이번에는 캔버스를 사용하여 검은 배경에 빨간색 불꽃을 만드는 방법을 보여 드리겠습니다. 캔버스를 사용하여 검은 배경에 빨간색 불꽃을 만들 때 주의 사항은 무엇입니까?
html
<canvas id="canvas"></canvas>
css
body { background: #000; margin: 0; }canvas { cursor: crosshair; display: block;}
js
// when animating on canvas, it is best to use requestAnimationFrame instead of setTimeout or setInterval// not supported in all browsers though and sometimes needs a prefix, so we need a shimwindow.requestAnimFrame = (function () { return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || function (callback) { window.setTimeout(callback, 1000 / 60); }; })();// now we will setup our basic variables for the demovar canvas = document.getElementById('canvas'), ctx = canvas.getContext('2d'), // full screen dimensions cw = window.innerWidth, ch = window.innerHeight, // firework collection fireworks = [], // particle collection particles = [], // starting hue hue = 120, // when launching fireworks with a click, too many get launched at once without a limiter, one launch per 5 loop ticks limiterTotal = 5, limiterTick = 0, // this will time the auto launches of fireworks, one launch per 80 loop ticks timerTotal = 80, timerTick = 0, mousedown = false, // mouse x coordinate, mx, // mouse y coordinate my;// set canvas dimensionscanvas.width = cw; canvas.height = ch;// now we are going to setup our function placeholders for the entire demo// get a random number within a rangefunction random(min, max) { return Math.random() * (max - min) + min; }// calculate the distance between two pointsfunction calculateDistance(p1x, p1y, p2x, p2y) { var xDistance = p1x - p2x, yDistance = p1y - p2y; return Math.sqrt(Math.pow(xDistance, 2) + Math.pow(yDistance, 2)); }// create fireworkfunction Firework(sx, sy, tx, ty) { // actual coordinates this.x = sx; this.y = sy; // starting coordinates this.sx = sx; this.sy = sy; // target coordinates this.tx = tx; this.ty = ty; // distance from starting point to target this.distanceToTarget = calculateDistance(sx, sy, tx, ty); this.distanceTraveled = 0; // track the past coordinates of each firework to create a trail effect, increase the coordinate count to create more prominent trails this.coordinates = []; this.coordinateCount = 3; // populate initial coordinate collection with the current coordinates while (this.coordinateCount--) { this.coordinates.push([this.x, this.y]); } this.angle = Math.atan2(ty - sy, tx - sx); this.speed = 2; this.acceleration = 1.05; this.brightness = random(50, 70); // circle target indicator radius this.targetRadius = 1; }// update fireworkFirework.prototype.update = function (index) { // remove last item in coordinates array this.coordinates.pop(); // add current coordinates to the start of the array this.coordinates.unshift([this.x, this.y]); // cycle the circle target indicator radius if (this.targetRadius < 8) { this.targetRadius += 0.3; } else { this.targetRadius = 1; } // speed up the firework this.speed *= this.acceleration; // get the current velocities based on angle and speed var vx = Math.cos(this.angle) * this.speed, vy = Math.sin(this.angle) * this.speed; // how far will the firework have traveled with velocities applied? this.distanceTraveled = calculateDistance(this.sx, this.sy, this.x + vx, this.y + vy); // if the distance traveled, including velocities, is greater than the initial distance to the target, then the target has been reached if (this.distanceTraveled >= this.distanceToTarget) { createParticles(this.tx, this.ty); // remove the firework, use the index passed into the update function to determine which to remove fireworks.splice(index, 1); } else { // target not reached, keep traveling this.x += vx; this.y += vy; } }// draw fireworkFirework.prototype.draw = function () { ctx.beginPath(); // move to the last tracked coordinate in the set, then draw a line to the current x and y ctx.moveTo(this.coordinates[this.coordinates.length - 1][0], this.coordinates[this.coordinates.length - 1][ 1 ]); ctx.lineTo(this.x, this.y); ctx.strokeStyle = 'hsl(' + hue + ', 100%, ' + this.brightness + '%)'; ctx.stroke(); ctx.beginPath(); // draw the target for this firework with a pulsing circle ctx.arc(this.tx, this.ty, this.targetRadius, 0, Math.PI * 2); ctx.stroke(); }// create particlefunction Particle(x, y) { this.x = x; this.y = y; // track the past coordinates of each particle to create a trail effect, increase the coordinate count to create more prominent trails this.coordinates = []; this.coordinateCount = 5; while (this.coordinateCount--) { this.coordinates.push([this.x, this.y]); } // set a