이 글은 주로 YiiFramework의 입문 지식 포인트를 소개하고 있으며, YiiFramework를 만드는 구체적인 단계와 관련 주의 사항을 그림과 텍스트 형태로 요약하고 분석하고 있습니다. 필요한 친구들이 참고할 수 있습니다
이 글은 입문을 요약한 것입니다. YiiFramework의 지식 포인트. 참조용으로 모든 사람과 공유하세요. 세부 사항은 다음과 같습니다.
Yii 애플리케이션 뼈대 만들기
web은 웹사이트의 루트 디렉터리입니다.
yiic webapp /web/demo
모델을 만들 때 주의해야 합니다. 그리고 GII를 통한 CURD
1. Model Generator 연산
테이블 접두사가 있더라도 테이블 이름에는 테이블 접두사를 포함하여 테이블의 전체 이름을 입력해야 합니다. 아래와 같이:
2. Crud Generator 작동
이 인터페이스에서 모델 클래스에 모델 이름을 입력합니다. 첫 글자를 대문자로 표기하세요. 모델을 생성할 때 proctected/models 디렉터리에서 모델 생성기가 생성한 파일 이름을 참조할 수도 있습니다. 아래와 같이
모델 생성기에서 news, newstype 및 statustype의 세 테이블에 대해 CURD 컨트롤러를 생성하는 경우 모델 클래스에 News, newsType 및 StatusType을 입력합니다. 생성된 파일명과 대소문자가 동일합니다. NEWS나 News로 작성하는 경우에는 허용되지 않습니다.
모듈 생성에 대한 참고 사항
GII를 통해 모듈을 생성합니다. 모듈 ID는 일반적으로 소문자입니다. 어떤 경우든 여기에 입력된 ID에 따라 main.php 구성 파일의 구성이 결정됩니다. 다음과 같습니다:
'modules'=>array( 'admin'=>array(//这行的admin为Module ID。与创建Module时填写的Module ID大写写一致 'class'=>'application.modules.admin.AdminModule',//这里的admin在windows os中大小写无所谓,但最好与实际目录一致。 ), ),
routing
system은 yii 프레임워크의 프레임워크 디렉터리를 나타냅니다.
application은 생성된 애플리케이션(예: d:wwwrootblog) 아래의 보호된 디렉터리를 나타냅니다.
application.modules.Admin.AdminModule
은 응용 프로그램 디렉터리(예: d:wwwrootblogprotected) 아래의 모듈 디렉터리 아래 Admin 디렉터리에 있는 AdminModules.php 파일을 나타냅니다(실제로는 파일의 클래스 이름을 가리킴)
system .db.*
는 YII 프레임워크 아래 프레임워크 디렉터리 아래의 db 디렉터리에 있는 모든 파일을 나타냅니다.
컨트롤러의 AccessRules 설명
/** * Specifies the access control rules. * This method is used by the 'accessControl' filter. * @return array access control rules */ public function accessRules() { return array( array('allow', // allow all users to perform 'index' and 'view' actions 'actions'=>array('index','view'),//表示任意用户可访问index、view方法 'users'=>array('*'),//表示任意用户 ), array('allow', // allow authenticated user to perform 'create' and 'update' actions 'actions'=>array('create','update'),//表示只有认证用户才可操作create、update方法 'users'=>array('@'),//表示认证用户 ), array('allow', // allow admin user to perform 'admin' and 'delete' actions 'actions'=>array('admin','delete'),//表示只有用户admin才能访问admin、delete方法 'users'=>array('admin'),//表示指定用户,这里指用户:admin ), array('deny', // deny all users 'users'=>array('*'), ), ); }
위의 코드 주석을 참조하세요.
user: 사용자 세션 정보를 나타냅니다. 자세한 내용은 API: CWebUser를 참조하세요.
CWebUser는 웹 애플리케이션의 영구 상태를 나타냅니다.
