Java에서 분산 잠금을 사용하여 분산 시스템을 동기화하는 방법은 무엇입니까?
소개:
분산 시스템에서는 여러 노드가 동시에 공유 리소스에 액세스할 때 데이터 충돌 및 동시성 문제가 발생할 수 있습니다. 데이터 일관성을 보장하려면 분산 잠금을 사용하여 분산 시스템을 동기화해야 합니다. Java는 분산 잠금을 구현하는 다양한 방법을 제공합니다. 이 기사에서는 ZooKeeper 및 Redis를 기반으로 하는 분산 잠금 구현 방법을 코드 예제와 함께 소개합니다.
1. ZooKeeper를 기반으로 한 분산 잠금 구현
ZooKeeper는 분산 시스템의 동기화 문제를 해결하기 위해 분산 잠금 메커니즘을 제공하는 분산 조정 서비스입니다. 다음은 ZooKeeper를 사용하여 분산 잠금을 구현하는 샘플 코드입니다.
import org.apache.zookeeper.*; import java.io.IOException; import java.util.Collections; import java.util.List; public class ZooKeeperDistributedLock implements Watcher { private ZooKeeper zooKeeper; private String lockPath; private String currentPath; private String waitPath; public ZooKeeperDistributedLock(String connectString, int sessionTimeout, String lockPath) throws IOException { zooKeeper = new ZooKeeper(connectString, sessionTimeout, this); this.lockPath = lockPath; } public void lock() throws KeeperException, InterruptedException { if (tryLock()) { return; } while (true) { List<String> children = zooKeeper.getChildren(lockPath, false); Collections.sort(children); int index = children.indexOf(currentPath.substring(lockPath.length() + 1)); if (index == 0) { return; } waitPath = lockPath + "/" + children.get(index - 1); zooKeeper.exists(waitPath, true); synchronized (this) { wait(); } } } public void unlock() throws KeeperException, InterruptedException { zooKeeper.delete(currentPath, -1); } private boolean tryLock() throws KeeperException, InterruptedException { currentPath = zooKeeper.create(lockPath + "/lock", new byte[0], ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL); List<String> children = zooKeeper.getChildren(lockPath, false); Collections.sort(children); if (currentPath.endsWith(children.get(0))) { return true; } String currentPathName = currentPath.substring(lockPath.length() + 1); int index = children.indexOf(currentPathName); if (index < 0) { throw new IllegalStateException("Node " + currentPathName + " no longer exists."); } else { waitPath = lockPath + "/" + children.get(index - 1); zooKeeper.exists(waitPath, true); synchronized (this) { wait(); } return false; } } @Override public void process(WatchedEvent event) { if (waitPath != null && event.getType() == Event.EventType.NodeDeleted && event.getPath().equals(waitPath)) { synchronized (this) { notifyAll(); } } } }
2. Redis 기반 분산 잠금 구현
Redis는 분산 잠금을 구현하기 위해 일부 원자 연산을 제공하는 고성능 키-값 저장 시스템입니다. 다음은 Redis를 사용하여 분산 잠금을 구현하는 샘플 코드입니다.
import redis.clients.jedis.Jedis; public class RedisDistributedLock { private Jedis jedis; private String lockKey; private String requestId; public RedisDistributedLock(String host, int port, String password, String lockKey, String requestId) { jedis = new Jedis(host, port); jedis.auth(password); this.lockKey = lockKey; this.requestId = requestId; } public boolean lock(long expireTimeMillis) { String result = jedis.set(lockKey, requestId, "NX", "PX", expireTimeMillis); return "OK".equals(result); } public boolean unlock() { Long result = (Long) jedis.eval( "if redis.call('get', KEYS[1]) == ARGV[1] then " + "return redis.call('del', KEYS[1]) " + "else " + "return 0 " + "end", 1, lockKey, requestId); return result != null && result == 1; } }
결론:
이 기사에서는 ZooKeeper와 Redis를 기반으로 Java에서 분산 잠금을 사용하여 분산 시스템의 동기화를 달성하는 두 가지 방법을 소개합니다. ZooKeeper를 사용하든 Redis를 사용하든 분산 시스템의 동기화를 효과적으로 달성하고 데이터 일관성을 보장할 수 있습니다. 실제 프로젝트에서는 특정 요구 사항과 성능 요구 사항을 기반으로 적절한 분산 잠금 솔루션을 선택해야 합니다. 이 글이 여러분에게 도움이 되기를 바랍니다. 읽어주셔서 감사합니다!
위 내용은 분산 시스템의 동기화를 달성하기 위해 Java에서 분산 잠금을 사용하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
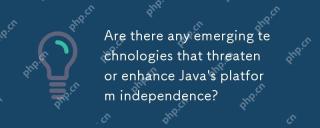
신흥 기술은 위협을 일으키고 Java의 플랫폼 독립성을 향상시킵니다. 1) Docker와 같은 클라우드 컴퓨팅 및 컨테이너화 기술은 Java의 플랫폼 독립성을 향상 시키지만 다양한 클라우드 환경에 적응하도록 최적화되어야합니다. 2) WebAssembly는 Graalvm을 통해 Java 코드를 컴파일하여 플랫폼 독립성을 확장하지만 성능을 위해 다른 언어와 경쟁해야합니다.
