


2696. Minimum String Length After Removing Substrings
Difficulty: Easy
Topics: String, Stack, Simulation
You are given a string s consisting only of uppercase English letters.
You can apply some operations to this string where, in one operation, you can remove any occurrence of one of the substrings "AB" or "CD" from s.
Return the minimum possible length of the resulting string that you can obtain.
Note that the string concatenates after removing the substring and could produce new "AB" or "CD" substrings.
Example 1:
- Input: s = "ABFCACDB"
- Output: 2
-
Explanation: We can do the following operations:
- Remove the substring "ABFCACDB", so s = "FCACDB".
- Remove the substring "FCACDB", so s = "FCAB".
- Remove the substring "FCAB", so s = "FC".
- So the resulting length of the string is 2.
- It can be shown that it is the minimum length that we can obtain.
Example 2:
- Input: s = "ACBBD"
- Output: 5
- Explanation: We cannot do any operations on the string so the length remains the same.
Constraints:
- 1
- s consists only of uppercase English letters.
Hint:
- Can we use brute force to solve the problem?
- Repeatedly traverse the string to find and remove the substrings “AB” and “CD” until no more occurrences exist.
Solution:
We'll use a stack to handle the removal of substrings "AB" and "CD". The stack approach ensures that we efficiently remove these substrings as they occur during traversal of the string.
Approach:
-
Use a Stack:
- Traverse the string character by character.
- Push each character onto the stack.
- If the top two characters on the stack form the substring "AB" or "CD", pop these two characters from the stack (remove them).
- Continue this process for all characters in the input string.
-
Final String:
- At the end of the traversal, the stack will contain the reduced string.
- The minimum possible length will be the size of the stack.
Let's implement this solution in PHP: 2696. Minimum String Length After Removing Substrings
<?php <br> /**
- @param String $s
- @return Integer /
- go to ./solution.php */
// Example usage:
echo minLengthAfterRemovals("ABFCACDB"); // Output: 2
echo "\n";
echo minLengthAfterRemovals("ACBBD"); // Output: 5
?>
Explanation:
- We initialize an empty stack ($stack).
- Loop through each character of the string s.
- Check the top character of the stack:
- If the top character and the current character form the substrings "AB" or "CD", we remove the top character using array_pop.
- Otherwise, push the current character onto the stack.
- The stack will hold the characters that remain after all possible removals.
- Finally, count($stack) gives the length of the resulting string.
Complexity:
- Time Complexity: O(n), where n is the length of the string. Each character is processed at most twice (once pushed, once popped).
- Space Complexity: O(n) for the stack, in the worst case where no removals are possible.
This solution effectively minimizes the string by removing all possible occurrences of "AB" and "CD" until no more can be found.
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
Atas ialah kandungan terperinci Panjang Rentetan Minimum Selepas Mengeluarkan Substring. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!
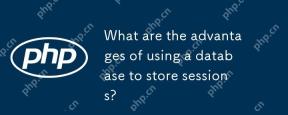
Kelebihan utama menggunakan sesi penyimpanan pangkalan data termasuk kegigihan, skalabilitas, dan keselamatan. 1. Kegigihan: Walaupun pelayan dimulakan semula, data sesi tidak dapat berubah. 2. Skalabiliti: Berkenaan dengan sistem yang diedarkan, memastikan data sesi disegerakkan di antara pelbagai pelayan. 3. Keselamatan: Pangkalan data menyediakan storan yang disulitkan untuk melindungi maklumat sensitif.
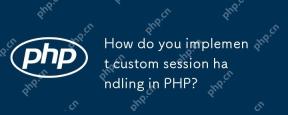
Melaksanakan pemprosesan sesi tersuai dalam PHP boleh dilakukan dengan melaksanakan antara muka sessionHandlerInterface. Langkah -langkah khusus termasuk: 1) mewujudkan kelas yang melaksanakan sessionHandlerInterface, seperti CustomSessionHandler; 2) kaedah penulisan semula dalam antara muka (seperti terbuka, rapat, membaca, menulis, memusnahkan, gc) untuk menentukan kitaran hayat dan kaedah penyimpanan data sesi; 3) Daftar pemproses sesi tersuai dalam skrip PHP dan mulakan sesi. Ini membolehkan data disimpan dalam media seperti MySQL dan REDIS untuk meningkatkan prestasi, keselamatan dan skalabiliti.
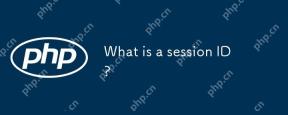
SesionID adalah mekanisme yang digunakan dalam aplikasi web untuk mengesan status sesi pengguna. 1. Ia adalah rentetan yang dijana secara rawak yang digunakan untuk mengekalkan maklumat identiti pengguna semasa pelbagai interaksi antara pengguna dan pelayan. 2. Pelayan menjana dan menghantarnya kepada klien melalui kuki atau parameter URL untuk membantu mengenal pasti dan mengaitkan permintaan ini dalam pelbagai permintaan pengguna. 3. Generasi biasanya menggunakan algoritma rawak untuk memastikan keunikan dan ketidakpastian. 4. Dalam pembangunan sebenar, pangkalan data dalam memori seperti REDIS boleh digunakan untuk menyimpan data sesi untuk meningkatkan prestasi dan keselamatan.
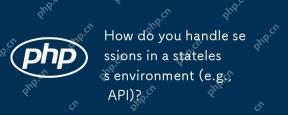
Menguruskan sesi dalam persekitaran tanpa kerakyatan seperti API boleh dicapai dengan menggunakan JWT atau cookies. 1. JWT sesuai untuk ketiadaan dan skalabilitas, tetapi ia adalah saiz yang besar ketika datang ke data besar. 2.Cookies lebih tradisional dan mudah dilaksanakan, tetapi mereka perlu dikonfigurasikan dengan berhati -hati untuk memastikan keselamatan.
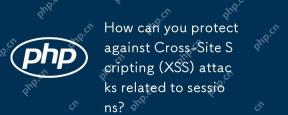
Untuk melindungi permohonan dari serangan XSS yang berkaitan dengan sesi, langkah-langkah berikut diperlukan: 1. Tetapkan bendera httponly dan selamat untuk melindungi kuki sesi. 2. Kod eksport untuk semua input pengguna. 3. Melaksanakan Dasar Keselamatan Kandungan (CSP) untuk mengehadkan sumber skrip. Melalui dasar-dasar ini, serangan XSS yang berkaitan dengan sesi dapat dilindungi dengan berkesan dan data pengguna dapat dipastikan.
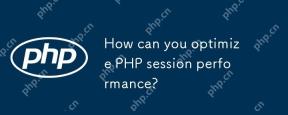
Kaedah untuk mengoptimumkan prestasi sesi PHP termasuk: 1. Mula sesi kelewatan, 2. Gunakan pangkalan data untuk menyimpan sesi, 3. Data sesi kompres, 4. Mengurus kitaran hayat sesi, dan 5. Melaksanakan perkongsian sesi. Strategi ini dapat meningkatkan kecekapan aplikasi dalam persekitaran konkurensi yang tinggi.
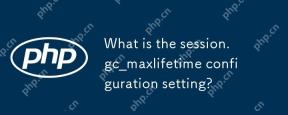
Thesession.gc_maxlifetimesettinginphpdeterminesthelifespanofsessiondata, setInseconds.1) it'sconfiguredinphp.iniorviaini_set (). 2) abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3) php'sgarbageCollectionisprobabilistic, influedbygc_probabi

