Spring Boot 및 MyBatis 구성 실습 가이드
소개:
Spring Boot는 Spring 애플리케이션의 시작 및 배포 프로세스를 단순화하는 데 사용되는 신속한 개발 프레임워크입니다. 그리고 MyBatis는 다양한 관계형 데이터베이스와 쉽게 상호 작용할 수 있는 인기 있는 지속성 프레임워크입니다. 이 기사에서는 Spring Boot 프로젝트에서 MyBatis를 구성하고 사용하는 방법을 소개하고 구체적인 코드 예제를 제공합니다.
1. 프로젝트 구성
1. 종속성 소개
pom.xml 파일에 다음 종속성을 추가합니다.
<dependencies> <!-- Spring Boot Web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- MyBatis --> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> </dependency> <!-- 数据库驱动(例如,MySQL)--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> </dependencies>
2. 데이터베이스 연결 구성
application.properties
파일에서 데이터베이스 연결 정보. 예를 들어, MySQL 데이터베이스를 사용하는 경우 다음 구성을 추가할 수 있습니다. application.properties
文件中,配置数据库连接信息。例如,如果使用MySQL数据库,可以添加以下配置:
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase spring.datasource.username=root spring.datasource.password=123456 spring.datasource.driver-class-name=com.mysql.jdbc.Driver
二、创建实体类
1.创建实体类
在 com.example.demo.entity
包中,创建一个名为 User
的实体类:
public class User { private int id; private String name; private String email; // 省略 getters 和 setters }
2.创建Mapper接口
在 com.example.demo.mapper
包中,创建一个名为 UserMapper
的接口:
public interface UserMapper { List<User> getAllUsers(); User getUserById(int id); void addUser(User user); void updateUser(User user); void deleteUser(int id); }
三、创建Mapper XML文件
创建 UserMapper
对应的Mapper XML文件 UserMapper.xml
,并配置相应的SQL操作:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.demo.mapper.UserMapper"> <resultMap id="BaseResultMap" type="com.example.demo.entity.User"> <id column="id" property="id"/> <result column="name" property="name"/> <result column="email" property="email"/> </resultMap> <select id="getAllUsers" resultMap="BaseResultMap"> SELECT * FROM user </select> <select id="getUserById" resultMap="BaseResultMap"> SELECT * FROM user WHERE id=#{id} </select> <insert id="addUser"> INSERT INTO user(name, email) VALUES (#{name}, #{email}) </insert> <update id="updateUser"> UPDATE user SET name=#{name}, email=#{email} WHERE id=#{id} </update> <delete id="deleteUser"> DELETE FROM user WHERE id=#{id} </delete> </mapper>
四、配置MyBatis
1.创建配置类
在 com.example.demo.config
包中,创建一个名为 MyBatisConfig
的配置类:
@Configuration @MapperScan("com.example.demo.mapper") public class MyBatisConfig { }
2.完成配置
在 application.properties
文件中,添加以下配置:
# MyBatis mybatis.mapper-locations=classpath*:com/example/demo/mapper/*.xml
至此,我们已经完成了项目的配置和准备工作。接下来,我们将了解如何在Spring Boot项目中使用MyBatis。
五、使用MyBatis
1.编写业务逻辑
在 com.example.demo.service
包中,创建名为 UserService
的服务类:
@Service public class UserService { @Autowired private UserMapper userMapper; public List<User> getAllUsers() { return userMapper.getAllUsers(); } public User getUserById(int id) { return userMapper.getUserById(id); } public void addUser(User user) { userMapper.addUser(user); } public void updateUser(User user) { userMapper.updateUser(user); } public void deleteUser(int id) { userMapper.deleteUser(id); } }
2.创建控制器
在 com.example.demo.controller
包中,创建名为 UserController
@RestController @RequestMapping("/users") public class UserController { @Autowired private UserService userService; @GetMapping("") public List<User> getAllUsers() { return userService.getAllUsers(); } @GetMapping("/{id}") public User getUserById(@PathVariable int id) { return userService.getUserById(id); } @PostMapping("") public void addUser(@RequestBody User user) { userService.addUser(user); } @PutMapping("/{id}") public void updateUser(@PathVariable int id, @RequestBody User user) { user.setId(id); userService.updateUser(user); } @DeleteMapping("/{id}") public void deleteUser(@PathVariable int id) { userService.deleteUser(id); } }2. 엔터티 클래스를 생성합니다.
1. com.example.demo.entity
에서 엔터티 클래스를 생성합니다. 패키지에서 사용자라는 파일을 생성합니다.
rrreee
- rrreee 3. 매퍼 XML 파일 생성
- 해당
UserMapper
매퍼 XML 파일UserMapper.xml
을 생성하고 해당 SQL 작업을 구성합니다. rrreee - 4. MyBatis 구성 1 구성 클래스를 생성합니다
-
com.example.demo.config
패키지에서MyBatisConfig
라는 구성 클래스를 생성합니다. rrreee - 2. 구성 완료
com.example.demo.mapper
패키지에서 UserMapper 인터페이스: application.properties
파일에 다음 구성을 추가합니다. 이제 프로젝트 구성 및 준비가 완료되었습니다. 다음으로 Spring Boot 프로젝트에서 MyBatis를 사용하는 방법을 알아봅니다.
