이번에는 webpack 초기화부터 사용까지 안내해드리며, webpack 초기화부터 사용까지의 주의사항은 무엇인지 실제 사례로 살펴보겠습니다.
노드를 설치한 후 html5와 같은 새 디렉터리를 만듭니다. cmd에서 현재 폴더로 전환합니다.
npm init -y
이 명령은 기본 package.json을 생성합니다. 여기에는 초기 설치를 수행할 수 있는 프로젝트의 일부 구성 매개변수가 포함되어 있습니다. 세부 매개변수: https://docs.npmjs.com/files/package.json.
y 매개 변수를 원하지 않으면 명령 상자에서 다양한 매개 변수를 설정하지만 꼭 필요한 것은 아니라고 생각합니다.
2. webpack을 설치합니다
npm install webpack --save-dev
현재 디렉토리에 webpack을 설치합니다. npm install webpack -g webpack을 전역적으로 설치할 수는 있지만 일부 모듈을 찾을 수 없다는 오류가 발생하기 쉬우므로 현재 디렉터리에 설치하는 것이 가장 좋습니다.
3. 디렉토리 구조
webpack은 다양한 리소스를 로드하고 패키징하는 모듈입니다. 따라서 먼저 다음과 같이 디렉터리 구조를 만듭니다.
앱에는 개발 중인 js 파일, 하나의 구성 요소 및 하나의 항목이 포함되어 있습니다. 빌드는 패키지된 파일을 저장하는 데 사용됩니다. webpack.config.js 이름에서 알 수 있듯이 webpack을 구성하는 데 사용됩니다. package.json은 말할 필요도 없습니다.
comComponent.js
export default function () { var element = document.createElement('h1'); element.innerHTML = 'Hello world'; return element; }
comComponent.js는 콘텐츠와 함께 h1 요소를 출력하는 것입니다. 내보내기 기본값은 ES6 구문으로, 이는 기본 출력을 지정한다는 의미입니다. 가져올 때 중괄호를 포함할 필요가 없습니다.
index.js
import component from './component'; document.body.appendChild(component());
index.js의 기능은 구성 요소 모듈을 참조하고 페이지에 h1 요소를 출력하는 것입니다. 하지만 현재 index.html 파일이 없기 때문에 이를 완료하려면 플러그인이 필요합니다.
npm install html-webpack-plugin --save-dev
html-webpack-plugin은 html을 생성하고 개발 디렉터리에 설치하는 데 사용됩니다.
4. webpack 구성 파일을 설정합니다
webpack.config.js 파일을 통해 webpack 시작 방법을 알려줘야 합니다. 구성 파일에는 최소한 하나의 항목과 하나의 출력이 필요합니다. 여러 페이지에는 여러 개의 입구가 필요합니다. 노드
const path = require('path'); const HtmlWebpackPlugin = require('html-webpack-plugin'); const PATHS = { app: path.join(__dirname, 'app'), build: path.join(__dirname, 'build'), }; module.exports = { entry: { app: PATHS.app, }, output: { path: PATHS.build, filename: '[name].js', }, plugins: [ new HtmlWebpackPlugin({ title: 'Webpack demo', }), ], };
의 경로 모듈은 처음 이 구성 파일을 봤을 때 약간 혼란스러웠습니다. 주로 내보내기이며 입구, 출력, 플러그인의 세 부분으로 나누어져 있습니다. 진입점은 앱 폴더를 가리킵니다. 기본적으로 "index.js"가 포함된 파일이 항목으로 사용됩니다. 출력은 빌드 주소와 파일 이름을 지정합니다. 여기서 [name]은 webpack에서 제공하는 변수로 간주될 수 있는 자리 표시자를 나타냅니다. 이에 대해서는 나중에 살펴보겠습니다. HtmlWebpackPlugin은 기본 html 파일을 생성합니다.
5. Packaging
위의 준비사항으로 webpack에 직접 들어가 실행하시면 됩니다.
