A preliminary study on ContentProvider
Introduction to this section:
This section brings you the last of the four major components of Android-ContentProvider (content provider), which may be for some readers I have a question, "Doesn't Android have five major components? And what about Intent?" Yes, Intent is also very important, but it Just maintain the connection between these components! We will explain Intent in the next chapter! Talking about this ContentProvider, when will we Will he be used? There are two types:
- 1.We want to access other applications in our own application, or some data exposed to us by ContentProvider, Such as mobile phone contacts, text messages, etc.! We want to read or modify this data, so we need to use ContentProvider!
- 2.For our own applications, if we want to expose some of our data for other applications to read or operate, we can also use To ContentProvider, we can also choose the data to be exposed, thus avoiding the leakage of our private data!
It seems like a cheat, but it is actually very simple to use. Let’s learn about ContentProvider~Official Document: ContentProviderIn this section we will Explain the concept of ContentProvder and write some commonly used examples of using system ContentProvider. And a custom ContentProvider!
ContentProvider concept explanation:
2. Use the ContentProvider provided by the system
In fact, many times when we use ContentProvider, we do not expose our own data. More often, we use ContentResolver to read other application information. The most commonly used one is to read system APP information. contact person, Multimedia information and more! If you want to call these ContentProviders, you need to check the relevant API information yourself! In addition, different versions may correspond to different URLs! Here is how to get the URL and the fields of the corresponding database table, Here we take the most commonly used contacts as an example. For others, google~
①Go to the system source code file: all-src.rar -> TeleponeProvider -> AndroidManifest.xml to find the corresponding API
②Open the emulator file explorer/data/data/com.android.providers.contacts/databases/contact2.db After exporting, use the SQLite graphics tool to view the three core tables: raw_contact table, data table, mimetypes table!
The following demonstrates some basic operation examples:
1) Simple reading of inbox information:
Core code:
Uri uri = Uri.parse("content://sms/");
ContentResolver resolver = getContentResolver();
//What is obtained Which columns of information
Cursor cursor = resolver.query(uri, new String[]{"address","date","type","body"}, null, null, null);
while( cursor.moveToNext())
{
String address = cursor.getString(0);
String date = cursor.getString(1);
String type = cursor.getString(2);
String body = cursor.getString(3);
System.out.println("Address:" + address);
System.out.println("Time:" + date);
System .out.println("Type:" + type);
System.out.println("Content:" + body);
System.out.println("========== ============");
}
cursor.close();
}
Don’t forget to add it to AndroidManifest.xml Permission to read inbox:
Running results:
Part of the running results are as follows:
2) Simply add it to the inbox Insert a message
Core code:
ContentResolver resolver = getContentResolver();
Uri uri = Uri .parse("content://sms/");
ContentValues conValues = new ContentValues();
conValues.put("address", "123456789");
conValues.put("type" , 1);
conValues.put("date", System.currentTimeMillis());
conValues.put("body", "no zuo no die why you try!");
resolver. insert(uri, conValues);
Log.e("HeHe", "SMS insertion completed~");
}
Running results:
##Notes:
The above code The function of writing text messages can be realized below 4.4, but it cannot be written on 5.0. The reasons are: Starting from 5.0, software other than the default SMS application cannot send text messages by writing to the SMS database!3) Simple reading of mobile phone contacts
Core code:
ContentResolver resolver = getContentResolver();
Uri uri = ContactsContract.CommonDataKinds.Phone.CONTENT_URI;
//Query contact data
cursor = resolver.query(uri, null, null, null, null);
while(cursor.moveToNext())
{
//Get the contact name, mobile phone number
String cName = cursor. getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DISPLAY_NAME));
String cNum = cursor.getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER));
System.out.println(" Name:" + cName);
System.out.println("Number:" + cNum);
System.out.println("================ ======");
}
cursor.close();
}
Running results:
Part of the running results are as follows:
4) Query the contact information of the specified phone number
Core code:
ContentResolver resolver = getContentResolver();
Cursor cursor = resolver.query(uri, new String[]{"display_name"}, null, null, null);
if (cursor.moveToFirst()) {
String name = cursor.getString(0);
System.out.println(number + "Corresponding contact name:" + name) ;
}
cursor.close();
}
Run result:
##5) Add a new contact
Core code:
//使用事务添加联系人
Uri uri = Uri.parse("content://com.android.contacts/raw_contacts");
Uri dataUri = Uri.parse("content://com.android.contacts/data");
ContentResolver resolver = getContentResolver();
ArrayList<ContentProviderOperation> operations = new ArrayList<ContentProviderOperation>();
ContentProviderOperation op1 = ContentProviderOperation.newInsert(uri)
.withValue("account_name", null)
.build();
operations.add(op1);
//依次是姓名,号码,邮编
