ionic Create APP
In the previous chapters we have learned how to import the ionic framework into the project.
Next we will introduce how to create an ionic APP application.
ionic creates APP using HTML, CSS and Javascript to build, so we can create a www directory and create an index.html file in the directory. The code is as follows:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Todo</title> <meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no, width=device-width"> <link href="lib/ionic/css/ionic.css" rel="stylesheet"> <script src="lib/ionic/js/ionic.bundle.js"></script> <!-- 在使用 Cordova/PhoneGap 创建的 APP 中包含的文件,由 Cordova/PhoneGap 提供,(开发过程中显示 404) --> <script src="cordova.js"></script> </head> <body> </body> </html>
In the above code, We introduced Ionic CSS files, Ionic JS files and Ionic AngularJS extension ionic.bundle.js (ionic.bundle.js).
ionic.bundle.js file already contains Ionic core JS, AngularJS, and Ionic’s AngularJS extension. If you need to introduce other Angular modules, you can call them from the lib/js/angular directory.
cordova.js is generated when using Cordova/PhoneGap to create an application. It does not need to be introduced manually. You can find this file in the Cordova/PhoneGap project, so it is normal to display 404 during the development process.
Create APP
Next we will implement an application including title, sidebar, list, etc. The design diagram is as follows:
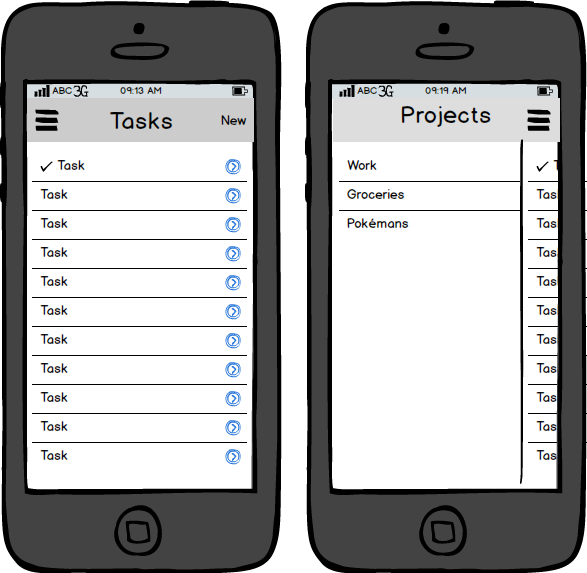
Create side Sidebar
The sidebar is created using the ion-side-menus controller.
Edit the index.html file we created previously and modify the content within <body> as follows:
<body> <ion-side-menus> <ion-side-menu-content> </ion-side-menu-content> <ion-side-menu side="left"> </ion-side-menu> </ion-side-menus> </body>
Controller parsing:
ion-side-menus: is a container with a sidebar menu, which can be expanded by clicking a button or sliding the screen.
ion-side-menu-content: A container that displays the main content. The menu can be expanded by sliding the screen.
ion-side-menu: Container for storing sidebar.
Initialize APP
Next we create a new AngularJS module and initialize it. The code is located in www/js/app.js:
angular.module('todo', ['ionic'])
<body ng-app="todo">
<script src="cordova.js"> in the index.html file ;</script> The app.js file is introduced above:
<script src="js/app.js"></script>
Modify the content of the body tag of the index.html file, the code is as follows:
<body ng-app="todo"> <ion-side-menus> <!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <h1 class="title">Todo</h1> </ion-header-bar> <ion-content> </ion-content> </ion-side-menu-content> <!-- 左侧菜单 --> <ion-side-menu side="left"> <ion-header-bar class="bar-dark"> <h1 class="title">Projects</h1> </ion-header-bar> </ion-side-menu> </ion-side-menus> </body>
We have completed the application of ionic basic APP in the above steps.
