라우팅은 외부 세계가 Laravel 애플리케이션에 액세스하는 방법입니다. 또는 라우팅은 Laravel 애플리케이션이 외부 세계에 서비스를 제공하는 특정 방식을 정의합니다. 지정된 URI, HTTP 요청 방법 및 라우팅 매개변수(선택 사항)를 통해서만 올바르게 작업할 수 있습니다. 라우팅 정의 프로그램 처리에 액세스합니다. URI에 해당하는 핸들러가 단순 클로저이거나 컨트롤러 메소드에 해당 경로가 없으면 외부 세계에서는 이에 접근할 수 없습니다. 오늘은 Laravel이 라우팅을 어떻게 설계하고 구현하는지 살펴보겠습니다.
우리는 일반적으로 라우팅 파일에서 다음과 같이 경로를 정의합니다.
Route::get('/user', 'UsersController@index');
위의 라우팅을 통해 클라이언트가 HTTP GET을 통해 URI "/user"를 요청하면 Laravel이 결국 요청을 발송한다는 것을 알 수 있습니다. 인덱스 메소드 제공 UsersController 클래스를 처리한 다음 인덱스 메서드에서 클라이언트에 응답을 반환합니다.
위의 경로를 등록할 때 사용한 Route 클래스는 Laravel에서 Facade라고 합니다. 이는 서비스 컨테이너에 바인딩된 서비스 라우터에 액세스하는 간단한 방법을 제공합니다. 앞으로 Facade의 설계 개념과 구현 방법을 사용할 계획입니다. 별도의 블로그 게시물을 작성하세요. 여기서는 호출되는 Route 파사드의 정적 메서드가 서비스 컨테이너의 라우터 서비스 메서드에 해당한다는 것만 알면 됩니다. 따라서 위의 경로가 다음과 같이 등록된 것을 볼 수도 있습니다:
app()->make('router')->get('user', 'UsersController@index');
router 이 서비스는 애플리케이션을 인스턴스화할 때 생성자에 RoutingServiceProvider를 등록하여 서비스 컨테이너에 바인딩됩니다. 애플리케이션:
//bootstrap/app.php $app = new Illuminate\Foundation\Application( realpath(__DIR__.'/../') ); //Application: 构造方法 public function __construct($basePath = null) { if ($basePath) { $this->setBasePath($basePath); } $this->registerBaseBindings(); $this->registerBaseServiceProviders(); $this->registerCoreContainerAliases(); } //Application: 注册基础的服务提供器 protected function registerBaseServiceProviders() { $this->register(new EventServiceProvider($this)); $this->register(new LogServiceProvider($this)); $this->register(new RoutingServiceProvider($this)); } //\Illuminate\Routing\RoutingServiceProvider: 绑定router到服务容器 protected function registerRouter() { $this->app->singleton('router', function ($app) { return new Router($app['events'], $app); }); }
위 코드를 통해 Route에서 호출한 정적 메서드는 모두 IlluminateRoutingRouter
에 해당한다는 것을 알 수 있습니다. code>클래스의 메소드, Router 클래스에는 라우팅 등록, 주소 지정 및 스케줄링과 관련된 메소드가 포함되어 있습니다. IlluminateRoutingRouter
类里的方法,Router这个类里包含了与路由的注册、寻址、调度相关的方法。
下面我们从路由的注册、加载、寻址这几个阶段来看一下laravel里是如何实现这些的。
路由加载
注册路由前需要先加载路由文件,路由文件的加载是在AppProvidersRouteServiceProvider
这个服务器提供者的boot方法里加载的:
class RouteServiceProvider extends ServiceProvider { public function boot() { parent::boot(); } public function map() { $this->mapApiRoutes(); $this->mapWebRoutes(); } protected function mapWebRoutes() { Route::middleware('web') ->namespace($this->namespace) ->group(base_path('routes/web.php')); } protected function mapApiRoutes() { Route::prefix('api') ->middleware('api') ->namespace($this->namespace) ->group(base_path('routes/api.php')); } }
namespace Illuminate\Foundation\Support\Providers; class RouteServiceProvider extends ServiceProvider { public function boot() { $this->setRootControllerNamespace(); if ($this->app->routesAreCached()) { $this->loadCachedRoutes(); } else { $this->loadRoutes(); $this->app->booted(function () { $this->app['router']->getRoutes()->refreshNameLookups(); $this->app['router']->getRoutes()->refreshActionLookups(); }); } } protected function loadCachedRoutes() { $this->app->booted(function () { require $this->app->getCachedRoutesPath(); }); } protected function loadRoutes() { if (method_exists($this, 'map')) { $this->app->call([$this, 'map']); } } } class Application extends Container implements ApplicationContract, HttpKernelInterface { public function routesAreCached() { return $this['files']->exists($this->getCachedRoutesPath()); } public function getCachedRoutesPath() { return $this->bootstrapPath().'/cache/routes.php'; } }
