C#에서 최단 경로 알고리즘을 구현하는 방법은 구체적인 코드 예제가 필요합니다
최단 경로 알고리즘은 그래프 이론에서 중요한 알고리즘으로, 그래프에서 두 정점 사이의 최단 경로를 찾는 데 사용됩니다. 이 기사에서는 C# 언어를 사용하여 Dijkstra 알고리즘과 Bellman-Ford 알고리즘이라는 두 가지 고전적인 최단 경로 알고리즘을 구현하는 방법을 소개합니다.
Dijkstra의 알고리즘은 널리 사용되는 단일 소스 최단 경로 알고리즘입니다. 기본 아이디어는 시작 정점에서 시작하여 점차적으로 다른 노드로 확장하고 발견된 노드의 최단 경로를 업데이트하는 것입니다. 아래는 Dijkstra의 알고리즘을 사용하여 최단 경로를 해결하는 샘플 코드입니다.
using System; using System.Collections.Generic; public class DijkstraAlgorithm { private int vertexCount; private int[] distance; private bool[] visited; private List<List<int>> adjacencyMatrix; public DijkstraAlgorithm(List<List<int>> graph) { vertexCount = graph.Count; distance = new int[vertexCount]; visited = new bool[vertexCount]; adjacencyMatrix = graph; } public void FindShortestPath(int startVertex) { // 初始化距离数组和访问数组 for (int i = 0; i < vertexCount; i++) { distance[i] = int.MaxValue; visited[i] = false; } // 起始顶点到自身的距离为0 distance[startVertex] = 0; for (int i = 0; i < vertexCount - 1; i++) { int u = FindMinDistance(); // 标记u为已访问 visited[u] = true; // 更新u的邻接顶点的距离 for (int v = 0; v < vertexCount; v++) { if (!visited[v] && adjacencyMatrix[u][v] != 0 && distance[u] != int.MaxValue && distance[u] + adjacencyMatrix[u][v] < distance[v]) { distance[v] = distance[u] + adjacencyMatrix[u][v]; } } } // 输出最短路径 Console.WriteLine("顶点 最短路径"); for (int i = 0; i < vertexCount; i++) { Console.WriteLine(i + " " + distance[i]); } } private int FindMinDistance() { int minDistance = int.MaxValue; int minDistanceIndex = -1; for (int i = 0; i < vertexCount; i++) { if (!visited[i] && distance[i] <= minDistance) { minDistance = distance[i]; minDistanceIndex = i; } } return minDistanceIndex; } } public class Program { public static void Main(string[] args) { // 构建示例图 List<List<int>> graph = new List<List<int>>() { new List<int>() {0, 4, 0, 0, 0, 0, 0, 8, 0}, new List<int>() {4, 0, 8, 0, 0, 0, 0, 11, 0}, new List<int>() {0, 8, 0, 7, 0, 4, 0, 0, 2}, new List<int>() {0, 0, 7, 0, 9, 14, 0, 0, 0}, new List<int>() {0, 0, 0, 9, 0, 10, 0, 0, 0}, new List<int>() {0, 0, 4, 0, 10, 0, 2, 0, 0}, new List<int>() {0, 0, 0, 14, 0, 2, 0, 1, 6}, new List<int>() {8, 11, 0, 0, 0, 0, 1, 0, 7}, new List<int>() {0, 0, 2, 0, 0, 0, 6, 7, 0} }; // 使用Dijkstra算法求解最短路径 DijkstraAlgorithm dijkstraAlgorithm = new DijkstraAlgorithm(graph); dijkstraAlgorithm.FindShortestPath(0); } }
Bellman-Ford 알고리즘은 음의 가중치 그래프를 사용하여 최단 경로 문제를 해결하기 위한 알고리즘입니다. 정점의 최단 경로를 점진적으로 업데이트하기 위해 동적 프로그래밍 아이디어를 사용합니다. 다음은 Bellman-Ford 알고리즘을 사용하여 최단 경로를 푸는 샘플 코드입니다.
