종속성 주입은 구성 요소 내에서 종속성을 하드 코딩하여 구성 요소에 제공하는 방식으로 대체하는 소프트웨어 설계 패턴입니다. 이를 통해 하나의 구성 요소가 종속성을 찾는 것부터 종속성 구성까지 완화됩니다. 이는 구성 요소를 재사용, 유지 관리 및 테스트 가능하게 만드는 데 도움이 됩니다.
AngularJS는 최고의 종속성 주입 메커니즘을 제공합니다. 서로 종속성을 주입할 수 있는 다음과 같은 핵심 구성 요소를 제공합니다.
- 가치
- 공장
- 서비스
- 제공자
- 상수값
가치
값은 구성 단계 컨트롤러 중에 값을 전달하는 데 사용되는 간단한 JavaScript 개체입니다.
//define a module var mainApp = angular.module("mainApp", []); //create a value object as "defaultInput" and pass it a data. mainApp.value("defaultInput", 5); ... //inject the value in the controller using its name "defaultInput" mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } });
공장
팩토리는 함수의 값을 반환하는 데 사용됩니다. 서비스나 컨트롤러가 필요할 때마다 수요에 따라 가치를 창출합니다. 일반적으로 팩토리 함수를 사용하여 해당 값을 계산하고 반환합니다
//define a module var mainApp = angular.module("mainApp", []); //create a factory "MathService" which provides a method multiply to return multiplication of two numbers mainApp.factory('MathService', function() { var factory = {}; factory.multiply = function(a, b) { return a * b } return factory; }); //inject the factory "MathService" in a service to utilize the multiply method of factory. mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); ...
서비스
서비스는 특정 작업을 수행하는 기능 집합이 포함된 단일 JavaScript 개체입니다. 서비스는 service() 함수를 사용하여 정의된 다음 컨트롤러에 주입됩니다.
//define a module var mainApp = angular.module("mainApp", []); ... //create a service which defines a method square to return square of a number. mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); //inject the service "CalcService" into the controller mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } });
공급자
AngularJS 내부 생성 프로세스의 구성 단계에서 사용되는 공급자 서비스, 팩토리 등(AngularJS가 자체적으로 부트스트랩하는 경우에 해당) 아래에 언급된 스크립트를 사용하여 이전에 이미 만든 MathService를 만들 수 있습니다. 공급자는 값/서비스/공장을 반환하는 특수 팩토리 메서드 및 get() 메서드입니다.
//define a module var mainApp = angular.module("mainApp", []); ... //create a service using provider which defines a method square to return square of a number. mainApp.config(function($provide) { $provide.provider('MathService', function() { this.$get = function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }; }); });
상시
상수는 구성 단계에서 값을 전달할 수 없고, 구성 단계에서 값을 전달할 수 없다는 사실을 설명하기 위해 사용됩니다.
mainApp.constant("configParam", "constant value");
예
다음 예에서는 위의 모든 명령을 보여줍니다.
testAngularJS.html
<html> <head> <title>AngularJS Dependency Injection</title> </head> <body> <h2 id="AngularJS-Sample-Application">AngularJS Sample Application</h2> <div ng-app="mainApp" ng-controller="CalcController"> <p>Enter a number: <input type="number" ng-model="number" /> <button ng-click="square()">X<sup>2</sup></button> <p>Result: {{result}}</p> </div> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> <script> var mainApp = angular.module("mainApp", []); mainApp.config(function($provide) { $provide.provider('MathService', function() { this.$get = function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }; }); }); mainApp.value("defaultInput", 5); mainApp.factory('MathService', function() { var factory = {}; factory.multiply = function(a, b) { return a * b; } return factory; }); mainApp.service('CalcService', function(MathService){ this.square = function(a) { return MathService.multiply(a,a); } }); mainApp.controller('CalcController', function($scope, CalcService, defaultInput) { $scope.number = defaultInput; $scope.result = CalcService.square($scope.number); $scope.square = function() { $scope.result = CalcService.square($scope.number); } }); </script> </body> </html>
결과
웹 브라우저에서 textAngularJS.html을 엽니다. 아래 결과를 참조하세요.
