Python built-in types and common problems
Python's built-in data types are very rich and can be roughly divided into five categories: None, numeric types, sequence types, mapping types and collection types. Below I will introduce them respectively and make a summary for future reference. The table below lists the specific data types under each category. *Note: The content discussed in this article is based on python2, and python3 will be somewhat different*.
1.None
None represents a null object, which is an empty object. If a function does not return a value, a null object is returned. None has no attributes and represents False in Boolean expressions.2.Numeric types
Except for Boolean types, all numeric types are signed. The representation range of integers is between -2147483648 and 2147483647, and long integers can represent any length, limited only by the size of available memory.#整数i = 123i = int(123)#长整数l = 123Ll = long(123)
Floating point number is a double precision type (64bit), which is the same as double in C language. The numerical range is -1.7*10(-308) to 1.7*10(308).
f = 12.3f = float(12.3)
Complex numbers are represented by a pair of floating point numbers, divided into real and imaginary parts, accessed using real and imag, and the imaginary part is suffixed with j or J.
c = 1.0 + 2.3jc = complex(1.0, 2.3)print c.real,c.imag
Boolean values only have two values: True and False, which are mapped to integers 1 and 0 respectively.
b = Falseb = bool(True)
2.1. Numerical operations
In addition to Boolean types, the operations that can be used for int, long, float and complex are: addition, subtraction, multiplication, division, Division, exponentiation and remainder. Examples are given below.>>> 1 + 2 # 加法3>>> 1 - 2 # 减法-1>>> 2 * 3 # 乘6>>> 2 / 4 # 整数除0>>> 2 / 4.0 # 浮点除0.5>>> 3 // 2.0 # 整除1.0>>> 2 % 3 # 取余2>>> 3 ** 2 # 幂运算 =3^29
Bit operations can also be performed on integers. There are 6 kinds of bit operations: negation (~), left shift (<<), right shift (>>) , exclusive OR (^), bitwise OR (|), bitwise AND (&).
>>> ~1 # 1取反后为-2的补码-2>>> 8 << 1 # 左移16>>> 8 >> 2 # 右移2>>> 1 ^ 3 # 01 和 11 异或得到10,就是22>>> 1 | 3 # 01 和 11 位或得到11,就是33>>> 1 & 3 # 01 和 11 位与得到01,就是11
Boolean types can be evaluated through Boolean expressions. There are six Boolean comparison operators: less than (<), less than or equal to (<=), greater than (>), greater than or equal to (> =), equal to (==), not equal to (!=).
>>> 1 < 2True>>> 1 <= 2True>>> 1 > 2False>>> 1 >= 2False>>> 1 == 2False>>> 1 != 2True
Logical operations can also be performed on Boolean types. There are three types of operations: logical NOT (not), logical NOT-OR (or), and logical AND (and).
>>> not TrueFalse>>> True or FalseTrue>>> True and FalseFalse
2.2. Commonly used numerical type functions
abs(): Returns the absolute value of the given object.>>> abs(-2)2
divmod(): Combines division and remainder operations, returning a tuple containing the quotient and remainder.
>>> divmod(10, 3)(3, 1)
pow(): two parameters, power operation. Three parameters, take the remainder after exponentiation.
>>> pow(2, 5),pow(2, 5, 10)(32, 2)
round(): rounding
>>> round(3.4),round(3.6)(3.0, 4.0)
floor(): round down, ceil(): round up, sqrt(): square root, requires math module.
>>> from math import floor,ceil,sqrt>>> floor(3.6),ceil(3.6),sqrt(9)(3.0, 4.0, 3.0)
conjugate(): Conjugate complex numbers
>>> c = 2 + 3j>>> c.conjugate()(2-3j)
3. Sequence type
Sequence represents an ordered collection of objects whose index is a non-negative number, Includes strings, lists, and tuples. Strings are sequences of characters, lists and tuples are sequences of arbitrary objects. Strings and tuples are immutable, whereas lists can be inserted, deleted, and replaced. All sequences support iteration.3.1. String
Creating a string is very simple, but there are many ways to represent a string.s = 'string's = "string" # 和上面单引号一样s = '''string''' # 三引号之间的内容都被保留,用于多行输入s = r"string" # 原生字符,关掉字符串中的转义。s = u"string" # unicode字符串
There are many methods for strings, but they will not change the contents of the string. The commonly used methods are as shown in the following table.
>>> "Here is {0},I'm {name}.".format("wuyuans.com", name="wuyuan")"Here is wuyuans.com,I'm wuyuan"
3.2. Lists and tuples
The content of the list is variable and can contain any object, represented by square brackets.The content of the tupleImmutable can contain any object and is represented by parentheses.
l = [1, 2, 3, '4', '5'] # 列表l = list((1, 2, 3, '4', '5'))t = (1, 2, 3, '4', '5') # 元组t = tuple("1234")
The methods of the list are mainly used to change the content inside. The commonly used methods of the list are listed below.
