Swift Operator
An operator is a symbol used to tell the compiler to perform a mathematical or logical operation.
Swift provides the following operators:
Arithmetic operators
Comparison operators
Logical operators
Bitwise operators
Assignment operators
Interval operator
Other operators
In this chapter we will introduce arithmetic operators, relational operators, and logical operations in detail operators, bitwise operators, assignment operators, and other operators.
Arithmetic operators
The following table lists the arithmetic operators supported by the Swift language, where variable A is 10 and variable B is 20:
Operator | Description | Instance |
---|
+ | Plus sign | A + B The result is 30 |
− | Minus sign | A − B The result is -10 |
* | Multiplication sign | A * B The result is 200 |
##/ | Division sign | B / A The result is 2 |
% | Find remainder | B % A The result is 0 |
++ | increment | A++ The result is 11 |
-- | decrement | A --The result is 9 |
Example
The following is a simple example of arithmetic operations:
import Cocoa
var A = 10
var B = 20
print("A + B 结果为:\(A + B)")
print("A - B 结果为:\(A - B)")
print("A * B 结果为:\(A * B)")
print("B / A 结果为:\(B / A)")
A++
print("A++ 后 A 的值为 \(A)")
B--
print("B-- 后 B 的值为 \(B)")
The execution result of the above program is:
A + B 结果为:30
A - B 结果为:-10
A * B 结果为:200
B / A 结果为:2
A++ 后 A 的值为 11
B-- 后 B 的值为 19
Comparison operators
The following table lists the comparison operators supported by the Swift language, where variable A is 10 and variable B is 20:
Operator | Description | Instance |
== | Equals | ( A == B) is false. |
!= | is not equal to | (A != B) is true. |
> | is greater than | (A > B) is false. |
< | is less than | (A < B) is true. |
>= | is greater than or equal to | (A >= B) is false. |
<= | is less than or equal to | (A <= B) is true. |
Example
The following is a simple example of comparison operation:
import Cocoa
var A = 10
var B = 20
print("A == B 结果为:\(A == B)")
print("A != B 结果为:\(A != B)")
print("A > B 结果为:\(A > B)")
print("A < B 结果为:\(A < B)")
print("A >= B 结果为:\(A >= B)")
print("A <= B 结果为:\(A <= B)")
The execution result of the above program is:
A == B 结果为:false
A != B 结果为:true
A > B 结果为:false
A < B 结果为:true
A >= B 结果为:false
A <= B 结果为:true
Logical operators
The following table lists the logical operators supported by the Swift language, where variable A is true and variable B is false:
Operator | Description | Instance |
&& | Logical AND. TRUE if both sides of the operator are TRUE. | (A && B) is false. |
|| | Logical OR. TRUE if at least one of the two sides of the operator is TRUE. | (A || B) is true. |
! | Logical negation. Negating a Boolean value makes true become false and false becomes true. | !(A && B) is true. |
The following is a simple example of logical operation:
import Cocoa
var A = true
var B = false
print("A && B 结果为:\(A && B)")
print("A || B 结果为:\(A || B)")
print("!A 结果为:\(!A)")
print("!B 结果为:\(!B)")
The execution result of the above program is:
A && B 结果为:false
A || B 结果为:true
!A 结果为:false
!B 结果为:true
Bit operators