using System; using System.Collections.Generic; public class BellmanFordAlgorithm { private int vertexCount; private int[] distance; private List<Edge> edges; private class Edge { public int source; public int destination; public int weight; public Edge(int source, int destination, int weight) { this.source = source; this.destination = destination; this.weight = weight; } } public BellmanFordAlgorithm(int vertexCount) { this.vertexCount = vertexCount; distance = new int[vertexCount]; edges = new List<Edge>(); } public void AddEdge(int source, int destination, int weight) { edges.Add(new Edge(source, destination, weight)); } public void FindShortestPath(int startVertex) { // 初始化距离数组 for (int i = 0; i < vertexCount; i++) { distance[i] = int.MaxValue; } // 起始顶点到自身的距离为0 distance[startVertex] = 0; // 迭代vertexCount-1次,更新距离 for (int i = 0; i < vertexCount - 1; i++) { foreach (Edge edge in edges) { if (distance[edge.source] != int.MaxValue && distance[edge.source] + edge.weight < distance[edge.destination]) { distance[edge.destination] = distance[edge.source] + edge.weight; } } } // 检查是否存在负权环路 foreach (Edge edge in edges) { if (distance[edge.source] != int.MaxValue && distance[edge.source] + edge.weight < distance[edge.destination]) { Console.WriteLine("图中存在负权环路"); return; } } // 输出最短路径 Console.WriteLine("顶点 最短路径"); for (int i = 0; i < vertexCount; i++) { Console.WriteLine(i + " " + distance[i]); } } } public class Program { public static void Main(string[] args) { // 构建示例图 int vertexCount = 5; BellmanFordAlgorithm bellmanFordAlgorithm = new BellmanFordAlgorithm(vertexCount); bellmanFordAlgorithm.AddEdge(0, 1, 6); bellmanFordAlgorithm.AddEdge(0, 2, 7); bellmanFordAlgorithm.AddEdge(1, 2, 8); bellmanFordAlgorithm.AddEdge(1, 4, -4); bellmanFordAlgorithm.AddEdge(1, 3, 5); bellmanFordAlgorithm.AddEdge(2, 4, 9); bellmanFordAlgorithm.AddEdge(2, 3, -3); bellmanFordAlgorithm.AddEdge(3, 1, -2); bellmanFordAlgorithm.AddEdge(4, 3, 7); // 使用Bellman-Ford算法求解最短路径 bellmanFordAlgorithm.FindShortestPath(0); } }
위는 C# 언어를 사용하여 Dijkstra 알고리즘과 Bellman-Ford 알고리즘을 구현한 샘플 코드입니다. 이 두 가지 알고리즘을 사용하면 그래프의 최단 경로 문제를 해결할 수 있습니다.
위 내용은 C#에서 최단 경로 알고리즘을 구현하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
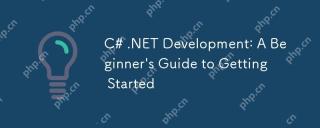
C# .NET 개발을 시작하려면 다음과 같은 것이 필요합니다. 1. C#의 기본 지식과 .NET 프레임 워크의 핵심 개념을 이해하십시오. 2. 변수, 데이터 유형, 제어 구조, 기능 및 클래스의 기본 개념을 마스터하십시오. 3. LINQ 및 비동기 프로그래밍과 같은 C#의 고급 기능을 배우십시오. 4. 일반적인 오류에 대한 디버깅 기술 및 성능 최적화 방법에 익숙해 지십시오. 이러한 단계를 통해 C#.NET의 세계를 점차적으로 침투하고 효율적인 응용 프로그램을 작성할 수 있습니다.
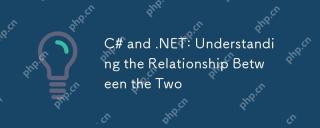
C#과 .NET의 관계는 분리 할 수 없지만 같은 것은 아닙니다. C#은 프로그래밍 언어이며 .NET은 개발 플랫폼입니다. C#은 코드를 작성하고 .NET의 중간 언어 (IL)로 컴파일하고 .NET 런타임 (CLR)에 의해 실행되는 데 사용됩니다.
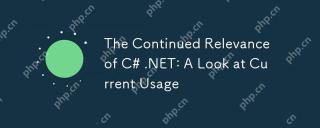
C#.NET은 여러 응용 프로그램 개발을 지원하는 강력한 도구 및 라이브러리를 제공하기 때문에 여전히 중요합니다. 1) C#은 .NET 프레임 워크를 결합하여 개발 효율적이고 편리하게 만듭니다. 2) C#의 타입 안전 및 쓰레기 수집 메커니즘은 장점을 향상시킵니다. 3) .NET은 크로스 플랫폼 실행 환경과 풍부한 API를 제공하여 개발 유연성을 향상시킵니다.
