首先在web.config | app.config 文件下增加如下代码:
IV:加密算法的初始向量。
Key:加密算法的密钥。
接着新建类CryptoHelper,作为加密帮助类。
首先要从配置文件中得到IV 和Key。所以基本代码如下:
public class CryptoHelper
{
//private readonly string IV = "SuFjcEmp/TE=";
private readonly string IV = string.Empty;
//private readonly string Key = "KIPSToILGp6fl+3gXJvMsN4IajizYBBT";
private readonly string Key = string.Empty;
///
///构造函数
///
public CryptoHelper()
{
IV = ConfigurationManager.AppSettings["IV"];
Key = ConfigurationManager.AppSettings["Key"];
}
}
注意添加System.Configuration.dll程序集引用。
在获得了IV 和Key 之后,需要获取提供加密服务的Service 类。
在这里,使用的是System.Security.Cryptography; 命名空间下的TripleDESCryptoServiceProvider类。
获取TripleDESCryptoServiceProvider 的方法如下:
///
/// 获取加密服务类
///
///
private TripleDESCryptoServiceProvider GetCryptoProvider()
{
TripleDESCryptoServiceProvider provider = new TripleDESCryptoServiceProvider();
provider.IV = Convert.FromBase64String(IV);
provider.Key = Convert.FromBase64String(Key);
return provider;
}
TripleDESCryptoServiceProvider 两个有用的方法
CreateEncryptor:创建对称加密器对象ICryptoTransform.
CreateDecryptor:创建对称解密器对象ICryptoTransform
加密器对象和解密器对象可以被CryptoStream对象使用。来对流进行加密和解密。
cryptoStream 的构造函数如下:
public CryptoStream(Stream stream, ICryptoTransform transform, CryptoStreamMode mode);
使用transform 对象对stream 进行转换。
完整的加密字符串代码如下:
///
/// 获取加密后的字符串
///
/// 输入值.
///
public string GetEncryptedValue(string inputValue)
{
TripleDESCryptoServiceProvider provider = this.GetCryptoProvider();
// 创建内存流来保存加密后的流
MemoryStream mStream = new MemoryStream();
// 创建加密转换流
CryptoStream cStream = new CryptoStream(mStream,
provider.CreateEncryptor(), CryptoStreamMode.Write);
// 使用UTF8编码获取输入字符串的字节。
byte[] toEncrypt = new UTF8Encoding().GetBytes(inputValue);
// 将字节写到转换流里面去。
cStream.Write(toEncrypt, 0, toEncrypt.Length);
cStream.FlushFinalBlock();
// 在调用转换流的FlushFinalBlock方法后,内部就会进行转换了,此时mStream就是加密后的流了。
byte[] ret = mStream.ToArray();
// Close the streams.
cStream.Close();
mStream.Close();
//将加密后的字节进行64编码。
return Convert.ToBase64String(ret);
}
解密方法也类似:
///
/// 获取解密后的值
///
/// 经过加密后的字符串.
///
public string GetDecryptedValue(string inputValue)
{
TripleDESCryptoServiceProvider provider = this.GetCryptoProvider();
byte[] inputEquivalent = Convert.FromBase64String(inputValue);
// 创建内存流保存解密后的数据
MemoryStream msDecrypt = new MemoryStream();
// 创建转换流。
CryptoStream csDecrypt = new CryptoStream(msDecrypt,
provider.CreateDecryptor(),
CryptoStreamMode.Write);
csDecrypt.Write(inputEquivalent, 0, inputEquivalent.Length);
csDecrypt.FlushFinalBlock();
csDecrypt.Close();
//获取字符串。
return new UTF8Encoding().GetString(msDecrypt.ToArray());
}
完整的CryptoHelper代码如下:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Security.Cryptography;
using System.IO;
using System.Configuration;
namespace WindowsFormsApplication1
{
public class CryptoHelper
{
//private readonly string IV = "SuFjcEmp/TE=";
private readonly string IV = string.Empty;
//private readonly string Key = "KIPSToILGp6fl+3gXJvMsN4IajizYBBT";
private readonly string Key = string.Empty;
public CryptoHelper()
{
IV = ConfigurationManager.AppSettings["IV"];
Key = ConfigurationManager.AppSettings["Key"];
}
///
/// 获取加密后的字符串
///
/// 输入值.
///
public string GetEncryptedValue(string inputValue)
{
TripleDESCryptoServiceProvider provider = this.GetCryptoProvider();
// 创建内存流来保存加密后的流
MemoryStream mStream = new MemoryStream();
// 创建加密转换流
CryptoStream cStream = new CryptoStream(mStream,
provider.CreateEncryptor(), CryptoStreamMode.Write);
// 使用UTF8编码获取输入字符串的字节。
byte[] toEncrypt = new UTF8Encoding().GetBytes(inputValue);
// 将字节写到转换流里面去。
cStream.Write(toEncrypt, 0, toEncrypt.Length);
cStream.FlushFinalBlock();
// 在调用转换流的FlushFinalBlock方法后,内部就会进行转换了,此时mStream就是加密后的流了。
byte[] ret = mStream.ToArray();
// Close the streams.
cStream.Close();
mStream.Close();
//将加密后的字节进行64编码。
return Convert.ToBase64String(ret);
}
///
/// 获取加密服务类
///
///
private TripleDESCryptoServiceProvider GetCryptoProvider()
{
TripleDESCryptoServiceProvider provider = new TripleDESCryptoServiceProvider();
provider.IV = Convert.FromBase64String(IV);
provider.Key = Convert.FromBase64String(Key);
return provider;
}
///
/// 获取解密后的值
///
/// 经过加密后的字符串.
///
public string GetDecryptedValue(string inputValue)
{
TripleDESCryptoServiceProvider provider = this.GetCryptoProvider();
byte[] inputEquivalent = Convert.FromBase64String(inputValue);
// 创建内存流保存解密后的数据
MemoryStream msDecrypt = new MemoryStream();
// 创建转换流。
CryptoStream csDecrypt = new CryptoStream(msDecrypt,
provider.CreateDecryptor(),
CryptoStreamMode.Write);
