여기에서는 Java를 사용하여 MySQL 데이터베이스의 모든 테이블을 표시하는 방법을 살펴보겠습니다. MySQL에서 show 명령을 사용하여 MySQL 데이터베이스의 모든 테이블을 가져올 수 있습니다.
우리 데이터베이스가 "test"라고 가정합니다. Java 코드는 다음과 같으며 "test" 데이터베이스의 모든 테이블 이름을 표시합니다.
Java 코드는 다음과 같습니다. 여기에서 MySQL과 Java 사이에 연결이 설정됩니다. -
import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import com.mysql.jdbc.Connection; import com.mysql.jdbc.DatabaseMetaData; public class GetAllTables { public static void main(String[] args) throws SQLException { Connection conn = null; try { try { Class.forName("com.mysql.jdbc.Driver"); } catch (Exception e) { System.out.println(e); } conn = (Connection) DriverManager.getConnection("jdbc:mysql://localhost/test", "Manish", "123456"); System.out.println("Connection is created succcessfully:"); } catch (Exception e) { System.out.println(e); } ResultSet rs = null; DatabaseMetaData meta = (DatabaseMetaData) conn.getMetaData(); rs = meta.getTables(null, null, null, new String[] { "TABLE" }); int count = 0; System.out.println("All table names are in test database:"); while (rs.next()) { String tblName = rs.getString("TABLE_NAME"); System.out.println(tblName); count++; } System.out.println(count + " Rows in set "); } }
아래는 데이터베이스 테스트의 모든 테이블을 보여주는 출력입니다. -
Wed Dec 12 14:55:28 IST 2018 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL = false, or set useSSL = true and provide truststore for server certificate verification. Connection is created succcessfully: All table names are in test database: add30minutesdemo addcolumn addoneday agecalculatesdemo aliasdemo allcharacterbeforespace allownulldemo appendingdatademo autoincrementdemo betweendatedemo bigintandintdemo bigintdemo bookdatedemo changecolumnpositiondemo changeenginetabledemo charsetdemo concatenatetwocolumnsdemo constraintdemo cumulativesumdemo currentdatetimedemo customers dateasstringdemo dateformatdemo dateinsertdemo datesofoneweek datetimedemo dayofweekdemo decimaltointdemo decrementdemo defaultdemo deleteallfromtable deletemanyrows destination differencetimestamp distinctdemo employee employeedesignation findlowercasevalue generatingnumbersdemo gmailsignin groupbytwofieldsdemo groupmonthandyeardemo highestidorderby highestnumberdemo ifnulldemo increasevarchardemo insert insertignoredemo insertwithmultipleandsigle int11demo intvsintanythingdemo lasttwocharacters likebinarydemo likedemo maxlengthfunctiondemo moviecollectiondemo myisamtoinnodbdemo newtableduplicate notequalsdemo nowandcurdatedemo nthrecorddemo nullandemptydemo orderbycharacterlength orderbynullfirstdemo orderindemo originaltable parsedatedemo passinganarraydemo persons prependstringoncolumnname pricedemo queryresultdemo replacedemo rowexistdemo rowpositiondemo rowwithsamevalue safedeletedemo searchtextdemo selectdataonyearandmonthdemo selectdistincttwocolumns selectdomainnameonly sha256demo skiplasttenrecords sortcolumnzeroatlastdemo storedproctable stringreplacedemo stringtodate student studentdemo studentmodifytabledemo studenttable subtract3hours temporarycolumnwithvaluedemo timetosecond timetoseconddemo toggledemo toogledemo truncatetabledemo updatealldemo updatevalueincrementally wheredemo wholewordmatchdemo zipcodepadwithzerodemo 103 Rows in set
교차 확인하려면 MySQL show 명령을 사용하여 데이터베이스 내의 모든 테이블을 표시합니다. ". 쿼리는 다음과 같습니다. -
mysql> use test; Database changed mysql> show tables;
