Java에서 비동기 병합 작업을 위해 CompletableFuture의 thenCompose 및 thenCombine 함수를 사용하는 방법
Java에서는 비동기 작업이 필요한 시나리오를 자주 접하게 됩니다. 이러한 상황을 위해 Java 8에서는 비동기 프로그래밍을 더 간단하고 쉽게 만들 수 있는 풍부한 비동기 프로그래밍 도구를 제공하는 CompletableFuture 클래스를 도입했습니다. 그중 thenCompose와 thenCombine은 CompletableFuture 클래스에서 일반적으로 사용되는 두 가지 결합 비동기 작업 메서드입니다.
1. thenCompose
ThethenCompose 메서드를 사용하면 CompletableFuture 인스턴스를 다른 CompletableFuture 인스턴스로 변환할 수 있습니다. 특히 이전 CompletableFuture에서 반환된 결과를 입력으로 사용하고 새 CompletableFuture 개체를 반환하는 Function 매개 변수를 받습니다. 예는 다음과 같습니다.
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } return 10; }); CompletableFuture<Integer> result = future.thenCompose(value -> CompletableFuture.supplyAsync(() -> value * 2)); result.whenComplete((res, ex) -> { if (ex != null) { ex.printStackTrace(); } else { System.out.println(res); } });
위 코드에서는 먼저 다른 스레드에서 계산 시간을 시뮬레이션하는 CompletableFuture 인스턴스를 만듭니다. 다음으로 thenCompose 메서드를 사용하여 이를 새로운 CompletableFuture 인스턴스로 변환합니다. 이 인스턴스는 이전 CompletableFuture에서 반환된 결과에 2를 곱합니다. 마지막으로 whenComplete 메서드를 사용하여 결과나 오류 메시지를 출력합니다.
2. thenCombine 사용
ThenCombine 메소드는 두 개의 CompletableFuture 인스턴스를 하나로 병합하는 데 사용됩니다. 특히, 또 다른 CompletableFuture 인스턴스와 두 CompletableFuture에서 반환된 결과를 입력으로 사용하고 새 CompletableFuture 객체를 반환하는 BiFunction 매개변수를 받습니다. 예는 다음과 같습니다.
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } return 10; }); CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } return 5; }); CompletableFuture<Integer> result = future1.thenCombine(future2, (value1, value2) -> value1 + value2); result.whenComplete((res, ex) -> { if (ex != null) { ex.printStackTrace(); } else { System.out.println(res); } });
위 코드에서는 두 개의 컴퓨팅 작업 실행을 각각 시뮬레이션하는 두 개의 CompletableFuture 인스턴스를 만듭니다. 다음으로 thenCombine 메서드를 사용하여 두 개의 CompletableFuture 인스턴스를 처음 두 개의 CompletableFuture에서 반환된 결과를 추가하는 새 인스턴스로 병합합니다. 마지막으로 결과나 오류 메시지를 출력하기 위해 whenComplete 메소드를 사용합니다.
3. thenCompose 및 thenCombine을 사용하여 복잡한 비동기 작업 구현
우리는 이미 thenCompose 및 thenCombine 메서드의 사용을 소개했으며 둘 다 매우 유용한 비동기 작업 메서드입니다. 실제로 이를 사용하여 여러 계산 결과에 대한 집계 작업과 같은 보다 복잡한 비동기 작업을 구현할 수도 있습니다.
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } return 10; }); CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } return 5; }); CompletableFuture<Integer> future3 = CompletableFuture.supplyAsync(() -> { // 模拟计算耗时 try { Thread.sleep(3000); } catch (InterruptedException e) { e.printStackTrace(); } return 20; }); CompletableFuture<Void> combinedFuture = CompletableFuture.allOf(future1, future2, future3); CompletableFuture<Integer> result = combinedFuture.thenCompose( voidResult -> CompletableFuture.supplyAsync(() -> { int sum = future1.join() + future2.join() + future3.join(); return sum; })); result.whenComplete((res, ex) -> { if (ex != null) { ex.printStackTrace(); } else { System.out.println(res); } });
위 코드에서는 세 개의 CompletableFuture 인스턴스를 만들었습니다. 각 인스턴스는 컴퓨팅 작업 실행을 시뮬레이션하고 해당 결과를 반환합니다. 다음으로 CompletableFuture.allOf 메서드를 사용하여 이 세 인스턴스를 결합하고 새 CompletableFuture 인스턴스를 만듭니다. 여기서 allOf 메소드는 Void 유형의 CompletableFuture 인스턴스를 반환하고 해당 반환 값은 null이라는 점에 유의해야 합니다.
