org.springframework.util.CollectionUtils
컬렉션 판단
boolean hasUniqueObject(Collection collection)
소스 코드 주석에서 List/Set의 각 요소가 고유한지, 즉 컬렉션이 존재하는지 여부를 확인하는 데 사용됩니다. 목록/집합에 고유 요소가 없습니다. 중복된 요소가 있습니다. 하지만 여기서는 이 방법을 사용하지 말라고 말씀드리고 싶습니다. 이 방법에는 버그가 있기 때문입니다. 왜일까요? 다음은 Spring-core-5.2.13.RELEASE.jar의 소스 코드입니다. 12행을 보면 두 객체가 동일한지 비교하는 데 !=가 사용된다는 것을 알 수 있습니다. "=="와 "같음"의 차이점을 기억하시나요? "==" 연산자는 두 변수의 값이 같은지 비교하는 데 특별히 사용되며, equals() 메서드는 두 독립 개체의 내용이 같은지 비교하는 데 사용됩니다. 따라서 컬렉션의 요소가 숫자 값인 경우 "=="를 사용하여 비교할 수 있습니다. 일반 참조 개체인 경우 올바른 결과를 얻을 수 없습니다.
public static boolean hasUniqueObject(Collection<?> collection) { if (isEmpty(collection)) { return false; } boolean hasCandidate = false; Object candidate = null; for (Object elem : collection) { if (!hasCandidate) { hasCandidate = true; candidate = elem; } else if (candidate != elem) { return false; } } return true; }
boolean containInstance(컬렉션 컬렉션, 객체 요소)
소스 코드의 주석으로 판단하면 컬렉션에 객체가 포함되어 있는지 확인하는 데 사용됩니다. 이 방법은 이전 방법과 동일한 문제가 있으므로 권장하지 않습니다. 소스코드 4번째 줄을 보면 여전히 "=="가 사용되고 있습니다.
public static boolean containsInstance(@Nullable Collection<?> collection, Object element) { if (collection != null) { for (Object candidate : collection) { if (candidate == element) { return true; } } } return false; }
boolean isEmpty(컬렉션 컬렉션)
이 메서드는 검증되었으며 안심하고 사용할 수 있습니다. 이는 List/Set이 비어 있는지 확인하는 데 사용됩니다.
@Test public void test1(){ Collection<String> list=new ArrayList<>(); boolean empty = CollectionUtils.isEmpty(list); Assert.isTrue(empty, "集合list不为空"); System.out.println("集合list增加一元素"); list.add("happy"); boolean empty2 = CollectionUtils.isEmpty(list); Assert.isTrue(empty2, "集合list不为空"); }
boolean isEmpty(Map map)
지도가 비어 있는지 확인합니다.
@Test public void test2(){ Map<String,String> map = new HashMap<>(); boolean empty = CollectionUtils.isEmpty(map); Assert.isTrue(empty, "map不为空"); System.out.println("map中增加元素"); map.put("name", "jack"); boolean empty2 = CollectionUtils.isEmpty(map); Assert.isTrue(empty2, "map不为空"); }
boolean containAny(컬렉션 소스, 컬렉션 후보)
소스 코드의 주석을 통해 컬렉션 소스에 다른 컬렉션 후보의 요소가 포함되어 있는지, 즉 컬렉션 후보의 요소가 다음과 같은지 여부를 확인하는 데 사용됩니다. 컬렉션 소스에 완전히 포함됩니다.
소스 코드에서 이 메서드의 요소 간 비교는 "equals" 메서드를 사용하며 컬렉션에 있는 개체의 equals 메서드가 호출됩니다. 따라서 올바른 결과를 얻으려면 이 메서드를 사용하는 것이 전제입니다. 비교된 객체는 중요해야 합니다. hashCode() 및 eauals() 메서드를 작성하세요.
@Test public void test4(){ Employee lisi = new Employee("lisi"); Employee zhangsan = new Employee("zhangsan"); Employee wangwu = new Employee("wangwu"); List<Employee > list=new ArrayList<>(); list.add(zhangsan); list.add(lisi); List<Employee> list2=new ArrayList<>(); list2.add(wangwu); //这里可以用是因为比较的时候调用的是equals方法 boolean b = CollectionUtils.containsAny(list, list2); Assert.isTrue(b, "list1没有包含有list2中任意一个元素"); }
Collection 연산
void mergeArrayIntoCollection(Object array, Collection collection)
배열 배열의 모든 요소를 List/Set에 추가합니다.
@Test public void test6(){ List<Employee > list=new ArrayList<>(); Employee lisi = new Employee("lisi"); list.add(lisi); Employee zhangsan = new Employee("zhangsan"); Employee[] employees={zhangsan}; CollectionUtils.mergeArrayIntoCollection(employees, list); Assert.isTrue(list.size()==2, "把数据中的元素合并到list失败了"); }
void mergePropertiesIntoMap(Properties props, Map map)
Properties의 모든 키-값 쌍을 Map에 추가하세요.
@Test public void test7(){ Properties properties = new Properties(); properties.setProperty("name", "zhangsan"); Map<String,String > map = new HashMap<>(); CollectionUtils.mergePropertiesIntoMap(properties, map); Assert.isTrue(map.get("name").equals("zhangsan"), "把properties中的元素合并到map中失败了"); } @Test public void test7(){ Properties properties = new Properties(); properties.setProperty("name", "zhangsan"); Map<String,String > map = new HashMap<>(); CollectionUtils.mergePropertiesIntoMap(properties, map); Assert.isTrue(map.get("name").equals("zhangsan"), "把properties中的元素合并到map中失败了"); }
T lastElement(List list)
List의 마지막 요소를 반환합니다.
@Test public void test9(){ Employee lisi = new Employee("lisi"); Employee zhangsan = new Employee("zhangsan"); List<Employee > list=new ArrayList<>(); list.add(zhangsan); list.add(lisi); Employee employee = CollectionUtils.firstElement(list); Assert.isTrue(employee.equals(zhangsan), "获取集合第一个元素失败了"); }
T firstElement(목록 목록)
컬렉션의 첫 번째 요소를 반환합니다.
@Test public void test10(){ Employee zhangsan = new Employee("zhangsan"); Employee[] employees={zhangsan}; List list = CollectionUtils.arrayToList(employees); Assert.isTrue(list.size()==1, "把数据转换成集合失败了"); }
List arrayToList(Object source)
배열을 컬렉션으로 변환합니다.
아아아아위 내용은 Springboot의 내장 도구 클래스 CollectionUtils를 사용하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전
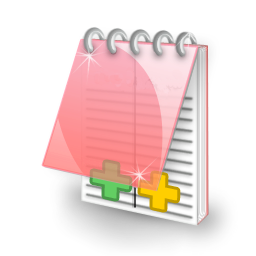
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
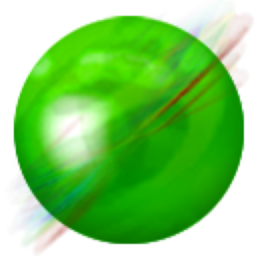
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