이 출력에는 해시(패키지 값이 매번 다름), 버전 및 시간(시간이 많이 소요됨)이 포함됩니다. 및 출력 파일 정보. 이때 build 폴더를 열고 추가 app.js 및 index.html 파일을 찾으세요. index.html을 두 번 클릭하세요.
package.json
{ "name": "Html5", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "build": "webpack" }, "keywords": [], "author": "", "license": "ISC", "devDependencies": { "html-webpack-plugin": "^2.28.0", "webpack": "^2.2.1" } }
을 수정하여 빌드를 지정할 수도 있습니다. cmd에서 npm run build를 실행하면 동일한 결과가 나옵니다
helloword가 나타납니다. 파일 내용을 다시 살펴보세요
index.html:
nbsp;html> <meta> <title>Webpack demo</title> <script></script>
app.js가 기본적으로 참조됩니다.
6. 분석
app.js
/******/ (function(modules) { // webpackBootstrap /******/ // The module cache /******/ var installedModules = {}; /******/ // The require function /******/ function __webpack_require__(moduleId) { /******/ // Check if module is in cache /******/ if(installedModules[moduleId]) /******/ return installedModules[moduleId].exports; /******/ // Create a new module (and put it into the cache) /******/ var module = installedModules[moduleId] = { /******/ i: moduleId, /******/ l: false, /******/ exports: {} /******/ }; /******/ // Execute the module function /******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__); /******/ // Flag the module as loaded /******/ module.l = true; /******/ // Return the exports of the module /******/ return module.exports; /******/ } /******/ // expose the modules object (__webpack_modules__) /******/ __webpack_require__.m = modules; /******/ // expose the module cache /******/ __webpack_require__.c = installedModules; /******/ // identity function for calling harmony imports with the correct context /******/ __webpack_require__.i = function(value) { return value; }; /******/ // define getter function for harmony exports /******/ __webpack_require__.d = function(exports, name, getter) { /******/ if(!__webpack_require__.o(exports, name)) { /******/ Object.defineProperty(exports, name, { /******/ configurable: false, /******/ enumerable: true, /******/ get: getter /******/ }); /******/ } /******/ }; /******/ // getDefaultExport function for compatibility with non-harmony modules /******/ __webpack_require__.n = function(module) { /******/ var getter = module && module.__esModule ? /******/ function getDefault() { return module['default']; } : /******/ function getModuleExports() { return module; }; /******/ __webpack_require__.d(getter, 'a', getter); /******/ return getter; /******/ }; /******/ // Object.prototype.hasOwnProperty.call /******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); }; /******/ // __webpack_public_path__ /******/ __webpack_require__.p = ""; /******/ // Load entry module and return exports /******/ return __webpack_require__(__webpack_require__.s = 1); /******/ }) /************************************************************************/ /******/ ([ /* 0 */ /***/ (function(module, __webpack_exports__, __webpack_require__) { "use strict"; /* harmony default export */ __webpack_exports__["a"] = function () { var element = document.createElement('h1'); element.innerHTML = 'Hello world'; return element; }; /***/ }), /* 1 */ /***/ (function(module, __webpack_exports__, __webpack_require__) { "use strict"; Object.defineProperty(__webpack_exports__, "__esModule", { value: true }); /* harmony import */ var __WEBPACK_IMPORTED_MODULE_0__component__ = __webpack_require__(0); document.body.appendChild(__webpack_require__.i(__WEBPACK_IMPORTED_MODULE_0__component__["a" /* default */])()); /***/ }) /******/ ]);
그리고 app.js에는 더 많은 콘텐츠가 있습니다. 모든 것은 익명 기능입니다.
(function(module) { })([(function (){}), function() {}])
app 폴더에 있는 두 개의 js 파일이 여기서 두 개의 모듈이 됩니다. 함수는 __webpack_require__
return __webpack_require__(__webpack_require__.s = 1);
에서 시작합니다. 모듈 1에서 실행되도록 지정됩니다(대입 문의 반환 값은 해당 값입니다). 모듈 1에 대한 호출은 __webpack_require__ 문장을 통해 실행됩니다.
코드 복사 코드는 다음과 같습니다.
modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
호출을 통해 모듈을 호출하는 주요 기능은 매개변수.
