Python을 사용하여 이미지에서 스타일 전송을 수행하는 방법
소개:
스타일 전송은 컴퓨터 비전 분야에서 흥미롭고 도전적인 작업으로, 한 이미지의 내용을 다른 이미지의 스타일과 합성할 수 있습니다. 독특한 예술적 효과는 이미지 처리, 디자인, 엔터테인먼트 및 기타 분야에서 널리 사용됩니다. 이 기사에서는 딥 러닝 알고리즘과 결합된 Python 프로그래밍 언어를 사용하여 그림의 스타일 이전을 달성하는 방법을 소개합니다.
1단계: 필수 라이브러리 가져오기
먼저 TensorFlow, Keras, NumPy 및 Matplotlib를 포함한 일부 필수 Python 라이브러리를 가져와야 합니다. 다음 코드를 실행하세요:
import tensorflow as tf from tensorflow import keras import numpy as np import matplotlib.pyplot as plt
2단계: 사전 훈련된 모델 로드
스타일 전송에서는 VGG19와 같은 사전 훈련된 컨벌루션 신경망 모델을 사용할 수 있습니다. 이 모델은 이미지 인식 작업에 적합하며 스타일 전송 작업에도 널리 사용됩니다. 다음 코드를 실행하세요:
vgg = tf.keras.applications.VGG19(include_top=False, weights='imagenet') vgg.trainable = False
3단계: 콘텐츠 손실 정의
콘텐츠 손실은 두 이미지 간의 콘텐츠 차이를 측정하는 데 사용됩니다. VGG 모델의 중간 레이어를 사용하여 이미지의 콘텐츠 특징을 추출할 수 있습니다. 구체적으로, VGG 모델의 특정 컨볼루셔널 레이어를 콘텐츠 레이어로 선택하고 이러한 레이어의 입력 이미지와 대상 이미지의 특징 표현을 비교할 수 있습니다. 다음 코드를 실행하세요:
content_layers = ['block5_conv2'] content_extractor = keras.Model(inputs=vgg.input, outputs=[vgg.get_layer(name).output for name in content_layers])
4단계: 스타일 손실 정의
스타일 손실은 두 이미지 간의 스타일 차이를 측정하는 데 사용됩니다. 그램 행렬을 사용하여 사진의 여러 채널 간의 상관 관계를 표현한 다음 질감, 색상 등의 측면에서 사진의 특성을 측정할 수 있습니다. 다음 코드를 실행하세요:
style_layers = ['block1_conv1', 'block2_conv1', 'block3_conv1', 'block4_conv1', 'block5_conv1'] style_extractor = keras.Model(inputs=vgg.input, outputs=[vgg.get_layer(name).output for name in style_layers]) def gram_matrix(input_tensor): channels = int(input_tensor.shape[-1]) a = tf.reshape(input_tensor, [-1, channels]) n = tf.shape(a)[0] gram = tf.matmul(a, a, transpose_a=True) return gram / tf.cast(n, tf.float32)
5단계: 전체 변형 손실 정의
전체 변형 손실은 합성 이미지를 매끄럽게 유지하는 데 사용됩니다. 합성 이미지의 각 픽셀과 인접 픽셀 간의 차이를 합산하여 노이즈와 불연속적인 가장자리를 줄일 수 있습니다. 다음 코드를 실행하세요:
def total_variation_loss(image): x = tf.image.image_gradients(image) return tf.reduce_sum(tf.abs(x[0])) + tf.reduce_sum(tf.abs(x[1]))
6단계: 목적 함수 정의
콘텐츠 손실, 스타일 손실, 전체 변형 손실을 결합하여 포괄적인 목적 함수를 형성합니다. 목적 함수는 이미지의 내용과 스타일 간의 차이를 최소화하고 제약 조건을 만족하는 합성 이미지를 생성하는 데 사용됩니다. 다음 코드를 실행하세요:
def compute_loss(image, content_features, style_features): content_output = content_extractor(image) style_output = style_extractor(image) content_loss = tf.reduce_mean(tf.square(content_output - content_features)) style_loss = tf.add_n([tf.reduce_mean(tf.square(style_output[i] - style_features[i])) for i in range(len(style_output))]) content_loss *= content_weight style_loss *= style_weight tv_loss = total_variation_loss(image) * total_variation_weight loss = content_loss + style_loss + tv_loss return loss @tf.function() def train_step(image, content_features, style_features, optimizer): with tf.GradientTape() as tape: loss = compute_loss(image, content_features, style_features) gradients = tape.gradient(loss, image) optimizer.apply_gradients([(gradients, image)]) image.assign(tf.clip_by_value(image, 0.0, 1.0))
7단계: 스타일 전송 수행
모델 정의를 완료한 후 사용자 지정 학습 기능을 사용하여 합성 이미지를 반복적으로 최적화하여 콘텐츠의 대상 이미지와 최대한 유사하도록 할 수 있습니다. 그리고 스타일. 다음 코드를 실행합니다.
