다음 편집기에서는 Python을 사용하여 원격 서버에 연결하고 paramiko를 사용하여 명령을 실행하는 방법에 대한 기사를 제공합니다. 편집자님이 꽤 좋다고 생각하셔서 지금 공유하고 모두에게 참고용으로 드리고자 합니다. 편집기를 따라가며 살펴보겠습니다. Python의 paramiko 모듈은 원격 서버에 대한 SSH 연결을 구현하는 데 사용되는 라이브러리입니다. 연결 시 명령을 실행하거나 파일을 업로드하는 데 사용할 수 있습니다.
1. 연결 개체 가져오기 연결할 때 다음 코드를 사용할 수 있습니다.
def connect(host): 'this is use the paramiko connect the host,return conn' ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) try: # ssh.connect(host,username='root',allow_agent=True,look_for_keys=True) ssh.connect(host,username='root',password='root',allow_agent=True) return ssh except: return None
연결 함수에서 매개변수는 호스트의 IP 주소 또는 호스트 이름입니다. 이 방법을 사용하면 서버 연결에 성공하면 sshclient 개체가 반환됩니다.
첫 번째 단계는 SSHClient 개체를 생성한 다음, Know_host 파일에 없는 컴퓨터에 대한 연결을 허용하도록 SSH 클라이언트를 설정한 다음 서버에 연결을 시도하는 것입니다. 서버에 연결할 때 두 가지 방법을 사용할 수 있습니다. : 한 가지 방법은 비밀을 사용하는 것입니다. 키 방법, 즉 매개변수 look_for_keys는 여기서 검색할 비밀번호를 설정하는 데 사용되거나 비밀번호 방법을 직접 사용할 수 있습니다. 즉 매개변수 비밀번호를 직접 사용하여 최종적으로 연결된 객체를 반환합니다.
2. set 명령 가져오기 paramiko 연결을 만든 후 다음 코드와 같이 실행해야 하는 명령을 가져와야 합니다.
def command(args,outpath): 'this is get the command the args to return the command' cmd = '%s %s' % (outpath,args) return cmd
매개변수 중 하나는 다음과 같습니다. args이고 다른 하나는 outpath 입니다. args는 명령의 매개변수를 나타내고 outpath는 /usr/bin/ls -l과 같은 실행 파일의 경로를 나타냅니다. 그 중 outpath는 /usr/bin/ls이고, 매개변수는 -l
입니다. 이 방법은 주로 명령을 결합하고, 명령의 일부로 별도의 매개변수를 조합하는 데 사용됩니다.
3. 명령 실행 연결 후 직접 명령을 실행할 수 있으며 다음 함수가 있습니다.
def exec_commands(conn,cmd): 'this is use the conn to excute the cmd and return the results of excute the command' stdin,stdout,stderr = conn.exec_command(cmd) results=stdout.read() return results
이 함수에서 전달된 매개 변수는 연결 개체 conn입니다. 실행해야 하는 명령 cmd를 실행하고 마지막으로 실행 결과인 stdout.read()를 가져와서 마지막으로 결과를 반환합니다.
4. 파일 업로드 연결 개체를 사용할 때 직접 수행할 수도 있습니다. 관련 파일 업로드, 다음 함수:
def copy_moddule(conn,inpath,outpath): 'this is copy the module to the remote server' ftp = conn.open_sftp() ftp.put(inpath,outpath) ftp.close() return outpath
이 함수의 주요 매개 변수는 하나는 연결 개체 conn이고 다른 하나는 업로드된 파일의 이름이며 업로드 후 파일의 이름입니다. 완료 파일 이름에는 경로가 포함됩니다.
주요 방법은 sftp 개체를 연 다음 put 메서드를 사용하여 파일을 업로드하고 마지막으로 sftp 연결을 닫은 다음 마지막으로 업로드된 파일 이름의 전체 경로를 반환하는 것입니다
5. the result마지막으로, 명령을 실행하고 반환된 결과를 얻으세요. 다음 코드는
def excutor(host,outpath,args): conn = connect(host) if not conn: return [host,None] exec_commands(conn,'chmod +x %s' % outpath) cmd =command(args,outpath) result = exec_commands(conn,cmd) print '%r' % result result = json.loads(result) return [host,result]
먼저 서버에 연결하고 연결에 실패하면 반환합니다. 연결에 성공하지 못했다면 파일의 실행 권한을 수정하여 파일을 실행할 수 있도록 한 다음 실행된 명령을 얻습니다. 실행하면 결과가 얻어지고 결과가 json 형식으로 반환되므로 결과를 아름다운 json 형식으로 얻을 수 있으며 최종적으로 호스트 이름과 함께 반환됩니다. 6.