다음은 출력입니다. -
+------------------------------+ | Tables_in_test | +------------------------------+ | add30minutesdemo | | addcolumn | | addoneday | | agecalculatesdemo | | aliasdemo | | allcharacterbeforespace | | allownulldemo | | appendingdatademo | | autoincrementdemo | | betweendatedemo | | bigintandintdemo | | bigintdemo | | bookdatedemo | | changecolumnpositiondemo | | changeenginetabledemo | | charsetdemo | | concatenatetwocolumnsdemo | | constraintdemo | | cumulativesumdemo | | currentdatetimedemo | | customers | | dateasstringdemo | | dateformatdemo | | dateinsertdemo | | datesofoneweek | | datetimedemo | | dayofweekdemo | | decimaltointdemo | | decrementdemo | | defaultdemo | | deleteallfromtable | | deletemanyrows | | destination | | differencetimestamp | | distinctdemo | | employee | | employeedesignation | | findlowercasevalue | | generatingnumbersdemo | | gmailsignin | | groupbytwofieldsdemo | | groupmonthandyeardemo | | highestidorderby | | highestnumberdemo | | ifnulldemo | | increasevarchardemo | | insert | | insertignoredemo | | insertwithmultipleandsigle | | int11demo | | intvsintanythingdemo | | lasttwocharacters | | likebinarydemo | | likedemo | | maxlengthfunctiondemo | | moviecollectiondemo | | myisamtoinnodbdemo | | newtableduplicate | | notequalsdemo | | nowandcurdatedemo | | nthrecorddemo | | nullandemptydemo | | orderbycharacterlength | | orderbynullfirstdemo | | orderindemo | | originaltable | | parsedatedemo | | passinganarraydemo | | persons | | prependstringoncolumnname | | pricedemo | | queryresultdemo | | replacedemo | | rowexistdemo | | rowpositiondemo | | rowwithsamevalue | | safedeletedemo | | searchtextdemo | | selectdataonyearandmonthdemo | | selectdistincttwocolumns | | selectdomainnameonly | | sha256demo | | skiplasttenrecords | | sortcolumnzeroatlastdemo | | storedproctable | | stringreplacedemo | | stringtodate | | student | | studentdemo | | studentmodifytabledemo | | studenttable | | subtract3hours | | temporarycolumnwithvaluedemo | | timetosecond | | timetoseconddemo | | toggledemo | | toogledemo | | truncatetabledemo | | updatealldemo | | updatevalueincrementally | | wheredemo | | wholewordmatchdemo | | zipcodepadwithzerodemo | +------------------------------+ 103 rows in set (0.01 sec)
위에서 볼 수 있듯이 모두 동일한 결과를 제공합니다.
위 내용은 Java를 사용하여 MySQL 데이터베이스의 모든 테이블을 표시하시겠습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

데이터베이스 및 프로그래밍에서 MySQL의 위치는 매우 중요합니다. 다양한 응용 프로그램 시나리오에서 널리 사용되는 오픈 소스 관계형 데이터베이스 관리 시스템입니다. 1) MySQL은 웹, 모바일 및 엔터프라이즈 레벨 시스템을 지원하는 효율적인 데이터 저장, 조직 및 검색 기능을 제공합니다. 2) 클라이언트 서버 아키텍처를 사용하고 여러 스토리지 엔진 및 인덱스 최적화를 지원합니다. 3) 기본 사용에는 테이블 작성 및 데이터 삽입이 포함되며 고급 사용에는 다중 테이블 조인 및 복잡한 쿼리가 포함됩니다. 4) SQL 구문 오류 및 성능 문제와 같은 자주 묻는 질문은 설명 명령 및 느린 쿼리 로그를 통해 디버깅 할 수 있습니다. 5) 성능 최적화 방법에는 인덱스의 합리적인 사용, 최적화 된 쿼리 및 캐시 사용이 포함됩니다. 모범 사례에는 거래 사용 및 준비된 체계가 포함됩니다
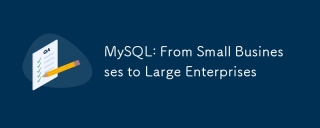
MySQL은 소규모 및 대기업에 적합합니다. 1) 소기업은 고객 정보 저장과 같은 기본 데이터 관리에 MySQL을 사용할 수 있습니다. 2) 대기업은 MySQL을 사용하여 대규모 데이터 및 복잡한 비즈니스 로직을 처리하여 쿼리 성능 및 트랜잭션 처리를 최적화 할 수 있습니다.
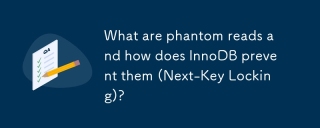
InnoDB는 팬텀 읽기를 차세대 점화 메커니즘을 통해 효과적으로 방지합니다. 1) Next-Keylocking은 Row Lock과 Gap Lock을 결합하여 레코드와 간격을 잠그기 위해 새로운 레코드가 삽입되지 않도록합니다. 2) 실제 응용 분야에서 쿼리를 최적화하고 격리 수준을 조정함으로써 잠금 경쟁을 줄이고 동시성 성능을 향상시킬 수 있습니다.
