PHP editor Xigua sering menghadapi masalah program tergantung semasa proses pengaturcaraan. Program hang bermakna program tiba-tiba berhenti bertindak balas semasa pelaksanaan tanpa sebarang mesej ralat. Keadaan ini sering menyebabkan orang keliru tentang apa yang salah. Kenapa sebenarnya program ini digantung? Dalam artikel ini, kami akan meneroka beberapa punca biasa program hang dan menyediakan penyelesaian untuk membantu menyelesaikan isu tersebut. Sama ada anda seorang pemula atau pembangun berpengalaman, saya percaya kandungan ini boleh membantu anda.
Kandungan soalan
Saya mempunyai kod untuk berkomunikasi antara saluran dalam go. Ia seolah-olah melakukan apa yang ia mahu, tetapi tergantung pada akhirnya. Saya cuba mendiagnosis mengapa ia tergantung.
Kod ini menggunakan httpbin.org
untuk mendapatkan uuid rawak dan kemudian menerbitkannya sambil mematuhi konkurensi dan had kadar yang saya tetapkan melalui saluran semaphore dan saluran kadar.
package main import ( "bytes" "encoding/json" "fmt" "io" "net/http" "sync" "time" ) type HttpBinGetRequest struct { url string } type HttpBinGetResponse struct { Uuid string `json:"uuid"` StatusCode int } type HttpBinPostRequest struct { url string uuid string // Item to post to API } type HttpBinPostResponse struct { Data string `json:"data"` StatusCode int } func main() { // Prepare GET requests for n requests var requests []*HttpBinGetRequest for i := 0; i < 10; i++ { uri := "https://httpbin.org/uuid" request := &HttpBinGetRequest{ url: uri, } requests = append(requests, request) } // Create semaphore and rate limit for the GET endpoint getSemaphore := make(chan struct{}, 10) getRate := make(chan struct{}, 10) defer close(getRate) defer close(getSemaphore) for i := 0; i < cap(getRate); i++ { getRate <- struct{}{} } go func() { // ticker corresponding to 1/nth of a second // where n = rate limit // basically (1000 / rps) * time.Millisecond ticker := time.NewTicker(100 * time.Millisecond) defer ticker.Stop() for range ticker.C { _, ok := <-getRate if !ok { return } } }() // Send our GET requests to obtain a random UUID respChan := make(chan HttpBinGetResponse) var wg sync.WaitGroup for _, request := range requests { wg.Add(1) // cnt := c // Go func to make request and receive the response go func(r *HttpBinGetRequest) { defer wg.Done() // Check the rate limiter and block if it is empty getRate <- struct{}{} // fmt.Printf("Request #%d at: %s\n", cnt, time.Now().UTC().Format("2006-01-02T15:04:05.000Z07:00")) resp, _ := get(r, getSemaphore) fmt.Printf("%+v\n", resp) // Place our response into the channel respChan <- *resp // fmt.Printf("%+v,%s\n", resp, time.Now().UTC().Format("2006-01-02T15:04:05.000Z07:00")) }(request) } // Set up for POST requests 10/s postSemaphore := make(chan struct{}, 10) postRate := make(chan struct{}, 10) defer close(postRate) defer close(postSemaphore) for i := 0; i < cap(postRate); i++ { postRate <- struct{}{} } go func() { // ticker corresponding to 1/nth of a second // where n = rate limit // basically (1000 / rps) * time.Millisecond ticker := time.NewTicker(100 * time.Millisecond) defer ticker.Stop() for range ticker.C { _, ok := <-postRate if !ok { return } } }() // Read responses as they become available for ele := range respChan { postReq := &HttpBinPostRequest{ url: "https://httpbin.org/post", uuid: ele.Uuid, } go func(r *HttpBinPostRequest) { postRate <- struct{}{} postResp, err := post(r, postSemaphore) if err != nil { fmt.Println(err) } fmt.Printf("%+v\n", postResp) }(postReq) } wg.Wait() close(respChan) } func get(hbgr *HttpBinGetRequest, sem chan struct{}) (*HttpBinGetResponse, error) { // Add a token to the semaphore sem <- struct{}{} // Remove