CWebUser는 ID 사용자가 있는 애플리케이션 구성 요소입니다. 따라서 사용자 상태는 Yii::app()->user
public function beforeSave() { if(parent::beforeSave()) { if($this->isNewRecord) { $this->password=md5($this->password); $this->create_user_id=Yii::app()->user->id;//一开始这样写,User::model()->user->id;(错误) //$this->user->id;(错误) $this->create_time=date('Y-m-d H:i:s'); } else { $this->update_user_id=Yii::app()->user->id; $this->update_time=date('Y-m-d H:i:s'); } return true; } else { return false; } }
getter 메소드 또는/및 setter 메소드
<?php /** * UserIdentity represents the data needed to identity a user. * It contains the authentication method that checks if the provided * data can identity the user. */ class UserIdentity extends CUserIdentity { /** * Authenticates a user. * The example implementation makes sure if the username and password * are both 'demo'. * In practical applications, this should be changed to authenticate * against some persistent user identity storage (e.g. database). * @return boolean whether authentication succeeds. */ private $_id; public function authenticate() { $username=strtolower($this->username); $user=User::model()->find('LOWER(username)=?',array($username)); if($user===null) { $this->errorCode=self::ERROR_USERNAME_INVALID; } else { //if(!User::model()->validatePassword($this->password)) if(!$user->validatePassword($this->password)) { $this->errorCode=self::ERROR_PASSWORD_INVALID; } else { $this->_id=$user->id; $this->username=$user->username; $this->errorCode=self::ERROR_NONE; } } return $this->errorCode===self::ERROR_NONE; } public function getId() { return $this->_id; } }
model/User.php
public function beforeSave() { if(parent::beforeSave()) { if($this->isNewRecord) { $this->password=md5($this->password); $this->create_user_id=Yii::app()->user->id;//====主要为此句。得到登陆帐号的ID $this->create_time=date('Y-m-d H:i:s'); } else { $this->update_user_id=Yii::app()->user->id; $this->update_time=date('Y-m-d H:i:s'); } return true; } else { return false; } }
를 통해 어디서나 액세스할 수 있습니다. 추가 관련 항목:
/* 由于CComponent是post最顶级父类,所以添加getUrl方法。。。。如下说明: CComponent 是所有组件类的基类。 CComponent 实现了定义、使用属性和事件的协议。 属性是通过getter方法或/和setter方法定义。访问属性就像访问普通的对象变量。读取或写入属性将调用应相的getter或setter方法 例如: $a=$component->text; // equivalent to $a=$component->getText(); $component->text='abc'; // equivalent to $component->setText('abc'); getter和setter方法的格式如下 // getter, defines a readable property 'text' public function getText() { ... } // setter, defines a writable property 'text' with $value to be set to the property public function setText($value) { ... } */ public function getUrl() { return Yii::app()->createUrl('post/view',array( 'id'=>$this->id, 'title'=>$this->title, )); }
모델의 규칙 방법
/* * rules方法:指定对模型属性的验证规则 * 模型实例调用validate或save方法时逐一执行 * 验证的必须是用户输入的属性。像id,作者id等通过代码或数据库设定的不用出现在rules中。 */ /** * @return array validation rules for model attributes. */ public function rules() { // NOTE: you should only define rules for those attributes that // will receive user inputs. return array( array('news_title, news_content', 'required'), array('news_title', 'length', 'max'=>128), array('news_content', 'length', 'max'=>8000), array('author_name, type_id, status_id,create_time, update_time, create_user_id, update_user_id', 'safe'), // The following rule is used by search(). // Please remove those attributes that should not be searched. array('id, news_title, news_content, author_name, type_id, status_id, create_time, update_time, create_user_id, update_user_id', 'safe', 'on'=>'search'), ); }
설명:
1 확인 필드는 사용자가 입력한 속성이어야 합니다. 사용자가 입력하지 않은 콘텐츠에는 유효성 검사가 필요하지 않습니다.