def style_transfer(content_path, style_path, num_iteration=1000, content_weight=1e3, style_weight=1e-2, total_variation_weight=30): content_image = load_image(content_path) style_image = load_image(style_path) content_features = content_extractor(content_image) style_features = style_extractor(style_image) opt = keras.optimizers.Adam(learning_rate=0.02, beta_1=0.99, epsilon=1e-1) image = tf.Variable(content_image) start_time = time.time() for i in range(num_iteration): train_step(image, content_features, style_features, opt) if i % 100 == 0: elapsed_time = time.time() - start_time print('Iteration: %d, Time: %.2fs' % (i, elapsed_time)) plt.imshow(image.read_value()[0]) plt.axis('off') plt.show() image = image.read_value()[0] return image
8단계: 스타일 마이그레이션 수행
마지막으로 콘텐츠 이미지와 스타일 이미지를 선택한 후 style_transfer()
함수를 호출하여 스타일 마이그레이션을 수행합니다. 다음 코드를 실행하세요:
content_path = 'content.jpg' style_path = 'style.jpg' output_image = style_transfer(content_path, style_path) plt.imshow(output_image) plt.axis('off') plt.show()
결론:
이 기사에서는 Python 프로그래밍 언어를 딥 러닝 알고리즘과 결합하여 그림의 스타일 전환을 달성하는 방법을 소개합니다. 사전 학습된 모델을 로딩하고, 콘텐츠 손실, 스타일 손실, 전체 변형 손실을 정의하고, 이를 맞춤형 학습 함수와 결합함으로써 콘텐츠 이미지와 스타일 이미지를 각각의 특성이 결합된 새로운 이미지로 합성할 수 있습니다. 지속적인 반복 최적화를 통해 주어진 제약 조건을 만족하는 최종 합성 이미지를 얻을 수 있습니다. 독자들이 이 기사의 소개를 통해 스타일 전송의 기본 원리와 구현 방법을 이해하고, 이미지 처리 및 예술적 창작과 같은 분야에서 이 기술의 잠재력을 더 탐구하고 적용할 수 있기를 바랍니다.
위 내용은 Python을 사용하여 이미지에 스타일 전송을 수행하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
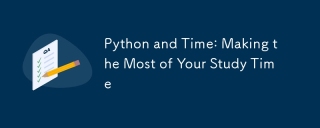
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.
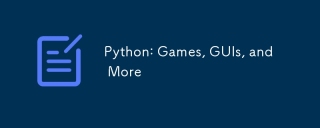
Python은 게임 및 GUI 개발에서 탁월합니다. 1) 게임 개발은 Pygame을 사용하여 드로잉, 오디오 및 기타 기능을 제공하며 2D 게임을 만드는 데 적합합니다. 2) GUI 개발은 Tkinter 또는 PYQT를 선택할 수 있습니다. Tkinter는 간단하고 사용하기 쉽고 PYQT는 풍부한 기능을 가지고 있으며 전문 개발에 적합합니다.
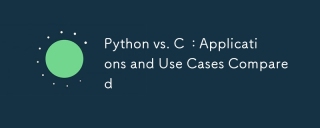
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
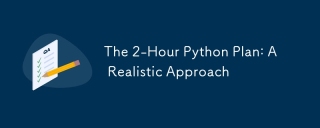
2 시간 이내에 Python의 기본 프로그래밍 개념과 기술을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우기, 2. 마스터 제어 흐름 (조건부 명세서 및 루프), 3. 기능의 정의 및 사용을 이해하십시오. 4. 간단한 예제 및 코드 스 니펫을 통해 Python 프로그래밍을 신속하게 시작하십시오.
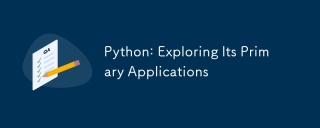
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
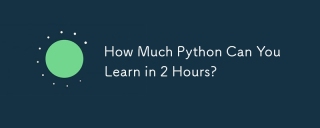
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
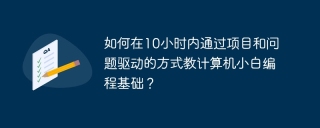
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
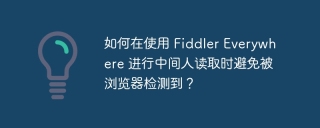
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
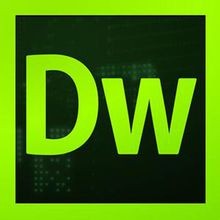
드림위버 CS6
시각적 웹 개발 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.
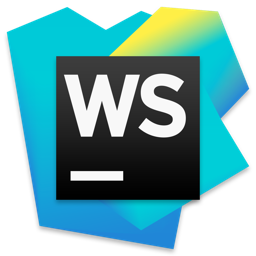
WebStorm Mac 버전
유용한 JavaScript 개발 도구