token when function is complete defer func() { <-sem }() httpResp := &HttpBinGetResponse{} client := &http.Client{} req, err := http.NewRequest("GET", hbgr.url, nil) if err != nil { fmt.Println("error making request") return httpResp, err } req.Header = http.Header{ "accept": {"application/json"}, } resp, err := client.Do(req) if err != nil { fmt.Println(err) fmt.Println("error getting response") return httpResp, err } // Read Response body, err := io.ReadAll(resp.Body) if err != nil { fmt.Println("error reading response body") return httpResp, err } json.Unmarshal(body, &httpResp) httpResp.StatusCode = resp.StatusCode return httpResp, nil } // Method to post data to httpbin func post(hbr *HttpBinPostRequest, sem chan struct{}) (*HttpBinPostResponse, error) { // Add a token to the semaphore sem <- struct{}{} defer func() { <-sem }() httpResp := &HttpBinPostResponse{} client := &http.Client{} req, err := http.NewRequest("POST", hbr.url, bytes.NewBuffer([]byte(hbr.uuid))) if err != nil { fmt.Println("error making request") return httpResp, err } req.Header = http.Header{ "accept": {"application/json"}, } resp, err := client.Do(req) if err != nil { fmt.Println("error getting response") return httpResp, err } // Read Response body, err := io.ReadAll(resp.Body) if err != nil { fmt.Println("error reading response body") return httpResp, err } json.Unmarshal(body, &httpResp) httpResp.StatusCode = resp.StatusCode return httpResp, nil }
Penyelesaian
Anda sedang membaca kandungan melalui range
语句从代码末尾的 respchan
. Kod ini tidak keluar sehingga saluran ditutup - yang berlaku selepas blok kod ini.
for ele := range respchan { // ... } wg.wait() close(respchan)
Jadi program tidak pernah keluar - kerana semua logik ini berada dalam goroutine yang sama.
Untuk membetulkan dan memastikan semua rekod diproses sebelum program keluar, simpan kod bacaan saluran dalam goroutine utama dan letakkan logik tunggu/tutup ke dalam goroutinenya sendiri:
go func() { wg.wait() // wait for workers to finish ... close(respchan) // ... now signal the main goroutine we're done }() for ele := range respchan { // ... }
Edit untuk menunggu perlawanan akhir range
mana-mana goroutine kanak-kanak dalam gelung - mungkin ada cara yang lebih bersih untuk hanya menggunakan kumpulan menunggu, tetapi penyelesaian pantas mungkin:
var swg sync.WaitGroup go func() { wg.Wait() // wait for workers to finish ... swg.Wait() // ... and sub-tasks close(respChan) // ... now signal the main goroutine we're done }() for ele := range respChan { // ... swg.Add(1) go func() { defer swg.Done() // ... }() }
Atas ialah kandungan terperinci Kenapa program ni hang?. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!
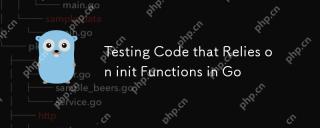
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
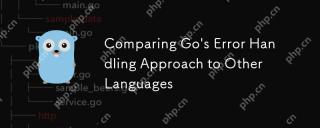
Go'SerrorHandlingReturnSerrorsArvalues, tidak seperti yang tidak seperti ini) go'SmethodensurexplIciterRorHandling, promMORPORUSustCodeBUtinceangeRningSniSik
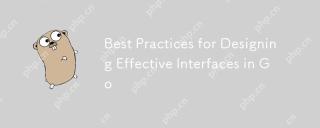
AneffectiveInterfaceingoisminimal, clear, andpromotesloosecoupling.1) minimizetheinterforflexabilityandeaseofimplementation.2) userInterfacesforabstractionToSwapImplementationswithoutchangingcallingcode.3) Rekabentuk DesignForTabilitybyPrementeMaceStomockDePdePode.3) Rekabentuk
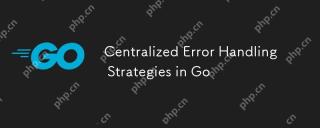
Pengendalian ralat berpusat boleh meningkatkan kebolehbacaan dan mengekalkan kod dalam bahasa Go. Kaedah dan kelebihan pelaksanaannya termasuk: 1. 2. Memastikan konsistensi pengendalian kesilapan dengan pengendalian berpusat. 3. Gunakan menangguhkan dan pulih untuk menangkap dan memproses panik untuk meningkatkan ketahanan program.
