배우기 시작하면서Python 자주 사용하는 "트릭"을 유지하기로 했습니다.” "멋지네요!"라고 생각하게 만드는 단락을 볼 때마다. ” 코드(예를 들어 StackOverflow, 오픈 소스 소프트웨어 등)를 이해할 때까지 시도해 본 다음 목록에 추가합니다. 이 게시물은 목록의 일부를 정리했습니다. 숙련된 Python 프로그래머라면 이미 일부를 알고 있을 수도 있지만 Python을 배우기 시작한 C, C++ 또는 Java 프로그래머라면 여전히 모르는 부분이 있을 수 있습니다. 🎜>프로그래밍하면 나처럼 매우 유용하다는 것을 알게 될 것입니다.
각각의 트릭이나 언어 기능은 너무 많은 설명 없이 예제를 통해서만 확인할 수 있습니다. 예는 명확하지만 익숙함에 따라 일부는 여전히 복잡해 보일 수 있으므로 예를 살펴본 후에도 명확하지 않은 경우 제목을 통해 Google을 통해 자세한 정보를 얻을 수 있습니다. 목록은 일반적으로 사용되는 언어 기능과 기술을 앞에 두고 난이도별로 정렬되어 있습니다. 1.15 단순 목록:>>> [3, 4], [5, 6]] >>>목록(itertools.chain.from_iterable(a))
[1, 2, 3, 4, 5, 6] >>> sum(a, []) [ 1, 2, 3, 4, 5, 6] >>> [x [1, 2, 3, 4, 5, 6] >>> a = [[[1, 2], [3, 4]] , [[5, 6], [7, 8]]] >>> [x for l1 in a for l2 in l1 for x in l2] [1 , 2, 3, 4, 5 , 6, 7, 8] >>> a = [1, 2, [3, 4], [[5, 6], [7, 8] ]] >>> flatten = 람다 x: [y for l in x for y in flatten(l)]if type( x) is list else [x]
>>> flatten(a) [1, 2, 3, 4, 5, 6, 7 , 8] 참고: Python 문서에 따르면 itertools.chain.from_iterable이 선호됩니다. 1.16 >>> g = (x ** 2 for x in x범위 (10))
>>다음(g)
0 >>> 1 >>> 다음(g) 4 >>> 다음(g) 9 >>> sum(x ** 3 for x in xrange(10)) 2025 >>> x ** 3 for x in xrange(10) if x % 3 == 1) 408 1.17 사전 반복 >>> x: x ** 2(5)} >>>m {0:0, 1:1, 2:4, 3:9, 4: 16} >>> m = {x: 'A' + str(x) for x in range(10)} > ;>>m {0: 'A0', 1: 'A1', 2: 'A2', 3: 'A3', 4: 'A4', 5: 'A5' , 6 : 'A6', 7: 'A7', 8: 'A8', 9: 'A9'} 1.18 사전을 반복하여 사전을 뒤집습니다 >>> = {'a': 1, 'b': 2, 'c': 3, 'd': 4} >>>m {'d': 4, 'a': 1, 'b': 2, 'c': 3} >>> {v: k for k, v in m.items()}{1: 'a', 2: 'b', 3: 'c', 4: 'd'} 1.19 명명된 시퀀스(collections.namedtuple)> ; >> Point = collections.namedtuple('Point', ['x', 'y']) >>> p = Point(x=1.0, y=2.0) >>>p 포인트(x=1.0, y=2.0) >>>p.x 1.0
>>> p.y 2.0 1.20
이름이 지정된 목록의 상속:
>>> classPoint(collections.namedtuple('PointBase', ['x', 'y'])): ... 슬롯 = ()
... def add(self, other):
... return Point(x=self.x + other.x, y=self.y + other. y)
...