2. 데이터베이스의 작업 필드(생성 시간, 업데이트 시간 및 기타 필드 등 시스템에서 생성된 경우라도 - boyLee에서 제공하는 yii_computer 소스 코드에서는 시스템에서 생성된 이러한 속성이 안전하게 보관되지 않습니다. 아래 코드를 참조하세요). 양식에서 제공되지 않은 데이터의 경우 규칙 방법으로 확인되지 않는 한 안전 장치에 추가해야 하며, 그렇지 않으면 데이터베이스에 쓸 수 없습니다.
yii_computer의 News.php 규칙 메소드에 대한 모델
/** * @return array validation rules for model attributes. */ public function rules() { // NOTE: you should only define rules for those attributes that // will receive user inputs. return array( array('news_title, news_content', 'required'), array('news_title', 'length', 'max'=>128, 'encoding'=>'utf-8'), array('news_content', 'length', 'max'=>8000, 'encoding'=>'utf-8'), array('author_name', 'length', 'max'=>10, 'encoding'=>'utf-8'), array('status_id, type_id', 'safe'), // The following rule is used by search(). // Please remove those attributes that should not be searched. array('id, news_title, news_content, author_name, type_id, status_id', 'safe', 'on'=>'search'), ); }
뷰에 동적 콘텐츠를 표시하는 세 가지 방법
1. 뷰 파일의 PHP 코드에서 직접 구현합니다. 예를 들어, 뷰에 현재 시간을 표시하려면:
<?php echo date("Y-m-d H:i:s");?>
2. 컨트롤러에 표시 콘텐츠를 구현하고 render의 두 번째 매개변수를 통해 뷰에 전달합니다.
컨트롤러 메서드에는 다음이 포함됩니다.
$theTime=date("Y-m-d H:i:s"); $this->render('helloWorld',array('time'=>$theTime));
파일 보기:
<?php echo $time;?>
Call render() 메소드의 두 번째 매개변수는 배열(array type)입니다. render() 메소드는 배열의 값을 추출하여 뷰 스크립트에 제공합니다. array는 뷰 스크립트 이름에 제공되는 변수입니다. 이 예에서는 배열의 키가 time이고 값이 $theTime이므로 추출된 변수 이름 $time이 뷰 스크립트에서 사용됩니다. 이는 컨트롤러에서 뷰로 데이터를 전달하는 방법입니다.
3. 뷰와 컨트롤러는 매우 가까운 형제이므로 뷰 파일의 $this는 이 뷰를 렌더링하는 컨트롤러를 나타냅니다. 이전 예제를 수정하고 값이 현재 날짜 및 시간인 로컬 변수 대신 컨트롤러에서 클래스의 공용 속성을 정의합니다. 그런 다음 뷰의 $this를 통해 이 클래스의 속성에 액세스합니다.
보기 이름 지정 규칙
보기 파일 이름 지정은 ActionID와 동일하게 해주세요. 하지만 이는 권장되는 명명 규칙일 뿐이라는 점을 기억하세요. 실제로 뷰 파일 이름은 ActionID와 동일할 필요는 없습니다. 파일 이름을 render()의 첫 번째 매개변수로 전달하기만 하면 됩니다.
DB 관련
$Prerfp = Prerfp::model()->findAll( array( 'limit'=>'5', 'order'=>'releasetime desc' ) );
$model = Finishrfp::model()->findAll( array( 'select' => 'companyname,title,releasetime', 'order'=>'releasetime desc', 'limit' => 10 ) ); foreach($model as $val){ $noticeArr[] = " 在".$val->title."竞标中,".$val->companyname."中标。"; }
$model = Cgnotice::model()->findAll ( array( 'select' => 'status,content,updatetime', 'condition'=> 'status = :status ', 'params' => array(':status'=>0), 'order'=>'updatetime desc', 'limit' => 10 ) ); foreach($model as $val){ $noticeArr[] = $val->content; }
$user=User::model()->find('LOWER(username)=?',array($username));
$noticetype = Dictionary::model()->find(array( 'condition' => '`type` = "noticetype"') );
// 查找postID=10 的那一行 $post=Post::model()->find('postID=:postID', array(':postID'=>10));
$condition을 사용하여 더 복잡한 쿼리 조건을 지정할 수도 있습니다. 문자열을 사용하는 대신 $condition을 CDbCriteria의 인스턴스로 만들 수 있으며 이를 통해 WHERE에 국한되지 않는 조건을 지정할 수 있습니다. 예:
$criteria=new CDbCriteria; $criteria->select='title'; // 只选择'title' 列 $criteria->condition='postID=:postID'; $criteria->params=array(':postID'=>10); $post=Post::model()->find($criteria); // $params 不需要了
CDbCriteria를 쿼리 기준으로 사용하는 경우 $params 매개변수는 위와 마찬가지로 CDbCriteria에 지정할 수 있으므로 더 이상 필요하지 않습니다.
