。
ServiceLocator.java
public class ServiceLocator { private static Cache cache; static { cache = new Cache(); } public static Service getService(String jndiName){ Service service = cache.getService(jndiName); if(service != null){ return service; } InitialContext context = new InitialContext(); Service service1 = (Service)context.lookup(jndiName); cache.addService(service1); return service1; } }
步驟 6
#使用 ServiceLocator 來示範服務定位器設計模式。
ServiceLocatorPatternDemo.java
public class ServiceLocatorPatternDemo { public static void main(String[] args) { Service service = ServiceLocator.getService("Service1"); service.execute(); service = ServiceLocator.getService("Service2"); service.execute(); service = ServiceLocator.getService("Service1"); service.execute(); service = ServiceLocator.getService("Service2"); service.execute(); } }
步驟 7
驗證輸出。
Looking up and creating a new Service1 object Executing Service1 Looking up and creating a new Service2 object Executing Service2 Returning cached Service1 object Executing Service1 Returning cached Service2 object Executing Service2