Bit operators are used to perform binary operations Bitwise operations, ~,&,|,^ are respectively negation, bitwise AND, bitwise AND, bitwise AND and XOR operations, as shown in the following table for examples:
##p | q | p & q | p | q | p ^ q |
##0 0 | 0 | 0 | 0 | | ##0
1 | 0 | 1 | 1 | ##1 |
11 | 1 | 0 | | 1 |
00 | 1 | 1 | | If you specify A = 60; and B = 13;, the binary corresponding to the two variables is: A = 0011 1100
B = 0000 1101 Perform bit operation: operator | Description | Illustration | Example |
---|
& | Bitwise AND. The bitwise AND operator operates on two numbers and returns a new number. Each bit of this number is 1 only if the same bit of both input numbers is 1. | 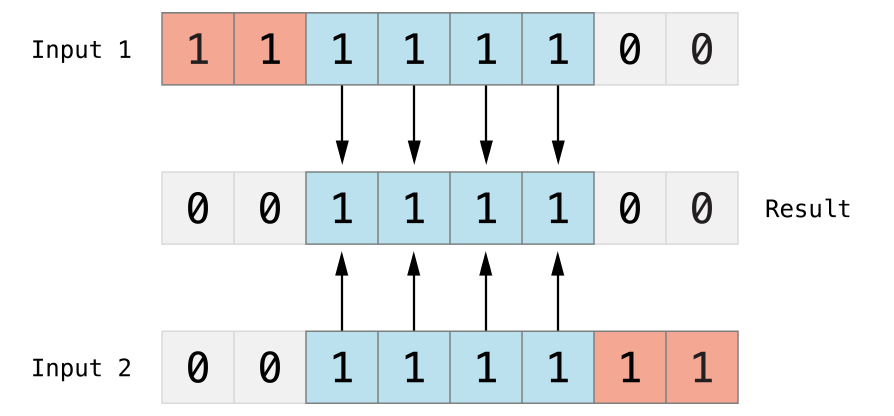 | (A & B) The result is 12, binary is 0000 1100 | | | Bitwise OR . Bitwise OR operator | Compares two numbers, and then returns a new number. The condition for setting each bit of this number to 1 is that the same bit of the two input numbers is not 0 (that is, either one is 1, or both are 1 1). | 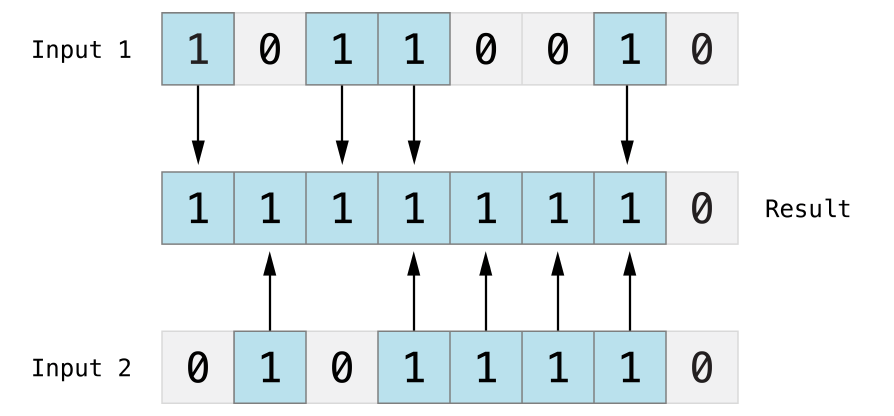 | (A | B) The result is 61, binary is 0011 1101 | ^ | bitwise exclusive Or. The bitwise XOR operator ^ compares two numbers, and then returns a number. The condition for each bit of this number to be set to 1 is that the same bit of the two input numbers is different. If it is the same, it is set to 0. | 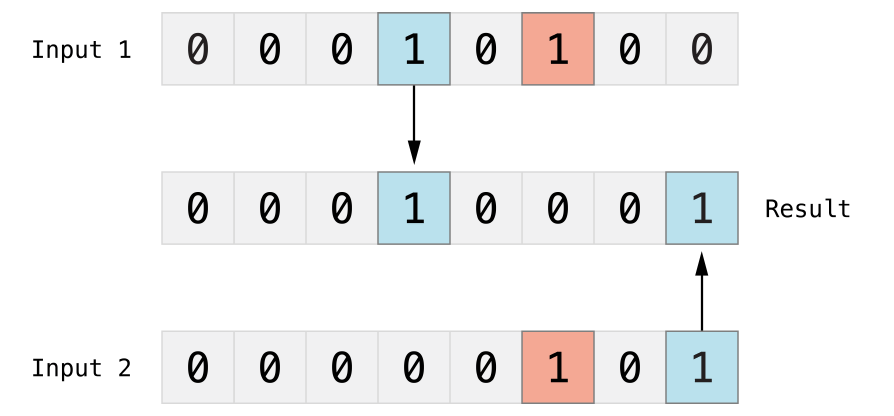 | (A ^ B) The result is 49, binary is 0011 0001 | ##~ | Take bitwise The negation operator ~ negates each bit of an operand. | 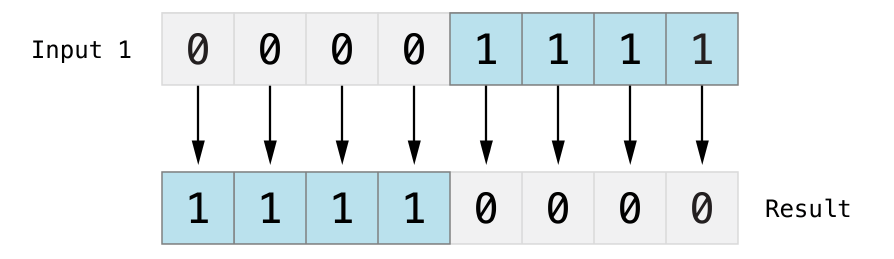 | (~A ) The result is -61, which in binary is 1100 0011 in 2's complement form. | ##<<Bitwise left shift. The left shift operator (<<) shifts all bits of the operand to the left by the specified number of bits. | | The following figure shows the result of 11111111 << 1 (11111111 shifted one bit to the left). Blue numbers represent shifted bits, gray represents discarded bits, and empty bits are filled with orange 0s. A << 2 The result is 240, binary is 1111 0000 | | >>bitwise Move right. The right shift operator (<<) moves all bits of the operand by the specified number of bits. | | The following figure shows the result of 11111111 >> 1 (11111111 shifted one position to the right). Blue numbers represent shifted bits, gray represents discarded bits, and empty bits are filled with orange 0s. 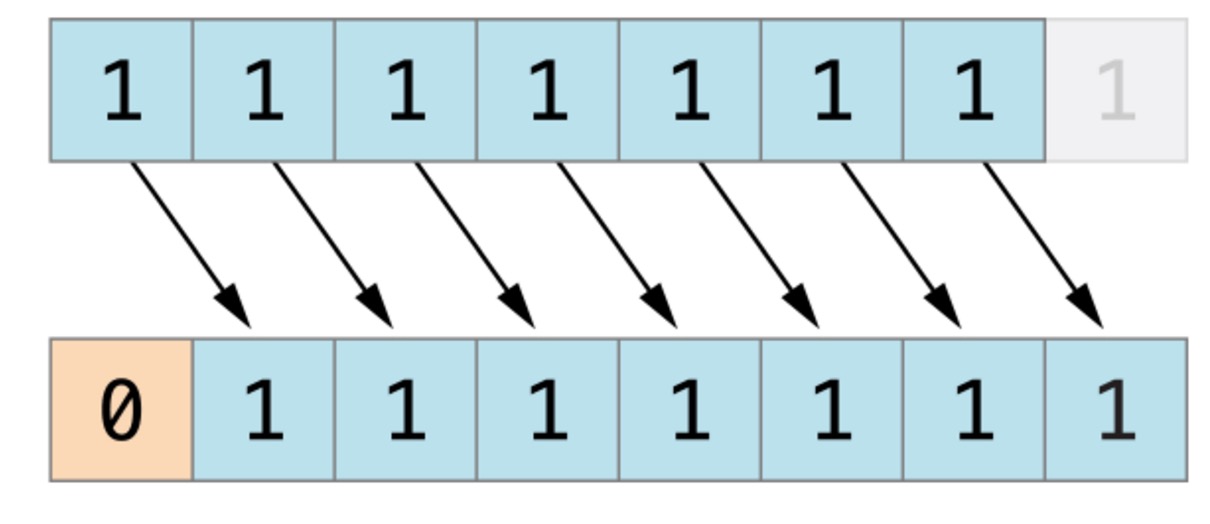 | A >> 2 The result is 15, binary is 0000 1111 |
The following is a simple example of bit operation: import Cocoa
var A = 60 // 二进制为 0011 1100
var B = 13 // 二进制为 0000 1101
print("A&B 结果为:\(A&B)")
print("A|B 结果为:\(A|B)")
print("A^B 结果为:\(A^B)")
print("~A 结果为:\(~A)") The execution result of the above program is: A&B 结果为:12
A|B 结果为:61
A^B 结果为:49
~A 结果为:-61
Assignment operationThe following table lists the basic assignment operations of Swift language: Operator | Description | Instance |
---|