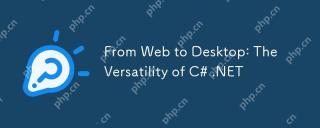
C#.NETISVERSATILEFORBOTHWEBBANDDESKTOPDEVENTROMMENT.1) FORWEB, useASP.NETFORRICHINTERFACES.3) FORDESKTOP.3) USEXAMARINFORCROSS-PLATFORMDEEVENTRIMMENT, LINABILEDEV, MACODEDEV, and MACODEDOWS, 및 MACODEDOWS.
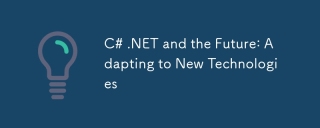
C# 및 .NET는 지속적인 업데이트 및 최적화를 통해 신흥 기술의 요구에 적응합니다. 1) C# 9.0 및 .NET5는 레코드 유형 및 성능 최적화를 소개합니다. 2) .NETCORE는 클라우드 네이티브 및 컨테이너화 된 지원을 향상시킵니다. 3) ASP.NETCORE는 최신 웹 기술과 통합됩니다. 4) ML.NET는 기계 학습 및 인공 지능을 지원합니다. 5) 비동기 프로그래밍 및 모범 사례는 성능을 향상시킵니다.
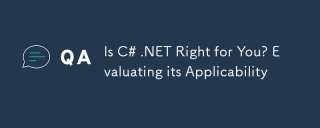
C#.netissuitable forenterprise-levelapplications는 richlibraries, androbustperformance, 그러나 itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical, wherelanguagesslikerustorthightordogrordogrognegrognegrognegrognecross-platformdevelopmentor.
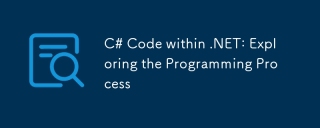
.NET에서 C#의 프로그래밍 프로세스에는 다음 단계가 포함됩니다. 1) C# 코드 작성, 2) 중간 언어 (IL)로 컴파일하고 .NET 런타임 (CLR)에 의해 실행됩니다. .NET에서 C#의 장점은 현대적인 구문, 강력한 유형 시스템 및 .NET 프레임 워크와의 긴밀한 통합으로 데스크탑 응용 프로그램에서 웹 서비스에 이르기까지 다양한 개발 시나리오에 적합합니다.
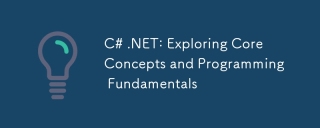
C#은 Microsoft가 개발 한 최신 객체 지향 프로그래밍 언어이며 .NET 프레임 워크의 일부로 개발되었습니다. 1.C#은 캡슐화, 상속 및 다형성을 포함한 객체 지향 프로그래밍 (OOP)을 지원합니다. 2. C#의 비동기 프로그래밍은 응용 프로그램 응답 성을 향상시키기 위해 비동기 및 키워드를 기다리는 키워드를 통해 구현됩니다. 3. LINQ를 사용하여 데이터 컬렉션을 간결하게 처리하십시오. 4. 일반적인 오류에는 NULL 참조 예외 및 인덱스 외 예외가 포함됩니다. 디버깅 기술에는 디버거 사용 및 예외 처리가 포함됩니다. 5. 성능 최적화에는 StringBuilder 사용 및 불필요한 포장 및 Unboxing을 피하는 것이 포함됩니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.
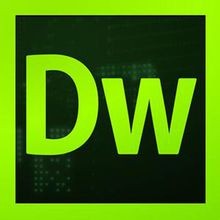
드림위버 CS6
시각적 웹 개발 도구
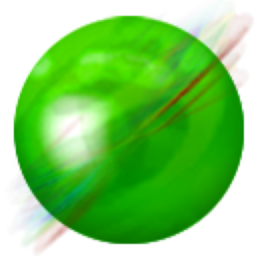
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