laravel 首先去寻找路由的缓存文件,没有缓存文件再去进行加载路由。缓存文件一般在 bootstrap/cache/routes.php 文件中。
方法loadRoutes会调用map方法来加载路由文件里的路由,map这个函数在AppProvidersRouteServiceProvider
类中,这个类继承自IlluminateFoundationSupportProvidersRouteServiceProvider
。通过map方法我们能看到laravel将路由分为两个大组:api、web。这两个部分的路由分别写在两个文件中:routes/web.php、routes/api.php。
Laravel5.5里是把路由分别放在了几个文件里,之前的版本是在app/Http/routes.php文件里。放在多个文件里能更方便地管理API路由和与WEB路由
路由注册
我们通常都是用Route这个Facade调用静态方法get, post, head, options, put, patch, delete......等来注册路由,上面我们也说了这些静态方法其实是调用了Router类里的方法:
public function get($uri, $action = null) { return $this->addRoute(['GET', 'HEAD'], $uri, $action); } public function post($uri, $action = null) { return $this->addRoute('POST', $uri, $action); } ....
可以看到路由的注册统一都是由router类的addRoute方法来处理的:
//注册路由到RouteCollection protected function addRoute($methods, $uri, $action) { return $this->routes->add($this->createRoute($methods, $uri, $action)); } //创建路由 protected function createRoute($methods, $uri, $action) { if ($this->actionReferencesController($action)) { //controller@action类型的路由在这里要进行转换 $action = $this->convertToControllerAction($action); } $route = $this->newRoute( $methods, $this->prefix($uri), $action ); if ($this->hasGroupStack()) { $this->mergeGroupAttributesIntoRoute($route); } $this->addWhereClausesToRoute($route); return $route; } protected function convertToControllerAction($action) { if (is_string($action)) { $action = ['uses' => $action]; } if (! empty($this->groupStack)) { $action['uses'] = $this->prependGroupNamespace($action['uses']); } $action['controller'] = $action['uses']; return $action; }
注册路由时传递给addRoute的第三个参数action可以闭包、字符串或者数组,数组就是类似['uses' => 'Controller@action', 'middleware' => '...']这种形式的。如果action是Controller@action
类型的路由将被转换为action数组, convertToControllerAction执行完后action的内容为:
[ 'uses' => 'App\Http\Controllers\SomeController@someAction', 'controller' => 'App\Http\Controllers\SomeController@someAction' ]
可以看到把命名空间补充到了控制器的名称前组成了完整的控制器类名,action数组构建完成接下里就是创建路由了,创建路由即用指定的HTTP请求方法、URI字符串和action数组来创建IlluminateRoutingRoute
AppProvidersRouteServiceProvider
의 부팅 메서드에 로드됩니다.
protected function newRoute($methods, $uri, $action) { return (new Route($methods, $uri, $action)) ->setRouter($this) ->setContainer($this->container); }
protected function addRoute($methods, $uri, $action) { return $this->routes->add($this->createRoute($methods, $uri, $action)); }laravel 먼저 캐시를 찾으세요. 경로 파일, 캐시된 파일이 없으면 경로를 로드합니다. 캐시 파일은 일반적으로 bootstrap/cache/routes.php 파일에 있습니다.
loadRoutes 메소드는 라우팅 파일에 경로를 로드하기 위해 map 메소드를 호출합니다. 지도 함수는
IlluminateFoundationSupportProvidersRouteServiceProvider
에서 상속되는 AppProvidersRouteServiceProvider
클래스에 있습니다. map 메소드를 통해 laravel이 라우팅을 api와 web이라는 두 개의 큰 그룹으로 나누는 것을 볼 수 있습니다. 이 두 부분에 대한 경로는 각각 Routes/web.php 및 Routes/api.php라는 두 개의 파일로 작성됩니다.