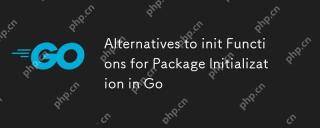
Ingo, alternativestoinitfunctionsincludecustominitialializationFunctionsandsingletons.1) custominitializationFunctionsAllowExplicitControloverwhenitializationoccurs, Constfordelayedorconditionalsetups.2) singletonsensenoneone-timeinitialializationcurncurncurmentasi
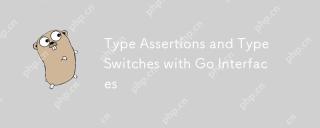
Gohandlesinterfacesandtypeassertionsefectively, enhancingcodeflexabilityandrobustness.1) snossersertionsallowruntimetypechecking, asseenwiththeshaphapeinterfaceandcircletype.2) typeWitchhandLemultipypeseficeMemently, gunaurvariousshapePhipeMemently, gunaurvariousshapePhipeMemently, gunaurvariousshapePhipeMemently, bermanfaat untuk
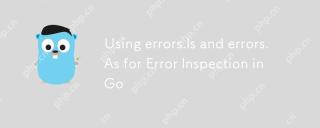
GO pengendalian kesilapan bahasa menjadi lebih fleksibel dan boleh dibaca melalui kesilapan dan kesilapan.as fungsi. 1.errors.is digunakan untuk memeriksa sama ada ralat adalah sama dengan ralat yang ditentukan dan sesuai untuk pemprosesan rantaian ralat. 2.Errors.as bukan sahaja boleh menyemak jenis ralat, tetapi juga menukar ralat kepada jenis tertentu, yang mudah untuk mengekstrak maklumat ralat. Menggunakan fungsi ini dapat memudahkan logik pengendalian ralat, tetapi perhatikan penyampaian rantaian ralat yang betul dan mengelakkan kebergantungan yang berlebihan untuk mencegah kerumitan kod.
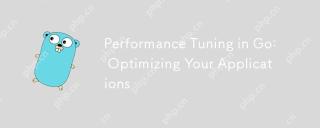
Tomakeoapplicationsrunfasterandmoreeficiently, useprofilingtools, leverageconcurrency, andmanagememoryeffectively.1) usepprofforcpuandmemoryprofiloidentificybottlenecks.2)


Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Penyesuai Pelayan SAP NetWeaver untuk Eclipse
Integrasikan Eclipse dengan pelayan aplikasi SAP NetWeaver.
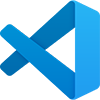
VSCode Windows 64-bit Muat Turun
Editor IDE percuma dan berkuasa yang dilancarkan oleh Microsoft

SublimeText3 Linux versi baharu
SublimeText3 Linux versi terkini

mPDF
mPDF ialah perpustakaan PHP yang boleh menjana fail PDF daripada HTML yang dikodkan UTF-8. Pengarang asal, Ian Back, menulis mPDF untuk mengeluarkan fail PDF "dengan cepat" dari tapak webnya dan mengendalikan bahasa yang berbeza. Ia lebih perlahan dan menghasilkan fail yang lebih besar apabila menggunakan fon Unicode daripada skrip asal seperti HTML2FPDF, tetapi menyokong gaya CSS dsb. dan mempunyai banyak peningkatan. Menyokong hampir semua bahasa, termasuk RTL (Arab dan Ibrani) dan CJK (Cina, Jepun dan Korea). Menyokong elemen peringkat blok bersarang (seperti P, DIV),
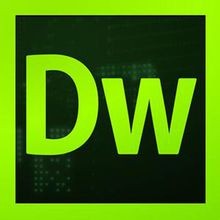
Dreamweaver CS6
Alat pembangunan web visual