(function(module, __webpack_exports__, __webpack_require__) { "use strict"; Object.defineProperty(__webpack_exports__, "__esModule", { value: true }); /* harmony import */ var __WEBPACK_IMPORTED_MODULE_0__component__ = __webpack_require__(0); document.body.appendChild(__webpack_require__.i(__WEBPACK_IMPORTED_MODULE_0__component__["a" /* default */])()); /***/ })
__webpack_require__ 모듈이 로드될 때마다 먼저 모듈 캐시에서 검색합니다. 그렇지 않은 경우 새 모듈 개체를 만듭니다.
var module = installedModules[moduleId] = { i: moduleId, l: false, exports: {} };
模块1中加载了模块0,
var __WEBPACK_IMPORTED_MODULE_0__component__ = __webpack_require__(0);
__WEBPACK_IMPORTED_MODULE_0__component__ 返回的是这个模块0的exports部分。而之前Component.js的默认方法定义成了
__webpack_exports__["a"] = function () { var element = document.createElement('h1'); element.innerHTML = 'Hello world'; return element; }
所以再模块1的定义通过"a“来获取这个方法:
复制代码 代码如下:
document.body.appendChild(__webpack_require__.i(__WEBPACK_IMPORTED_MODULE_0__component__["a" /* default */])());
这样就完整了,但这里使用了__webpack_require__.i 将原值返回。
/******/ // identity function for calling harmony imports with the correct context /******/ __webpack_require__.i = function(value) { return value; };
不太明白这个i函数有什么作用。这个注释也不太明白,路过的大神希望可以指点下。
小结:
webpack通过一个立即执行的匿名函数将各个开发模块作为参数初始化,每个js文件(module)对应一个编号,每个js中export的方法或者对象有各自指定的关键字。通过这种方式将所有的模块和接口方法管理起来。然后先加载最后的一个模块(应该是引用别的模块的模块),这样进而去触发别的模块的加载,使整个js运行起来。到这基本了解了webpack的功能和部分原理,但略显复杂,且没有感受到有多大的好处。继续探索。
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
위 내용은 웹팩 초기화부터 사용까지의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
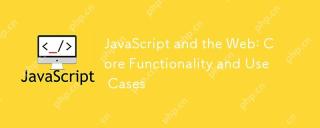
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
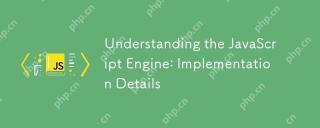
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.

Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
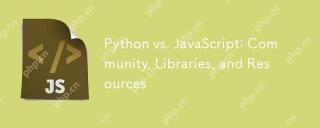
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
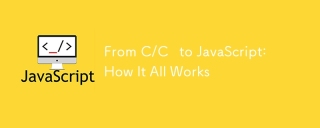
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
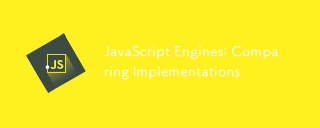
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
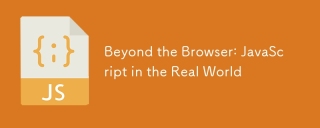
실제 세계에서 JavaScript의 응용 프로그램에는 서버 측 프로그래밍, 모바일 애플리케이션 개발 및 사물 인터넷 제어가 포함됩니다. 1. 서버 측 프로그래밍은 Node.js를 통해 실현되며 동시 요청 처리에 적합합니다. 2. 모바일 애플리케이션 개발은 재교육을 통해 수행되며 크로스 플랫폼 배포를 지원합니다. 3. Johnny-Five 라이브러리를 통한 IoT 장치 제어에 사용되며 하드웨어 상호 작용에 적합합니다.
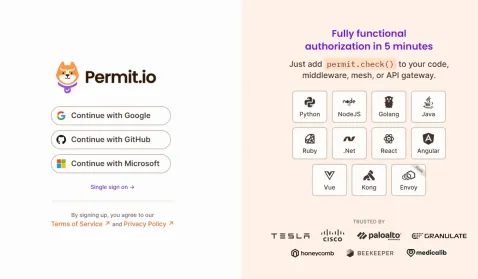
일상적인 기술 도구를 사용하여 기능적 다중 테넌트 SaaS 응용 프로그램 (Edtech 앱)을 구축했으며 동일한 작업을 수행 할 수 있습니다. 먼저, 다중 테넌트 SaaS 응용 프로그램은 무엇입니까? 멀티 테넌트 SAAS 응용 프로그램은 노래에서 여러 고객에게 서비스를 제공 할 수 있습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