Laravel 5.5에서는 경로가 여러 파일에 배치됩니다. 이전 버전은 app/Http/routes.php 파일에 있었습니다. 여러 파일에 배치하면 API 경로 및 WEB 경로를 더 쉽게 관리할 수 있습니다.Route 등록우리는 일반적으로 Route Facade를 사용하여 정적 메소드 get, post, head, options, put을 호출합니다. , patch, delete... 등을 사용하여 경로를 등록합니다. 위에서 말했듯이 이러한 정적 메서드는 실제로 Router 클래스의 메서드를 호출합니다.
class RouteCollection implements Countable, IteratorAggregate { public function add(Route $route) { $this->addToCollections($route); $this->addLookups($route); return $route; } protected function addToCollections($route) { $domainAndUri = $route->getDomain().$route->uri(); foreach ($route->methods() as $method) { $this->routes[$method][$domainAndUri] = $route; } $this->allRoutes[$method.$domainAndUri] = $route; } protected function addLookups($route) { $action = $route->getAction(); if (isset($action['as'])) { //如果时命名路由,将route对象映射到以路由名为key的数组值中方便查找 $this->nameList[$action['as']] = $route; } if (isset($action['controller'])) { $this->addToActionList($action, $route); } } }경로 등록이 라우터 클래스에 의해 균일하게 수행되는 것을 볼 수 있습니다. addRoute 메소드:
[ 'GET' => [ $routeUri1 => $routeObj1 ... ] ... ]경로 등록 시 addRoute에 전달되는 세 번째 매개변수 작업은 클로저, 문자열 또는 배열이 될 수 있습니다. 배열은 ['uses' => 'Controller@action', 'middleware'와 유사합니다. = > '...'] 형식으로 표시됩니다. 액션이
Controller@action
유형의 경로인 경우 액션 배열로 변환됩니다. ConvertToControllerAction이 실행된 후 액션의 내용은 다음과 같습니다. [
'GET' . $routeUri1 => $routeObj1
'GET' . $routeUri2 => $routeObj2
...
]
네임스페이스가 컨트롤러 이름 앞에 추가됩니다. 다음 단계는 경로를 만드는 것입니다. 지정된 HTTP 요청 메서드, URI 문자열 및 작업 배열을 사용하여 IlluminateRoutingRoute
클래스:
[ $routeName1 => $routeObj1 ... ]경로 생성이 완료된 후 RouteCollection에 경로를 추가합니다.
[ 'App\Http\Controllers\ControllerOne@ActionOne' => $routeObj1 ]라우터의 $routes 속성은 RouteCollection에 경로를 추가할 때입니다.
//Illuminate\Foundation\Http\Kernel class Kernel implements KernelContract { protected function sendRequestThroughRouter($request) { $this->app->instance('request', $request); Facade::clearResolvedInstance('request'); $this->bootstrap(); return (new Pipeline($this->app)) ->send($request) ->through($this->app->shouldSkipMiddleware() ? [] : $this->middleware) ->then($this->dispatchToRouter()); } protected function dispatchToRouter() { return function ($request) { $this->app->instance('request', $request); return $this->router->dispatch($request); }; } }RouteCollection 4개의 routes 속성은 HTTP 요청 메소드와 라우팅 객체 간의 매핑을 저장합니다. 🎜
$destination = function ($request) { $this->app->instance('request', $request); return $this->router->dispatch($request); };🎜allRoutes 속성에 저장된 콘텐츠는 다음과 같습니다. 경로 속성의 두 자리 배열을 한 자리 배열로 프로그래밍한 후의 내용: 🎜
class Router implements RegistrarContract, BindingRegistrar { public function dispatch(Request $request) { $this->currentRequest = $request; return $this->dispatchToRoute($request); } public function dispatchToRoute(Request $request) { return $this->runRoute($request, $this->findRoute($request)); } protected function findRoute($request) { $this->current = $route = $this->routes->match($request); $this->container->instance(Route::class, $route); return $route; } }🎜nameList는 라우팅 이름과 라우팅 개체 간의 매핑 테이블입니다. 🎜