csDecrypt.Write(inputEquivalent, 0, inputEquivalent.Length);
csDecrypt.FlushFinalBlock();
csDecrypt.Close();
//获取字符串。
return new UTF8Encoding().GetString(msDecrypt.ToArray());
}
}
}
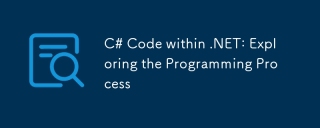
Proses pengaturcaraan C# dalam .NET termasuk langkah -langkah berikut: 1) Menulis C# Code, 2) Menyusun bahasa pertengahan (IL), dan 3) yang dilaksanakan oleh Runtime .NET (CLR). Kelebihan C# dalam .NET adalah sintaks moden, sistem jenis yang kuat dan integrasi yang ketat dengan Rangka Kerja .NET, sesuai untuk pelbagai senario pembangunan dari aplikasi desktop ke perkhidmatan web.
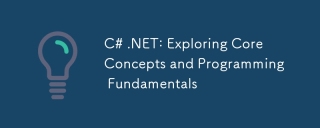
C# adalah bahasa pengaturcaraan yang berorientasikan objek moden yang dibangunkan oleh Microsoft dan sebagai sebahagian daripada Rangka Kerja .NET. 1.C# menyokong pengaturcaraan berorientasikan objek (OOP), termasuk enkapsulasi, warisan dan polimorfisme. 2. Pengaturcaraan Asynchronous dalam C# dilaksanakan melalui Async dan menunggu kata kunci untuk meningkatkan respons aplikasi. 3. Gunakan LINQ untuk memproses koleksi data dengan ringkas. 4. Kesilapan umum termasuk pengecualian rujukan null dan pengecualian indeks luar. Kemahiran penyahpepijatan termasuk menggunakan debugger dan pengendalian pengecualian. 5. Pengoptimuman Prestasi termasuk menggunakan StringBuilder dan mengelakkan pembungkusan yang tidak perlu dan unboxing.
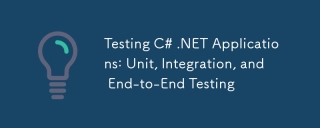
Strategi ujian untuk aplikasi C#. NET termasuk ujian unit, ujian integrasi, dan ujian akhir-ke-akhir. 1. Ujian unit memastikan bahawa unit minimum kod berfungsi secara bebas, menggunakan rangka kerja MSTest, Nunit atau Xunit. 2. Ujian Bersepadu Mengesahkan fungsi pelbagai unit yang digabungkan, data simulasi yang biasa digunakan dan perkhidmatan luaran. 3. Ujian akhir-ke-akhir mensimulasikan proses operasi lengkap pengguna, dan selenium biasanya digunakan untuk ujian automatik.
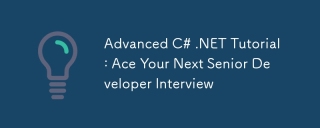
Temu bual dengan pemaju kanan C# memerlukan menguasai pengetahuan teras seperti pengaturcaraan asynchronous, LINQ, dan prinsip kerja dalaman Rangka .NET. 1. Pengaturcaraan Asynchronous memudahkan operasi melalui async dan menunggu untuk meningkatkan respons aplikasi. 2.Linq mengendalikan data dalam gaya SQL dan perhatikan prestasi. 3. CLR kerangka bersih menguruskan ingatan, dan pengumpulan sampah perlu digunakan dengan berhati -hati.
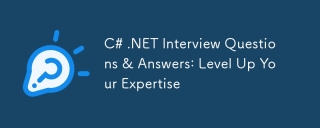
C#.NET Soalan dan jawapan wawancara termasuk pengetahuan asas, konsep teras, dan penggunaan lanjutan. 1) Pengetahuan asas: C# adalah bahasa berorientasikan objek yang dibangunkan oleh Microsoft dan digunakan terutamanya dalam rangka .NET. 2) Konsep teras: Delegasi dan peristiwa membolehkan kaedah mengikat dinamik, dan LINQ menyediakan fungsi pertanyaan yang kuat. 3) Penggunaan Lanjutan: Pengaturcaraan Asynchronous meningkatkan respons, dan pokok ekspresi digunakan untuk pembinaan kod dinamik.
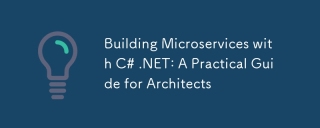
C#.NET adalah pilihan yang popular untuk membina microservices kerana ekosistem yang kuat dan sokongan yang kaya. 1) Buat RestfulAPi menggunakan ASP.Netcore untuk memproses penciptaan pesanan dan pertanyaan. 2) Gunakan GRPC untuk mencapai komunikasi yang cekap antara microservices, menentukan dan melaksanakan perkhidmatan pesanan. 3) Memudahkan penggunaan dan pengurusan melalui microservices kontena Docker.
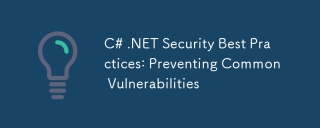
Amalan terbaik keselamatan untuk C# dan .NET termasuk pengesahan input, pengekodan output, pengendalian pengecualian, serta pengesahan dan kebenaran. 1) Gunakan ungkapan biasa atau kaedah terbina dalam untuk mengesahkan input untuk mengelakkan data berniat jahat memasuki sistem. 2) Pengekodan output Untuk mencegah serangan XSS, gunakan kaedah httputility.htmlencode. 3) Pengendalian Pengecualian Menghindari kebocoran maklumat, ralat rekod tetapi tidak mengembalikan maklumat terperinci kepada pengguna. 4) Gunakan Asp.Netidentity dan kebenaran berasaskan tuntutan untuk melindungi aplikasi daripada akses yang tidak dibenarkan.
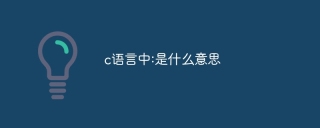
Makna kolon (':') dalam bahasa C: Penyataan bersyarat: Memisahkan ekspresi bersyarat dan pernyataan blok pernyataan pernyataan: Memisahkan permulaan, bersyarat dan tambahan ekspresi makro Definisi: Memisahkan nama makro dan nilai makro Single Line Comment: Mewakili kandungan dari kolon hingga akhir garis sebagai dimensi array komen: Tentukan dimensi array


Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

AI Hentai Generator
Menjana ai hentai secara percuma.

Artikel Panas

Alat panas
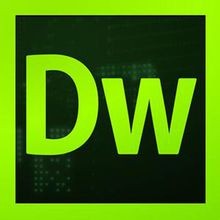
Dreamweaver CS6
Alat pembangunan web visual

SecLists
SecLists ialah rakan penguji keselamatan muktamad. Ia ialah koleksi pelbagai jenis senarai yang kerap digunakan semasa penilaian keselamatan, semuanya di satu tempat. SecLists membantu menjadikan ujian keselamatan lebih cekap dan produktif dengan menyediakan semua senarai yang mungkin diperlukan oleh penguji keselamatan dengan mudah. Jenis senarai termasuk nama pengguna, kata laluan, URL, muatan kabur, corak data sensitif, cangkerang web dan banyak lagi. Penguji hanya boleh menarik repositori ini ke mesin ujian baharu dan dia akan mempunyai akses kepada setiap jenis senarai yang dia perlukan.

PhpStorm versi Mac
Alat pembangunan bersepadu PHP profesional terkini (2018.2.1).
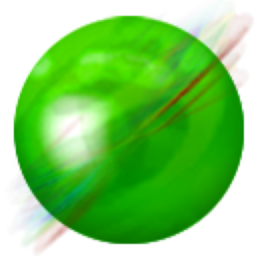
ZendStudio 13.5.1 Mac
Persekitaran pembangunan bersepadu PHP yang berkuasa

SublimeText3 Linux versi baharu
SublimeText3 Linux versi terkini