메서드는 객체 속성에 할당된 함수입니다. 객체의 속성에 함수가 정의되면 이를 함수라기 보다는 객체의 메소드라고 부릅니다. 이 글에서는 자바스크립트에서 메소드를 사용하는 방법을 소개합니다.
속성은 이름(속성 이름)이 추가되는 미리 설정된 특정 정보(값)입니다. 해당 속성에서는 함수를 구체적으로 "메서드"라고 부릅니다.
구체적인 예를 살펴보겠습니다
아래 프로그램에서는 신호등 파란색, 노란색, 빨간색을 기반으로 객체를 생성하고 이를 Traffic_light라는 변수에 넣습니다.
<!DOCTYPE html> <html> <head> <meta charset = "utf-8"> <title>JavaScript에서 메소드를 사용하는 방법에서 메소드를 사용하는 방법에서 메소드를 사용하는 방법</title> </head> <body> <script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop" } </script> </body> </html>
여기에 current라는 속성을 추가했습니다. 현재 신호등의 색상을 포함합니다.
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " " } </script>
신호등의 색상을 변경하는 함수는change_light로 정의되어 있습니다. 그런 다음,change_light라는 메서드(함수)를 호출하여 이 전류 값을 변경합니다.
먼저 Change_light 함수를 정의합니다
위 프로그램에서 함수를 계속 사용하면서 임시로 함수를 Change_light로 정의하겠습니다.
change_light를 호출하는 다음 신호를 고려하여 당시의 현재 상태에 따라 호출될 다음 속성의 동작을 결정해 보겠습니다.
change_light를 4가지 모드로 설정하려면 스위치 문을 사용하세요.
현재에 포함된 속성이 파란색이면 다음 속성은 노란색으로 변경됩니다.
현재에 포함된 속성이 노란색이면 다음 속성은 빨간색으로 변경됩니다.
현재에 포함된 속성이 빨간색이면 다음 속성은 파란색으로 변경됩니다.
기본값은 파란색입니다.
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " " } function change_light(){ switch(traffic_light.current){ case traffic_light.blue: traffic_light.current = traffic_light.yellow; break; case traffic_light.yellow: traffic_light.current = traffic_light.red; break; case traffic_light.red: traffic_light.current = traffic_light.blue; break; default: traffic_light.current = traffic_light.blue; break } } </script>
다음으로 console.log에서 current를 호출하여 결과를 확인합니다
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " " } function change_light(){ switch(traffic_light.current){ case traffic_light.blue: traffic_light.current = traffic_light.yellow; break; case traffic_light.yellow: traffic_light.current = traffic_light.red; break; case traffic_light.red: traffic_light.current = traffic_light.blue; break; default: traffic_light.current = traffic_light.blue; break } } change_light(); console.log(traffic_light.current); </script>
기본 속성이 blue로 설정되어 있기 때문에 blue의 값이 go로 출력됩니다.
console.log를 사용하여 세 번의 호출을 반복합니다...
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " " } function change_light(){ switch(traffic_light.current){ case traffic_light.blue: traffic_light.current = traffic_light.yellow; break; case traffic_light.yellow: traffic_light.current = traffic_light.red; break; case traffic_light.red: traffic_light.current = traffic_light.blue; break; default: traffic_light.current = traffic_light.blue; break } } change_light(); console.log(traffic_light.current); change_light(); console.log(traffic_light.current); change_light(); console.log(traffic_light.current); change_light(); console.log(traffic_light.current); </script>
현재 변경 사항은 파란색->노란색->빨간색->파란색
값 출력은 go->slow down-입니다. >stop->go
마지막으로 change_light를 Traffic_light
의 방법으로 사용하는 방법을 살펴보겠습니다. 해야 할 일은 속성 이름을 current: "" 뒤에 설정하고 The를 사용하는 것입니다. 다음 함수는 이를 구분합니다(예: ":"를 사용하여 구분). (이때 연속된 함수명인change_light가 중복되어 있어서 제거가 가능합니다.)