= | Simple assignment operation, assign the right operand to The operand on the left. | C = A + B Assign the operation result of A + B to C | += | , add it and then assign it, add the left and right The operands are added and then assigned to the operand on the left. | C += A is equivalent to C = C + A | -= | subtract and then assign, subtract the left and right operands Then assign the value to the operand on the left. | C -= A is equivalent to C = C - A | *= | . Multiply and then assign. Multiply the left and right operands. Then assign the value to the operand on the left. | C *= A is equivalent to C = C * A | /= | and then assign the value. Divide the left and right operands. Then assign the value to the operand on the left. | C /= A is equivalent to C = C / A | ##%= | Find the remainder and then assign the value. Find the remainder of the left and right operands. Then assign the value to the operand on the left. | C %= A is equivalent to C = C % A | <<= | Shift left bitwise and then assign value | C <<= 2 is equivalent to C = C << 2 | >>= | Shift right bitwise and then assign the value | C >>= 2 is equivalent to C = C >> 2 | ##&=Assignment after bitwise AND operation | C &= 2 is equivalent to C = C & 2 | | ^=bitwise XOR operator and then assignment | C ^= 2 is equivalent to C = C ^ 2 | | |=bitwise OR operation and then assignment | C |= 2 is equivalent In C = C | 2 | | The following is a simple example of assignment operation:
import Cocoa
var A = 10
var B = 20
var C = 100
C = A + B
print("C 结果为:\(C)")
C += A
print("C 结果为:\(C)")
C -= A
print("C 结果为:\(C)")
C *= A
print("C 结果为:\(C)")
C /= A
print("C 结果为:\(C)")
//以下测试已注释,可去掉注释测试每个实例
/*
C %= A
print("C 结果为:\(C)")
C <<= A
print("C 结果为:\(C)")
C >>= A
print("C 结果为:\(C)")
C &= A
print("C 结果为:\(C)")
C ^= A
print("C 结果为:\(C)")
C |= A
print("C 结果为:\(C)")
*/ The execution result of the above program is: C 结果为:30
C 结果为:40
C 结果为:30
C 结果为:300
C 结果为:30 Interval Operator
Swift provides two interval operators. OperatorDescription | Example | | Closed interval operator The closed interval operator (a...b) defines an interval containing all values from a to b (including a and b), and b must be greater than or equal to a. The closed range operator is very useful when iterating over all values in a range, such as in a for-in loop: | 1...5 The range values are 1, 2, 3, 4 and 5 | | Half-open interval operatorHalf-open interval (a..<b) defines an interval from a to b but not including b. It is called a half-open interval because the interval includes the first value but not the last value. | 1..< 5 interval values are 1, 2, 3, and 4 | | The following is a simple example of interval operation: import Cocoa
print("闭区间运算符:")
for index in 1...5 {
print("\(index) * 5 = \(index * 5)")
}
print("半开区间运算符:")
for index in 1..<5 {
print("\(index) * 5 = \(index * 5)")
} The execution result of the above program is: 闭区间运算符:
1 * 5 = 5
2 * 5 = 10
3 * 5 = 15
4 * 5 = 20
5 * 5 = 25
半开区间运算符:
1 * 5 = 5
2 * 5 = 10
3 * 5 = 15
4 * 5 = 20 Other operatorsSwift provides other types of operators, Such as unary, binary and ternary operators. Unary operators operate on a single operand (such as -a ). Unary operators are divided into pre-operators and post-operators. The pre-operator must be immediately before the operation object (such as !b ), and the post-operation operator must be immediately after the operation object (such as i++ ). Binary operators operate on two operands (such as 2 + 3 ) and are centered because they appear between the two operands. The ternary operator operates three operands. Like the C language, Swift has only one ternary operator, which is the ternary operator (a ? b : c ).