一种替代CDbCriteria 的方法是给find 方法传递一个数组。数组的键和值各自对应标准(criterion)的属性名和值,上面的例子可以重写为如下:
$post=Post::model()->find(array( 'select'=>'title', 'condition'=>'postID=:postID', 'params'=>array(':postID'=>10), ));
其它
1、链接
<span class="tt"><?php echo CHtml::link(Controller::utf8_substr($val->title,0,26),array('prerfp/details','id'=>$val->rfpid),array('target'=>'_blank'));?></a> </span>
具体查找API文档:CHtml的link()方法
<span class="tt"><a target="_blank" title="<?php echo $val->title;?>" href="<?php echo $this->createUrl('prerfp/details',array('id'=>$val->rfpid)) ;?>" ><?php echo Controller::utf8_substr($val->title,0,26); ?></a> </span>
具体请查找API文档:CController的createUrl()方法
以上两个连接效果等同
组件包含
一个示例:
在视图中底部有如下代码:
<?php $this->widget ( 'Notice' ); ?>
打开protected/components下的Notice.php文件,内容如下:
<?php Yii::import('zii.widgets.CPortlet'); class Banner extends CPortlet { protected function renderContent() { $this->render('banner'); } }
渲染的视图banner,是在protected/components/views目录下。
具体查看API,关键字:CPortlet
获取当前host
Yii::app()->request->getServerName(); //and $_SERVER['HTTP_HOST']; $url = 'http://'.Yii::app()->request->getServerName(); $url .= CController::createUrl('user/activateEmail', array('emailActivationKey'=>$activationKey)); echo $url;
关于在发布新闻时添加ckeditor扩展中遇到的情况
$this->widget('application.extensions.editor.CKkceditor',array( "model"=>$model, # Data-Model "attribute"=>'news_content', # Attribute in the Data-Model "height"=>'300px', "width"=>'80%', "filespath"=>Yii::app()->basePath."/../up/", "filesurl"=>Yii::app()->baseUrl."/up/", );
echo Yii::app()->basePath
如果项目目录在:d:\wwwroot\blog目录下。则上面的值为d:\wwwroot\blog\protected。注意路径最后没有返斜杠。
echo Yii::app()->baseUrl;
如果项目目录在:d:\wwwroot\blog目录下。则上面的值为/blog。注意路径最后没有返斜杠。
(d:\wwwroot为网站根目录),注意上面两个区别。一个是basePath,一个是baseUrl
其它(不一定正确)
在一个控制器A对应的A视图中,调用B模型中的方法,采用:B::model()->B模型中的方法名();
前期需要掌握的一些API
CHtml
以上就是本文的全部内容,希望对大家的学习有所帮助,更多相关内容请关注PHP中文网!
相关推荐:
关于Yii
Framework框架获取分类下面的所有子类的方法
위 내용은 YiiFramework 입문 지식 포인트 요약의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
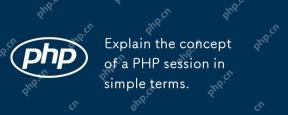
phpsessionstrackuserdataacrossmultiplepagerequestsususingauniqueIdStoredInAcookie.here'showtomanagetheMeftically : 1) STARTASESSIONSTART_START () andSTAREDATAIN $ _SESSION.2) RegenerATERATESSESSIDIDAFTERLOGINWITHSESSION_RATERATERATES (True) TopreventSES
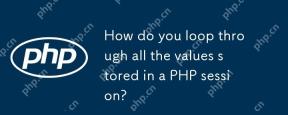
PHP에서 세션 데이터를 통한 반복은 다음 단계를 통해 달성 할 수 있습니다. 1. Session_start ()를 사용하여 세션을 시작하십시오. 2. $ _session 배열의 모든 키 값 쌍을 통해 Foreach 루프를 통과합니다. 3. 복잡한 데이터 구조를 처리 할 때 is_array () 또는 is_object () 함수를 사용하고 print_r ()를 사용하여 자세한 정보를 출력하십시오. 4. Traversal을 최적화 할 때 페이징을 사용하여 한 번에 많은 양의 데이터를 처리하지 않도록 할 수 있습니다. 이를 통해 실제 프로젝트에서 PHP 세션 데이터를보다 효율적으로 관리하고 사용하는 데 도움이됩니다.