random angle in all possible directions, in radians this.angle = random(0, Math.PI * 2); this.speed = random(1, 10); // friction will slow the particle down this.friction = 0.95; // gravity will be applied and pull the particle down this.gravity = 1; // set the hue to a random number +-20 of the overall hue variable this.hue = random(hue - 20, hue + 20); this.brightness = random(50, 80); this.alpha = 1; // set how fast the particle fades out this.decay = random(0.015, 0.03); }// update particleParticle.prototype.update = function (index) { // remove last item in coordinates array this.coordinates.pop(); // add current coordinates to the start of the array this.coordinates.unshift([this.x, this.y]); // slow down the particle this.speed *= this.friction; // apply velocity this.x += Math.cos(this.angle) * this.speed; this.y += Math.sin(this.angle) * this.speed + this.gravity; // fade out the particle this.alpha -= this.decay; // remove the particle once the alpha is low enough, based on the passed in index if (this.alpha <= this.decay) { particles.splice(index, 1); } }// draw particleParticle.prototype.draw = function () { ctx.beginPath(); // move to the last tracked coordinates in the set, then draw a line to the current x and y ctx.moveTo(this.coordinates[this.coordinates.length - 1][0], this.coordinates[this.coordinates.length - 1][ 1 ]); ctx.lineTo(this.x, this.y); ctx.strokeStyle = 'hsla(' + this.hue + ', 100%, ' + this.brightness + '%, ' + this.alpha + ')'; ctx.stroke(); }// create particle group/explosionfunction createParticles(x, y) { // increase the particle count for a bigger explosion, beware of the canvas performance hit with the increased particles though var particleCount = 30; while (particleCount--) { particles.push(new Particle(x, y)); } }// main demo loopfunction loop() { // this function will run endlessly with requestAnimationFrame requestAnimFrame(loop); // increase the hue to get different colored fireworks over time hue += 0.5; // normally, clearRect() would be used to clear the canvas // we want to create a trailing effect though // setting the composite operation to destination-out will allow us to clear the canvas at a specific opacity, rather than wiping it entirely ctx.globalCompositeOperation = 'destination-out'; // decrease the alpha property to create more prominent trails ctx.fillStyle = 'rgba(0, 0, 0, 0.5)'; ctx.fillRect(0, 0, cw, ch); // change the composite operation back to our main mode // lighter creates bright highlight points as the fireworks and particles overlap each other ctx.globalCompositeOperation = 'lighter'; var text = "HAPPY NEW YEAR !"; ctx.font = "50px sans-serif"; var textData = ctx.measureText(text); ctx.fillStyle = "rgba(" + parseInt(random(0, 255)) + "," + parseInt(random(0, 255)) + "," + parseInt(random(0, 255)) + ",0.3)"; ctx.fillText(text, cw / 2 - textData.width / 2, ch / 2); // loop over each firework, draw it, update it var i = fireworks.length; while (i--) { fireworks[i].draw(); fireworks[i].update(i); } // loop over each particle, draw it, update it var i = particles.length; while (i--) { particles[i].draw(); particles[i].update(i); } // launch fireworks automatically to random coordinates, when the mouse isn't down if (timerTick >= timerTotal) { if (!mousedown) { // start the firework at the bottom middle of the screen, then set the random target coordinates, the random y coordinates will be set within the range of the top half of the screen for (var h = 0; h < 50; h++) { fireworks.push(new Firework(cw / 2, ch / 2, random(0, cw), random(0, ch))); } timerTick = 0; } } else { timerTick++; } // limit the rate at which fireworks get launched when mouse is down if (limiterTick >= limiterTotal) { if (mousedown) { // start the firework at the bottom middle of the screen, then set the current mouse coordinates as the target fireworks.push(new Firework(cw / 2, ch / 2, mx, my)); limiterTick = 0; } } else { limiterTick++; } }// mouse event bindings// update the mouse coordinates on mousemovecanvas.addEventListener('mousemove', function (e) { mx = e.pageX - canvas.offsetLeft; my = e.pageY - canvas.offsetTop; });// toggle mousedown state and prevent canvas from being selectedcanvas.addEventListener('mousedown', function (e) { e.preventDefault(); mousedown = true; }); canvas.addEventListener('mouseup', function (e) { e.preventDefault(); mousedown = false; });// once the window loads, we are ready for some fireworks!window.onload = loop;
이 기사의 사례를 읽으신 후 방법을 마스터하셨다고 생각합니다. 더 흥미로운 정보를 보려면 PHP 중국어 웹사이트의 다른 관련 기사에 주목하세요.
