Python While loop statement
In Python programming, the while statement is used to execute a program in a loop, that is, under certain conditions, execute a certain program in a loop to handle the same task that needs to be processed repeatedly. Its basic form is:
Execution statement...
The execution statement can be a single statement or a statement block. The judgment condition can be any expression, and any non-zero or non-null value is true.
When the judgment condition is false, the loop ends.
The execution flow chart is as follows:
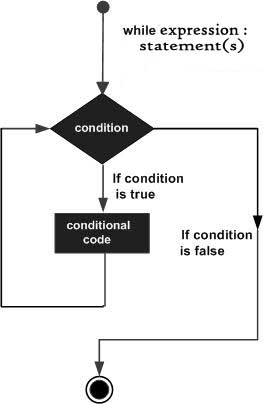
Example:
count = 0
while ( count < 9):
print 'The count is:', count
count = count + 1
print "Good bye!"
The above code execution output result:
The count is : 1
The count is: 2
The count is: 3
The count is: 4
The count is: 5
The count is: 6
The count is: 7
The count is: 8
Good bye!
There are two other important commands in the while statement: continue, break to skip the loop, and continue to skip the loop. , break is used to exit the loop. In addition, the "judgment condition" can also be a constant value, indicating that the loop must be established. The specific usage is as follows:
i = 1
while i < 10:
i += 1
if i%2 > 0: # Skip output when non-even number
Continue
print i # Output even number 2, 4, 6, 8, 10
i = 1
While 1:#cycle condition is 1 must be established
PRINT I#output 1 ~ 10
i+= 1
if i > 10: # Break out of the loop when i is greater than 10
break
Infinite Loop
If the conditional judgment statement is always true, the loop will execute indefinitely, as shown in the following example:
# -*- coding: UTF-8 -*-
var = 1
while var == 1 : # This condition is always true and the loop will execute indefinitely
num = raw_input("Enter a number :")
print "You entered: ", num
print "Good bye!"
Output result of the above example:
Enter a number :20
You entered: 20
Enter a number :29
You entered: 29
Enter a number :3
You entered: 3
Enter a number between :Traceback (most recent call last):
File "test.py", line 5, in <module>
num = raw_input("Enter a number:")
KeyboardInterrupt
Note: You can use CTRL+C to interrupt the infinite loop above.
Looping using else statements
In python, for...else means this. The statements in for are no different from ordinary ones, and the statements in else will be in It is executed when the loop is executed normally (that is, for is not interrupted by break), and the same is true for while...else.
count = 0
while count < 5:
print count, " is less than 5"
count = count + 1
else:
print count, " is not less than 5"
The output result of the above example is:
1 is less than 5
2 is less than 5
3 is less than 5
4 is less than 5
5 is not less than 5
Simple statement group
Similar to the syntax of if statement, if there is only one statement in your while loop body, you can write the statement and while in the same line, As shown below:
flag = 1
while (flag): print 'Given flag is really true!'
print "Good bye!"
Note: You can use CTRL+C to interrupt the infinite loop above.