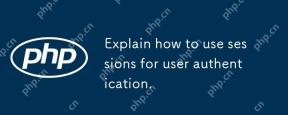
이 세션은 서버 측 상태 관리 메커니즘을 통해 사용자 인증을 인식합니다. 1) 세션 생성 및 고유 ID의 세션 생성, 2) ID는 쿠키를 통해 전달됩니다. 3) ID를 통해 서버 저장 및 세션 데이터에 액세스합니다. 4) 사용자 인증 및 상태 관리가 실현되어 응용 프로그램 보안 및 사용자 경험이 향상됩니다.
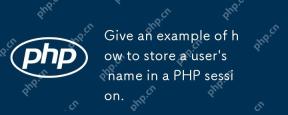
tostoreauser'snameinaphpsession, startSessionstart_start (), wathsignthenameto $ _session [ 'username']. 1) useSentess_start () toinitializethesession.2) assimeuser'snameto $ _session [ 'username']
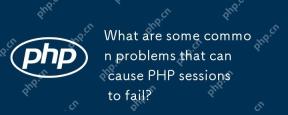
phpsession 실패 이유에는 구성 오류, 쿠키 문제 및 세션 만료가 포함됩니다. 1. 구성 오류 : 올바른 세션을 확인하고 설정합니다. 2. 쿠키 문제 : 쿠키가 올바르게 설정되어 있는지 확인하십시오. 3. 세션 만료 : 세션 시간을 연장하기 위해 세션을 조정합니다 .GC_MAXLIFETIME 값을 조정하십시오.
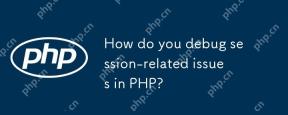
PHP에서 세션 문제를 디버그하는 방법 : 1. 세션이 올바르게 시작되었는지 확인하십시오. 2. 세션 ID의 전달을 확인하십시오. 3. 세션 데이터의 저장 및 읽기를 확인하십시오. 4. 서버 구성을 확인하십시오. 세션 ID 및 데이터를 출력, 세션 파일 컨텐츠보기 등을 통해 세션 관련 문제를 효과적으로 진단하고 해결할 수 있습니다.
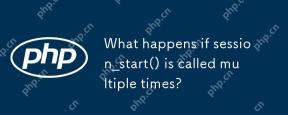
Session_Start ()로 여러 통화를하면 경고 메시지와 가능한 데이터 덮어 쓰기가 발생합니다. 1) PHP는 세션이 시작되었다는 경고를 발행합니다. 2) 세션 데이터의 예상치 못한 덮어 쓰기를 유발할 수 있습니다. 3) Session_status ()를 사용하여 반복 통화를 피하기 위해 세션 상태를 확인하십시오.
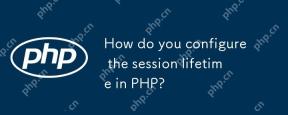
SESSION.GC_MAXLIFETIME 및 SESSION.COOKIE_LIFETIME을 설정하여 PHP에서 세션 수명을 구성 할 수 있습니다. 1) SESSION.GC_MAXLIFETIME 서버 측 세션 데이터의 생존 시간을 제어합니다. 2) 세션 .Cookie_Lifetime 클라이언트 쿠키의 수명주기를 제어합니다. 0으로 설정하면 브라우저가 닫히면 쿠키가 만료됩니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
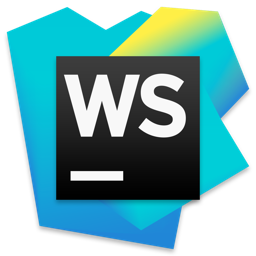
WebStorm Mac 버전
유용한 JavaScript 개발 도구

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
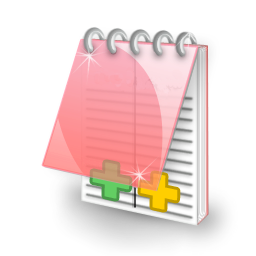
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