ContentProviderOperation op2 = ContentProviderOperation.newInsert(dataUri)
.withValueBackReference("raw_contact_id", 0)
.withValue("mimetype", "vnd.android.cursor.item/name")
.withValue("data2", "Coder-pig")
.build();
operations.add(op2);
ContentProviderOperation op3 = ContentProviderOperation.newInsert(dataUri)
.withValueBackReference("raw_contact_id", 0)
.withValue("mimetype", "vnd.android.cursor.item/phone_v2")
.withValue("data1", "13798988888")
.withValue("data2", "2")
.build();
operations.add(op3);
ContentProviderOperation op4 = ContentProviderOperation.newInsert(dataUri)
.withValueBackReference("raw_contact_id", 0)
.withValue("mimetype", "vnd.android.cursor.item/email_v2")
.withValue("data1", "779878443@qq.com")
.withValue("data2", "2" )
.build();
operations.add(op4);
//Add the above content to the mobile phone contacts~
resolver.applyBatch("com.android.contacts", operations );
Toast.makeText(getApplicationContext(), "Added successfully", Toast.LENGTH_SHORT).show();
}
##Run result:
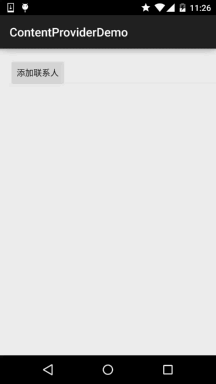
Don’t forget permissions:
3. Custom ContentProvider
We rarely define ContentProvider ourselves because we often don’t want our application data to be exposed to For other applications, despite this, it is still necessary to learn how to use ContentProvider. There is one more way of data transmission, right~
This is a flow chart drawn before:
Next we will implement it step by step:
Before we start, we must first create a database creation class (the database content will be discussed later~):
DBOpenHelper .java
final String CREATE_SQL = "CREATE TABLE test(_id INTEGER PRIMARY KEY AUTOINCREMENT,name)";
public DBOpenHelper(Context context, String name, CursorFactory factory,
int version) {
super(context, name, null, 1);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_SQL);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// TODO Auto-generated method stub
Step 1: Customize the ContentProvider class, implement onCreate(), getType(), and rewrite the corresponding addition, deletion, modification and query methods according to needs:
NameContentProvider.java
//Initialize some constants
private static UriMatcher matcher = new UriMatcher(UriMatcher.NO_MATCH);
private DBOpenHelper dbOpenHelper;
//In order to facilitate the direct use of UriMatcher, addURI here, and then call Matcher to match
static{
matcher.addURI("com.jay.example.providers.myprovider", "test", 1); ## RETURN TRUE;
}
# @OVERRIDE
Public Cursor Query (Uri Uri, String [] Project, String Selection,
String [] Selectionargs, String Ortorder) {
return null;
}
@Override
public String getType(Uri uri) {
return null;
}
@Override
public Uri insert (Uri uri, ContentValues values) {
‐ ‐ ‐ --
sqliteddatabase db = dbopenhelper.getReadabledatabase ();
Long Rowid = db.insert ("test", null, value);//Append ID to the existing Uri
Uri nameUri = ContentUris.withAppendedId(uri, rowId);
Notify that the data has changed
getContext().getContentResolver().notifyChange (nameUri , null);
return nameUri;
}
return null;
}
@Override
public int delete(Uri uri, String selection, String [] selectionArgs) {
return 0;
}
@Override
public int update(Uri uri, ContentValues values, String selection,
String[] selectionArgs) {
return 0;
}
}
Step 2: Register ContentProvider in AndroidManifest.xml:
android:authorities="com.jay.example.providers.myprovider"
android:authorities="com.jay.example.providers.myprovider"
android:exported="true" />
Okay, the part as ContentProvider is complete!
Next, create a new project and let’s implement the ContentResolver part. We insert a piece of data directly by clicking the button:
MainActivity.java
private Button btninsert;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate( savedInstanceState);
setContentView(R.layout.activity_main);
btninsert = (Button) findViewById(R.id.btninsert);
//Read contentprovider data
final ContentResolver resolver = this.getContentResolver (); public void onClick(View v) {
ContentValues values = new ContentValues();
values.put("name", "Test");
Uri uri = Uri.parse("content://com.jay.example.providers.myprovider/test ");
resolver.insert(uri, values);
Toast.makeText(getApplicationContext(), "Data insertion successful", Toast.LENGTH_SHORT).show();
}
});
how to use? Well, the code is quite simple. First run the project as ContentProvider, and then run the project as ContentResolver. Click the button to insert a piece of data, then open file explorer to take out the db database of ContentProvider, and use the graphical viewing tool You can find the inserted data and time relationship by viewing it. The results will not be demonstrated~
4. Monitor the data changes of ContentProvider through ContentObserver
Usage Guide:
After running the program, leave it alone. After receiving the text message, you can see the content of the message on logcat, and you can customize it according to your needs. Change Activtiy to Service and do this kind of thing in the background~
Summary of this section:
Okay, that’s it for the preliminary exploration of ContentProvider. In this section we learned: The concept and process of ContentProvider, using some ContentProvider provided by the system, and customizing your own ContentProvider, Finally, I also explained how to monitor the data changes of ContentProvider through ContentObserver, and the content of ContentProvider is almost mastered. Okay, let’s go through the documentation in the next section to see what we don’t know~Thank you