게임 전체가 게임 루프에서 진행되기 때문에 게임 루프는 게임의 핵심이라고 할 수 있습니다. 루프를 실행할 때마다 게임 개체의 속성이 업데이트되고 게임 요소가 그려집니다.
이전 리소스 로딩 기사에서 언급했듯이 리소스 로딩이 완료된 후 게임을 시작할 때 게임 루프가 시작됩니다. 이제 코드의 이 부분을 검토해 보겠습니다.
/** *图像加载完毕的处理程序 **/ var imgLoad=function(self){ return function(){ self.loadedCount+=1; self.loadedImgs[this.srcPath]=this; this.onLoad=null; //保证图片的onLoad执行一次后销毁 self.loadedPercent=Math.floor(self.loadedCount/self.sum*100); self.onLoad&&self.onLoad(self.loadedPercent); if(self.loadedPercent===100){ self.loadedCount=0; self.loadedPercent=0; loadingImgs={}; if(self.gameObj&&self.gameObj.initialize){ self.gameObj.initialize(); if(cg.loop&&!cg.loop.stop){//结束上一个循环 cg.loop.end(); } cg.loop=new cg.GameLoop(self.gameObj);//开始新游戏循环 cg.loop.start(); } } } }
이미지 리소스가 로드된 후 게임 개체의 초기화 메서드를 호출하고 게임 루프 개체가 존재하는지 확인합니다. 존재하는 경우 이전 루프를 종료합니다(이 상황은 일반적으로 레벨을 전환하고 새 게임 개체를 전달할 때 발생합니다). 그렇지 않으면 게임 루프가 시작됩니다.
좋습니다. 이제 게임 루프의 구현 코드를 살펴보겠습니다.
var gameLoop=function(gameObj,options){ if(!(this instanceof arguments.callee)){ return new arguments.callee(gameObj,options); } this.init(gameObj,options); }
우선 게임 루프 함수 도 이를 기반으로 하는지 확인해야 합니다. 생성자 에서 호출 후 개체를 초기화합니다.
/** *初始化 **/ init:function(gameObj,options){ /** *默认对象 **/ var defaultObj={ fps:30 }; options=options||{}; options=cg.core.extend(defaultObj,options); this.gameObj=gameObj; this.fps=options.fps; interval=1000/this.fps; this.pause=false; this.stop=true; },
사용자가 설정해야 하는 매개변수는 fps(초당 프레임 수) 단 하나뿐입니다. 이 매개변수는 초당 실행되는 프레임 수입니다. 이 매개변수에 따라 게임 루프를 실행하는 데 몇 밀리초가 소요되는지 계산할 수 있습니다(간격 매개변수). 또한 루프는 일시 중지와 중지의 두 가지 모드를 지원합니다.
/** *开始循环 **/ start:function(){ if(this.stop){ //如果是结束状态则可以开始 this.stop=false; this.now=new Date().getTime(); this.startTime=new Date().getTime(); this.duration=0; loop.call(this)(); } },
루프가 시작되면 시작 시간을 저장하여 루프 지속 시간을 지속적으로 업데이트할 수 있습니다. 그런 다음 루프 함수를 호출하여 루프를 구현합니다.
var timeId; var interval; /** *循环方法 **/ var loop=function(){ var self=this; return function(){ if(!self.pause&&!self.stop){ self.now=new Date().getTime(); self.duration=self.startTime-self.now; if(self.gameObj.update){ self.gameObj.update(); } if(self.gameObj.draw){ cg.context.clearRect(0,0,cg.width,cg.height); self.gameObj.draw(); } } timeId=window.setTimeout(arguments.callee,interval); } }
일시 중지되거나 중지되지 않으면 게임 개체의 업데이트 및 그리기가 호출됩니다. (게임 개체의 업데이트는 레벨의 모든 요소 업데이트를 호출하는 역할을 담당하며, 무승부는 사실입니다). 그런 다음 setTimeout을재귀적으로호출하여 자신을 호출하여 루프를 구현합니다.
게임루프 전체 소스코드 :
/** * *游戏循环 * **/ cnGame.register("cnGame",function(cg){ var timeId; var interval; /** *循环方法 **/ var loop=function(){ var self=this; return function(){ if(!self.pause&&!self.stop){ self.now=new Date().getTime(); self.duration=self.startTime-self.now; if(self.gameObj.update){ self.gameObj.update(); } if(self.gameObj.draw){ cg.context.clearRect(0,0,cg.width,cg.height); self.gameObj.draw(); } } timeId=window.setTimeout(arguments.callee,interval); } } var gameLoop=function(gameObj,options){ if(!(this instanceof arguments.callee)){ return new arguments.callee(gameObj,options); } this.init(gameObj,options); } gameLoop.prototype={ /** *初始化 **/ init:function(gameObj,options){ /** *默认对象 **/ var defaultObj={ fps:30 }; options=options||{}; options=cg.core.extend(defaultObj,options); this.gameObj=gameObj; this.fps=options.fps; interval=1000/this.fps; this.pause=false; this.stop=true; }, /** *开始循环 **/ start:function(){ if(this.stop){ //如果是结束状态则可以开始 this.stop=false; this.now=new Date().getTime(); this.startTime=new Date().getTime(); this.duration=0; loop.call(this)(); } }, /** *继续循环 **/ run:function(){ this.pause=false; }, /** *暂停循环 **/ pause:function(){ this.pause=true; }, /** *停止循环 **/ end:function(){ this.stop=true; window.clearTimeout(timeId); } } this.GameLoop=gameLoop; });
위 내용은 HTML5 게임 프레임워크 cnGameJS 개발 기록 - 게임 루프의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

H5 및 HTML5는 다른 개념입니다. HTML5는 새로운 요소 및 API를 포함하는 HTML의 버전입니다. H5는 HTML5를 기반으로 한 모바일 애플리케이션 개발 프레임 워크입니다. HTML5는 브라우저를 통해 코드를 구문 분석하고 렌더링하는 반면 H5 응용 프로그램은 컨테이너를 실행하고 JavaScript를 통해 기본 코드와 상호 작용해야합니다.