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " ", change_light:function(){ switch(traffic_light.current){ case traffic_light.blue: traffic_light.current = traffic_light.yellow; break; case traffic_light.yellow: traffic_light.current = traffic_light.red; break; case traffic_light.red: traffic_light.current = traffic_light.blue; break; default: traffic_light.current = traffic_light.blue; break } } } </script>
이제, teaffic_light 객체는change_light라는 메소드를 가지게 됩니다.
consoe.log에서도 같은 방식으로 호출합니다. 이 시간을 네 번 반복합니다.
개체의 각 속성을 호출할 때 변수 이름 뒤에 "."을 입력하면 속성 값을 호출할 수 있습니다. 그래서 Traffic_light 변수에 포함된 객체에서 메소드(속성)를 호출하려고 하면 이렇게 됩니다.
<script> var traffic_light = { blue: "go", yellow: "slow down", red: "stop", current: " ", change_light:function() { switch(traffic_light.current){ case traffic_light.blue: traffic_light.current = traffic_light.yellow; break; case traffic_light.yellow: traffic_light.current = traffic_light.red; break; case traffic_light.red: traffic_light.current = traffic_light.blue; break; default: traffic_light.current = traffic_light.blue; break } } } traffic_light.change_light(); console.log(traffic_light.current); traffic_light.change_light(); console.log(traffic_light.current); traffic_light.change_light(); console.log(traffic_light.current); traffic_light.change_light(); console.log(traffic_light.current); </script>
실행 결과는 다음과 같습니다.
change_light 함수는 단지 Traffic_light 객체의 메서드이므로 결과는 변경되지 않았습니다.
위 내용은 JavaScript에서 메소드를 사용하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
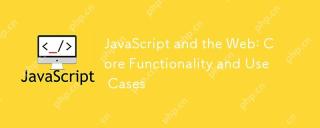
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
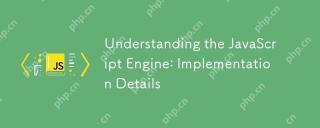
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.

Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
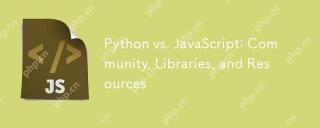
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
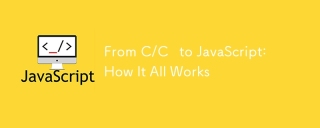
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
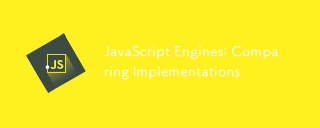
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
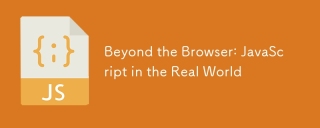
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
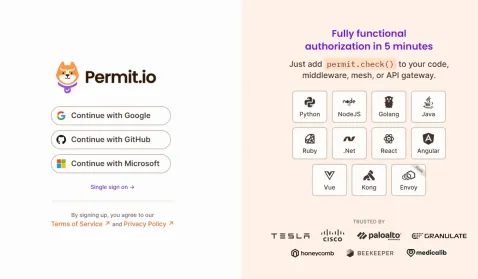
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
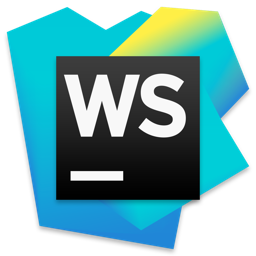
WebStorm Mac 버전
유용한 JavaScript 개발 도구
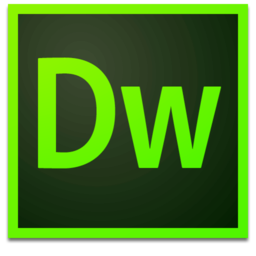
Dreamweaver Mac版
시각적 웹 개발 도구
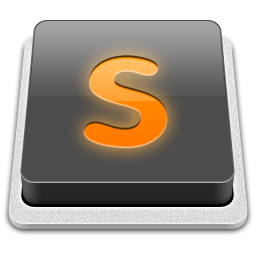
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