class RouteCollection implements Countable, IteratorAggregate { public function match(Request $request) { $routes = $this->get($request->getMethod()); $route = $this->matchAgainstRoutes($routes, $request); if (! is_null($route)) { return $route->bind($request); } $others = $this->checkForAlternateVerbs($request); if (count($others) > 0) { return $this->getRouteForMethods($request, $others); } throw new NotFoundHttpException; } protected function matchAgainstRoutes(array $routes, $request, $includingMethod = true) { return Arr::first($routes, function ($value) use ($request, $includingMethod) { return $value->matches($request, $includingMethod); }); } } class Route { public function matches(Request $request, $includingMethod = true) { $this->compileRoute(); foreach ($this->getValidators() as $validator) { if (! $includingMethod && $validator instanceof MethodValidator) { continue; } if (! $validator->matches($this, $request)) { return false; } } return true; } }🎜actionList는 라우팅 컨트롤러 메서드 문자열과 라우팅 개체 간의 매핑 테이블입니다. 🎜
class UriValidator implements ValidatorInterface { public function matches(Route $route, Request $request) { $path = $request->path() == '/' ? '/' : '/'.$request->path(); return preg_match($route->getCompiled()->getRegex(), rawurldecode($path)); } }🎜 이렇게 경로가 등록됩니다. 🎜🎜Routing Addressing🎜🎜미들웨어에 대한 글에서 HTTP 요청이 Pipeline 채널에서 미들웨어의 전처리 과정을 거쳐 목적지에 도달한다고 했는데요:🎜
class Route { public function bind(Request $request) { $this->compileRoute(); $this->parameters = (new RouteParameterBinder($this)) ->parameters($request); return $this; } } class RouteParameterBinder { public function parameters($request) { $parameters = $this->bindPathParameters($request); if (! is_null($this->route->compiled->getHostRegex())) { $parameters = $this->bindHostParameters( $request, $parameters ); } return $this->replaceDefaults($parameters); } protected function bindPathParameters($request) { preg_match($this->route->compiled->getRegex(), '/'.$request->decodedPath(), $matches); return $this->matchToKeys(array_slice($matches, 1)); } protected function matchToKeys(array $matches) { if (empty($parameterNames = $this->route->parameterNames())) { return []; } $parameters = array_intersect_key($matches, array_flip($parameterNames)); return array_filter($parameters, function ($value) { return is_string($value) && strlen($value) > 0; }); } }🎜위의 코드를 보면 목적지가 파이프라인은 DispatchToRouter 함수 클로저에 의해 반환됩니다. 🎜
class Router implements RegistrarContract, BindingRegistrar { public function dispatch(Request $request) { $this->currentRequest = $request; return $this->dispatchToRoute($request); } public function dispatchToRoute(Request $request) { return $this->runRoute($request, $this->findRoute($request)); } protected function runRoute(Request $request, Route $route) { $request->setRouteResolver(function () use ($route) { return $route; }); $this->events->dispatch(new Events\RouteMatched($route, $request)); return $this->prepareResponse($request, $this->runRouteWithinStack($route, $request) ); } }🎜 라우터의 디스패치 메서드는 클로저에서 호출됩니다. 경로 주소 지정은 디스패치의 첫 번째 단계인 findRoute에서 발생합니다. 🎜rrreee🎜 경로를 찾는 작업은 RouteCollection에 의해 처리됩니다. 이 함수는 경로 일치를 담당하고 요청 URL 매개변수를 경로에 바인딩합니다. 🎜
class RouteCollection implements Countable, IteratorAggregate { public function match(Request $request) { $routes = $this->get($request->getMethod()); $route = $this->matchAgainstRoutes($routes, $request); if (! is_null($route)) { return $route->bind($request); } $others = $this->checkForAlternateVerbs($request); if (count($others) > 0) { return $this->getRouteForMethods($request, $others); } throw new NotFoundHttpException; } protected function matchAgainstRoutes(array $routes, $request, $includingMethod = true) { return Arr::first($routes, function ($value) use ($request, $includingMethod) { return $value->matches($request, $includingMethod); }); } } class Route { public function matches(Request $request, $includingMethod = true) { $this->compileRoute(); foreach ($this->getValidators() as $validator) { if (! $includingMethod && $validator instanceof MethodValidator) { continue; } if (! $validator->matches($this, $request)) { return false; } } return true; } }