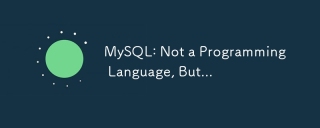
MySQL은 프로그래밍 언어가 아니지만 쿼리 언어 SQL은 프로그래밍 언어의 특성을 가지고 있습니다. 1. SQL은 조건부 판단, 루프 및 가변 작업을 지원합니다. 2. 저장된 절차, 트리거 및 기능을 통해 사용자는 데이터베이스에서 복잡한 논리 작업을 수행 할 수 있습니다.
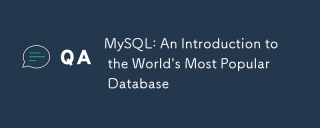
MySQL은 오픈 소스 관계형 데이터베이스 관리 시스템으로, 주로 데이터를 신속하고 안정적으로 저장하고 검색하는 데 사용됩니다. 작업 원칙에는 클라이언트 요청, 쿼리 해상도, 쿼리 실행 및 반환 결과가 포함됩니다. 사용의 예로는 테이블 작성, 데이터 삽입 및 쿼리 및 조인 작업과 같은 고급 기능이 포함됩니다. 일반적인 오류에는 SQL 구문, 데이터 유형 및 권한이 포함되며 최적화 제안에는 인덱스 사용, 최적화 된 쿼리 및 테이블 분할이 포함됩니다.
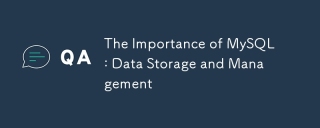
MySQL은 데이터 저장, 관리, 쿼리 및 보안에 적합한 오픈 소스 관계형 데이터베이스 관리 시스템입니다. 1. 다양한 운영 체제를 지원하며 웹 응용 프로그램 및 기타 필드에서 널리 사용됩니다. 2. 클라이언트-서버 아키텍처 및 다양한 스토리지 엔진을 통해 MySQL은 데이터를 효율적으로 처리합니다. 3. 기본 사용에는 데이터베이스 및 테이블 작성, 데이터 삽입, 쿼리 및 업데이트가 포함됩니다. 4. 고급 사용에는 복잡한 쿼리 및 저장 프로 시저가 포함됩니다. 5. 설명 진술을 통해 일반적인 오류를 디버깅 할 수 있습니다. 6. 성능 최적화에는 인덱스의 합리적인 사용 및 최적화 된 쿼리 문이 포함됩니다.
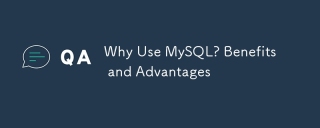
MySQL은 성능, 신뢰성, 사용 편의성 및 커뮤니티 지원을 위해 선택됩니다. 1.MYSQL은 효율적인 데이터 저장 및 검색 기능을 제공하여 여러 데이터 유형 및 고급 쿼리 작업을 지원합니다. 2. 고객-서버 아키텍처 및 다중 스토리지 엔진을 채택하여 트랜잭션 및 쿼리 최적화를 지원합니다. 3. 사용하기 쉽고 다양한 운영 체제 및 프로그래밍 언어를 지원합니다. 4. 강력한 지역 사회 지원을 받고 풍부한 자원과 솔루션을 제공합니다.
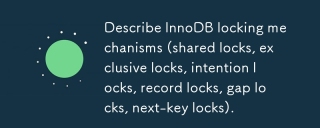
InnoDB의 잠금 장치에는 공유 잠금 장치, 독점 잠금, 의도 잠금 장치, 레코드 잠금, 갭 잠금 및 다음 키 잠금 장치가 포함됩니다. 1. 공유 잠금을 사용하면 다른 트랜잭션을 읽지 않고 트랜잭션이 데이터를 읽을 수 있습니다. 2. 독점 잠금은 다른 트랜잭션이 데이터를 읽고 수정하는 것을 방지합니다. 3. 의도 잠금은 잠금 효율을 최적화합니다. 4. 레코드 잠금 잠금 인덱스 레코드. 5. 갭 잠금 잠금 장치 색인 기록 간격. 6. 다음 키 잠금은 데이터 일관성을 보장하기 위해 레코드 잠금과 갭 잠금의 조합입니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
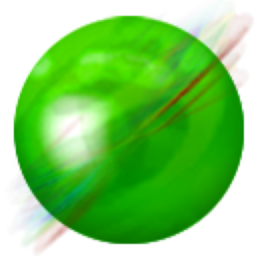
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
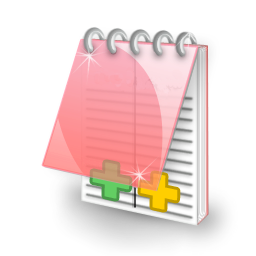
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