Operator | Description | Instance |
---|
One dollar Subtract the | number by adding the - number prefix | -3 or -4 | One dollar plus the | digital money by adding the + number prefix | +6 The result is 6 | Ternary operator | Condition? X : Y | If added is true, the value is X, otherwise Y |
The following are simple examples of unary, binary, and ternary operations: import Cocoa
var A = 1
var B = 2
var C = true
var D = false
print("-A 的值为:\(-A)")
print("A + B 的值为:\(A + B)")
print("三元运算:\(C ? A : B )")
print("三元运算:\(D ? A : B )") The execution result of the above program is: -A 的值为:-1
A + B 的值为:3
三元运算:1
三元运算:2
Operator priorityAn expression may contain multiple data objects of different data types connected by different operators; since the expression has multiple operations, different operation orders may produce different results or even incorrect operation errors, because When an expression contains multiple operations, they must be combined in a certain order to ensure the rationality of the operations and the correctness and uniqueness of the results. The priorities decrease from top to bottom, with the top one having the highest priority and the comma operator having the lowest priority. In the same priority, the calculation is based on the order of combination. Most operations are calculated from left to right, and only three precedence levels are combined from right to left. They are unary operators, conditional operators, and assignment operators. Basic priorities need to be remembered: Pointers are optimal, and monocular operations are better than binocular operations. Such as plus and minus signs. First multiply and divide (modulo), then add and subtract. Arithmetic operation first, then shift operation, and finally bit operation. Please pay special attention to: 1 << 3 + 2 & 7 is equivalent to (1 << (3 + 2))&7 Final calculation of logical operation
Operator type | Operator | Combining direction |
---|
Expression Operation | () [] . expr++ expr-- | Left to right | Unary operator | * & + - ! ~ ++expr --expr * / % + - >> << < > <= >= == != | right to left | bit operator | & ^ | && || | Left to right | Ternary operator | ?: | Right to left | Assignment operator | = += -= *= /= %= >>= <<= &= ^= |= | right to left | comma | , | Left to right |
The following is a simple example of operator precedence: import Cocoa
var A = 0
A = 2 + 3 * 4 % 5
print("A 的值为:\(A)") The execution result of the above program For: A 的值为:4 Instance analysis: According to the operator priority, the operation of the above program can be parsed into the following steps, and the expression is equivalent to: 2 + ((3 * 4) % 5) The first step is to calculate:
(3 * 4) = 12, so the expression is equivalent to:
2 + (12 % 5) The second step is to calculate 12 % 5 = 2, so the expression is equivalent to: 2 + 2 At this point it can be easily seen that the calculated result is 4. |