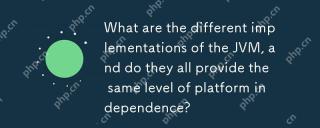
다른 JVM 구현은 플랫폼 독립성을 제공 할 수 있지만 성능은 약간 다릅니다. 1. OracleHotspot 및 OpenJDKJVM 플랫폼 독립성에서 유사하게 수행되지만 OpenJDK에는 추가 구성이 필요할 수 있습니다. 2. IBMJ9JVM은 특정 운영 체제에서 최적화를 수행합니다. 3. Graalvm은 여러 언어를 지원하며 추가 구성이 필요합니다. 4. AzulzingJVM에는 특정 플랫폼 조정이 필요합니다.
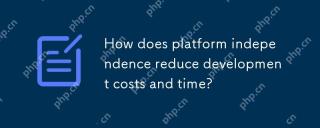
플랫폼 독립성은 여러 운영 체제에서 동일한 코드 세트를 실행하여 개발 비용을 줄이고 개발 시간을 단축시킵니다. 구체적으로, 그것은 다음과 같이 나타납니다. 1. 개발 시간을 줄이면 하나의 코드 세트 만 필요합니다. 2. 유지 보수 비용을 줄이고 테스트 프로세스를 통합합니다. 3. 배포 프로세스를 단순화하기위한 빠른 반복 및 팀 협업.
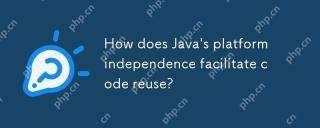
Java'SplatformIndenceFacilitatesCodereScoderEByWatHeAveringByTeCodetOrunonAnyPlatformwitHajvm.1) DevelopersCanwriteCodeOnceforConsentEStentBehaviorAcRossPlatforms.2) MAINTENDUCEDSCODEDOES.3) LIBRRIESASHSCORAREDERSCRAPERAREDERSPROJ
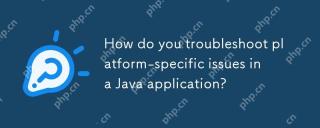
Java 응용 프로그램의 플랫폼 별 문제를 해결하려면 다음 단계를 수행 할 수 있습니다. 1. Java의 시스템 클래스를 사용하여 시스템 속성을보고 실행중인 환경을 이해합니다. 2. 파일 클래스 또는 java.nio.file 패키지를 사용하여 파일 경로를 처리하십시오. 3. 운영 체제 조건에 따라 로컬 라이브러리를로드하십시오. 4. visualVM 또는 JProfiler를 사용하여 크로스 플랫폼 성능을 최적화하십시오. 5. 테스트 환경이 Docker Containerization을 통해 생산 환경과 일치하는지 확인하십시오. 6. githubactions를 사용하여 여러 플랫폼에서 자동 테스트를 수행하십시오. 이러한 방법은 Java 응용 프로그램에서 플랫폼 별 문제를 효과적으로 해결하는 데 도움이됩니다.
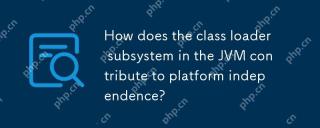
클래스 로더는 통합 클래스 파일 형식, 동적로드, 부모 위임 모델 및 플랫폼 독립적 인 바이트 코드를 통해 다른 플랫폼에서 Java 프로그램의 일관성과 호환성을 보장하고 플랫폼 독립성을 달성합니다.
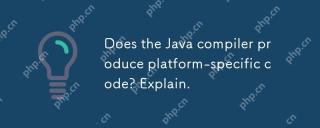
Java 컴파일러가 생성 한 코드는 플랫폼 독립적이지만 궁극적으로 실행되는 코드는 플랫폼 별입니다. 1. Java 소스 코드는 플랫폼 독립적 인 바이트 코드로 컴파일됩니다. 2. JVM은 바이트 코드를 특정 플랫폼의 기계 코드로 변환하여 크로스 플랫폼 작동을 보장하지만 성능이 다를 수 있습니다.
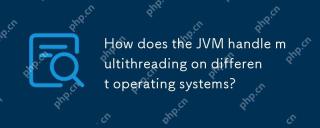
멀티 스레딩은 프로그램 대응 성과 리소스 활용을 향상시키고 복잡한 동시 작업을 처리 할 수 있기 때문에 현대 프로그래밍에서 중요합니다. JVM은 스레드 매핑, 스케줄링 메커니즘 및 동기화 잠금 메커니즘을 통해 다양한 운영 체제에서 멀티 스레드의 일관성과 효율성을 보장합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구
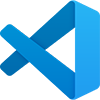
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
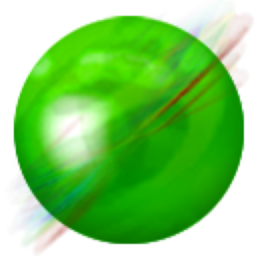
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.