Create a list
First create a controllerTodoCtrl:
<body ng-app="todo" ng-controller="TodoCtrl">
In the app.js file, use the controller to define the list data :
angular.module('todo', ['ionic']) .controller('TodoCtrl', function($scope) { $scope.tasks = [ { title: 'php中文网' }, { title: 'www.php.cn' }, { title: 'php中文网' }, { title: 'www.php.cn' } ]; });
In the data list in the index.html page, we use Angular ng-repeat to iterate the data:
<!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <h1 class="title">Todo</h1> </ion-header-bar> <ion-content> <!-- 列表 --> <ion-list> <ion-item ng-repeat="task in tasks"> {{task.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu-content>
The code in the modified index.html body tag is as follows:
<body ng-app="todo" ng-controller="TodoCtrl"> <ion-side-menus> <!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <h1 class="title">Todo</h1> </ion-header-bar> <ion-content> <!-- 列表 --> <ion-list> <ion-item ng-repeat="task in tasks"> {{task.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu-content> <!-- 左侧菜单 --> <ion-side-menu side="left"> <ion-header-bar class="bar-dark"> <h1 class="title">Projects</h1> </ion-header-bar> </ion-side-menu> </ion-side-menus> <script src="http://www.php.cn/static/ionic/js/app.js"></script> <script src="cordova.js"></script> </body>
Create and add a page
Modify index.html and add the following code after </ion-side-menus>:
<script id="new-task.html" type="text/ng-template"> <div class="modal"> <!-- Modal header bar --> <ion-header-bar class="bar-secondary"> <h1 class="title">New Task</h1> <button class="button button-clear button-positive" ng-click="closeNewTask()">Cancel</button> </ion-header-bar> <!-- Modal content area --> <ion-content> <form ng-submit="createTask(task)"> <div class="list"> <label class="item item-input"> <input type="text" placeholder="What do you need to do?" ng-model="task.title"> </label> </div> <div class="padding"> <button type="submit" class="button button-block button-positive">Create Task</button> </div> </form> </ion-content> </div> </script>
In the above code, we define a new template page through<script id="new-task.html" type="text/ng-template">.
The createTask(task) function is triggered when the form is submitted.
ng-model="task.title" will assign the input data of the form to the title attribute of the task object.
Modify the content in <ion-side-menu-content>, the code is as follows:
<!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <h1 class="title">Todo</h1> <!-- 新增按钮--> <button class="button button-icon" ng-click="newTask()"> <i class="icon ion-compose"></i> </button> </ion-header-bar> <ion-content> <!-- 列表 --> <ion-list> <ion-item ng-repeat="task in tasks"> {{task.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu-content>
In the app.js file, the controller code is as follows:
angular.module('todo', ['ionic']) .controller('TodoCtrl', function($scope, $ionicModal) { $scope.tasks = [ { title: 'php中文网' }, { title: 'www.php.cn' }, { title: 'php中文网' }, { title: 'www.php.cn' } ]; // 创建并载入模型 $ionicModal.fromTemplateUrl('new-task.html', function(modal) { $scope.taskModal = modal; }, { scope: $scope, animation: 'slide-in-up' }); // 表单提交时调用 $scope.createTask = function(task) { $scope.tasks.push({ title: task.title }); $scope.taskModal.hide(); task.title = ""; }; // 打开新增的模型 $scope.newTask = function() { $scope.taskModal.show(); }; // 关闭新增的模型 $scope.closeNewTask = function() { $scope.taskModal.hide(); }; });
Create a sidebar
Through the above steps, we have implemented new functions, and now we add sidebar functions to the app.