Dalam PHP, anda boleh menggunakan fungsi session_name () untuk mengkonfigurasi nama sesi. Langkah -langkah tertentu adalah seperti berikut: 1. Gunakan fungsi session_name () untuk menetapkan nama sesi, seperti session_name ("my_session"). 2. Selepas menetapkan nama sesi, hubungi session_start () untuk memulakan sesi. Mengkonfigurasi nama sesi boleh mengelakkan konflik data sesi antara pelbagai aplikasi dan meningkatkan keselamatan, tetapi memberi perhatian kepada keunikan, keselamatan, panjang dan penetapan masa sesi.


Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

MantisBT
Mantis ialah alat pengesan kecacatan berasaskan web yang mudah digunakan yang direka untuk membantu dalam pengesanan kecacatan produk. Ia memerlukan PHP, MySQL dan pelayan web. Lihat perkhidmatan demo dan pengehosan kami.
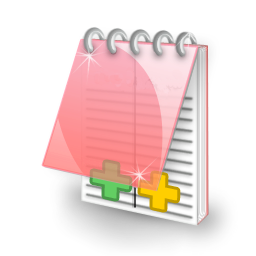
EditPlus versi Cina retak
Saiz kecil, penyerlahan sintaks, tidak menyokong fungsi gesaan kod
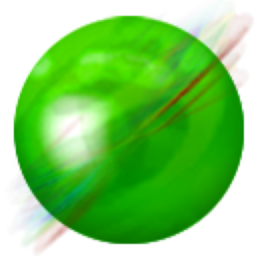
ZendStudio 13.5.1 Mac
Persekitaran pembangunan bersepadu PHP yang berkuasa

Pelayar Peperiksaan Selamat
Pelayar Peperiksaan Selamat ialah persekitaran pelayar selamat untuk mengambil peperiksaan dalam talian dengan selamat. Perisian ini menukar mana-mana komputer menjadi stesen kerja yang selamat. Ia mengawal akses kepada mana-mana utiliti dan menghalang pelajar daripada menggunakan sumber yang tidak dibenarkan.
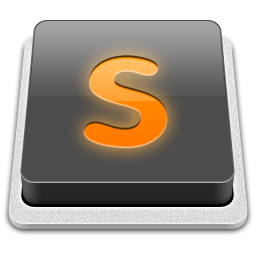
SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)