HTML5의 주요 요소에는 최신 웹 페이지를 작성하는 데 사용되는 ,,,,, 등이 포함됩니다. 1. 헤드 컨텐츠 정의, 2. 링크를 탐색하는 데 사용됩니다. 3. 독립 기사의 내용을 나타내고, 4. 페이지 내용을 구성하고, 5. 사이드 바 컨텐츠 표시, 6. 바닥 글을 정의하면, 이러한 요소는 웹 페이지의 구조와 기능을 향상시킵니다.

HTML5와 H5 사이에는 차이가 없으며, 이는 HTML5의 약어입니다. 1.HTML5는 HTML의 다섯 번째 버전으로 웹 페이지의 멀티미디어 및 대화식 기능을 향상시킵니다. 2.H5는 종종 HTML5 기반 모바일 웹 페이지 또는 응용 프로그램을 참조하는 데 사용되며 다양한 모바일 장치에 적합합니다.

HTML5는 W3C에 의해 표준화 된 하이퍼 텍스트 마크 업 언어의 최신 버전입니다. HTML5는 새로운 시맨틱 태그, 멀티미디어 지원 및 양식 향상을 도입하여 웹 구조, 사용자 경험 및 SEO 효과를 개선합니다. HTML5는 웹 페이지 구조를 더 명확하게하고 SEO 효과를 더 좋게하기 위해, 등 등과 같은 새로운 시맨틱 태그를 소개합니다. HTML5는 멀티미디어 요소를 지원하며 타사 플러그인이 필요하지 않으므로 사용자 경험을 향상시키고 속도를로드합니다. HTML5는 양식 함수를 향상시키고 사용자 경험을 향상시키고 양식 검증 효율성을 향상시키는 새로운 입력 유형을 도입합니다.

깨끗하고 효율적인 HTML5 코드를 작성하는 방법은 무엇입니까? 답은 태그, 구조화 된 코드, 성능 최적화 및 일반적인 실수를 피함으로써 일반적인 실수를 피하는 것입니다. 1. 코드 가독성 및 SEO 효과를 향상시키기 위해 시맨틱 태그 등을 사용하십시오. 2. 적절한 계약과 의견을 사용하여 코드를 구성하고 읽을 수 있도록하십시오. 3. CDN 및 압축 코드를 사용하여 불필요한 태그를 줄임으로써 성능을 최적화합니다. 4. 태그가 닫히지 않은 것과 같은 일반적인 실수를 피하고 코드의 유효성을 확인하십시오.

H5는 멀티미디어 지원, 오프라인 스토리지 및 성능 최적화로 웹 사용자 경험을 향상시킵니다. 1) 멀티미디어 지원 : H5 및 요소는 개발을 단순화하고 사용자 경험을 향상시킵니다. 2) 오프라인 스토리지 : WebStorage 및 IndexedDB는 오프라인으로 사용하여 경험을 향상시킵니다. 3) 성능 최적화 : 웹 워즈 및 요소는 성능을 최적화하여 대역폭 소비를 줄입니다.

HTML5 코드는 태그, 요소 및 속성으로 구성됩니다. 1. 태그는 컨텐츠 유형을 정의하고 다음과 같은 각도 브래킷으로 둘러싸여 있습니다. 2. 요소는 컨텐츠와 같은 시작 태그, 내용 및 엔드 태그로 구성됩니다. 3. 속성 시작 태그에서 키 값 쌍을 정의하고 기능을 향상시킵니다. 웹 구조를 구축하기위한 기본 단위입니다.

HTML5는 현대적인 웹 페이지를 구축하는 핵심 기술로 많은 새로운 요소와 기능을 제공합니다. 1. HTML5는 웹 페이지 구조 및 SEO를 향상시키는 의미 론적 요소를 소개합니다. 2. 멀티미디어 요소를 지원하고 플러그인없이 미디어를 포함시킵니다. 3. 양식은 새로운 입력 유형 및 검증 속성을 향상시켜 검증 프로세스를 단순화합니다. 4. 웹 페이지 성능 및 사용자 경험을 향상시키기 위해 오프라인 및 로컬 스토리지 기능을 제공합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.
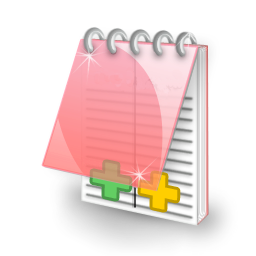
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음
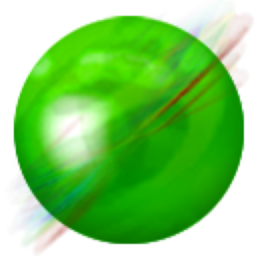
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
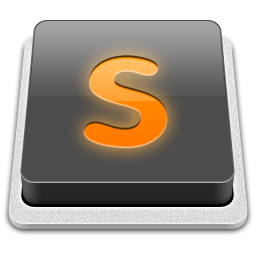
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