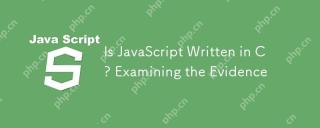
예, JavaScript의 엔진 코어는 C로 작성되었습니다. 1) C 언어는 효율적인 성능과 기본 제어를 제공하며, 이는 JavaScript 엔진 개발에 적합합니다. 2) V8 엔진을 예를 들어, 핵심은 C로 작성되며 C의 효율성 및 객체 지향적 특성을 결합하여 C로 작성됩니다.
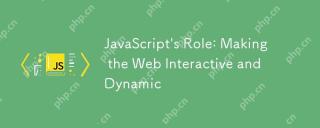
JavaScript는 웹 페이지의 상호 작용과 역학을 향상시키기 때문에 현대 웹 사이트의 핵심입니다. 1) 페이지를 새로 고치지 않고 콘텐츠를 변경할 수 있습니다. 2) Domapi를 통해 웹 페이지 조작, 3) 애니메이션 및 드래그 앤 드롭과 같은 복잡한 대화식 효과를 지원합니다. 4) 성능 및 모범 사례를 최적화하여 사용자 경험을 향상시킵니다.
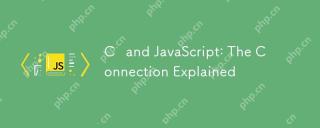
C 및 JavaScript는 WebAssembly를 통한 상호 운용성을 달성합니다. 1) C 코드는 WebAssembly 모듈로 컴파일되어 컴퓨팅 전력을 향상시키기 위해 JavaScript 환경에 도입됩니다. 2) 게임 개발에서 C는 물리 엔진 및 그래픽 렌더링을 처리하며 JavaScript는 게임 로직 및 사용자 인터페이스를 담당합니다.
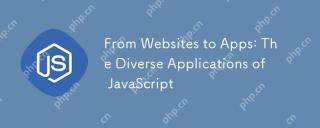
JavaScript는 웹 사이트, 모바일 응용 프로그램, 데스크탑 응용 프로그램 및 서버 측 프로그래밍에서 널리 사용됩니다. 1) 웹 사이트 개발에서 JavaScript는 HTML 및 CSS와 함께 DOM을 운영하여 동적 효과를 달성하고 jQuery 및 React와 같은 프레임 워크를 지원합니다. 2) 반응 및 이온 성을 통해 JavaScript는 크로스 플랫폼 모바일 애플리케이션을 개발하는 데 사용됩니다. 3) 전자 프레임 워크를 사용하면 JavaScript가 데스크탑 애플리케이션을 구축 할 수 있습니다. 4) node.js는 JavaScript가 서버 측에서 실행되도록하고 동시 요청이 높은 높은 요청을 지원합니다.
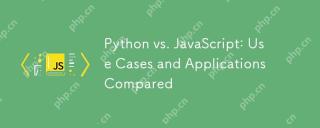
Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
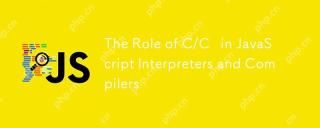
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
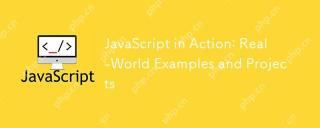
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
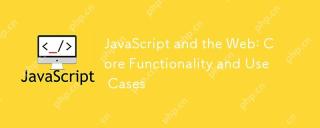
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
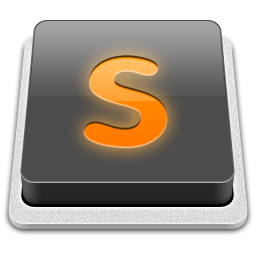
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
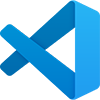
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