com.example.demo.service
패키지에서 UserService
라는 서비스 클래스를 만듭니다. 🎜rrreee🎜2 . 컨트롤러 만들기🎜 com.example.demo.controller
패키지에서 UserController
라는 컨트롤러 클래스를 만듭니다. 🎜rrreee🎜3 API 테스트🎜Spring Boot 애플리케이션을 시작합니다. API를 테스트하려면 브라우저에서 다음 URL을 방문하십시오. 🎜🎜🎜 모든 사용자 가져오기: http://localhost:8080/users 🎜🎜 단일 사용자 가져오기: http://localhost:8080/users/{id}🎜 🎜 사용자 추가: POST http://localhost:8080/users, 요청 본문은 JSON 형식의 사용자 개체입니다. 🎜🎜사용자 업데이트: PUT http://localhost:8080/users/{id}, 요청 본문은 사용자입니다. JSON 형식의 Object 🎜🎜사용자 삭제: DELETE http://localhost:8080/users/{id}🎜🎜🎜요약: 🎜이 글에서는 Spring Boot 프로젝트에서 MyBatis를 사용하는 구성 실습 방법을 소개하고 구체적인 코드 예제를 제공합니다. 이 기사가 독자들이 Spring Boot와 MyBatis의 조합을 빠르게 이해하고 사용하여 Spring 애플리케이션을 보다 효율적으로 개발하는 데 도움이 되기를 바랍니다. 🎜위 내용은 스프링 부트와 MyBatis 구성 팁 가이드의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
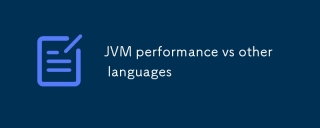
JVM 'sperformanceIscompetitive, ontotherRuntimes, 안전 및 생산성을 제공합니다

javaachievesplatformincendenceThermeThoughthejavavirtualMachine (JVM), codeiscompiledintobytecode, notmachine-specificcode.2) bytecodeistredbythejvm, anblingcross- shoughtshoughts
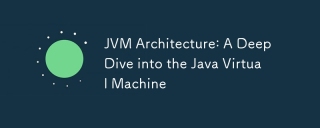
thejvmisanabstractcomputingmachinecrucialforrunningjavaprogramsduetoitsplatform-independentarchitection.itincludes : 1) classloaderforloadingclasses, 2) runtimeDataAreaFordatorage, 3) executionEnginewithgringreter, jitcompiler 및 ggarocubucbugecutec
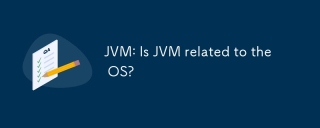
Theosasittranslatesjavabytecodeintomachine-specificinstructions, ManagesMemory 및 HandlesgarbageCollection의 Jvmhasacloserelationship
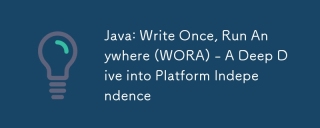
Java 구현 "Write Once, Run Everywhere"는 바이트 코드로 컴파일되어 JVM (Java Virtual Machine)에서 실행됩니다. 1) Java 코드를 작성하여 바이트 코드로 컴파일하십시오. 2) 바이트 코드는 JVM이 설치된 모든 플랫폼에서 실행됩니다. 3) JNI (Java Native Interface)를 사용하여 플랫폼 별 기능을 처리하십시오. JVM 일관성 및 플랫폼 별 라이브러리 사용과 같은 과제에도 불구하고 Wora는 개발 효율성 및 배포 유연성을 크게 향상시킵니다.
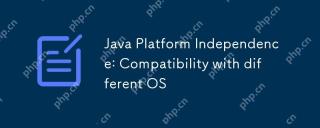
javaachievesplatformincendenceThoughthehoughthejavavirtualmachine (JVM), hittoutModification.thejvmcompileSjavacodeIntOplatform-independentByTecode, whatitTengretsAndexeSontheSpecoS, toplacetSonthecificos, toacketSecificos
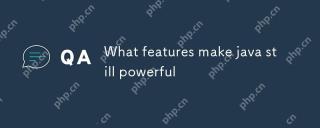
javaispowerfuldueToitsplatformincendence, 객체 지향적, RichandardLibrary, PerformanceCapabilities 및 StrongSecurityFeatures.1) Platform IndependenceAllowsApplicationStorunannyDevicesUpportingjava.2) 대상 지향적 프로그래밍 프로모션 Modulara
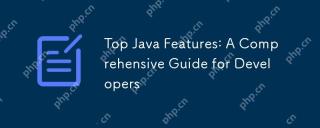
최고 Java 기능에는 다음이 포함됩니다. 1) 객체 지향 프로그래밍, 다형성 지원, 코드 유연성 및 유지 관리 가능성 향상; 2) 예외 처리 메커니즘, 시도 캐치-패치 블록을 통한 코드 견고성 향상; 3) 쓰레기 수집, 메모리 관리 단순화; 4) 제네릭, 유형 안전 강화; 5) 코드를보다 간결하고 표현력있게 만들기위한 AMBDA 표현 및 기능 프로그래밍; 6) 최적화 된 데이터 구조 및 알고리즘을 제공하는 풍부한 표준 라이브러리.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는