>>> p = 점(x=1.0, y=2.0)
>>> q = 점(x=2.0, y=3.0)
>>> p + q
점(x=3.0, y=5.0)
1.21 集合及集합操작
>>> A = {1, 2, 3, 3}
>>> A
세트([1, 2, 3])
>>> B = {3, 4, 5, 6, 7}
>>> B
세트([3, 4, 5, 6, 7])
>>> A | B
set([1, 2, 3, 4, 5, 6, 7])
>>> A & B
세트([3])
>>> A - B
set([1, 2])
>>> B - A
세트([4, 5, 6, 7])
>>> A ^ B
set([1, 2, 4, 5, 6, 7])
>>> (A ^ B) == ((A - B) | (B - A))
참
1.22 多중集及其操작(컬렉션.개수er )
>>> A = collections.Counter([1, 2, 2])
>>> B = collections.Counter([2, 2, 3])
>>> A
카운터({2:2, 1:1})
>>> B
카운터({2:2, 3:1})
>>> A | B
카운터({2:2, 1:1, 3:1})
>>> A & B
카운터({2: 2})
>>> A + B
카운터({2:4, 1:1, 3:1})
>>> A - B
카운터({1: 1})
>>> B - A
카운터({3: 1})
1.23 迭代中最常见的元素 (collections.Counter)
>>> A = collections.Counter([1, 1, 2, 2, 3, 3, 3, 3, 4, 5, 6, 7])
>>> A
카운터({3:4, 1:2, 2:2, 4:1, 5:1, 6:1, 7:1})
>>> ; A.most_common(1)
[(3, 4)]
>>> A.most_common(3)
[(3, 4), (1, 2), (2, 2)]
1.24 双端队列 (컬렉션. 데크)
>>> Q = collections.deque()
>>> Q.app종료(1)
>>> Q.추가왼쪽(2)
>>> Q.extend([3, 4])
>>> Q.extendleft([5, 6])
>>> Q
deque([6, 5, 2, 1, 3, 4])
>>> Q.pop()
4
>>> Q.popleft()
6
>>> Q
deque([5, 2, 1, 3])
>>> Q.rotate(3)
>>> Q
deque([2, 1, 3, 5])
>>> Q.rotate(-3)
>>> Q
deque([5, 2, 1, 3])
1.25 有最大长島的双端队列 (collections.deque)
>>> last_ three = collections.deque(maxlen=3)
>>> for i in xrange(10):
... last_ three.append(i)
... 인쇄 ', '.join(str(x) for x의 마지막_3)
...
0
0, 1
0, 1, 2
1, 2, 3
2, 3, 4
3, 4, 5
4, 5, 6
5, 6, 7
6, 7, 8
7, 8, 9
1.26 字典排序(컬렉션.OrderedDict)
>>> m = dict((str(x), x) for x in range(10))
>>> print ', '.join(m.keys())
1, 0, 3, 2, 5, 4, 7, 6, 9, 8
>>>> m = collections.OrderedDict((str(x), x) for x in range(10))
>>> print ', '.join(m.keys())
0, 1, 2, 3, 4, 5, 6, 7, 8, 9
>>> m = collections.OrderedDict((str(x), x) for x in range(10, 0, -1))
>>> print ', '.join(m.keys())
10, 9, 8, 7, 6, 5, 4, 3, 2, 1
1.27 缺省字典(컬렉션 .defaultdict)
>>> m = dict()
>>> m['a']
추적(최근 c모두 마지막):
파일 "
KeyError: 'a'
>>>
>>> m = collections.defaultdict(int)
>>> m['a']
0
>>> m['b']
0
>>> m = collections.defaultdict(str)
>>> m['a']
''
>>> m['b'] += 'a'
>>> m['b']
'a'
>>> m = collections.defaultdict(lambda: '[기본값]')
>>> m['a']
'[기본값]'
>>> m['b']
'[기본값]'
1.28 用缺省字典表示简单的树
>>> import json
>>> tree = 람다: collections.defaultdict(tree)
>>> root = tree()
>>> root['menu']['id'] = '파일'
>>> root['menu']['value'] = '파일'
>>> root['menu']['menuitems']['new']['value'] = '새로 만들기'