테스트 코드는 다음과 같습니다.
if __name__ == '__main__': print json.dumps(excutor('192.168.1.165','ls',' -l'),indent=4,sort_keys=True) print copy_module(connect('192.168.1.165'),'kel.txt','/root/kel.1.txt') exec_commands(connect('192.168.1.165'),'chmod +x %s' % '/root/kel.1.txt')첫 번째 단계에서는 명령 실행을 테스트하고, 두 번째 단계에서는 업로드된 파일을 테스트하고, 세 번째 단계에서는 업로드된 파일을 수정할 수 있는 권한을 테스트합니다. 전체 코드는 다음과 같습니다.
#!/usr/bin/env python import json import paramiko def connect(host): 'this is use the paramiko connect the host,return conn' ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) try: # ssh.connect(host,username='root',allow_agent=True,look_for_keys=True) ssh.connect(host,username='root',password='root',allow_agent=True) return ssh except: return None def command(args,outpath): 'this is get the command the args to return the command' cmd = '%s %s' % (outpath,args) return cmd def exec_commands(conn,cmd): 'this is use the conn to excute the cmd and return the results of excute the command' stdin,stdout,stderr = conn.exec_command(cmd) results=stdout.read() return results def excutor(host,outpath,args): conn = connect(host) if not conn: return [host,None] #exec_commands(conn,'chmod +x %s' % outpath) cmd =command(args,outpath) result = exec_commands(conn,cmd) result = json.dumps(result) return [host,result]
def copy_module(conn,inpath,outpath): 'this is copy the module to the remote server' ftp = conn.open_sftp() ftp.put(inpath,outpath) ftp.close() return outpath if __name__ == '__main__': print json.dumps(excutor('192.168.1.165','ls',' -l'),indent=4,sort_keys=True) print copy_module(connect('192.168.1.165'),'kel.txt','/root/kel.1.txt') exec_commands(connect('192.168.1.165'),'chmod +x %s' % '/root/kel.1.txt')
주로 Python에서 paramiko 모듈을 사용하여 ssh를 통해 Linux 서버에 접속한 후 해당 명령어를 실행하고 파일을 서버에 업로드합니다.
위 내용은 paramiko를 사용하여 원격 서버에 연결하고 명령을 실행하는 Python용 샘플 코드의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
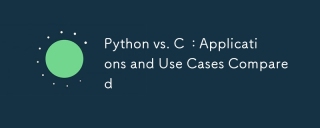
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
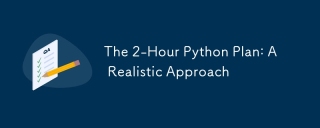
2 시간 이내에 Python의 기본 프로그래밍 개념과 기술을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우기, 2. 마스터 제어 흐름 (조건부 명세서 및 루프), 3. 기능의 정의 및 사용을 이해하십시오. 4. 간단한 예제 및 코드 스 니펫을 통해 Python 프로그래밍을 신속하게 시작하십시오.
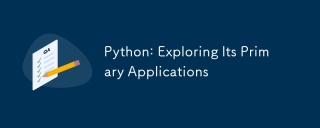
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
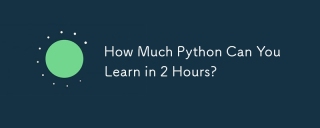
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
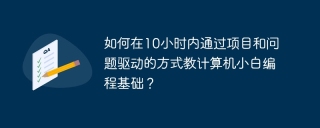
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
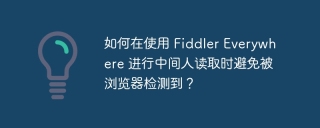
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...
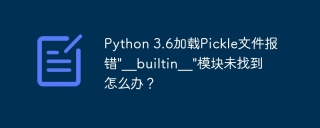
Python 3.6에 피클 파일로드 3.6 환경 보고서 오류 : modulenotfounderror : nomodulename ...
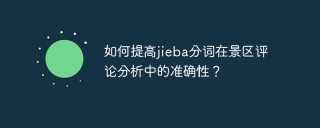
경치 좋은 스팟 댓글 분석에서 Jieba Word 세분화 문제를 해결하는 방법은 무엇입니까? 경치가 좋은 스팟 댓글 및 분석을 수행 할 때 종종 Jieba Word 세분화 도구를 사용하여 텍스트를 처리합니다 ...


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
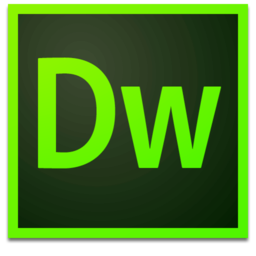
Dreamweaver Mac版
시각적 웹 개발 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전
