이것은 HTML5 및 JavaScript를 기반으로 하는 진행률 표시줄 애플리케이션으로, 진행률이 매우 독특할 때 표시됩니다. 입자 애니메이션 효과, 즉 진행률 표시줄이 미끄러지는 동안 일부 작은 입자가 떨어지며 효과가 매우 멋집니다. 또 다른 특징은 진행률이 변경됨에 따라 진행률 표시줄의 색상도 변경된다는 것입니다.
온라인 데모 소스 코드 다운로드
JavaScript 코드
/*========================================================*/ /* Light Loader /*========================================================*/ var lightLoader = function(c, cw, ch){ var _this = this; this.c = c; this.ctx = c.getContext('2d'); this.cw = cw; this.ch = ch; this.loaded = 0; this.loaderSpeed = .6; this.loaderHeight = 10; this.loaderWidth = 310; this.loader = { x: (this.cw/2) - (this.loaderWidth/2), y: (this.ch/2) - (this.loaderHeight/2) }; this.particles = []; this.particleLift = 180; this.hueStart = 0 this.hueEnd = 120; this.hue = 0; this.gravity = .15; this.particleRate = 4; /*========================================================*/ /* Initialize /*========================================================*/ this.init = function(){ this.loop(); }; /*========================================================*/ /* Utility Functions /*========================================================*/ this.rand = function(rMi, rMa){return ~~((Math.random()*(rMa-rMi+1))+rMi);}; this.hitTest = function(x1, y1, w1, h1, x2, y2, w2, h2){return !(x1 + w1 < x2 || x2 + w2 < x1 || y1 + h1 < y2 || y2 + h2 < y1);}; /*========================================================*/ /* Update Loader /*========================================================*/ this.updateLoader = function(){ if(this.loaded < 100){ this.loaded += this.loaderSpeed; } else { this.loaded = 0; } }; /*========================================================*/ /* Render Loader /*========================================================*/ this.renderLoader = function(){ this.ctx.fillStyle = '#000'; this.ctx.fillRect(this.loader.x, this.loader.y, this.loaderWidth, this.loaderHeight); this.hue = this.hueStart + (this.loaded/100)*(this.hueEnd - this.hueStart); var newWidth = (this.loaded/100)*this.loaderWidth; this.ctx.fillStyle = 'hsla('+this.hue+', 100%, 40%, 1)'; this.ctx.fillRect(this.loader.x, this.loader.y, newWidth, this.loaderHeight); this.ctx.fillStyle = '#222'; this.ctx.fillRect(this.loader.x, this.loader.y, newWidth, this.loaderHeight/2); }; /*========================================================*/ /* Particles /*========================================================*/ this.Particle = function(){ this.x = _this.loader.x + ((_this.loaded/100)*_this.loaderWidth) - _this.rand(0, 1); this.y = _this.ch/2 + _this.rand(0,_this.loaderHeight)-_this.loaderHeight/2; this.vx = (_this.rand(0,4)-2)/100; this.vy = (_this.rand(0,_this.particleLift)-_this.particleLift*2)/100; this.width = _this.rand(1,4)/2; this.height = _this.rand(1,4)/2; this.hue = _this.hue; }; this.Particle.prototype.update = function(i){ this.vx += (_this.rand(0,6)-3)/100; this.vy += _this.gravity; this.x += this.vx; this.y += this.vy; if(this.y > _this.ch){ _this.particles.splice(i, 1); } }; this.Particle.prototype.render = function(){ _this.ctx.fillStyle = 'hsla('+this.hue+', 100%, '+_this.rand(50,70)+'%, '+_this.rand(20,100)/100+')'; _this.ctx.fillRect(this.x, this.y, this.width, this.height); }; this.createParticles = function(){ var i = this.particleRate; while(i--){ this.particles.push(new this.Particle()); }; }; this.updateParticles = function(){ var i = this.particles.length; while(i--){ var p = this.particles[i]; p.update(i); }; }; this.renderParticles = function(){ var i = this.particles.length; while(i--){ var p = this.particles[i]; p.render(); }; }; /*========================================================*/ /* Clear Canvas /*========================================================*/ this.clearCanvas = function(){ this.ctx.globalCompositeOperation = 'source-over'; this.ctx.clearRect(0,0,this.cw,this.ch); this.ctx.globalCompositeOperation = 'lighter'; }; /*========================================================*/ /* Animation Loop /*========================================================*/ this.loop = function(){ var loopIt = function(){ requestAnimationFrame(loopIt, _this.c); _this.clearCanvas(); _this.createParticles(); _this.updateLoader(); _this.updateParticles(); _this.renderLoader(); _this.renderParticles(); }; loopIt(); }; }; /*========================================================*/ /* Check Canvas Support /*========================================================*/ var isCanvasSupported = function(){ var elem = document.createElement('canvas'); return !!(elem.getContext && elem.getContext('2d')); }; /*========================================================*/ /* Setup requestAnimationFrame /*========================================================*/ var setupRAF = function(){ var lastTime = 0; var vendors = ['ms', 'moz', 'webkit', 'o']; for(var x = 0; x < vendors.length && !window.requestAnimationFrame; ++x){ window.requestAnimationFrame = window[vendors[x]+'RequestAnimationFrame']; window.cancelAnimationFrame = window[vendors[x]+'CancelAnimationFrame'] || window[vendors[x]+'CancelRequestAnimationFrame']; }; if(!window.requestAnimationFrame){ window.requestAnimationFrame = function(callback, element){ var currTime = new Date().getTime(); var timeToCall = Math.max(0, 16 - (currTime - lastTime)); var id = window.setTimeout(function() { callback(currTime + timeToCall); }, timeToCall); lastTime = currTime + timeToCall; return id; }; }; if (!window.cancelAnimationFrame){ window.cancelAnimationFrame = function(id){ clearTimeout(id); }; }; }; /*========================================================*/ /* Define Canvas and Initialize /*========================================================*/ if(isCanvasSupported){ var c = document.createElement('canvas'); c.width = 400; c.height = 100; var cw = c.width; var ch = c.height; document.body.appendChild(c); var cl = new lightLoader(c, cw, ch); setupRAF(); cl.init(); }
위는 HTML5 super의 진행 표시줄 그래픽과 텍스트입니다. 멋진 입자 효과 코드에 대한 자세한 소개는 PHP 중국어 웹사이트(www.php.cn)에 더 많은 관련 내용이 있으니 참고해주세요!