추천 도서:
위 내용은 캔버스에 검은색 배경에 빨간 불꽃놀이 만드는 법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
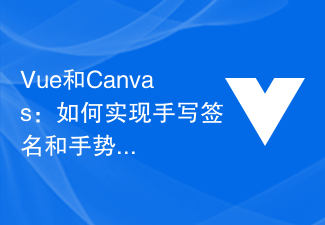
Vue和Canvas:如何实现手写签名和手势识别功能引言:手写签名和手势识别功能在现代应用程序中越来越常见,它们可以为用户提供更加直观和自然的交互方式。Vue.js作为一款流行的前端框架,搭配Canvas元素可以实现这两个功能。本文将介绍如何使用Vue.js和Canvas元素来实现手写签名和手势识别功能,并给出相应的代码示例。一、手写签名功能实现要实现手写签
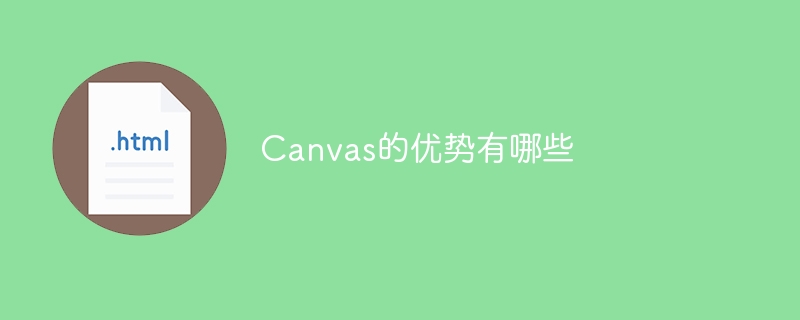
canvas的优势有强大的绘图功能、高性能、跨平台兼容性、支持多种图形格式、可以与其他Web技术集成、可以实现动态效果和可以实现复杂的图像处理。详细介绍:1、Canvas提供了丰富的绘图功能,可以绘制各种形状、线条、文本、图像等;2、Canvas在浏览器中直接操作像素,因此具有很高的性能;3、Canvas是基于HTML5标准的一部分,可以在各种现代浏览器上运行等等。
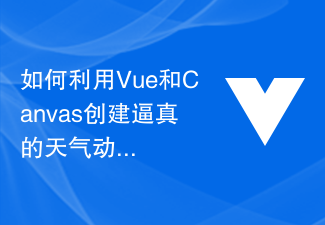
如何利用Vue和Canvas创建逼真的天气动态背景引言:在现代网页设计中,动态背景效果是吸引用户眼球的重要元素之一。本文将介绍如何利用Vue和Canvas技术来创建一个逼真的天气动态背景效果。通过代码示例,你将学习如何编写Vue组件和利用Canvas绘制不同天气场景,从而实现一个独特而吸引人的背景效果。步骤一:创建Vue项目首先,我们需要创建一个Vue项目。
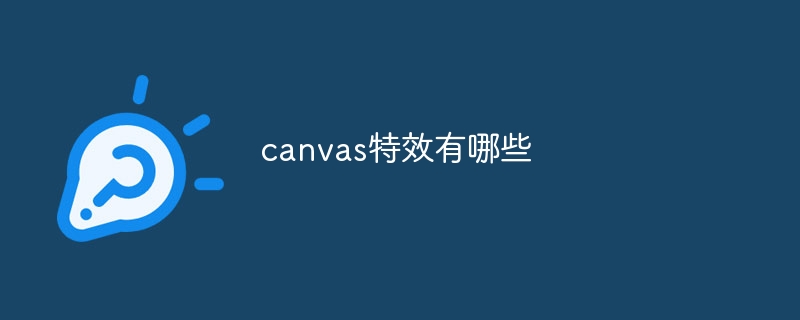
canvas特效有粒子效果、线条动画、图片处理、文字动画、音频可视化、3D效果、游戏开发等。详细介绍:1、粒子效果,通过控制粒子的位置、速度和颜色等属性来实现各种效果,如烟花、雨滴、星空等;2、线条动画,通过在画布上绘制连续的线条,创建出各种动态的线条效果;3、图片处理,通过对图片进行处理,可以实现各种炫酷的效果,如图片切换、图片特效等;4、文字动画等等特性。
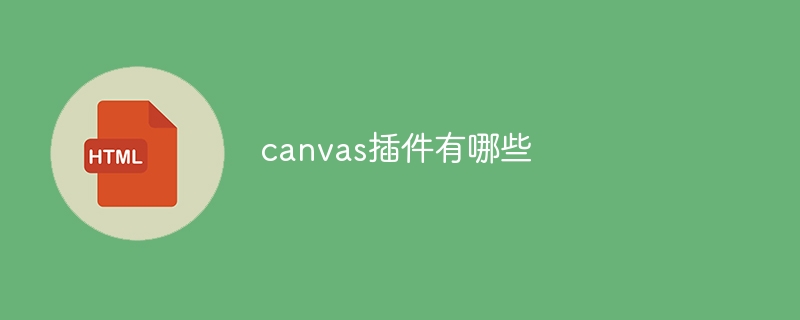
canvas插件有Fabric.js、EaselJS、Konva.js、Three.js、Paper.js、Chart.js和Phaser。详细介绍:1、Fabric.js 是一个基于Canvas的开源 JavaScript 库,它提供了一些强大的功能;2、EaselJS是CreateJS库中的一个模块,它提供了一套简化了Canvas编程的API;3、Konva.js等等。