그런 다음 thenCompose 메서드를 사용하여 null을 반환하는 위의 CompletableFuture 인스턴스를 새로운 CompletableFuture 인스턴스로 변환합니다. 이 인스턴스는 이전 세 개의 CompletableFuture에서 반환된 결과를 추가합니다. thenCompose 메소드의 콜백 함수에서는 Join() 메소드를 사용하여 각 CompletableFuture 인스턴스의 결과 값을 얻고 해당 계산을 수행합니다. 마지막으로 결과나 오류 메시지를 출력하기 위해 whenComplete 메소드를 사용합니다.
일반적으로 thenCompose와 thenCombine은 CompletableFuture 클래스에서 매우 유용한 메서드로, 비동기 작업을 보다 편리하게 수행하고 프로그램의 동시성과 실행 효율성을 향상시키는 데 도움이 됩니다.
위 내용은 Java에서 비동기 병합 작업을 위해 CompletableFuture의 thenCompose 및 thenCombine 함수를 사용하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

이 기사에서는 Java 프로젝트 관리, 구축 자동화 및 종속성 해상도에 Maven 및 Gradle을 사용하여 접근 방식과 최적화 전략을 비교합니다.
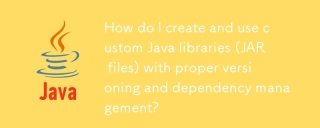
이 기사에서는 Maven 및 Gradle과 같은 도구를 사용하여 적절한 버전 및 종속성 관리로 사용자 정의 Java 라이브러리 (JAR Files)를 작성하고 사용하는 것에 대해 설명합니다.
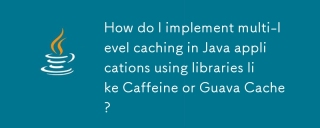
이 기사는 카페인 및 구아바 캐시를 사용하여 자바에서 다단계 캐싱을 구현하여 응용 프로그램 성능을 향상시키는 것에 대해 설명합니다. 구성 및 퇴거 정책 관리 Best Pra와 함께 설정, 통합 및 성능 이점을 다룹니다.

이 기사는 캐싱 및 게으른 하중과 같은 고급 기능을 사용하여 객체 관계 매핑에 JPA를 사용하는 것에 대해 설명합니다. 잠재적 인 함정을 강조하면서 성능을 최적화하기위한 설정, 엔티티 매핑 및 모범 사례를 다룹니다. [159 문자]
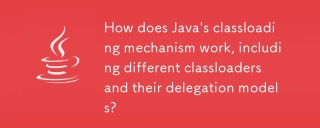
Java의 클래스 로딩에는 부트 스트랩, 확장 및 응용 프로그램 클래스 로더가있는 계층 적 시스템을 사용하여 클래스로드, 링크 및 초기화 클래스가 포함됩니다. 학부모 위임 모델은 핵심 클래스가 먼저로드되어 사용자 정의 클래스 LOA에 영향을 미치도록합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
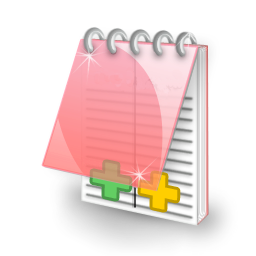
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
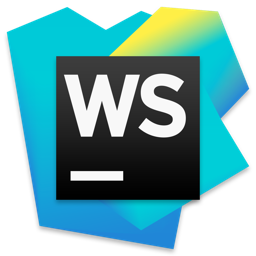
WebStorm Mac 버전
유용한 JavaScript 개발 도구
