실제 개발에서는 종종 다음과 같은 요구 사항이 있습니다. 특정 기능 모듈이나 작업이 동일한 기간 내에 주기적으로 실행되어야 합니다. 여기에는 타이머라는 개념이 있습니다. 구체적으로 타이머를 어떻게 구현해야 할까요? 타이머에는 스레드 실행을 제어하고 시스템 소비를 줄이는 등 매우 실용적인 기능이 많이 있습니다. 이제 Python3에서 타이밍 함수 구현을 연습해 보겠습니다.
예를 들어 Python을 사용하여 크롤러 시스템을 개발할 때 스레드 서비스를 구현하여 백그라운드에서 데이터 캡처 상태를 모니터링하려면 타이머가 도움이 될 수 있습니다. .
[동영상 추천: Python3 동영상 튜토리얼]
[수동 권장사항: Python 중국어 매뉴얼]
Python 문서를 통해 타이밍 기능을 구현하는 threading.Timer()를 찾을 수 있습니다:
간단한 구현 코드:
import threading def func1(a): #Do something print('Do something') a+=1 print(a) print('当前线程数为{}'.format(threading.activeCount())) if a>5: return t=threading.Timer(5,func1,(a,)) t.start()
렌더링:
파이썬을 사용하여 세 가지 타이밍 작업 실행 방법을 구현할 수 있습니다.
1 타이밍 작업 코드
#!/user/bin/env python #定时执行任务命令 import time,os,sched schedule = sched.scheduler(time.time,time.sleep) def perform_command(cmd,inc): os.system(cmd) print('task') def timming_exe(cmd,inc=60): schedule.enter(inc,0,perform_command,(cmd,inc)) schedule.run() print('show time after 2 seconds:') timming_exe('echo %time%',2)
2. 주기적 작업 실행
#!/user/bin/env python import time,os,sched schedule = sched.scheduler(time.time,time.sleep) def perform_command(cmd,inc): #在inc秒后再次运行自己,即周期运行 schedule.enter(inc, 0, perform_command, (cmd, inc)) os.system(cmd) def timming_exe(cmd,inc=60): schedule.enter(inc,0,perform_command,(cmd,inc)) schedule.run()#持续运行,直到计划时间队列变成空为止 print('show time after 2 seconds:') timming_exe('echo %time%',2)
3. 명령어 실행
#!/user/bin/env python import time,os def re_exe(cmd,inc = 60): while True: os.system(cmd) time.sleep(inc) re_exe("echo %time%",5)
요약: Python에서 타이머를 구현하는 방법은 스케줄 및 스레딩 구현이며 구체적인 사용법은 실제 상황에 따라 유연하게 사용해야 합니다.
가장 일반적으로 사용되는 두 가지 모듈: 스레딩, Sched
threading 모듈 사용법:
import threading ,time from time import sleep, ctime class Timer(threading.Thread): """ very simple but useless timer. """ def __init__(self, seconds): self.runTime = seconds threading.Thread.__init__(self) def run(self): time.sleep(self.runTime) print ("Buzzzz!! Time's up!") class CountDownTimer(Timer): """ a timer that can counts down the seconds. """ def run(self): counter = self.runTime for sec in range(self.runTime): print (counter) time.sleep(1.0) counter -= 1 print ("Done") class CountDownExec(CountDownTimer): """ a timer that execute an action at the end of the timer run. """ def __init__(self, seconds, action, args=[]): self.args = args self.action = action CountDownTimer.__init__(self, seconds) def run(self): CountDownTimer.run(self) self.action(self.args) def myAction(args=[]): print ("Performing my action with args:") print (args) if __name__ == "__main__": t = CountDownExec(3, myAction, ["hello", "world"]) t.start() print("2333")
Sched 모듈 사용법:
''' 使用sched模块实现的timer,sched模块不是循环的,一次调度被执行后就Over了,如果想再执行, 可以使用while循环的方式不停的调用该方法 ''' import time, sched #被调度触发的函数 def event_func(msg): print("Current Time:", time.strftime("%y-%m-%d %H:%M:%S"), 'msg:', msg) def run_function(): #初始化sched模块的scheduler类 s = sched.scheduler(time.time, time.sleep) #设置一个调度,因为time.sleep()的时间是一秒,所以timer的间隔时间就是sleep的时间,加上enter的第一个参数 s.enter(0, 2, event_func, ("Timer event.",)) s.run() def timer1(): while True: #sched模块不是循环的,一次调度被执行后就Over了,如果想再执行,可以使用while循环的方式不停的调用该方法 time.sleep(1) run_function() if __name__ == "__main__": timer1()
위 내용은 Python3을 통해 작업의 타임 루프 실행 구현의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
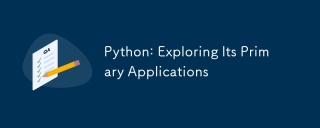
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
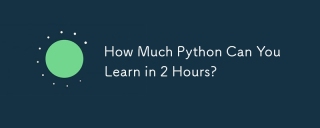
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
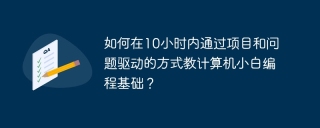
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
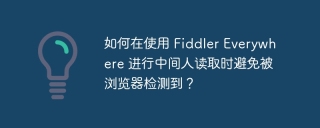
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...
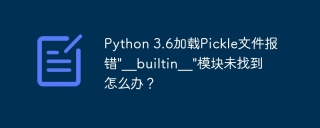
Python 3.6에 피클 파일로드 3.6 환경 보고서 오류 : modulenotfounderror : nomodulename ...
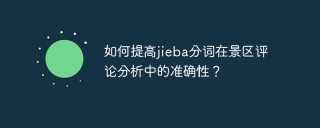
경치 좋은 스팟 댓글 분석에서 Jieba Word 세분화 문제를 해결하는 방법은 무엇입니까? 경치가 좋은 스팟 댓글 및 분석을 수행 할 때 종종 Jieba Word 세분화 도구를 사용하여 텍스트를 처리합니다 ...

정규 표현식을 사용하여 첫 번째 닫힌 태그와 정지와 일치하는 방법은 무엇입니까? HTML 또는 기타 마크 업 언어를 다룰 때는 정규 표현식이 종종 필요합니다.
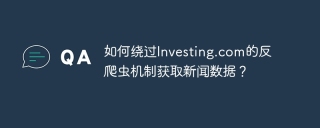
Investing.com의 크롤링 전략 이해 많은 사람들이 종종 Investing.com (https://cn.investing.com/news/latest-news)에서 뉴스 데이터를 크롤링하려고합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
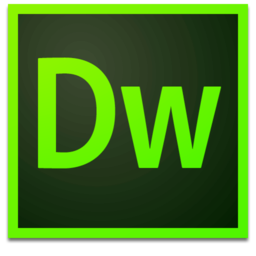
Dreamweaver Mac版
시각적 웹 개발 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

뜨거운 주제



