1.ThreadLocal 스레드 변수는 현재 스레드에 바인딩되어 있으며 현재 스레드의 변수만 저장하며 다른 스레드와 격리되어 내부 데이터에 액세스할 수 없습니다.
2. ThreadLocal은 Looper에서 사용되며 ThreadLocal에 Looper가 생성되어 저장됩니다.
//这里用到了泛型,ThreadLocal中只保存Looper对象。 static final ThreadLocal<Looper> sThreadLocal = new ThreadLocal<Looper>(); private static void prepare(boolean quitAllowed) { if (sThreadLocal.get() != null) { //保证Looper只被创建一次。 throw new RuntimeException("Only one Looper may be created per thread"); } sThreadLocal.set(new Looper(quitAllowed)); }
sThreadLocal.set() 메서드가 데이터를 저장하는 방법을 살펴보세요.
먼저 현재 스레드를 가져온 다음 스레드의 ThreadLocalMap 멤버 변수를 가져온 다음 이 맵에 저장합니다.
key는 생성된 ThreadLocal 개체이고 value는 전달된 값입니다.
public void set(T value) { //拿到当前线程 Thread t = Thread.currentThread(); //得到一个map ThreadLocalMap map = getMap(t); if (map != null){ // 这个map是以当前对象为key的,这个this就是 ThreadLocal的实例 sThreadLocal map.set(this, value); }else{ createMap(t, value); } }
//getMap 是从Thread中拿到了一个threadLocals变量,是ThreadLocal.ThreadLocalMap 的实例。 //保存的数据也是存在了这个map中,这也就是为什么ThreadLocal是和线程绑定的,对其他线程来说是隔离的原因所在。 ThreadLocalMap getMap(Thread t) { return t.threadLocals; }
1) 지도가 비어 있지 않으면 데이터를 저장하세요. 위의 if 판단의 첫 번째 분기를 사용하세요. 이 저장 방식은 HashMap
private void set(ThreadLocal<?> key, Object value) { Entry[] tab = table; int len = tab.length; // 计算出key在集合中的索引,index int i = key.threadLocalHashCode & (len-1); //开始遍历整个数组, //取出索引为i的Entry,如果不为空,取出下一个,进行遍历 for (Entry e = tab[i]; e != null; e = tab[i = nextIndex(i, len)]) { ThreadLocal<?> k = e.get(); //如果取出的k和传进来的key一致,则把新的值存起来。 if (k == key) { e.value = value; return; } //直到取出最有一个,k==null则进行存储。 if (k == null) { replaceStaleEntry(key, value, i); return; } } //如果索引i的位置,没有Entry,则把传进来的key和value保存在这个位置。 tab[i] = new Entry(key, value); int sz = ++size; //如果大于阈值了,则进行扩容 if (!cleanSomeSlots(i, sz) && sz >= threshold) rehash(); }
과 유사합니다. 2) 맵이 비어 있으면 데이터를 저장합니다. 그런 다음 ThreadLocalMap을 생성하고 이를 현재 스레드 t에 할당합니다.
void createMap(Thread t, T firstValue) { t.threadLocals = new ThreadLocalMap(this, firstValue); }
ThreadLocalMap(ThreadLocal<?> firstKey, Object firstValue) { //创建一个大小为16的数组 table = new Entry[INITIAL_CAPACITY]; //计算得到的i是数组的角标。可以参考hashMap源码分析 int i = firstKey.threadLocalHashCode & (INITIAL_CAPACITY - 1); //赋值,保存数据 table[i] = new Entry(firstKey, firstValue); size = 1; //扩容的阈值 setThreshold(INITIAL_CAPACITY); }
3. ThreadLocal이 어떻게 가치를 얻는지 살펴보겠습니다.
또한 현재 스레드 t를 먼저 가져온 다음 t를 통해 멤버 변수 ThreadLocalMap을 가져옵니다.
public T get() { Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t); //如果map不为空,则从map中取值 if (map != null) { ThreadLocalMap.Entry e = map.getEntry(this); if (e != null) { @SuppressWarnings("unchecked") T result = (T)e.value; return result; } } //如果map为空 return setInitialValue(); }
1) 맵이 비어 있지 않으면 맵에서 값을 가져옵니다.
//如果map不为空 private Entry getEntry(ThreadLocal<?> key) { //拿到key对应的索引 int i = key.threadLocalHashCode & (table.length - 1); //从数组中拿到Entry Entry e = table[i]; if (e != null && e.get() == key){如果key一样直接返回 return e; }else{//如果不一致则开始遍历 return getEntryAfterMiss(key, i, e); } }
private Entry getEntryAfterMiss(ThreadLocal<?> key, int i, Entry e) { Entry[] tab = table; int len = tab.length; while (e != null) { ThreadLocal<?> k = e.get(); if (k == key) return e; if (k == null) expungeStaleEntry(i); else i = nextIndex(i, len); e = tab[i]; } return null; }
2) 획득한 맵이 비어 있으면 이때 초기화해야 합니다
//如果map为空,则调用这个方法,initialValue由用户去实现。 private T setInitialValue() { T value = initialValue(); Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t); if (map != null) map.set(this, value); else createMap(t, value); return value; }
//下面是Choreographer中的例子: private static final ThreadLocal<Choreographer> sThreadInstance = new ThreadLocal<Choreographer>() { @Override protected Choreographer initialValue() { Looper looper = Looper.myLooper(); if (looper == null) { throw new IllegalStateException("The current thread must have a looper!"); } Choreographer choreographer = new Choreographer(looper, VSYNC_SOURCE_APP); if (looper == Looper.getMainLooper()) { mMainInstance = choreographer; } return choreographer; } };
ThreadLocalMap은 ThreadLocal 데이터를 저장하는 데 사용됩니다. 이 ThreadLocalMap 객체는
을 통해 현재 스레드에서 획득되고 현재 스레드에서 획득됩니다. ThreadLocalMap의 설계는 서로 다른 스레드 간의 데이터 격리를 실현하고 해당 스레드의 데이터만 저장합니다.
ThreadLocal 객체 자체는 ThreadLocalMap에 데이터를 저장하는 키로 사용됩니다. map.set(this, value);
ThreadLocalMap에 더 많은 데이터를 저장하려면 여러 개체를 만들어야 합니다.
위 내용은 Java 스레드 변수 ThreadLocal 소스 코드 분석의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
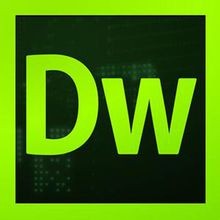
드림위버 CS6
시각적 웹 개발 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