>>> l = [1, 2, 3, 4, 5]>>> del l[1]>>> l[1, 3, 4, 5]
3.3. Sequence general operations
All sequences can be accessed by index. The index of the first element is 0, -1 is the last element, and -2 is the last element. Two, once and so on.Slicing operation: Specify the index range and return the corresponding subsequence.
There are also some commonly used ones, as shown below
>>> t = (1, 2, 3, 4, 5)>>> l = [1, 2, 3, 4, 5]>>> t[0]1>>> t[1:4] # 索引1~4(2, 3, 4)>>> t[1:4:2] # 索引1~4,步进为2(2, 4)>>> len(t) # 序列长度5>>> max(t) # 最大值5>>> min(t) # 最小值1>>> sum(t) # 序列求和15
3.4.xrange() object
xrange() object is special. It represents an integer range, and its value will only be calculated when accessing it, so slicing operations cannot be used for xrange objects. The xrange object is created by the xrange([i, ] j [, stride]) function, where i is the starting value and stride is the step value.>>> x = xrange(1,10,2)>>> for i in x: print i 13579
4. Mapping type
Dictionary (dict) is python’s only built-in mapping type. Any immutable object can be used as Dictionary key values, such as strings, numbers, tuples, etc. Dictionaries are represented by curly braces, keys and values are separated by colons, and each key value is separated by commas. Map objects are unordered.d = dict((['name', 'wuyuan'], ['age', 23]))d = {'name': 'wuyuan', 'blog': 'wuyuans.com', 'age': 23}d['school'] = 'HDU' # 添加一项
Common methods and operations of dictionaries
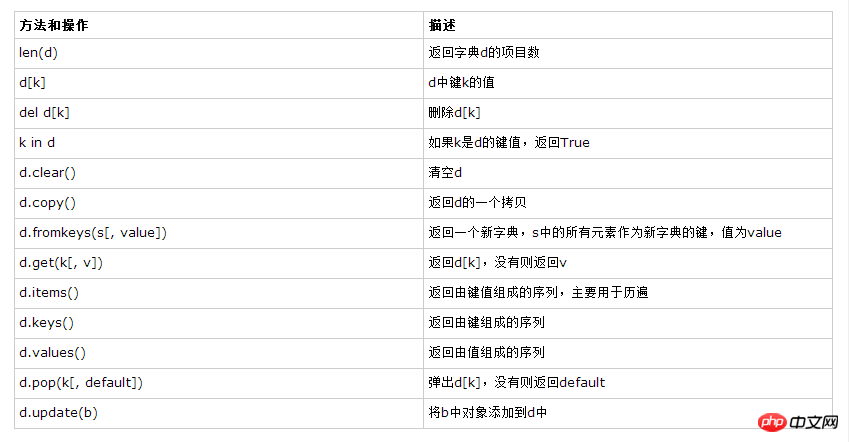
#使用键历遍for i in d: print i,d[i]#使用键值历遍for k,v in d.items(): print k,v
5. Collection type
A set is an unordered set of unique items. The set does not provide indexing or slicing operations. The length of the set is variable, but the items placed inside must be immutable. There are two types of sets: mutable sets (sets) and immutable sets (frozensets).s = set([1, 2, 3])fs = frozenset([1, 2, 3])
General methods and operations of collections
FAQ about python built-in types
What are the basic built-in data types in python?
Some basic data types, such as: integer (number), string, tuple, list, dictionary and Boolean type. As the learning progress deepens, everyone will come into contact with more and more interesting data types. Beginners of Python can just understand these types first when getting started.
Corresponding symbols for basic built-in data types?
1) Integer type——int——Number
Python has 5 number types, the most common one is the integer type. For example: 1234, -1234
2) Boolean type - bool - represented by the symbol ==
Boolean type is a special python number type. It has only two values, True and False. It is mainly used for comparison and judgment, and the result is called a Boolean value. For example: 3==3 gives True, 3==5 gives False
3) String - str - use ' ' or " " to represent
For example: 'www.phpcnpython.com' or "hello"
4) List - list - represented by [ ] symbol
For example: [1, 2,3,4]
5) Tuple - tuple - represented by ( ) symbol
For example: ('d',300)
6) Dictionary - dict - represented by { } symbol
For example: {'name':'coco','country':'china'}
Which of the basic data types in Python are mutable and which are immutable?
python variable data type: list list[ ], dictionary dict{ }
python immutable data type: int, character String str' ', tuple tuple()