$routes = $this->get($request->getMethod());
会先加载注册路由阶段在RouteCollection里生成的routes属性里的值,routes中存放了HTTP请求方法与路由对象的映射。
然后依次调用这堆路由里路由对象的matches方法, matches方法, matches方法里会对HTTP请求对象进行一些验证,验证对应的Validator是:UriValidator、MethodValidator、SchemeValidator、HostValidator。
在验证之前在$this->compileRoute()
里会将路由的规则转换成正则表达式。
UriValidator主要是看请求对象的URI是否与路由的正则规则匹配能匹配上:
class UriValidator implements ValidatorInterface { public function matches(Route $route, Request $request) { $path = $request->path() == '/' ? '/' : '/'.$request->path(); return preg_match($route->getCompiled()->getRegex(), rawurldecode($path)); } }
MethodValidator验证请求方法, SchemeValidator验证协议是否正确(http|https), HostValidator验证域名, 如果路由中不设置host属性,那么这个验证不会进行。
一旦某个路由通过了全部的认证就将会被返回,接下来就要将请求对象URI里的路径参数绑定复制给路由参数:
路由参数绑定
class Route { public function bind(Request $request) { $this->compileRoute(); $this->parameters = (new RouteParameterBinder($this)) ->parameters($request); return $this; } } class RouteParameterBinder { public function parameters($request) { $parameters = $this->bindPathParameters($request); if (! is_null($this->route->compiled->getHostRegex())) { $parameters = $this->bindHostParameters( $request, $parameters ); } return $this->replaceDefaults($parameters); } protected function bindPathParameters($request) { preg_match($this->route->compiled->getRegex(), '/'.$request->decodedPath(), $matches); return $this->matchToKeys(array_slice($matches, 1)); } protected function matchToKeys(array $matches) { if (empty($parameterNames = $this->route->parameterNames())) { return []; } $parameters = array_intersect_key($matches, array_flip($parameterNames)); return array_filter($parameters, function ($value) { return is_string($value) && strlen($value) > 0; }); } }
赋值路由参数完成后路由寻址的过程就结束了,结下来就该运行通过匹配路由中对应的控制器方法返回响应对象了。
class Router implements RegistrarContract, BindingRegistrar { public function dispatch(Request $request) { $this->currentRequest = $request; return $this->dispatchToRoute($request); } public function dispatchToRoute(Request $request) { return $this->runRoute($request, $this->findRoute($request)); } protected function runRoute(Request $request, Route $route) { $request->setRouteResolver(function () use ($route) { return $route; }); $this->events->dispatch(new Events\RouteMatched($route, $request)); return $this->prepareResponse($request, $this->runRouteWithinStack($route, $request) ); } }
这里我们主要介绍路由相关的内容,在runRoute的过程通过上面的源码可以看到其实也很复杂, 会收集路由和控制器里的中间件,将请求通过中间件过滤才会最终调用控制器方法来生成响应对象,这个过程还会设计到我们以前介绍过的中间件过滤、服务解析、依赖注入方面的信息,如果在看源码时有不懂的地方可以翻看我之前写的文章。
依赖注入
服务容器
中间件
相关推荐:
위 내용은 Laravel 라우팅 경로에 대한 자세한 설명의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
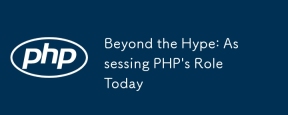
PHP는 현대적인 프로그래밍, 특히 웹 개발 분야에서 강력하고 널리 사용되는 도구로 남아 있습니다. 1) PHP는 사용하기 쉽고 데이터베이스와 완벽하게 통합되며 많은 개발자에게 가장 먼저 선택됩니다. 2) 동적 컨텐츠 생성 및 객체 지향 프로그래밍을 지원하여 웹 사이트를 신속하게 작성하고 유지 관리하는 데 적합합니다. 3) 데이터베이스 쿼리를 캐싱하고 최적화함으로써 PHP의 성능을 향상시킬 수 있으며, 광범위한 커뮤니티와 풍부한 생태계는 오늘날의 기술 스택에 여전히 중요합니다.
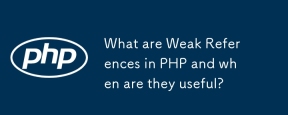
PHP에서는 약한 참조가 약한 회의 클래스를 통해 구현되며 쓰레기 수집가가 물체를 되 찾는 것을 방해하지 않습니다. 약한 참조는 캐싱 시스템 및 이벤트 리스너와 같은 시나리오에 적합합니다. 물체의 생존을 보장 할 수 없으며 쓰레기 수집이 지연 될 수 있음에 주목해야합니다.
