es6에서는 콜백 함수를 통해 배열에서 조건을 충족하는 첫 번째 요소의 값을 찾는 데 find()가 사용되며 구문은 "array.find(function(...),thisValue)"입니다. find()는 배열의 각 요소에 대해 함수 실행을 호출합니다. 조건을 테스트할 때 배열의 요소가 true를 반환하면 find()는 조건을 충족하는 요소를 반환하고 이후 값에 대해서는 실행 함수가 호출되지 않습니다. ; 일치하는 요소가 없으면 정의되지 않은 값이 반환됩니다.
이 튜토리얼의 운영 환경: Windows 7 시스템, ECMAScript 버전 6, Dell G3 컴퓨터.
es6 find() 소개
find() 메서드는 테스트를 통과한 배열의 첫 번째 요소 값을 반환합니다(함수 내에서 판단).
find() 메서드는 배열의 각 요소에 대해 한 번씩 함수 실행을 호출합니다.
조건을 테스트할 때 배열의 요소가 true를 반환하면 find()는 조건을 충족하는 요소와 후속 값을 반환합니다. will not 그런 다음 실행 함수를 호출합니다.
일치하는 요소가 없으면 정의되지 않은 값을 반환합니다.
구문:
array.find(function(currentValue, index, arr),thisValue)
Parameters | Description |
---|---|
function(currentValue, index, arr) | 필수입니다. 배열의 각 요소에 대해 실행되어야 하는 함수입니다. 함수 매개변수: 매개변수 설명 currentValue가 필요합니다. 현재 요소 인덱스는 선택 사항입니다. 현재 요소의 인덱스 값 arr은 선택 사항입니다. 현재 요소가 속한 배열 객체 |
thisValue | 선택 사항입니다. 함수에 전달되는 값은 일반적으로 "this" 값을 사용합니다. 이 매개변수가 비어 있으면 "정의되지 않음"이 "this" 값으로 전달됩니다 |
返回值:返回符合测试条件的第一个数组元素值,如果没有符合条件的则返回 undefined
。
注意:
find() 对于空数组,函数是不会执行的。
find() 并没有改变数组的原始值。
基本使用
Array.prototype.find
返回第一个满足条件的数组元素
const arr = [1, 2, 3, 4, 5]; const item = arr.find(function (item) { return item > 3; }); console.log(item);//4
如果没有一个元素满足条件 返回undefined
const arr = [1, 2, 3, 4, 5]; const item = arr.find(function (item) { return item > 5; }); console.log(item); //undefined
返回的元素和数组对应下标的元素是同一个引用
const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find((item) => item.name === '李四'); console.log(item);
回调函数的返回值是boolean 第一个返回true的对应数组元素作为find的返回值
const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find(function (item) { return item.id > 1; }); console.log(item);
回调的参数
当前遍历的元素 当前遍历出的元素对应的下标 当前的数组
const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find(function (item, index, arr) { console.log(item, index, arr); });
find的第二个参数
更改回调函数内部的this指向
const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find( function (item, index, arr) { console.log(item, index, arr); console.log(this); }, { a: 1 } );
如果没有第二个参数
非严格模式下 this -> window
const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find(function (item, index, arr) { console.log(item, index, arr); console.log(this); });
在严格模式下
不传入第二个参数 this为undefined 与严格模式规定相同
'use strict'; const arr = [ { id: 1, name: '张三', }, { id: 2, name: '李四', }, { id: 3, name: '王五', }, ]; const item = arr.find(function (item, index, arr) { console.log(item, index, arr); console.log(this); });
稀疏数组find
find会遍历稀疏数组的空隙 empty
具体遍历出的值 由undefined占位
const arr = Array(5); arr[0] = 1; arr[2] = 3; arr[4] = 5; const item = arr.find(function (item) { console.log(item); return false; });
而ES5数组扩展方法forEach,map,filter,reduce,reduceRight,every,some 只会遍历有值的数组
find的遍历效率是低于ES5数组扩展方法的
find不会更改数组
虽然新增了元素 但是find会在第一次执行回调函数的时候 拿到这个数组最初的索引范围
const arr = [1, 2, 3, 4, 5]; const item = arr.find(function (item) { arr.push(6); console.log(item); }); console.log(arr);
const arr = [1, 2, 3, 4, 5]; const item = arr.find(function (item) { arr.splice(1, 1); console.log(item); }); console.log(arr);
splice 删除对应项 该项位置不保留 在数据最后补上undefined
const arr = [1, 2, 3, , , , 7, 8, 9]; arr.find(function (item, index) { if (index === 0) { arr.splice(1, 1); } console.log(item); });
delete
删除该项的值 并填入undefined
const arr = [1, 2, 3, , , , 7, 8, 9]; arr.find(function (item, index) { if (index === 0) { delete arr[2]; } console.log(item); });
pop
删除该项的值 并填入undefined
const arr = [1, 2, 3, , , , 7, 8, 9]; arr.find(function (item, index) { if (index === 0) { arr.pop(); } console.log(item); });
创建myFind
Array.prototype.myFind = function (cb) { if (this === null) { throw new TypeError('"this" is null'); } if (typeof cb !== 'function') { throw new TypeError('Callback must be a function type'); } var obj = Object(this), len = obj.length >>> 0, arg2 = arguments[1], step = 0; while (step <p>【相关推荐:<a href="https://www.php.cn/course/list/17.html" target="_blank" textvalue="javascript视频教程">javascript视频教程</a>、<a href="https://www.php.cn/course.html" target="_blank" textvalue="编程视频">编程视频</a>】</p>
위 내용은 es6에서 find()를 사용하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