>>> root['menu']['menuitems']['new']['onclick'] = 'new();'
>>> root['menu']['menuitems']['open']['value'] = '열기'
>>> root['menu']['menuitems']['open']['onclick'] = 'open();'
>>> root['menu']['menuitems']['close']['value'] = '닫기'
>>> root['menu']['menuitems']['close']['onclick'] = 'close();'
>>> print json.dumps(root, sort_keys=True, indent=4, 구분 기호=(',', ': '))
{
"메뉴" : {
"id": "file",
"menuitems": {
"close": {
"onclick" : "닫기 ();",
"값": "닫기"
},
"새 항목": {
"onclick": "새로 만들기( );",
"값": "새로 만들기"
},
"열기": {
"onclick": "열기() ;",
"value": "열기"
}
},
"value": "파일"
}
}
(到https://gist.github.com/hrldcpr/2012250查看详情)
1.29 映射对象到唯一的序列数 (collections.defaultdict)
>>> itertools, 컬렉션 가져오기
>>> value_to_numeric_map = collections.defaultdict(itertools.count().next)
>>> value_to_numeric_map['a']
0
>>> value_to_numeric_map['b']
1
>>> value_to_numeric_map['c']
2
>>> value_to_numeric_map['a']
0
>>> value_to_numeric_map['b']
1
위 내용은 Python 언어의 꼭 봐야 할 기능과 기술 30가지(2)의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
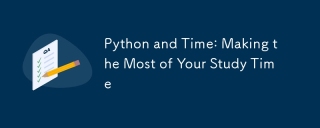
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.
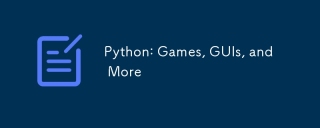
Python은 게임 및 GUI 개발에서 탁월합니다. 1) 게임 개발은 Pygame을 사용하여 드로잉, 오디오 및 기타 기능을 제공하며 2D 게임을 만드는 데 적합합니다. 2) GUI 개발은 Tkinter 또는 PYQT를 선택할 수 있습니다. Tkinter는 간단하고 사용하기 쉽고 PYQT는 풍부한 기능을 가지고 있으며 전문 개발에 적합합니다.
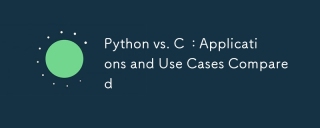
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
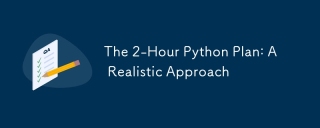
2 시간 이내에 Python의 기본 프로그래밍 개념과 기술을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우기, 2. 마스터 제어 흐름 (조건부 명세서 및 루프), 3. 기능의 정의 및 사용을 이해하십시오. 4. 간단한 예제 및 코드 스 니펫을 통해 Python 프로그래밍을 신속하게 시작하십시오.
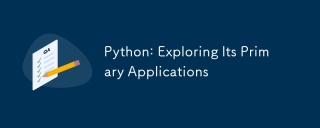
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
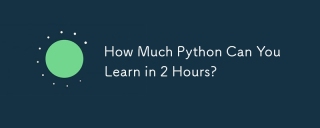
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
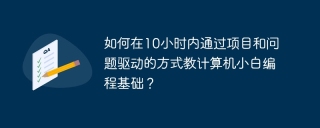
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
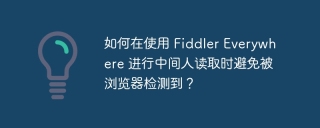
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
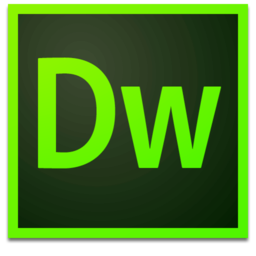
Dreamweaver Mac版
시각적 웹 개발 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
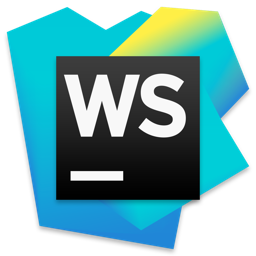
WebStorm Mac 버전
유용한 JavaScript 개발 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
