PHP 연구 노트: 데이터 구조 및 알고리즘
개요:
데이터 구조와 알고리즘은 컴퓨터 과학에서 매우 중요한 두 가지 개념입니다. 문제를 해결하고 코드 성능을 최적화하는 데 핵심입니다. PHP 프로그래밍에서는 데이터를 저장하고 조작하기 위해 다양한 데이터 구조를 사용해야 하는 경우가 많고, 다양한 기능을 구현하기 위해 알고리즘을 사용해야 하는 경우도 있습니다. 이 기사에서는 일반적으로 사용되는 데이터 구조와 알고리즘을 소개하고 해당 PHP 코드 예제를 제공합니다.
1. 선형 구조
- Array
배열은 가장 일반적으로 사용되는 데이터 구조 중 하나이며 정렬된 데이터 세트를 저장하는 데 사용할 수 있습니다. PHP의 배열은 순서가 지정된 맵(키-값)의 모음이며 아래 첨자를 사용하여 배열의 요소에 액세스할 수 있습니다. 다음은 몇 가지 일반적인 배열 작업입니다.
- 배열 만들기: $arr = array(1, 2, 3);
- 요소 추가: $arr[] = 4
- 액세스 요소: $arr[0] ;
- 요소 삭제: unset($arr[0]);
- 배열 길이: count($arr);
- 루프 순회: foreach ($arr as $value) { ... }
- 링크된 목록 목록)
연결된 목록은 일련의 노드로 구성된 데이터 구조이며, 각 노드에는 데이터와 다음 노드에 대한 포인터가 포함됩니다. 연결된 목록은 효율적인 삽입 및 삭제 작업을 구현할 수 있지만 검색 작업은 느립니다. 다음은 연결된 목록의 간단한 예입니다.
class Node { public $data; public $next; public function __construct($data = null) { $this->data = $data; $this->next = null; } } class LinkedList { public $head; public function __construct() { $this->head = null; } public function insert($data) { $newNode = new Node($data); if ($this->head === null) { $this->head = $newNode; } else { $currentNode = $this->head; while ($currentNode->next !== null) { $currentNode = $currentNode->next; } $currentNode->next = $newNode; } } public function display() { $currentNode = $this->head; while ($currentNode !== null) { echo $currentNode->data . " "; $currentNode = $currentNode->next; } } } $linkedList = new LinkedList(); $linkedList->insert(1); $linkedList->insert(2); $linkedList->insert(3); $linkedList->display();
2. 비선형 구조
- Stack(스택) 스택은 배열을 사용하여 구현할 수 있는 LIFO(후입선출) 데이터 구조입니다. 또는 연결리스트. 다음은 간단한 스택 예입니다.
class Stack { private $arr; public function __construct() { $this->arr = array(); } public function push($data) { array_push($this->arr, $data); } public function pop() { if (!$this->isEmpty()) { return array_pop($this->arr); } } public function isEmpty() { return empty($this->arr); } } $stack = new Stack(); $stack->push(1); $stack->push(2); $stack->push(3); echo $stack->pop(); // 输出 3
- Queue
- Queue는 배열 또는 연결 목록을 사용하여 구현할 수 있는 FIFO(선입선출) 데이터 구조입니다. 다음은 간단한 대기열의 예입니다.
class Queue { private $arr; public function __construct() { $this->arr = array(); } public function enqueue($data) { array_push($this->arr, $data); } public function dequeue() { if (!$this->isEmpty()) { return array_shift($this->arr); } } public function isEmpty() { return empty($this->arr); } } $queue = new Queue(); $queue->enqueue(1); $queue->enqueue(2); $queue->enqueue(3); echo $queue->dequeue(); // 输出 1
- 정렬 알고리즘
- 버블 정렬
- 선택 정렬
- 삽입 정렬
- 빠른 정렬
- 병합 정렬
- 검색 알고리즘
- 바이너리 검색
- Recursive Algorithm
- Factorial
- Fibonacci Sequence
위 내용은 PHP 연구 노트: 데이터 구조 및 알고리즘의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
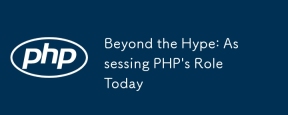
PHP는 현대적인 프로그래밍, 특히 웹 개발 분야에서 강력하고 널리 사용되는 도구로 남아 있습니다. 1) PHP는 사용하기 쉽고 데이터베이스와 완벽하게 통합되며 많은 개발자에게 가장 먼저 선택됩니다. 2) 동적 컨텐츠 생성 및 객체 지향 프로그래밍을 지원하여 웹 사이트를 신속하게 작성하고 유지 관리하는 데 적합합니다. 3) 데이터베이스 쿼리를 캐싱하고 최적화함으로써 PHP의 성능을 향상시킬 수 있으며, 광범위한 커뮤니티와 풍부한 생태계는 오늘날의 기술 스택에 여전히 중요합니다.