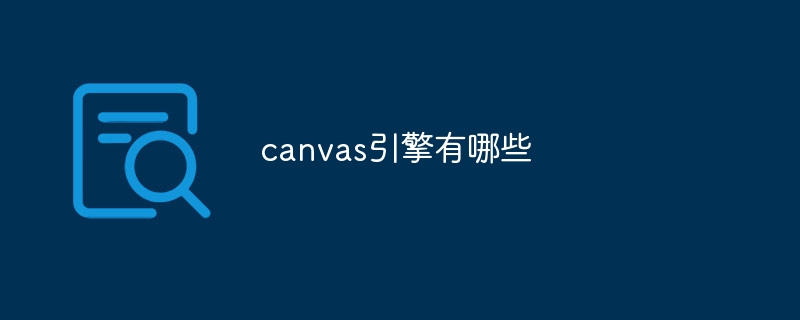
canvas引擎有Three.js、Pixi.js、EaselJS、Konva.js、Paper.js等。详细介绍:1、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,同时还提供了丰富的工具和插件,方便开发者进行交互、动画和优化等操作;2、Pixi.js,提供了简单易用的API,支持精灵、纹理、滤镜等功能,还提供了丰富的工具和插件;3、EaselJS等等。
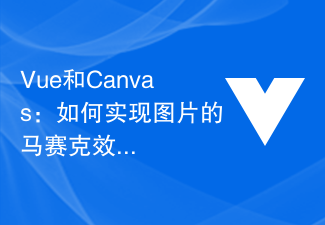
Vue和Canvas:如何实现图片的马赛克效果引言:随着Web技术的不断发展,越来越多的人开始使用Vue框架来构建交互式的前端应用。而在前端开发中,常常需要为用户提供图片处理的功能。本文将介绍如何利用Vue和Canvas实现图片的马赛克效果,为用户带来更好的视觉体验。一、马赛克效果概述马赛克效果是一种将图像的细节部分进行像素化处理,使得整个图像变得模糊和抽象
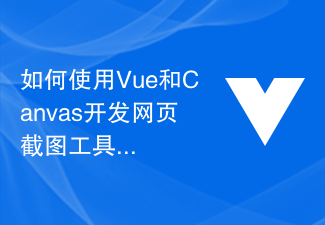
如何使用Vue和Canvas开发网页截图工具简介:随着互联网的发展,网页截图工具在我们的日常生活中扮演着越来越重要的角色。它们可以用来捕捉网页上的信息、制作教程或者分享你的见解。本文将介绍如何使用Vue和Canvas来开发一个简单的网页截图工具,以帮助读者了解如何实现这个常见但又有趣的功能。准备工作:在开始之前,我们需要准备好以下的开发环境和工具:安装Nod


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
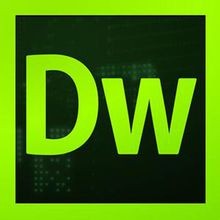
드림위버 CS6
시각적 웹 개발 도구

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경
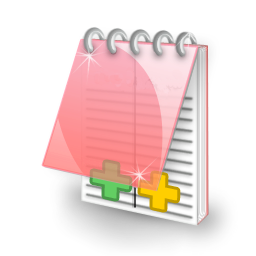
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!
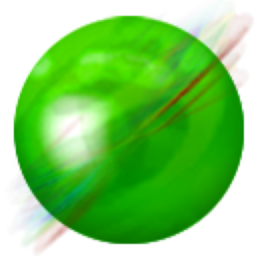
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