HTML5의 핵심 기능에는 시맨틱 태그, 멀티미디어 지원, 양식 향상, 오프라인 스토리지 및 로컬 스토리지가 포함됩니다. 1. 코드 가독성 및 SEO 효과 향상과 같은 시맨틱 태그. 2. 멀티미디어 지원은 미디어 컨텐츠 및 태그를 포함하는 프로세스를 단순화합니다. 3. 양식 향상은 새로운 입력 유형 및 검증 특성을 도입하여 양식 개발을 단순화합니다. 4. 오프라인 스토리지 및 로컬 스토리지는 ApplicationCache 및 LocalStorage를 통해 웹 페이지 성능 및 사용자 경험을 향상시킵니다.

html5isamajorrevisionof thehtml thatrevolutions webdevelopments and capabilitiess.1) itenhancescodereadabilitys 및 and .2) html5enablestriCher, Interactive Experiences, Withoutplugs를 허용합니다

H5에 대한 고급 팁에는 다음이 포함됩니다. 1. 복잡한 그래픽 사용, 2. 웹 워크를 사용하여 성능 향상, 3. WebStorage, 4. 응답 디자인 구현, 5. WebRTC를 사용하여 실시간 커뮤니케이션을 달성하기 위해, 6. 성능 최적화 및 모범 사례를 수행하십시오. 이 팁은 개발자가보다 역동적이고 대화식 및 효율적인 웹 응용 프로그램을 구축 할 수 있도록 도와줍니다.

H5 (HTML5)는 새로운 요소와 API를 통해 웹 컨텐츠와 디자인을 개선합니다. 1) H5는 시맨틱 태깅 및 멀티미디어 지원을 향상시킵니다. 2) 웹 디자인을 풍부하게하는 캔버스 및 SVG를 소개합니다. 3) H5는 새로운 태그와 API를 통해 HTML 기능을 확장하여 작동합니다. 4) 기본 사용에는이를 사용하여 그래픽 생성이 포함되며, 고급 사용량은 WebStorageapi와 관련이 있습니다. 5) 개발자는 브라우저 호환성 및 성능 최적화에주의를 기울여야합니다.

H5는 여러 가지 새로운 기능과 기능을 제공하여 웹 페이지의 상호 작용 및 개발 효율성을 크게 향상시킵니다. 1. Enhance SEO와 같은 시맨틱 태그. 2. 멀티미디어 지원은 오디오 및 비디오 재생 및 태그를 단순화합니다. 3. 캔버스 드로잉은 역동적 인 그래픽 드로잉 도구를 제공합니다. 4. 로컬 스토리지는 LocalStorage 및 SessionStorage를 통해 데이터 스토리지를 단순화합니다. 5. Geolocation API는 위치 기반 서비스의 개발을 용이하게합니다.

HTML5는 5 가지 주요 개선 사항을 제공합니다. 1. 시맨틱 태그는 코드 선명도 및 SEO 효과를 향상시킵니다. 2. 멀티미디어 지원은 비디오 및 오디오 임베딩을 단순화합니다. 3. 형태 향상은 검증을 단순화한다. 4. 오프라인 및 로컬 스토리지는 사용자 경험을 향상시킵니다. 5. 캔버스 및 그래픽 기능은 웹 페이지의 시각화를 향상시킵니다.

HTML5의 핵심 기능에는 시맨틱 태그, 멀티미디어 지원, 오프라인 저장 및 로컬 스토리지 및 형태 향상이 포함됩니다. 1. 코드 가독성 및 SEO 효과를 향상시키는 시맨틱 태그 등. 2. 레이블로 멀티미디어 임베딩을 단순화하십시오. 3. ApplicationCache 및 LocalStorage와 같은 오프라인 스토리지 및 로컬 스토리지는 네트워크없는 작동 및 데이터 저장을 지원합니다. 4. 양식 향상은 처리 및 검증을 단순화하기 위해 새로운 입력 유형 및 검증 속성을 도입합니다.

H5는 다양한 새로운 기능과 기능을 제공하여 프론트 엔드 개발 기능을 크게 향상시킵니다. 1. 멀티미디어 지원 : 미디어를 포함하고 요소를 포함하여 플러그인이 필요하지 않습니다. 2. 캔버스 : 요소를 사용하여 2D 그래픽 및 애니메이션을 동적으로 렌더링합니다. 3. 로컬 스토리지 : LocalStorage 및 SessionStorage를 통해 지속적인 데이터 저장을 구현하여 사용자 경험을 향상시킵니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
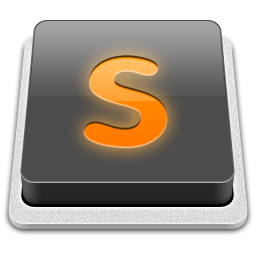
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
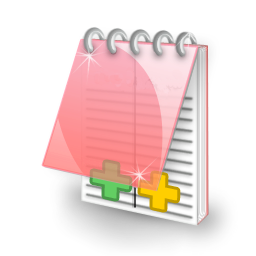
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음