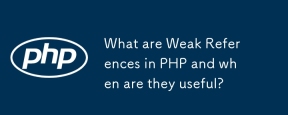
PHP에서는 약한 참조가 약한 회의 클래스를 통해 구현되며 쓰레기 수집가가 물체를 되 찾는 것을 방해하지 않습니다. 약한 참조는 캐싱 시스템 및 이벤트 리스너와 같은 시나리오에 적합합니다. 물체의 생존을 보장 할 수 없으며 쓰레기 수집이 지연 될 수 있음에 주목해야합니다.
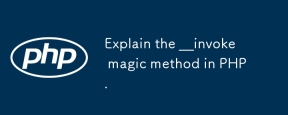
\ _ \ _ 호출 메소드를 사용하면 객체를 함수처럼 호출 할 수 있습니다. 1. 객체를 호출 할 수 있도록 메소드를 호출하는 \ _ \ _ 정의하십시오. 2. $ obj (...) 구문을 사용할 때 PHP는 \ _ \ _ invoke 메소드를 실행합니다. 3. 로깅 및 계산기, 코드 유연성 및 가독성 향상과 같은 시나리오에 적합합니다.
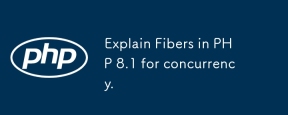
섬유는 PHP8.1에 도입되어 동시 처리 기능을 향상시켰다. 1) 섬유는 코 루틴과 유사한 가벼운 동시성 모델입니다. 2) 개발자는 작업의 실행 흐름을 수동으로 제어 할 수 있으며 I/O 집약적 작업을 처리하는 데 적합합니다. 3) 섬유를 사용하면보다 효율적이고 반응이 좋은 코드를 작성할 수 있습니다.
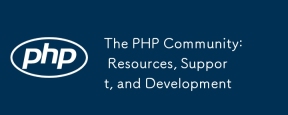
PHP 커뮤니티는 개발자 성장을 돕기 위해 풍부한 자원과 지원을 제공합니다. 1) 자료에는 공식 문서, 튜토리얼, 블로그 및 Laravel 및 Symfony와 같은 오픈 소스 프로젝트가 포함됩니다. 2) 지원은 StackoverFlow, Reddit 및 Slack 채널을 통해 얻을 수 있습니다. 3) RFC에 따라 개발 동향을 배울 수 있습니다. 4) 적극적인 참여, 코드에 대한 기여 및 학습 공유를 통해 커뮤니티에 통합 될 수 있습니다.
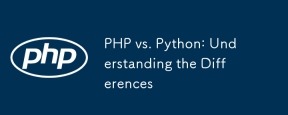
PHP와 Python은 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1.PHP는 간단한 구문과 높은 실행 효율로 웹 개발에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리를 갖춘 데이터 과학 및 기계 학습에 적합합니다.
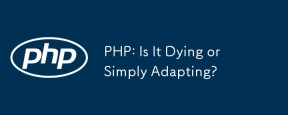
PHP는 죽지 않고 끊임없이 적응하고 진화합니다. 1) PHP는 1994 년부터 새로운 기술 트렌드에 적응하기 위해 여러 버전 반복을 겪었습니다. 2) 현재 전자 상거래, 컨텐츠 관리 시스템 및 기타 분야에서 널리 사용됩니다. 3) PHP8은 성능과 현대화를 개선하기 위해 JIT 컴파일러 및 기타 기능을 소개합니다. 4) Opcache를 사용하고 PSR-12 표준을 따라 성능 및 코드 품질을 최적화하십시오.
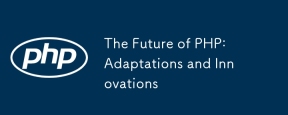
PHP의 미래는 새로운 기술 트렌드에 적응하고 혁신적인 기능을 도입함으로써 달성 될 것입니다. 1) 클라우드 컴퓨팅, 컨테이너화 및 마이크로 서비스 아키텍처에 적응, Docker 및 Kubernetes 지원; 2) 성능 및 데이터 처리 효율을 향상시키기 위해 JIT 컴파일러 및 열거 유형을 도입합니다. 3) 지속적으로 성능을 최적화하고 모범 사례를 홍보합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
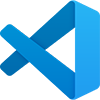
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