ID 선택기를 사용하는 것은 본질적으로 CSS에서 나쁘지 않지만주의해서 사용해야합니다. 1) ID 선택기는 고유 한 요소 또는 JavaScript 후크에 적합합니다. 2) 일반적인 스타일의 경우 클래스 선택기가보다 유연하고 유지 관리 가능하므로 사용해야합니다. ID 및 클래스 사용의 균형을 유지함으로써보다 강력하고 효율적인 CSS 아키텍처를 구현할 수 있습니다.

HTML5'SGOALSIN2024FOCUSONERFINEMENTANDENDEND 및 최적화, NOTNEWFEATURES.1) 최적화 된 렌더링을 향상시킵니다

html5aimedtoimprovewebdevelopmentinfourkeyareas : 1) Multimediasupport, 2) Semantictructure, 3) Formcapabilities, 및 4) OfflineandStorageOptions.1) Html5intrudceDandlements, Simplifying MediaembeddingandenUsereXperxpercepence.2) NewSmanticallementalmentalmentementlementmentmentmentmentmentmentmentmentmentmentmentmentmentmentmentmentmentmentalments

idsshouldBeusedforjavaScriptThooks, whileclassesarebetterforstyling.1) 1) USECLASSESTYLINGTOWALLOWFOREASIEREASEANDAVOIDSPECIFICITISUES.2) USEDSFORJAVASCRIPTHOOKSTOUNIQUELIDINTIFYELEMENTS.3) 피할 수있는 TeepSelectorsSimpleApperforformance.4

classselectorsareversatiledreusable, whileDselectorsareUniqueAndspecific.1) USECLASSSELECTORS (DENOTEDBY.) ForstylingMultipleElementSwithSharedCharacteristics

idsareUniqueIndifiersforsinglelemes, whileclassesstylemultipleements.1) useidsforuniqueElements 및 Javascripthooks.2) useclassessforusable, flexiblestylingacrossmultipleelements.

클래스 전용 선택기를 사용하면 코드 재사용 성과 유지 관리가 향상 될 수 있지만 클래스 이름 및 우선 순위를 관리해야합니다. 1. 재사용 성과 유연성 향상, 2. 여러 클래스를 결합하여 복잡한 스타일을 만들고, 3. 긴 클래스 이름과 우선 순위로 이어질 수 있습니다.

ID 및 클래스 선택기는 각각 고유 및 멀티 요소 스타일 설정에 CSS에서 사용됩니다. 1. ID 선택기 (#)는 특정 탐색 메뉴와 같은 단일 요소에 적합합니다. 2. 클래스 선택기 (.)는 통합 버튼 스타일과 같은 여러 요소에 사용됩니다. ID는주의해서 사용하고 과도한 특이성을 피하며 스타일 재사용 성과 유연성을 향상시키기 위해 클래스를 우선시해야합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
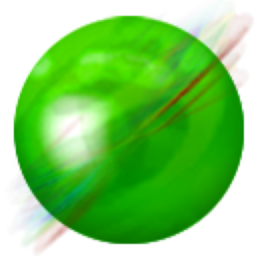
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
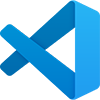
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.