Modify the content in <ion-side-menu-content>, the code is as follows:<!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <button class="button button-icon" ng-click="toggleProjects()"> <i class="icon ion-navicon"></i> </button> <h1 class="title">{{activeProject.title}}</h1> <!-- 新增按钮 --> <button class="button button-icon" ng-click="newTask()"> <i class="icon ion-compose"></i> </button> </ion-header-bar> <ion-content scroll="false"> <ion-list> <ion-item ng-repeat="task in activeProject.tasks"> {{task.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu-content>
Add a sidebar:
<!-- 左边栏 --> <ion-side-menu side="left"> <ion-header-bar class="bar-dark"> <h1 class="title">Projects</h1> <button class="button button-icon ion-plus" ng-click="newProject()"> </button> </ion-header-bar> <ion-content scroll="false"> <ion-list> <ion-item ng-repeat="project in projects" ng-click="selectProject(project, $index)" ng-class="{active: activeProject == project}"> {{project.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu># The ng-class directive in ##<ion-item> sets the menu activation style. Here we create a new js file app2.js (in order not to be confused with the previous one), the code is as follows:
angular.module('todo', ['ionic']) /** * The Projects factory handles saving and loading projects * from local storage, and also lets us save and load the * last active project index. */ .factory('Projects', function() { return { all: function() { var projectString = window.localStorage['projects']; if(projectString) { return angular.fromJson(projectString); } return []; }, save: function(projects) { window.localStorage['projects'] = angular.toJson(projects); }, newProject: function(projectTitle) { // Add a new project return { title: projectTitle, tasks: [] }; }, getLastActiveIndex: function() { return parseInt(window.localStorage['lastActiveProject']) || 0; }, setLastActiveIndex: function(index) { window.localStorage['lastActiveProject'] = index; } } }) .controller('TodoCtrl', function($scope, $timeout, $ionicModal, Projects, $ionicSideMenuDelegate) { // A utility function for creating a new project // with the given projectTitle var createProject = function(projectTitle) { var newProject = Projects.newProject(projectTitle); $scope.projects.push(newProject); Projects.save($scope.projects); $scope.selectProject(newProject, $scope.projects.length-1); } // Load or initialize projects $scope.projects = Projects.all(); // Grab the last active, or the first project $scope.activeProject = $scope.projects[Projects.getLastActiveIndex()]; // Called to create a new project $scope.newProject = function() { var projectTitle = prompt('Project name'); if(projectTitle) { createProject(projectTitle); } }; // Called to select the given project $scope.selectProject = function(project, index) { $scope.activeProject = project; Projects.setLastActiveIndex(index); $ionicSideMenuDelegate.toggleLeft(false); }; // Create our modal $ionicModal.fromTemplateUrl('new-task.html', function(modal) { $scope.taskModal = modal; }, { scope: $scope }); $scope.createTask = function(task) { if(!$scope.activeProject || !task) { return; } $scope.activeProject.tasks.push({ title: task.title }); $scope.taskModal.hide(); // Inefficient, but save all the projects Projects.save($scope.projects); task.title = ""; }; $scope.newTask = function() { $scope.taskModal.show(); }; $scope.closeNewTask = function() { $scope.taskModal.hide(); } $scope.toggleProjects = function() { $ionicSideMenuDelegate.toggleLeft(); }; // Try to create the first project, make sure to defer // this by using $timeout so everything is initialized // properly $timeout(function() { if($scope.projects.length == 0) { while(true) { var projectTitle = prompt('Your first project title:'); if(projectTitle) { createProject(projectTitle); break; } } } }); });The ion-side-menus code in the body is as follows::
<ion-side-menus> <!-- 中心内容 --> <ion-side-menu-content> <ion-header-bar class="bar-dark"> <button class="button button-icon" ng-click="toggleProjects()"> <i class="icon ion-navicon"></i> </button> <h1 class="title">{{activeProject.title}}</h1> <!-- 新增按钮 --> <button class="button button-icon" ng-click="newTask()"> <i class="icon ion-compose"></i> </button> </ion-header-bar> <ion-content scroll="false"> <ion-list> <ion-item ng-repeat="task in activeProject.tasks"> {{task.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu-content> <!-- 左边栏 --> <ion-side-menu side="left"> <ion-header-bar class="bar-dark"> <h1 class="title">Projects</h1> <button class="button button-icon ion-plus" ng-click="newProject()"> </button> </ion-header-bar> <ion-content scroll="false"> <ion-list> <ion-item ng-repeat="project in projects" ng-click="selectProject(project, $index)" ng-class="{active: activeProject == project}"> {{project.title}} </ion-item> </ion-list> </ion-content> </ion-side-menu> </ion-side-menus>