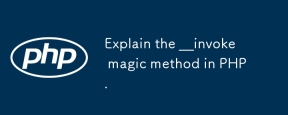
\ _ \ _ 호출 메소드를 사용하면 객체를 함수처럼 호출 할 수 있습니다. 1. 객체를 호출 할 수 있도록 메소드를 호출하는 \ _ \ _ 정의하십시오. 2. $ obj (...) 구문을 사용할 때 PHP는 \ _ \ _ invoke 메소드를 실행합니다. 3. 로깅 및 계산기, 코드 유연성 및 가독성 향상과 같은 시나리오에 적합합니다.
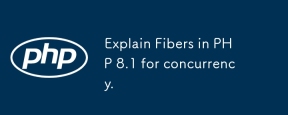
섬유는 PHP8.1에 도입되어 동시 처리 기능을 향상시켰다. 1) 섬유는 코 루틴과 유사한 가벼운 동시성 모델입니다. 2) 개발자는 작업의 실행 흐름을 수동으로 제어 할 수 있으며 I/O 집약적 작업을 처리하는 데 적합합니다. 3) 섬유를 사용하면보다 효율적이고 반응이 좋은 코드를 작성할 수 있습니다.
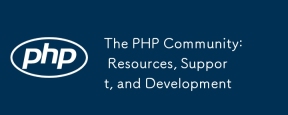
PHP 커뮤니티는 개발자 성장을 돕기 위해 풍부한 자원과 지원을 제공합니다. 1) 자료에는 공식 문서, 튜토리얼, 블로그 및 Laravel 및 Symfony와 같은 오픈 소스 프로젝트가 포함됩니다. 2) 지원은 StackoverFlow, Reddit 및 Slack 채널을 통해 얻을 수 있습니다. 3) RFC에 따라 개발 동향을 배울 수 있습니다. 4) 적극적인 참여, 코드에 대한 기여 및 학습 공유를 통해 커뮤니티에 통합 될 수 있습니다.
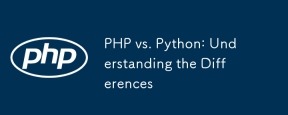
PHP와 Python은 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1.PHP는 간단한 구문과 높은 실행 효율로 웹 개발에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리를 갖춘 데이터 과학 및 기계 학습에 적합합니다.
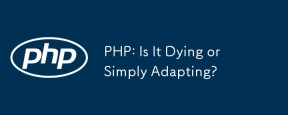
PHP는 죽지 않고 끊임없이 적응하고 진화합니다. 1) PHP는 1994 년부터 새로운 기술 트렌드에 적응하기 위해 여러 버전 반복을 겪었습니다. 2) 현재 전자 상거래, 컨텐츠 관리 시스템 및 기타 분야에서 널리 사용됩니다. 3) PHP8은 성능과 현대화를 개선하기 위해 JIT 컴파일러 및 기타 기능을 소개합니다. 4) Opcache를 사용하고 PSR-12 표준을 따라 성능 및 코드 품질을 최적화하십시오.
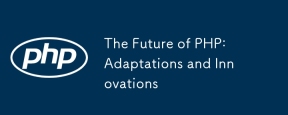
PHP의 미래는 새로운 기술 트렌드에 적응하고 혁신적인 기능을 도입함으로써 달성 될 것입니다. 1) 클라우드 컴퓨팅, 컨테이너화 및 마이크로 서비스 아키텍처에 적응, Docker 및 Kubernetes 지원; 2) 성능 및 데이터 처리 효율을 향상시키기 위해 JIT 컴파일러 및 열거 유형을 도입합니다. 3) 지속적으로 성능을 최적화하고 모범 사례를 홍보합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
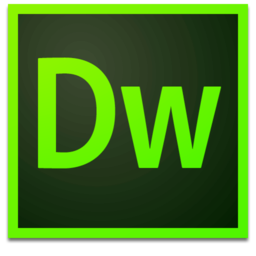
Dreamweaver Mac版
시각적 웹 개발 도구

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.
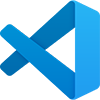
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
